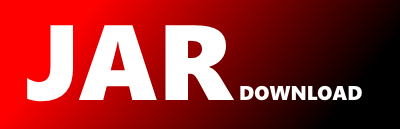
com.pulumi.azurenative.networkcloud.outputs.GetL2NetworkResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.networkcloud.outputs;
import com.pulumi.azurenative.networkcloud.outputs.ExtendedLocationResponse;
import com.pulumi.azurenative.networkcloud.outputs.SystemDataResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class GetL2NetworkResult {
/**
* @return The list of resource IDs for the other Microsoft.NetworkCloud resources that have attached this network.
*
*/
private List associatedResourceIds;
/**
* @return The resource ID of the Network Cloud cluster this L2 network is associated with.
*
*/
private String clusterId;
/**
* @return The more detailed status of the L2 network.
*
*/
private String detailedStatus;
/**
* @return The descriptive message about the current detailed status.
*
*/
private String detailedStatusMessage;
/**
* @return The extended location of the cluster associated with the resource.
*
*/
private ExtendedLocationResponse extendedLocation;
/**
* @return Field Deprecated. These fields will be empty/omitted. The list of Hybrid AKS cluster resource ID(s) that are associated with this L2 network.
*
*/
private List hybridAksClustersAssociatedIds;
/**
* @return Field Deprecated. The field was previously optional, now it will have no defined behavior and will be ignored. The network plugin type for Hybrid AKS.
*
*/
private @Nullable String hybridAksPluginType;
/**
* @return Fully qualified resource ID for the resource. E.g. "/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/{resourceProviderNamespace}/{resourceType}/{resourceName}"
*
*/
private String id;
/**
* @return The default interface name for this L2 network in the virtual machine. This name can be overridden by the name supplied in the network attachment configuration of that virtual machine.
*
*/
private @Nullable String interfaceName;
/**
* @return The resource ID of the Network Fabric l2IsolationDomain.
*
*/
private String l2IsolationDomainId;
/**
* @return The geo-location where the resource lives
*
*/
private String location;
/**
* @return The name of the resource
*
*/
private String name;
/**
* @return The provisioning state of the L2 network.
*
*/
private String provisioningState;
/**
* @return Azure Resource Manager metadata containing createdBy and modifiedBy information.
*
*/
private SystemDataResponse systemData;
/**
* @return Resource tags.
*
*/
private @Nullable Map tags;
/**
* @return The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
private String type;
/**
* @return Field Deprecated. These fields will be empty/omitted. The list of virtual machine resource ID(s), excluding any Hybrid AKS virtual machines, that are currently using this L2 network.
*
*/
private List virtualMachinesAssociatedIds;
private GetL2NetworkResult() {}
/**
* @return The list of resource IDs for the other Microsoft.NetworkCloud resources that have attached this network.
*
*/
public List associatedResourceIds() {
return this.associatedResourceIds;
}
/**
* @return The resource ID of the Network Cloud cluster this L2 network is associated with.
*
*/
public String clusterId() {
return this.clusterId;
}
/**
* @return The more detailed status of the L2 network.
*
*/
public String detailedStatus() {
return this.detailedStatus;
}
/**
* @return The descriptive message about the current detailed status.
*
*/
public String detailedStatusMessage() {
return this.detailedStatusMessage;
}
/**
* @return The extended location of the cluster associated with the resource.
*
*/
public ExtendedLocationResponse extendedLocation() {
return this.extendedLocation;
}
/**
* @return Field Deprecated. These fields will be empty/omitted. The list of Hybrid AKS cluster resource ID(s) that are associated with this L2 network.
*
*/
public List hybridAksClustersAssociatedIds() {
return this.hybridAksClustersAssociatedIds;
}
/**
* @return Field Deprecated. The field was previously optional, now it will have no defined behavior and will be ignored. The network plugin type for Hybrid AKS.
*
*/
public Optional hybridAksPluginType() {
return Optional.ofNullable(this.hybridAksPluginType);
}
/**
* @return Fully qualified resource ID for the resource. E.g. "/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/{resourceProviderNamespace}/{resourceType}/{resourceName}"
*
*/
public String id() {
return this.id;
}
/**
* @return The default interface name for this L2 network in the virtual machine. This name can be overridden by the name supplied in the network attachment configuration of that virtual machine.
*
*/
public Optional interfaceName() {
return Optional.ofNullable(this.interfaceName);
}
/**
* @return The resource ID of the Network Fabric l2IsolationDomain.
*
*/
public String l2IsolationDomainId() {
return this.l2IsolationDomainId;
}
/**
* @return The geo-location where the resource lives
*
*/
public String location() {
return this.location;
}
/**
* @return The name of the resource
*
*/
public String name() {
return this.name;
}
/**
* @return The provisioning state of the L2 network.
*
*/
public String provisioningState() {
return this.provisioningState;
}
/**
* @return Azure Resource Manager metadata containing createdBy and modifiedBy information.
*
*/
public SystemDataResponse systemData() {
return this.systemData;
}
/**
* @return Resource tags.
*
*/
public Map tags() {
return this.tags == null ? Map.of() : this.tags;
}
/**
* @return The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
public String type() {
return this.type;
}
/**
* @return Field Deprecated. These fields will be empty/omitted. The list of virtual machine resource ID(s), excluding any Hybrid AKS virtual machines, that are currently using this L2 network.
*
*/
public List virtualMachinesAssociatedIds() {
return this.virtualMachinesAssociatedIds;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetL2NetworkResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private List associatedResourceIds;
private String clusterId;
private String detailedStatus;
private String detailedStatusMessage;
private ExtendedLocationResponse extendedLocation;
private List hybridAksClustersAssociatedIds;
private @Nullable String hybridAksPluginType;
private String id;
private @Nullable String interfaceName;
private String l2IsolationDomainId;
private String location;
private String name;
private String provisioningState;
private SystemDataResponse systemData;
private @Nullable Map tags;
private String type;
private List virtualMachinesAssociatedIds;
public Builder() {}
public Builder(GetL2NetworkResult defaults) {
Objects.requireNonNull(defaults);
this.associatedResourceIds = defaults.associatedResourceIds;
this.clusterId = defaults.clusterId;
this.detailedStatus = defaults.detailedStatus;
this.detailedStatusMessage = defaults.detailedStatusMessage;
this.extendedLocation = defaults.extendedLocation;
this.hybridAksClustersAssociatedIds = defaults.hybridAksClustersAssociatedIds;
this.hybridAksPluginType = defaults.hybridAksPluginType;
this.id = defaults.id;
this.interfaceName = defaults.interfaceName;
this.l2IsolationDomainId = defaults.l2IsolationDomainId;
this.location = defaults.location;
this.name = defaults.name;
this.provisioningState = defaults.provisioningState;
this.systemData = defaults.systemData;
this.tags = defaults.tags;
this.type = defaults.type;
this.virtualMachinesAssociatedIds = defaults.virtualMachinesAssociatedIds;
}
@CustomType.Setter
public Builder associatedResourceIds(List associatedResourceIds) {
if (associatedResourceIds == null) {
throw new MissingRequiredPropertyException("GetL2NetworkResult", "associatedResourceIds");
}
this.associatedResourceIds = associatedResourceIds;
return this;
}
public Builder associatedResourceIds(String... associatedResourceIds) {
return associatedResourceIds(List.of(associatedResourceIds));
}
@CustomType.Setter
public Builder clusterId(String clusterId) {
if (clusterId == null) {
throw new MissingRequiredPropertyException("GetL2NetworkResult", "clusterId");
}
this.clusterId = clusterId;
return this;
}
@CustomType.Setter
public Builder detailedStatus(String detailedStatus) {
if (detailedStatus == null) {
throw new MissingRequiredPropertyException("GetL2NetworkResult", "detailedStatus");
}
this.detailedStatus = detailedStatus;
return this;
}
@CustomType.Setter
public Builder detailedStatusMessage(String detailedStatusMessage) {
if (detailedStatusMessage == null) {
throw new MissingRequiredPropertyException("GetL2NetworkResult", "detailedStatusMessage");
}
this.detailedStatusMessage = detailedStatusMessage;
return this;
}
@CustomType.Setter
public Builder extendedLocation(ExtendedLocationResponse extendedLocation) {
if (extendedLocation == null) {
throw new MissingRequiredPropertyException("GetL2NetworkResult", "extendedLocation");
}
this.extendedLocation = extendedLocation;
return this;
}
@CustomType.Setter
public Builder hybridAksClustersAssociatedIds(List hybridAksClustersAssociatedIds) {
if (hybridAksClustersAssociatedIds == null) {
throw new MissingRequiredPropertyException("GetL2NetworkResult", "hybridAksClustersAssociatedIds");
}
this.hybridAksClustersAssociatedIds = hybridAksClustersAssociatedIds;
return this;
}
public Builder hybridAksClustersAssociatedIds(String... hybridAksClustersAssociatedIds) {
return hybridAksClustersAssociatedIds(List.of(hybridAksClustersAssociatedIds));
}
@CustomType.Setter
public Builder hybridAksPluginType(@Nullable String hybridAksPluginType) {
this.hybridAksPluginType = hybridAksPluginType;
return this;
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetL2NetworkResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder interfaceName(@Nullable String interfaceName) {
this.interfaceName = interfaceName;
return this;
}
@CustomType.Setter
public Builder l2IsolationDomainId(String l2IsolationDomainId) {
if (l2IsolationDomainId == null) {
throw new MissingRequiredPropertyException("GetL2NetworkResult", "l2IsolationDomainId");
}
this.l2IsolationDomainId = l2IsolationDomainId;
return this;
}
@CustomType.Setter
public Builder location(String location) {
if (location == null) {
throw new MissingRequiredPropertyException("GetL2NetworkResult", "location");
}
this.location = location;
return this;
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("GetL2NetworkResult", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder provisioningState(String provisioningState) {
if (provisioningState == null) {
throw new MissingRequiredPropertyException("GetL2NetworkResult", "provisioningState");
}
this.provisioningState = provisioningState;
return this;
}
@CustomType.Setter
public Builder systemData(SystemDataResponse systemData) {
if (systemData == null) {
throw new MissingRequiredPropertyException("GetL2NetworkResult", "systemData");
}
this.systemData = systemData;
return this;
}
@CustomType.Setter
public Builder tags(@Nullable Map tags) {
this.tags = tags;
return this;
}
@CustomType.Setter
public Builder type(String type) {
if (type == null) {
throw new MissingRequiredPropertyException("GetL2NetworkResult", "type");
}
this.type = type;
return this;
}
@CustomType.Setter
public Builder virtualMachinesAssociatedIds(List virtualMachinesAssociatedIds) {
if (virtualMachinesAssociatedIds == null) {
throw new MissingRequiredPropertyException("GetL2NetworkResult", "virtualMachinesAssociatedIds");
}
this.virtualMachinesAssociatedIds = virtualMachinesAssociatedIds;
return this;
}
public Builder virtualMachinesAssociatedIds(String... virtualMachinesAssociatedIds) {
return virtualMachinesAssociatedIds(List.of(virtualMachinesAssociatedIds));
}
public GetL2NetworkResult build() {
final var _resultValue = new GetL2NetworkResult();
_resultValue.associatedResourceIds = associatedResourceIds;
_resultValue.clusterId = clusterId;
_resultValue.detailedStatus = detailedStatus;
_resultValue.detailedStatusMessage = detailedStatusMessage;
_resultValue.extendedLocation = extendedLocation;
_resultValue.hybridAksClustersAssociatedIds = hybridAksClustersAssociatedIds;
_resultValue.hybridAksPluginType = hybridAksPluginType;
_resultValue.id = id;
_resultValue.interfaceName = interfaceName;
_resultValue.l2IsolationDomainId = l2IsolationDomainId;
_resultValue.location = location;
_resultValue.name = name;
_resultValue.provisioningState = provisioningState;
_resultValue.systemData = systemData;
_resultValue.tags = tags;
_resultValue.type = type;
_resultValue.virtualMachinesAssociatedIds = virtualMachinesAssociatedIds;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy