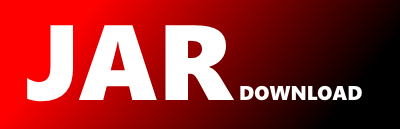
com.pulumi.azurenative.operationalinsights.ClusterArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.operationalinsights;
import com.pulumi.azurenative.operationalinsights.enums.BillingType;
import com.pulumi.azurenative.operationalinsights.inputs.ClusterSkuArgs;
import com.pulumi.azurenative.operationalinsights.inputs.IdentityArgs;
import com.pulumi.azurenative.operationalinsights.inputs.KeyVaultPropertiesArgs;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.String;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class ClusterArgs extends com.pulumi.resources.ResourceArgs {
public static final ClusterArgs Empty = new ClusterArgs();
/**
* The cluster's billing type.
*
*/
@Import(name="billingType")
private @Nullable Output> billingType;
/**
* @return The cluster's billing type.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy