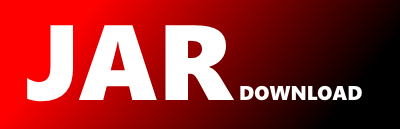
com.pulumi.azurenative.networkcloud.VirtualMachineArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.networkcloud;
import com.pulumi.azurenative.networkcloud.enums.VirtualMachineBootMethod;
import com.pulumi.azurenative.networkcloud.enums.VirtualMachineDeviceModelType;
import com.pulumi.azurenative.networkcloud.enums.VirtualMachineIsolateEmulatorThread;
import com.pulumi.azurenative.networkcloud.enums.VirtualMachineVirtioInterfaceType;
import com.pulumi.azurenative.networkcloud.inputs.ExtendedLocationArgs;
import com.pulumi.azurenative.networkcloud.inputs.ImageRepositoryCredentialsArgs;
import com.pulumi.azurenative.networkcloud.inputs.NetworkAttachmentArgs;
import com.pulumi.azurenative.networkcloud.inputs.SshPublicKeyArgs;
import com.pulumi.azurenative.networkcloud.inputs.StorageProfileArgs;
import com.pulumi.azurenative.networkcloud.inputs.VirtualMachinePlacementHintArgs;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.core.internal.Codegen;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Double;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class VirtualMachineArgs extends com.pulumi.resources.ResourceArgs {
public static final VirtualMachineArgs Empty = new VirtualMachineArgs();
/**
* The name of the administrator to which the ssh public keys will be added into the authorized keys.
*
*/
@Import(name="adminUsername", required=true)
private Output adminUsername;
/**
* @return The name of the administrator to which the ssh public keys will be added into the authorized keys.
*
*/
public Output adminUsername() {
return this.adminUsername;
}
/**
* Selects the boot method for the virtual machine.
*
*/
@Import(name="bootMethod")
private @Nullable Output> bootMethod;
/**
* @return Selects the boot method for the virtual machine.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy