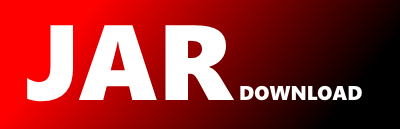
com.pulumi.azurenative.operationalinsights.MachineGroup Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.operationalinsights;
import com.pulumi.azurenative.Utilities;
import com.pulumi.azurenative.operationalinsights.MachineGroupArgs;
import com.pulumi.azurenative.operationalinsights.outputs.MachineReferenceWithHintsResponse;
import com.pulumi.core.Alias;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* A user-defined logical grouping of machines.
* Azure REST API version: 2015-11-01-preview. Prior API version in Azure Native 1.x: 2015-11-01-preview.
*
* ## Example Usage
* ### SMMachineGroupsUpdatePut
*
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.operationalinsights.MachineGroup;
* import com.pulumi.azurenative.operationalinsights.MachineGroupArgs;
* import com.pulumi.azurenative.operationalinsights.inputs.MachineReferenceWithHintsArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var machineGroup = new MachineGroup("machineGroup", MachineGroupArgs.builder()
* .count(1)
* .displayName("Foo")
* .kind("machineGroup")
* .machineGroupName("ccfbf4bf-dc08-4371-9e9b-00a8d875d45a")
* .machines(MachineReferenceWithHintsArgs.builder()
* .id("/subscriptions/63BE4E24-FDF0-4E9C-9342-6A5D5A359722/resourceGroups/rg-sm/providers/Microsoft.OperationalInsights/workspaces/D6F79F14-E563-469B-84B5-9286D2803B2F/machines/m-0fe4b501-7ac9-41d7-a4e1-1591a0789519")
* .kind("ref:machinewithhints")
* .build())
* .resourceGroupName("rg-sm")
* .workspaceName("D6F79F14-E563-469B-84B5-9286D2803B2F")
* .build());
*
* }
* }
*
* }
*
*
* ## Import
*
* An existing resource can be imported using its type token, name, and identifier, e.g.
*
* ```sh
* $ pulumi import azure-native:operationalinsights:MachineGroup ccfbf4bf-dc08-4371-9e9b-00a8d875d45a /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.OperationalInsights/workspaces/{workspaceName}/features/serviceMap/machineGroups/{machineGroupName}
* ```
*
*/
@ResourceType(type="azure-native:operationalinsights:MachineGroup")
public class MachineGroup extends com.pulumi.resources.CustomResource {
/**
* Count of machines in this group. The value of count may be bigger than the number of machines in case of the group has been truncated due to exceeding the max number of machines a group can handle.
*
*/
@Export(name="count", refs={Integer.class}, tree="[0]")
private Output* @Nullable */ Integer> count;
/**
* @return Count of machines in this group. The value of count may be bigger than the number of machines in case of the group has been truncated due to exceeding the max number of machines a group can handle.
*
*/
public Output> count() {
return Codegen.optional(this.count);
}
/**
* User defined name for the group
*
*/
@Export(name="displayName", refs={String.class}, tree="[0]")
private Output displayName;
/**
* @return User defined name for the group
*
*/
public Output displayName() {
return this.displayName;
}
/**
* Resource ETAG.
*
*/
@Export(name="etag", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> etag;
/**
* @return Resource ETAG.
*
*/
public Output> etag() {
return Codegen.optional(this.etag);
}
/**
* Type of the machine group
*
*/
@Export(name="groupType", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> groupType;
/**
* @return Type of the machine group
*
*/
public Output> groupType() {
return Codegen.optional(this.groupType);
}
/**
* Additional resource type qualifier.
* Expected value is 'machineGroup'.
*
*/
@Export(name="kind", refs={String.class}, tree="[0]")
private Output kind;
/**
* @return Additional resource type qualifier.
* Expected value is 'machineGroup'.
*
*/
public Output kind() {
return this.kind;
}
/**
* References of the machines in this group. The hints within each reference do not represent the current value of the corresponding fields. They are a snapshot created during the last time the machine group was updated.
*
*/
@Export(name="machines", refs={List.class,MachineReferenceWithHintsResponse.class}, tree="[0,1]")
private Output* @Nullable */ List> machines;
/**
* @return References of the machines in this group. The hints within each reference do not represent the current value of the corresponding fields. They are a snapshot created during the last time the machine group was updated.
*
*/
public Output>> machines() {
return Codegen.optional(this.machines);
}
/**
* Resource name.
*
*/
@Export(name="name", refs={String.class}, tree="[0]")
private Output name;
/**
* @return Resource name.
*
*/
public Output name() {
return this.name;
}
/**
* Resource type.
*
*/
@Export(name="type", refs={String.class}, tree="[0]")
private Output type;
/**
* @return Resource type.
*
*/
public Output type() {
return this.type;
}
/**
*
* @param name The _unique_ name of the resulting resource.
*/
public MachineGroup(java.lang.String name) {
this(name, MachineGroupArgs.Empty);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
*/
public MachineGroup(java.lang.String name, MachineGroupArgs args) {
this(name, args, null);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
* @param options A bag of options that control this resource's behavior.
*/
public MachineGroup(java.lang.String name, MachineGroupArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("azure-native:operationalinsights:MachineGroup", name, makeArgs(args, options), makeResourceOptions(options, Codegen.empty()), false);
}
private MachineGroup(java.lang.String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("azure-native:operationalinsights:MachineGroup", name, null, makeResourceOptions(options, id), false);
}
private static MachineGroupArgs makeArgs(MachineGroupArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
if (options != null && options.getUrn().isPresent()) {
return null;
}
var builder = args == null ? MachineGroupArgs.builder() : MachineGroupArgs.builder(args);
return builder
.kind("machineGroup")
.build();
}
private static com.pulumi.resources.CustomResourceOptions makeResourceOptions(@Nullable com.pulumi.resources.CustomResourceOptions options, @Nullable Output id) {
var defaultOptions = com.pulumi.resources.CustomResourceOptions.builder()
.version(Utilities.getVersion())
.aliases(List.of(
Output.of(Alias.builder().type("azure-native:operationalinsights/v20151101preview:MachineGroup").build())
))
.build();
return com.pulumi.resources.CustomResourceOptions.merge(defaultOptions, options, id);
}
/**
* Get an existing Host resource's state with the given name, ID, and optional extra
* properties used to qualify the lookup.
*
* @param name The _unique_ name of the resulting resource.
* @param id The _unique_ provider ID of the resource to lookup.
* @param options Optional settings to control the behavior of the CustomResource.
*/
public static MachineGroup get(java.lang.String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
return new MachineGroup(name, id, options);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy