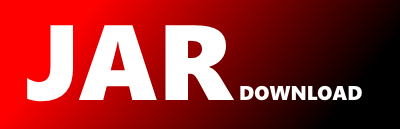
com.pulumi.azurenative.operationalinsights.MachineGroupArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.operationalinsights;
import com.pulumi.azurenative.operationalinsights.enums.MachineGroupType;
import com.pulumi.azurenative.operationalinsights.inputs.MachineReferenceWithHintsArgs;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.core.internal.Codegen;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class MachineGroupArgs extends com.pulumi.resources.ResourceArgs {
public static final MachineGroupArgs Empty = new MachineGroupArgs();
/**
* Count of machines in this group. The value of count may be bigger than the number of machines in case of the group has been truncated due to exceeding the max number of machines a group can handle.
*
*/
@Import(name="count")
private @Nullable Output count;
/**
* @return Count of machines in this group. The value of count may be bigger than the number of machines in case of the group has been truncated due to exceeding the max number of machines a group can handle.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy