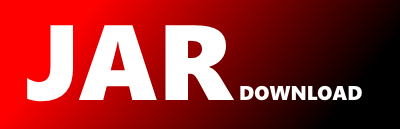
com.pulumi.azurenative.networkcloud.outputs.KubernetesClusterNodeResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.networkcloud.outputs;
import com.pulumi.azurenative.networkcloud.outputs.KubernetesLabelResponse;
import com.pulumi.azurenative.networkcloud.outputs.NetworkAttachmentResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Double;
import java.lang.String;
import java.util.List;
import java.util.Objects;
@CustomType
public final class KubernetesClusterNodeResponse {
/**
* @return The resource ID of the agent pool that this node belongs to. This value is not represented on control plane nodes.
*
*/
private String agentPoolId;
/**
* @return The availability zone this node is running within.
*
*/
private String availabilityZone;
/**
* @return The resource ID of the bare metal machine that hosts this node.
*
*/
private String bareMetalMachineId;
/**
* @return The number of CPU cores configured for this node, derived from the VM SKU specified.
*
*/
private Double cpuCores;
/**
* @return The detailed state of this node.
*
*/
private String detailedStatus;
/**
* @return The descriptive message about the current detailed status.
*
*/
private String detailedStatusMessage;
/**
* @return The size of the disk configured for this node.
*
*/
private Double diskSizeGB;
/**
* @return The machine image used to deploy this node.
*
*/
private String image;
/**
* @return The currently running version of Kubernetes and bundled features running on this node.
*
*/
private String kubernetesVersion;
/**
* @return The list of labels on this node that have been assigned to the agent pool containing this node.
*
*/
private List labels;
/**
* @return The amount of memory configured for this node, derived from the vm SKU specified.
*
*/
private Double memorySizeGB;
/**
* @return The mode of the agent pool containing this node. Not applicable for control plane nodes.
*
*/
private String mode;
/**
* @return The name of this node, as realized in the Kubernetes cluster.
*
*/
private String name;
/**
* @return The NetworkAttachments made to this node.
*
*/
private List networkAttachments;
/**
* @return The power state of this node.
*
*/
private String powerState;
/**
* @return The role of this node in the cluster.
*
*/
private String role;
/**
* @return The list of taints that have been assigned to the agent pool containing this node.
*
*/
private List taints;
/**
* @return The VM SKU name that was used to create this cluster node.
*
*/
private String vmSkuName;
private KubernetesClusterNodeResponse() {}
/**
* @return The resource ID of the agent pool that this node belongs to. This value is not represented on control plane nodes.
*
*/
public String agentPoolId() {
return this.agentPoolId;
}
/**
* @return The availability zone this node is running within.
*
*/
public String availabilityZone() {
return this.availabilityZone;
}
/**
* @return The resource ID of the bare metal machine that hosts this node.
*
*/
public String bareMetalMachineId() {
return this.bareMetalMachineId;
}
/**
* @return The number of CPU cores configured for this node, derived from the VM SKU specified.
*
*/
public Double cpuCores() {
return this.cpuCores;
}
/**
* @return The detailed state of this node.
*
*/
public String detailedStatus() {
return this.detailedStatus;
}
/**
* @return The descriptive message about the current detailed status.
*
*/
public String detailedStatusMessage() {
return this.detailedStatusMessage;
}
/**
* @return The size of the disk configured for this node.
*
*/
public Double diskSizeGB() {
return this.diskSizeGB;
}
/**
* @return The machine image used to deploy this node.
*
*/
public String image() {
return this.image;
}
/**
* @return The currently running version of Kubernetes and bundled features running on this node.
*
*/
public String kubernetesVersion() {
return this.kubernetesVersion;
}
/**
* @return The list of labels on this node that have been assigned to the agent pool containing this node.
*
*/
public List labels() {
return this.labels;
}
/**
* @return The amount of memory configured for this node, derived from the vm SKU specified.
*
*/
public Double memorySizeGB() {
return this.memorySizeGB;
}
/**
* @return The mode of the agent pool containing this node. Not applicable for control plane nodes.
*
*/
public String mode() {
return this.mode;
}
/**
* @return The name of this node, as realized in the Kubernetes cluster.
*
*/
public String name() {
return this.name;
}
/**
* @return The NetworkAttachments made to this node.
*
*/
public List networkAttachments() {
return this.networkAttachments;
}
/**
* @return The power state of this node.
*
*/
public String powerState() {
return this.powerState;
}
/**
* @return The role of this node in the cluster.
*
*/
public String role() {
return this.role;
}
/**
* @return The list of taints that have been assigned to the agent pool containing this node.
*
*/
public List taints() {
return this.taints;
}
/**
* @return The VM SKU name that was used to create this cluster node.
*
*/
public String vmSkuName() {
return this.vmSkuName;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(KubernetesClusterNodeResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String agentPoolId;
private String availabilityZone;
private String bareMetalMachineId;
private Double cpuCores;
private String detailedStatus;
private String detailedStatusMessage;
private Double diskSizeGB;
private String image;
private String kubernetesVersion;
private List labels;
private Double memorySizeGB;
private String mode;
private String name;
private List networkAttachments;
private String powerState;
private String role;
private List taints;
private String vmSkuName;
public Builder() {}
public Builder(KubernetesClusterNodeResponse defaults) {
Objects.requireNonNull(defaults);
this.agentPoolId = defaults.agentPoolId;
this.availabilityZone = defaults.availabilityZone;
this.bareMetalMachineId = defaults.bareMetalMachineId;
this.cpuCores = defaults.cpuCores;
this.detailedStatus = defaults.detailedStatus;
this.detailedStatusMessage = defaults.detailedStatusMessage;
this.diskSizeGB = defaults.diskSizeGB;
this.image = defaults.image;
this.kubernetesVersion = defaults.kubernetesVersion;
this.labels = defaults.labels;
this.memorySizeGB = defaults.memorySizeGB;
this.mode = defaults.mode;
this.name = defaults.name;
this.networkAttachments = defaults.networkAttachments;
this.powerState = defaults.powerState;
this.role = defaults.role;
this.taints = defaults.taints;
this.vmSkuName = defaults.vmSkuName;
}
@CustomType.Setter
public Builder agentPoolId(String agentPoolId) {
if (agentPoolId == null) {
throw new MissingRequiredPropertyException("KubernetesClusterNodeResponse", "agentPoolId");
}
this.agentPoolId = agentPoolId;
return this;
}
@CustomType.Setter
public Builder availabilityZone(String availabilityZone) {
if (availabilityZone == null) {
throw new MissingRequiredPropertyException("KubernetesClusterNodeResponse", "availabilityZone");
}
this.availabilityZone = availabilityZone;
return this;
}
@CustomType.Setter
public Builder bareMetalMachineId(String bareMetalMachineId) {
if (bareMetalMachineId == null) {
throw new MissingRequiredPropertyException("KubernetesClusterNodeResponse", "bareMetalMachineId");
}
this.bareMetalMachineId = bareMetalMachineId;
return this;
}
@CustomType.Setter
public Builder cpuCores(Double cpuCores) {
if (cpuCores == null) {
throw new MissingRequiredPropertyException("KubernetesClusterNodeResponse", "cpuCores");
}
this.cpuCores = cpuCores;
return this;
}
@CustomType.Setter
public Builder detailedStatus(String detailedStatus) {
if (detailedStatus == null) {
throw new MissingRequiredPropertyException("KubernetesClusterNodeResponse", "detailedStatus");
}
this.detailedStatus = detailedStatus;
return this;
}
@CustomType.Setter
public Builder detailedStatusMessage(String detailedStatusMessage) {
if (detailedStatusMessage == null) {
throw new MissingRequiredPropertyException("KubernetesClusterNodeResponse", "detailedStatusMessage");
}
this.detailedStatusMessage = detailedStatusMessage;
return this;
}
@CustomType.Setter
public Builder diskSizeGB(Double diskSizeGB) {
if (diskSizeGB == null) {
throw new MissingRequiredPropertyException("KubernetesClusterNodeResponse", "diskSizeGB");
}
this.diskSizeGB = diskSizeGB;
return this;
}
@CustomType.Setter
public Builder image(String image) {
if (image == null) {
throw new MissingRequiredPropertyException("KubernetesClusterNodeResponse", "image");
}
this.image = image;
return this;
}
@CustomType.Setter
public Builder kubernetesVersion(String kubernetesVersion) {
if (kubernetesVersion == null) {
throw new MissingRequiredPropertyException("KubernetesClusterNodeResponse", "kubernetesVersion");
}
this.kubernetesVersion = kubernetesVersion;
return this;
}
@CustomType.Setter
public Builder labels(List labels) {
if (labels == null) {
throw new MissingRequiredPropertyException("KubernetesClusterNodeResponse", "labels");
}
this.labels = labels;
return this;
}
public Builder labels(KubernetesLabelResponse... labels) {
return labels(List.of(labels));
}
@CustomType.Setter
public Builder memorySizeGB(Double memorySizeGB) {
if (memorySizeGB == null) {
throw new MissingRequiredPropertyException("KubernetesClusterNodeResponse", "memorySizeGB");
}
this.memorySizeGB = memorySizeGB;
return this;
}
@CustomType.Setter
public Builder mode(String mode) {
if (mode == null) {
throw new MissingRequiredPropertyException("KubernetesClusterNodeResponse", "mode");
}
this.mode = mode;
return this;
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("KubernetesClusterNodeResponse", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder networkAttachments(List networkAttachments) {
if (networkAttachments == null) {
throw new MissingRequiredPropertyException("KubernetesClusterNodeResponse", "networkAttachments");
}
this.networkAttachments = networkAttachments;
return this;
}
public Builder networkAttachments(NetworkAttachmentResponse... networkAttachments) {
return networkAttachments(List.of(networkAttachments));
}
@CustomType.Setter
public Builder powerState(String powerState) {
if (powerState == null) {
throw new MissingRequiredPropertyException("KubernetesClusterNodeResponse", "powerState");
}
this.powerState = powerState;
return this;
}
@CustomType.Setter
public Builder role(String role) {
if (role == null) {
throw new MissingRequiredPropertyException("KubernetesClusterNodeResponse", "role");
}
this.role = role;
return this;
}
@CustomType.Setter
public Builder taints(List taints) {
if (taints == null) {
throw new MissingRequiredPropertyException("KubernetesClusterNodeResponse", "taints");
}
this.taints = taints;
return this;
}
public Builder taints(KubernetesLabelResponse... taints) {
return taints(List.of(taints));
}
@CustomType.Setter
public Builder vmSkuName(String vmSkuName) {
if (vmSkuName == null) {
throw new MissingRequiredPropertyException("KubernetesClusterNodeResponse", "vmSkuName");
}
this.vmSkuName = vmSkuName;
return this;
}
public KubernetesClusterNodeResponse build() {
final var _resultValue = new KubernetesClusterNodeResponse();
_resultValue.agentPoolId = agentPoolId;
_resultValue.availabilityZone = availabilityZone;
_resultValue.bareMetalMachineId = bareMetalMachineId;
_resultValue.cpuCores = cpuCores;
_resultValue.detailedStatus = detailedStatus;
_resultValue.detailedStatusMessage = detailedStatusMessage;
_resultValue.diskSizeGB = diskSizeGB;
_resultValue.image = image;
_resultValue.kubernetesVersion = kubernetesVersion;
_resultValue.labels = labels;
_resultValue.memorySizeGB = memorySizeGB;
_resultValue.mode = mode;
_resultValue.name = name;
_resultValue.networkAttachments = networkAttachments;
_resultValue.powerState = powerState;
_resultValue.role = role;
_resultValue.taints = taints;
_resultValue.vmSkuName = vmSkuName;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy