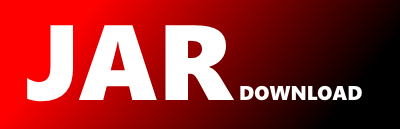
com.pulumi.azurenative.networkcloud.NetworkcloudFunctions Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.networkcloud;
import com.pulumi.azurenative.Utilities;
import com.pulumi.azurenative.networkcloud.inputs.GetAgentPoolArgs;
import com.pulumi.azurenative.networkcloud.inputs.GetAgentPoolPlainArgs;
import com.pulumi.azurenative.networkcloud.inputs.GetBareMetalMachineArgs;
import com.pulumi.azurenative.networkcloud.inputs.GetBareMetalMachineKeySetArgs;
import com.pulumi.azurenative.networkcloud.inputs.GetBareMetalMachineKeySetPlainArgs;
import com.pulumi.azurenative.networkcloud.inputs.GetBareMetalMachinePlainArgs;
import com.pulumi.azurenative.networkcloud.inputs.GetBmcKeySetArgs;
import com.pulumi.azurenative.networkcloud.inputs.GetBmcKeySetPlainArgs;
import com.pulumi.azurenative.networkcloud.inputs.GetCloudServicesNetworkArgs;
import com.pulumi.azurenative.networkcloud.inputs.GetCloudServicesNetworkPlainArgs;
import com.pulumi.azurenative.networkcloud.inputs.GetClusterArgs;
import com.pulumi.azurenative.networkcloud.inputs.GetClusterManagerArgs;
import com.pulumi.azurenative.networkcloud.inputs.GetClusterManagerPlainArgs;
import com.pulumi.azurenative.networkcloud.inputs.GetClusterPlainArgs;
import com.pulumi.azurenative.networkcloud.inputs.GetConsoleArgs;
import com.pulumi.azurenative.networkcloud.inputs.GetConsolePlainArgs;
import com.pulumi.azurenative.networkcloud.inputs.GetKubernetesClusterArgs;
import com.pulumi.azurenative.networkcloud.inputs.GetKubernetesClusterFeatureArgs;
import com.pulumi.azurenative.networkcloud.inputs.GetKubernetesClusterFeaturePlainArgs;
import com.pulumi.azurenative.networkcloud.inputs.GetKubernetesClusterPlainArgs;
import com.pulumi.azurenative.networkcloud.inputs.GetL2NetworkArgs;
import com.pulumi.azurenative.networkcloud.inputs.GetL2NetworkPlainArgs;
import com.pulumi.azurenative.networkcloud.inputs.GetL3NetworkArgs;
import com.pulumi.azurenative.networkcloud.inputs.GetL3NetworkPlainArgs;
import com.pulumi.azurenative.networkcloud.inputs.GetMetricsConfigurationArgs;
import com.pulumi.azurenative.networkcloud.inputs.GetMetricsConfigurationPlainArgs;
import com.pulumi.azurenative.networkcloud.inputs.GetRackArgs;
import com.pulumi.azurenative.networkcloud.inputs.GetRackPlainArgs;
import com.pulumi.azurenative.networkcloud.inputs.GetStorageApplianceArgs;
import com.pulumi.azurenative.networkcloud.inputs.GetStorageAppliancePlainArgs;
import com.pulumi.azurenative.networkcloud.inputs.GetTrunkedNetworkArgs;
import com.pulumi.azurenative.networkcloud.inputs.GetTrunkedNetworkPlainArgs;
import com.pulumi.azurenative.networkcloud.inputs.GetVirtualMachineArgs;
import com.pulumi.azurenative.networkcloud.inputs.GetVirtualMachinePlainArgs;
import com.pulumi.azurenative.networkcloud.inputs.GetVolumeArgs;
import com.pulumi.azurenative.networkcloud.inputs.GetVolumePlainArgs;
import com.pulumi.azurenative.networkcloud.outputs.GetAgentPoolResult;
import com.pulumi.azurenative.networkcloud.outputs.GetBareMetalMachineKeySetResult;
import com.pulumi.azurenative.networkcloud.outputs.GetBareMetalMachineResult;
import com.pulumi.azurenative.networkcloud.outputs.GetBmcKeySetResult;
import com.pulumi.azurenative.networkcloud.outputs.GetCloudServicesNetworkResult;
import com.pulumi.azurenative.networkcloud.outputs.GetClusterManagerResult;
import com.pulumi.azurenative.networkcloud.outputs.GetClusterResult;
import com.pulumi.azurenative.networkcloud.outputs.GetConsoleResult;
import com.pulumi.azurenative.networkcloud.outputs.GetKubernetesClusterFeatureResult;
import com.pulumi.azurenative.networkcloud.outputs.GetKubernetesClusterResult;
import com.pulumi.azurenative.networkcloud.outputs.GetL2NetworkResult;
import com.pulumi.azurenative.networkcloud.outputs.GetL3NetworkResult;
import com.pulumi.azurenative.networkcloud.outputs.GetMetricsConfigurationResult;
import com.pulumi.azurenative.networkcloud.outputs.GetRackResult;
import com.pulumi.azurenative.networkcloud.outputs.GetStorageApplianceResult;
import com.pulumi.azurenative.networkcloud.outputs.GetTrunkedNetworkResult;
import com.pulumi.azurenative.networkcloud.outputs.GetVirtualMachineResult;
import com.pulumi.azurenative.networkcloud.outputs.GetVolumeResult;
import com.pulumi.core.Output;
import com.pulumi.core.TypeShape;
import com.pulumi.deployment.Deployment;
import com.pulumi.deployment.InvokeOptions;
import java.util.concurrent.CompletableFuture;
public final class NetworkcloudFunctions {
/**
* Get properties of the provided Kubernetes cluster agent pool.
* Azure REST API version: 2023-10-01-preview.
*
* Other available API versions: 2023-07-01, 2024-06-01-preview, 2024-07-01.
*
*/
public static Output getAgentPool(GetAgentPoolArgs args) {
return getAgentPool(args, InvokeOptions.Empty);
}
/**
* Get properties of the provided Kubernetes cluster agent pool.
* Azure REST API version: 2023-10-01-preview.
*
* Other available API versions: 2023-07-01, 2024-06-01-preview, 2024-07-01.
*
*/
public static CompletableFuture getAgentPoolPlain(GetAgentPoolPlainArgs args) {
return getAgentPoolPlain(args, InvokeOptions.Empty);
}
/**
* Get properties of the provided Kubernetes cluster agent pool.
* Azure REST API version: 2023-10-01-preview.
*
* Other available API versions: 2023-07-01, 2024-06-01-preview, 2024-07-01.
*
*/
public static Output getAgentPool(GetAgentPoolArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:networkcloud:getAgentPool", TypeShape.of(GetAgentPoolResult.class), args, Utilities.withVersion(options));
}
/**
* Get properties of the provided Kubernetes cluster agent pool.
* Azure REST API version: 2023-10-01-preview.
*
* Other available API versions: 2023-07-01, 2024-06-01-preview, 2024-07-01.
*
*/
public static CompletableFuture getAgentPoolPlain(GetAgentPoolPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:networkcloud:getAgentPool", TypeShape.of(GetAgentPoolResult.class), args, Utilities.withVersion(options));
}
/**
* Get properties of the provided bare metal machine.
* Azure REST API version: 2023-10-01-preview.
*
* Other available API versions: 2023-07-01, 2024-06-01-preview, 2024-07-01.
*
*/
public static Output getBareMetalMachine(GetBareMetalMachineArgs args) {
return getBareMetalMachine(args, InvokeOptions.Empty);
}
/**
* Get properties of the provided bare metal machine.
* Azure REST API version: 2023-10-01-preview.
*
* Other available API versions: 2023-07-01, 2024-06-01-preview, 2024-07-01.
*
*/
public static CompletableFuture getBareMetalMachinePlain(GetBareMetalMachinePlainArgs args) {
return getBareMetalMachinePlain(args, InvokeOptions.Empty);
}
/**
* Get properties of the provided bare metal machine.
* Azure REST API version: 2023-10-01-preview.
*
* Other available API versions: 2023-07-01, 2024-06-01-preview, 2024-07-01.
*
*/
public static Output getBareMetalMachine(GetBareMetalMachineArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:networkcloud:getBareMetalMachine", TypeShape.of(GetBareMetalMachineResult.class), args, Utilities.withVersion(options));
}
/**
* Get properties of the provided bare metal machine.
* Azure REST API version: 2023-10-01-preview.
*
* Other available API versions: 2023-07-01, 2024-06-01-preview, 2024-07-01.
*
*/
public static CompletableFuture getBareMetalMachinePlain(GetBareMetalMachinePlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:networkcloud:getBareMetalMachine", TypeShape.of(GetBareMetalMachineResult.class), args, Utilities.withVersion(options));
}
/**
* Get bare metal machine key set of the provided cluster.
* Azure REST API version: 2023-10-01-preview.
*
* Other available API versions: 2023-07-01, 2024-06-01-preview, 2024-07-01.
*
*/
public static Output getBareMetalMachineKeySet(GetBareMetalMachineKeySetArgs args) {
return getBareMetalMachineKeySet(args, InvokeOptions.Empty);
}
/**
* Get bare metal machine key set of the provided cluster.
* Azure REST API version: 2023-10-01-preview.
*
* Other available API versions: 2023-07-01, 2024-06-01-preview, 2024-07-01.
*
*/
public static CompletableFuture getBareMetalMachineKeySetPlain(GetBareMetalMachineKeySetPlainArgs args) {
return getBareMetalMachineKeySetPlain(args, InvokeOptions.Empty);
}
/**
* Get bare metal machine key set of the provided cluster.
* Azure REST API version: 2023-10-01-preview.
*
* Other available API versions: 2023-07-01, 2024-06-01-preview, 2024-07-01.
*
*/
public static Output getBareMetalMachineKeySet(GetBareMetalMachineKeySetArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:networkcloud:getBareMetalMachineKeySet", TypeShape.of(GetBareMetalMachineKeySetResult.class), args, Utilities.withVersion(options));
}
/**
* Get bare metal machine key set of the provided cluster.
* Azure REST API version: 2023-10-01-preview.
*
* Other available API versions: 2023-07-01, 2024-06-01-preview, 2024-07-01.
*
*/
public static CompletableFuture getBareMetalMachineKeySetPlain(GetBareMetalMachineKeySetPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:networkcloud:getBareMetalMachineKeySet", TypeShape.of(GetBareMetalMachineKeySetResult.class), args, Utilities.withVersion(options));
}
/**
* Get baseboard management controller key set of the provided cluster.
* Azure REST API version: 2023-10-01-preview.
*
* Other available API versions: 2023-07-01, 2024-06-01-preview, 2024-07-01.
*
*/
public static Output getBmcKeySet(GetBmcKeySetArgs args) {
return getBmcKeySet(args, InvokeOptions.Empty);
}
/**
* Get baseboard management controller key set of the provided cluster.
* Azure REST API version: 2023-10-01-preview.
*
* Other available API versions: 2023-07-01, 2024-06-01-preview, 2024-07-01.
*
*/
public static CompletableFuture getBmcKeySetPlain(GetBmcKeySetPlainArgs args) {
return getBmcKeySetPlain(args, InvokeOptions.Empty);
}
/**
* Get baseboard management controller key set of the provided cluster.
* Azure REST API version: 2023-10-01-preview.
*
* Other available API versions: 2023-07-01, 2024-06-01-preview, 2024-07-01.
*
*/
public static Output getBmcKeySet(GetBmcKeySetArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:networkcloud:getBmcKeySet", TypeShape.of(GetBmcKeySetResult.class), args, Utilities.withVersion(options));
}
/**
* Get baseboard management controller key set of the provided cluster.
* Azure REST API version: 2023-10-01-preview.
*
* Other available API versions: 2023-07-01, 2024-06-01-preview, 2024-07-01.
*
*/
public static CompletableFuture getBmcKeySetPlain(GetBmcKeySetPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:networkcloud:getBmcKeySet", TypeShape.of(GetBmcKeySetResult.class), args, Utilities.withVersion(options));
}
/**
* Get properties of the provided cloud services network.
* Azure REST API version: 2023-10-01-preview.
*
* Other available API versions: 2023-07-01, 2024-06-01-preview, 2024-07-01.
*
*/
public static Output getCloudServicesNetwork(GetCloudServicesNetworkArgs args) {
return getCloudServicesNetwork(args, InvokeOptions.Empty);
}
/**
* Get properties of the provided cloud services network.
* Azure REST API version: 2023-10-01-preview.
*
* Other available API versions: 2023-07-01, 2024-06-01-preview, 2024-07-01.
*
*/
public static CompletableFuture getCloudServicesNetworkPlain(GetCloudServicesNetworkPlainArgs args) {
return getCloudServicesNetworkPlain(args, InvokeOptions.Empty);
}
/**
* Get properties of the provided cloud services network.
* Azure REST API version: 2023-10-01-preview.
*
* Other available API versions: 2023-07-01, 2024-06-01-preview, 2024-07-01.
*
*/
public static Output getCloudServicesNetwork(GetCloudServicesNetworkArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:networkcloud:getCloudServicesNetwork", TypeShape.of(GetCloudServicesNetworkResult.class), args, Utilities.withVersion(options));
}
/**
* Get properties of the provided cloud services network.
* Azure REST API version: 2023-10-01-preview.
*
* Other available API versions: 2023-07-01, 2024-06-01-preview, 2024-07-01.
*
*/
public static CompletableFuture getCloudServicesNetworkPlain(GetCloudServicesNetworkPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:networkcloud:getCloudServicesNetwork", TypeShape.of(GetCloudServicesNetworkResult.class), args, Utilities.withVersion(options));
}
/**
* Get properties of the provided cluster.
* Azure REST API version: 2023-10-01-preview.
*
* Other available API versions: 2023-07-01, 2024-06-01-preview, 2024-07-01.
*
*/
public static Output getCluster(GetClusterArgs args) {
return getCluster(args, InvokeOptions.Empty);
}
/**
* Get properties of the provided cluster.
* Azure REST API version: 2023-10-01-preview.
*
* Other available API versions: 2023-07-01, 2024-06-01-preview, 2024-07-01.
*
*/
public static CompletableFuture getClusterPlain(GetClusterPlainArgs args) {
return getClusterPlain(args, InvokeOptions.Empty);
}
/**
* Get properties of the provided cluster.
* Azure REST API version: 2023-10-01-preview.
*
* Other available API versions: 2023-07-01, 2024-06-01-preview, 2024-07-01.
*
*/
public static Output getCluster(GetClusterArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:networkcloud:getCluster", TypeShape.of(GetClusterResult.class), args, Utilities.withVersion(options));
}
/**
* Get properties of the provided cluster.
* Azure REST API version: 2023-10-01-preview.
*
* Other available API versions: 2023-07-01, 2024-06-01-preview, 2024-07-01.
*
*/
public static CompletableFuture getClusterPlain(GetClusterPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:networkcloud:getCluster", TypeShape.of(GetClusterResult.class), args, Utilities.withVersion(options));
}
/**
* Get the properties of the provided cluster manager.
* Azure REST API version: 2023-10-01-preview.
*
* Other available API versions: 2023-07-01, 2024-06-01-preview, 2024-07-01.
*
*/
public static Output getClusterManager(GetClusterManagerArgs args) {
return getClusterManager(args, InvokeOptions.Empty);
}
/**
* Get the properties of the provided cluster manager.
* Azure REST API version: 2023-10-01-preview.
*
* Other available API versions: 2023-07-01, 2024-06-01-preview, 2024-07-01.
*
*/
public static CompletableFuture getClusterManagerPlain(GetClusterManagerPlainArgs args) {
return getClusterManagerPlain(args, InvokeOptions.Empty);
}
/**
* Get the properties of the provided cluster manager.
* Azure REST API version: 2023-10-01-preview.
*
* Other available API versions: 2023-07-01, 2024-06-01-preview, 2024-07-01.
*
*/
public static Output getClusterManager(GetClusterManagerArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:networkcloud:getClusterManager", TypeShape.of(GetClusterManagerResult.class), args, Utilities.withVersion(options));
}
/**
* Get the properties of the provided cluster manager.
* Azure REST API version: 2023-10-01-preview.
*
* Other available API versions: 2023-07-01, 2024-06-01-preview, 2024-07-01.
*
*/
public static CompletableFuture getClusterManagerPlain(GetClusterManagerPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:networkcloud:getClusterManager", TypeShape.of(GetClusterManagerResult.class), args, Utilities.withVersion(options));
}
/**
* Get properties of the provided virtual machine console.
* Azure REST API version: 2023-10-01-preview.
*
* Other available API versions: 2023-07-01, 2024-06-01-preview, 2024-07-01.
*
*/
public static Output getConsole(GetConsoleArgs args) {
return getConsole(args, InvokeOptions.Empty);
}
/**
* Get properties of the provided virtual machine console.
* Azure REST API version: 2023-10-01-preview.
*
* Other available API versions: 2023-07-01, 2024-06-01-preview, 2024-07-01.
*
*/
public static CompletableFuture getConsolePlain(GetConsolePlainArgs args) {
return getConsolePlain(args, InvokeOptions.Empty);
}
/**
* Get properties of the provided virtual machine console.
* Azure REST API version: 2023-10-01-preview.
*
* Other available API versions: 2023-07-01, 2024-06-01-preview, 2024-07-01.
*
*/
public static Output getConsole(GetConsoleArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:networkcloud:getConsole", TypeShape.of(GetConsoleResult.class), args, Utilities.withVersion(options));
}
/**
* Get properties of the provided virtual machine console.
* Azure REST API version: 2023-10-01-preview.
*
* Other available API versions: 2023-07-01, 2024-06-01-preview, 2024-07-01.
*
*/
public static CompletableFuture getConsolePlain(GetConsolePlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:networkcloud:getConsole", TypeShape.of(GetConsoleResult.class), args, Utilities.withVersion(options));
}
/**
* Get properties of the provided the Kubernetes cluster.
* Azure REST API version: 2023-10-01-preview.
*
* Other available API versions: 2023-07-01, 2024-06-01-preview, 2024-07-01.
*
*/
public static Output getKubernetesCluster(GetKubernetesClusterArgs args) {
return getKubernetesCluster(args, InvokeOptions.Empty);
}
/**
* Get properties of the provided the Kubernetes cluster.
* Azure REST API version: 2023-10-01-preview.
*
* Other available API versions: 2023-07-01, 2024-06-01-preview, 2024-07-01.
*
*/
public static CompletableFuture getKubernetesClusterPlain(GetKubernetesClusterPlainArgs args) {
return getKubernetesClusterPlain(args, InvokeOptions.Empty);
}
/**
* Get properties of the provided the Kubernetes cluster.
* Azure REST API version: 2023-10-01-preview.
*
* Other available API versions: 2023-07-01, 2024-06-01-preview, 2024-07-01.
*
*/
public static Output getKubernetesCluster(GetKubernetesClusterArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:networkcloud:getKubernetesCluster", TypeShape.of(GetKubernetesClusterResult.class), args, Utilities.withVersion(options));
}
/**
* Get properties of the provided the Kubernetes cluster.
* Azure REST API version: 2023-10-01-preview.
*
* Other available API versions: 2023-07-01, 2024-06-01-preview, 2024-07-01.
*
*/
public static CompletableFuture getKubernetesClusterPlain(GetKubernetesClusterPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:networkcloud:getKubernetesCluster", TypeShape.of(GetKubernetesClusterResult.class), args, Utilities.withVersion(options));
}
/**
* Get properties of the provided the Kubernetes cluster feature.
* Azure REST API version: 2024-06-01-preview.
*
* Other available API versions: 2024-07-01.
*
*/
public static Output getKubernetesClusterFeature(GetKubernetesClusterFeatureArgs args) {
return getKubernetesClusterFeature(args, InvokeOptions.Empty);
}
/**
* Get properties of the provided the Kubernetes cluster feature.
* Azure REST API version: 2024-06-01-preview.
*
* Other available API versions: 2024-07-01.
*
*/
public static CompletableFuture getKubernetesClusterFeaturePlain(GetKubernetesClusterFeaturePlainArgs args) {
return getKubernetesClusterFeaturePlain(args, InvokeOptions.Empty);
}
/**
* Get properties of the provided the Kubernetes cluster feature.
* Azure REST API version: 2024-06-01-preview.
*
* Other available API versions: 2024-07-01.
*
*/
public static Output getKubernetesClusterFeature(GetKubernetesClusterFeatureArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:networkcloud:getKubernetesClusterFeature", TypeShape.of(GetKubernetesClusterFeatureResult.class), args, Utilities.withVersion(options));
}
/**
* Get properties of the provided the Kubernetes cluster feature.
* Azure REST API version: 2024-06-01-preview.
*
* Other available API versions: 2024-07-01.
*
*/
public static CompletableFuture getKubernetesClusterFeaturePlain(GetKubernetesClusterFeaturePlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:networkcloud:getKubernetesClusterFeature", TypeShape.of(GetKubernetesClusterFeatureResult.class), args, Utilities.withVersion(options));
}
/**
* Get properties of the provided layer 2 (L2) network.
* Azure REST API version: 2023-10-01-preview.
*
* Other available API versions: 2023-07-01, 2024-06-01-preview, 2024-07-01.
*
*/
public static Output getL2Network(GetL2NetworkArgs args) {
return getL2Network(args, InvokeOptions.Empty);
}
/**
* Get properties of the provided layer 2 (L2) network.
* Azure REST API version: 2023-10-01-preview.
*
* Other available API versions: 2023-07-01, 2024-06-01-preview, 2024-07-01.
*
*/
public static CompletableFuture getL2NetworkPlain(GetL2NetworkPlainArgs args) {
return getL2NetworkPlain(args, InvokeOptions.Empty);
}
/**
* Get properties of the provided layer 2 (L2) network.
* Azure REST API version: 2023-10-01-preview.
*
* Other available API versions: 2023-07-01, 2024-06-01-preview, 2024-07-01.
*
*/
public static Output getL2Network(GetL2NetworkArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:networkcloud:getL2Network", TypeShape.of(GetL2NetworkResult.class), args, Utilities.withVersion(options));
}
/**
* Get properties of the provided layer 2 (L2) network.
* Azure REST API version: 2023-10-01-preview.
*
* Other available API versions: 2023-07-01, 2024-06-01-preview, 2024-07-01.
*
*/
public static CompletableFuture getL2NetworkPlain(GetL2NetworkPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:networkcloud:getL2Network", TypeShape.of(GetL2NetworkResult.class), args, Utilities.withVersion(options));
}
/**
* Get properties of the provided layer 3 (L3) network.
* Azure REST API version: 2023-10-01-preview.
*
* Other available API versions: 2023-07-01, 2024-06-01-preview, 2024-07-01.
*
*/
public static Output getL3Network(GetL3NetworkArgs args) {
return getL3Network(args, InvokeOptions.Empty);
}
/**
* Get properties of the provided layer 3 (L3) network.
* Azure REST API version: 2023-10-01-preview.
*
* Other available API versions: 2023-07-01, 2024-06-01-preview, 2024-07-01.
*
*/
public static CompletableFuture getL3NetworkPlain(GetL3NetworkPlainArgs args) {
return getL3NetworkPlain(args, InvokeOptions.Empty);
}
/**
* Get properties of the provided layer 3 (L3) network.
* Azure REST API version: 2023-10-01-preview.
*
* Other available API versions: 2023-07-01, 2024-06-01-preview, 2024-07-01.
*
*/
public static Output getL3Network(GetL3NetworkArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:networkcloud:getL3Network", TypeShape.of(GetL3NetworkResult.class), args, Utilities.withVersion(options));
}
/**
* Get properties of the provided layer 3 (L3) network.
* Azure REST API version: 2023-10-01-preview.
*
* Other available API versions: 2023-07-01, 2024-06-01-preview, 2024-07-01.
*
*/
public static CompletableFuture getL3NetworkPlain(GetL3NetworkPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:networkcloud:getL3Network", TypeShape.of(GetL3NetworkResult.class), args, Utilities.withVersion(options));
}
/**
* Get metrics configuration of the provided cluster.
* Azure REST API version: 2023-10-01-preview.
*
* Other available API versions: 2023-07-01, 2024-06-01-preview, 2024-07-01.
*
*/
public static Output getMetricsConfiguration(GetMetricsConfigurationArgs args) {
return getMetricsConfiguration(args, InvokeOptions.Empty);
}
/**
* Get metrics configuration of the provided cluster.
* Azure REST API version: 2023-10-01-preview.
*
* Other available API versions: 2023-07-01, 2024-06-01-preview, 2024-07-01.
*
*/
public static CompletableFuture getMetricsConfigurationPlain(GetMetricsConfigurationPlainArgs args) {
return getMetricsConfigurationPlain(args, InvokeOptions.Empty);
}
/**
* Get metrics configuration of the provided cluster.
* Azure REST API version: 2023-10-01-preview.
*
* Other available API versions: 2023-07-01, 2024-06-01-preview, 2024-07-01.
*
*/
public static Output getMetricsConfiguration(GetMetricsConfigurationArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:networkcloud:getMetricsConfiguration", TypeShape.of(GetMetricsConfigurationResult.class), args, Utilities.withVersion(options));
}
/**
* Get metrics configuration of the provided cluster.
* Azure REST API version: 2023-10-01-preview.
*
* Other available API versions: 2023-07-01, 2024-06-01-preview, 2024-07-01.
*
*/
public static CompletableFuture getMetricsConfigurationPlain(GetMetricsConfigurationPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:networkcloud:getMetricsConfiguration", TypeShape.of(GetMetricsConfigurationResult.class), args, Utilities.withVersion(options));
}
/**
* Get properties of the provided rack.
* Azure REST API version: 2023-10-01-preview.
*
* Other available API versions: 2023-07-01, 2024-06-01-preview, 2024-07-01.
*
*/
public static Output getRack(GetRackArgs args) {
return getRack(args, InvokeOptions.Empty);
}
/**
* Get properties of the provided rack.
* Azure REST API version: 2023-10-01-preview.
*
* Other available API versions: 2023-07-01, 2024-06-01-preview, 2024-07-01.
*
*/
public static CompletableFuture getRackPlain(GetRackPlainArgs args) {
return getRackPlain(args, InvokeOptions.Empty);
}
/**
* Get properties of the provided rack.
* Azure REST API version: 2023-10-01-preview.
*
* Other available API versions: 2023-07-01, 2024-06-01-preview, 2024-07-01.
*
*/
public static Output getRack(GetRackArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:networkcloud:getRack", TypeShape.of(GetRackResult.class), args, Utilities.withVersion(options));
}
/**
* Get properties of the provided rack.
* Azure REST API version: 2023-10-01-preview.
*
* Other available API versions: 2023-07-01, 2024-06-01-preview, 2024-07-01.
*
*/
public static CompletableFuture getRackPlain(GetRackPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:networkcloud:getRack", TypeShape.of(GetRackResult.class), args, Utilities.withVersion(options));
}
/**
* Get properties of the provided storage appliance.
* Azure REST API version: 2023-10-01-preview.
*
* Other available API versions: 2023-07-01, 2024-06-01-preview, 2024-07-01.
*
*/
public static Output getStorageAppliance(GetStorageApplianceArgs args) {
return getStorageAppliance(args, InvokeOptions.Empty);
}
/**
* Get properties of the provided storage appliance.
* Azure REST API version: 2023-10-01-preview.
*
* Other available API versions: 2023-07-01, 2024-06-01-preview, 2024-07-01.
*
*/
public static CompletableFuture getStorageAppliancePlain(GetStorageAppliancePlainArgs args) {
return getStorageAppliancePlain(args, InvokeOptions.Empty);
}
/**
* Get properties of the provided storage appliance.
* Azure REST API version: 2023-10-01-preview.
*
* Other available API versions: 2023-07-01, 2024-06-01-preview, 2024-07-01.
*
*/
public static Output getStorageAppliance(GetStorageApplianceArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:networkcloud:getStorageAppliance", TypeShape.of(GetStorageApplianceResult.class), args, Utilities.withVersion(options));
}
/**
* Get properties of the provided storage appliance.
* Azure REST API version: 2023-10-01-preview.
*
* Other available API versions: 2023-07-01, 2024-06-01-preview, 2024-07-01.
*
*/
public static CompletableFuture getStorageAppliancePlain(GetStorageAppliancePlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:networkcloud:getStorageAppliance", TypeShape.of(GetStorageApplianceResult.class), args, Utilities.withVersion(options));
}
/**
* Get properties of the provided trunked network.
* Azure REST API version: 2023-10-01-preview.
*
* Other available API versions: 2023-07-01, 2024-06-01-preview, 2024-07-01.
*
*/
public static Output getTrunkedNetwork(GetTrunkedNetworkArgs args) {
return getTrunkedNetwork(args, InvokeOptions.Empty);
}
/**
* Get properties of the provided trunked network.
* Azure REST API version: 2023-10-01-preview.
*
* Other available API versions: 2023-07-01, 2024-06-01-preview, 2024-07-01.
*
*/
public static CompletableFuture getTrunkedNetworkPlain(GetTrunkedNetworkPlainArgs args) {
return getTrunkedNetworkPlain(args, InvokeOptions.Empty);
}
/**
* Get properties of the provided trunked network.
* Azure REST API version: 2023-10-01-preview.
*
* Other available API versions: 2023-07-01, 2024-06-01-preview, 2024-07-01.
*
*/
public static Output getTrunkedNetwork(GetTrunkedNetworkArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:networkcloud:getTrunkedNetwork", TypeShape.of(GetTrunkedNetworkResult.class), args, Utilities.withVersion(options));
}
/**
* Get properties of the provided trunked network.
* Azure REST API version: 2023-10-01-preview.
*
* Other available API versions: 2023-07-01, 2024-06-01-preview, 2024-07-01.
*
*/
public static CompletableFuture getTrunkedNetworkPlain(GetTrunkedNetworkPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:networkcloud:getTrunkedNetwork", TypeShape.of(GetTrunkedNetworkResult.class), args, Utilities.withVersion(options));
}
/**
* Get properties of the provided virtual machine.
* Azure REST API version: 2023-10-01-preview.
*
* Other available API versions: 2023-07-01, 2024-06-01-preview, 2024-07-01.
*
*/
public static Output getVirtualMachine(GetVirtualMachineArgs args) {
return getVirtualMachine(args, InvokeOptions.Empty);
}
/**
* Get properties of the provided virtual machine.
* Azure REST API version: 2023-10-01-preview.
*
* Other available API versions: 2023-07-01, 2024-06-01-preview, 2024-07-01.
*
*/
public static CompletableFuture getVirtualMachinePlain(GetVirtualMachinePlainArgs args) {
return getVirtualMachinePlain(args, InvokeOptions.Empty);
}
/**
* Get properties of the provided virtual machine.
* Azure REST API version: 2023-10-01-preview.
*
* Other available API versions: 2023-07-01, 2024-06-01-preview, 2024-07-01.
*
*/
public static Output getVirtualMachine(GetVirtualMachineArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:networkcloud:getVirtualMachine", TypeShape.of(GetVirtualMachineResult.class), args, Utilities.withVersion(options));
}
/**
* Get properties of the provided virtual machine.
* Azure REST API version: 2023-10-01-preview.
*
* Other available API versions: 2023-07-01, 2024-06-01-preview, 2024-07-01.
*
*/
public static CompletableFuture getVirtualMachinePlain(GetVirtualMachinePlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:networkcloud:getVirtualMachine", TypeShape.of(GetVirtualMachineResult.class), args, Utilities.withVersion(options));
}
/**
* Get properties of the provided volume.
* Azure REST API version: 2023-10-01-preview.
*
* Other available API versions: 2023-07-01, 2024-06-01-preview, 2024-07-01.
*
*/
public static Output getVolume(GetVolumeArgs args) {
return getVolume(args, InvokeOptions.Empty);
}
/**
* Get properties of the provided volume.
* Azure REST API version: 2023-10-01-preview.
*
* Other available API versions: 2023-07-01, 2024-06-01-preview, 2024-07-01.
*
*/
public static CompletableFuture getVolumePlain(GetVolumePlainArgs args) {
return getVolumePlain(args, InvokeOptions.Empty);
}
/**
* Get properties of the provided volume.
* Azure REST API version: 2023-10-01-preview.
*
* Other available API versions: 2023-07-01, 2024-06-01-preview, 2024-07-01.
*
*/
public static Output getVolume(GetVolumeArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:networkcloud:getVolume", TypeShape.of(GetVolumeResult.class), args, Utilities.withVersion(options));
}
/**
* Get properties of the provided volume.
* Azure REST API version: 2023-10-01-preview.
*
* Other available API versions: 2023-07-01, 2024-06-01-preview, 2024-07-01.
*
*/
public static CompletableFuture getVolumePlain(GetVolumePlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:networkcloud:getVolume", TypeShape.of(GetVolumeResult.class), args, Utilities.withVersion(options));
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy