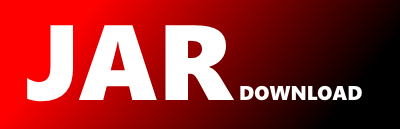
com.pulumi.azurenative.operationalinsights.OperationalinsightsFunctions Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.operationalinsights;
import com.pulumi.azurenative.Utilities;
import com.pulumi.azurenative.operationalinsights.inputs.GetClusterArgs;
import com.pulumi.azurenative.operationalinsights.inputs.GetClusterPlainArgs;
import com.pulumi.azurenative.operationalinsights.inputs.GetDataExportArgs;
import com.pulumi.azurenative.operationalinsights.inputs.GetDataExportPlainArgs;
import com.pulumi.azurenative.operationalinsights.inputs.GetDataSourceArgs;
import com.pulumi.azurenative.operationalinsights.inputs.GetDataSourcePlainArgs;
import com.pulumi.azurenative.operationalinsights.inputs.GetLinkedServiceArgs;
import com.pulumi.azurenative.operationalinsights.inputs.GetLinkedServicePlainArgs;
import com.pulumi.azurenative.operationalinsights.inputs.GetLinkedStorageAccountArgs;
import com.pulumi.azurenative.operationalinsights.inputs.GetLinkedStorageAccountPlainArgs;
import com.pulumi.azurenative.operationalinsights.inputs.GetMachineGroupArgs;
import com.pulumi.azurenative.operationalinsights.inputs.GetMachineGroupPlainArgs;
import com.pulumi.azurenative.operationalinsights.inputs.GetQueryArgs;
import com.pulumi.azurenative.operationalinsights.inputs.GetQueryPackArgs;
import com.pulumi.azurenative.operationalinsights.inputs.GetQueryPackPlainArgs;
import com.pulumi.azurenative.operationalinsights.inputs.GetQueryPlainArgs;
import com.pulumi.azurenative.operationalinsights.inputs.GetSavedSearchArgs;
import com.pulumi.azurenative.operationalinsights.inputs.GetSavedSearchPlainArgs;
import com.pulumi.azurenative.operationalinsights.inputs.GetSharedKeysArgs;
import com.pulumi.azurenative.operationalinsights.inputs.GetSharedKeysPlainArgs;
import com.pulumi.azurenative.operationalinsights.inputs.GetStorageInsightConfigArgs;
import com.pulumi.azurenative.operationalinsights.inputs.GetStorageInsightConfigPlainArgs;
import com.pulumi.azurenative.operationalinsights.inputs.GetTableArgs;
import com.pulumi.azurenative.operationalinsights.inputs.GetTablePlainArgs;
import com.pulumi.azurenative.operationalinsights.inputs.GetWorkspaceArgs;
import com.pulumi.azurenative.operationalinsights.inputs.GetWorkspacePlainArgs;
import com.pulumi.azurenative.operationalinsights.inputs.GetWorkspaceSharedKeysArgs;
import com.pulumi.azurenative.operationalinsights.inputs.GetWorkspaceSharedKeysPlainArgs;
import com.pulumi.azurenative.operationalinsights.outputs.GetClusterResult;
import com.pulumi.azurenative.operationalinsights.outputs.GetDataExportResult;
import com.pulumi.azurenative.operationalinsights.outputs.GetDataSourceResult;
import com.pulumi.azurenative.operationalinsights.outputs.GetLinkedServiceResult;
import com.pulumi.azurenative.operationalinsights.outputs.GetLinkedStorageAccountResult;
import com.pulumi.azurenative.operationalinsights.outputs.GetMachineGroupResult;
import com.pulumi.azurenative.operationalinsights.outputs.GetQueryPackResult;
import com.pulumi.azurenative.operationalinsights.outputs.GetQueryResult;
import com.pulumi.azurenative.operationalinsights.outputs.GetSavedSearchResult;
import com.pulumi.azurenative.operationalinsights.outputs.GetSharedKeysResult;
import com.pulumi.azurenative.operationalinsights.outputs.GetStorageInsightConfigResult;
import com.pulumi.azurenative.operationalinsights.outputs.GetTableResult;
import com.pulumi.azurenative.operationalinsights.outputs.GetWorkspaceResult;
import com.pulumi.azurenative.operationalinsights.outputs.GetWorkspaceSharedKeysResult;
import com.pulumi.core.Output;
import com.pulumi.core.TypeShape;
import com.pulumi.deployment.Deployment;
import com.pulumi.deployment.InvokeOptions;
import java.util.concurrent.CompletableFuture;
public final class OperationalinsightsFunctions {
/**
* Gets a Log Analytics cluster instance.
* Azure REST API version: 2021-06-01.
*
* Other available API versions: 2019-08-01-preview, 2020-08-01, 2022-10-01.
*
*/
public static Output getCluster(GetClusterArgs args) {
return getCluster(args, InvokeOptions.Empty);
}
/**
* Gets a Log Analytics cluster instance.
* Azure REST API version: 2021-06-01.
*
* Other available API versions: 2019-08-01-preview, 2020-08-01, 2022-10-01.
*
*/
public static CompletableFuture getClusterPlain(GetClusterPlainArgs args) {
return getClusterPlain(args, InvokeOptions.Empty);
}
/**
* Gets a Log Analytics cluster instance.
* Azure REST API version: 2021-06-01.
*
* Other available API versions: 2019-08-01-preview, 2020-08-01, 2022-10-01.
*
*/
public static Output getCluster(GetClusterArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:operationalinsights:getCluster", TypeShape.of(GetClusterResult.class), args, Utilities.withVersion(options));
}
/**
* Gets a Log Analytics cluster instance.
* Azure REST API version: 2021-06-01.
*
* Other available API versions: 2019-08-01-preview, 2020-08-01, 2022-10-01.
*
*/
public static CompletableFuture getClusterPlain(GetClusterPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:operationalinsights:getCluster", TypeShape.of(GetClusterResult.class), args, Utilities.withVersion(options));
}
/**
* Gets a data export instance.
* Azure REST API version: 2020-08-01.
*
*/
public static Output getDataExport(GetDataExportArgs args) {
return getDataExport(args, InvokeOptions.Empty);
}
/**
* Gets a data export instance.
* Azure REST API version: 2020-08-01.
*
*/
public static CompletableFuture getDataExportPlain(GetDataExportPlainArgs args) {
return getDataExportPlain(args, InvokeOptions.Empty);
}
/**
* Gets a data export instance.
* Azure REST API version: 2020-08-01.
*
*/
public static Output getDataExport(GetDataExportArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:operationalinsights:getDataExport", TypeShape.of(GetDataExportResult.class), args, Utilities.withVersion(options));
}
/**
* Gets a data export instance.
* Azure REST API version: 2020-08-01.
*
*/
public static CompletableFuture getDataExportPlain(GetDataExportPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:operationalinsights:getDataExport", TypeShape.of(GetDataExportResult.class), args, Utilities.withVersion(options));
}
/**
* Gets a datasource instance.
* Azure REST API version: 2020-08-01.
*
* Other available API versions: 2015-11-01-preview.
*
*/
public static Output getDataSource(GetDataSourceArgs args) {
return getDataSource(args, InvokeOptions.Empty);
}
/**
* Gets a datasource instance.
* Azure REST API version: 2020-08-01.
*
* Other available API versions: 2015-11-01-preview.
*
*/
public static CompletableFuture getDataSourcePlain(GetDataSourcePlainArgs args) {
return getDataSourcePlain(args, InvokeOptions.Empty);
}
/**
* Gets a datasource instance.
* Azure REST API version: 2020-08-01.
*
* Other available API versions: 2015-11-01-preview.
*
*/
public static Output getDataSource(GetDataSourceArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:operationalinsights:getDataSource", TypeShape.of(GetDataSourceResult.class), args, Utilities.withVersion(options));
}
/**
* Gets a datasource instance.
* Azure REST API version: 2020-08-01.
*
* Other available API versions: 2015-11-01-preview.
*
*/
public static CompletableFuture getDataSourcePlain(GetDataSourcePlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:operationalinsights:getDataSource", TypeShape.of(GetDataSourceResult.class), args, Utilities.withVersion(options));
}
/**
* Gets a linked service instance.
* Azure REST API version: 2020-08-01.
*
* Other available API versions: 2015-11-01-preview.
*
*/
public static Output getLinkedService(GetLinkedServiceArgs args) {
return getLinkedService(args, InvokeOptions.Empty);
}
/**
* Gets a linked service instance.
* Azure REST API version: 2020-08-01.
*
* Other available API versions: 2015-11-01-preview.
*
*/
public static CompletableFuture getLinkedServicePlain(GetLinkedServicePlainArgs args) {
return getLinkedServicePlain(args, InvokeOptions.Empty);
}
/**
* Gets a linked service instance.
* Azure REST API version: 2020-08-01.
*
* Other available API versions: 2015-11-01-preview.
*
*/
public static Output getLinkedService(GetLinkedServiceArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:operationalinsights:getLinkedService", TypeShape.of(GetLinkedServiceResult.class), args, Utilities.withVersion(options));
}
/**
* Gets a linked service instance.
* Azure REST API version: 2020-08-01.
*
* Other available API versions: 2015-11-01-preview.
*
*/
public static CompletableFuture getLinkedServicePlain(GetLinkedServicePlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:operationalinsights:getLinkedService", TypeShape.of(GetLinkedServiceResult.class), args, Utilities.withVersion(options));
}
/**
* Gets all linked storage account of a specific data source type associated with the specified workspace.
* Azure REST API version: 2020-08-01.
*
*/
public static Output getLinkedStorageAccount(GetLinkedStorageAccountArgs args) {
return getLinkedStorageAccount(args, InvokeOptions.Empty);
}
/**
* Gets all linked storage account of a specific data source type associated with the specified workspace.
* Azure REST API version: 2020-08-01.
*
*/
public static CompletableFuture getLinkedStorageAccountPlain(GetLinkedStorageAccountPlainArgs args) {
return getLinkedStorageAccountPlain(args, InvokeOptions.Empty);
}
/**
* Gets all linked storage account of a specific data source type associated with the specified workspace.
* Azure REST API version: 2020-08-01.
*
*/
public static Output getLinkedStorageAccount(GetLinkedStorageAccountArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:operationalinsights:getLinkedStorageAccount", TypeShape.of(GetLinkedStorageAccountResult.class), args, Utilities.withVersion(options));
}
/**
* Gets all linked storage account of a specific data source type associated with the specified workspace.
* Azure REST API version: 2020-08-01.
*
*/
public static CompletableFuture getLinkedStorageAccountPlain(GetLinkedStorageAccountPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:operationalinsights:getLinkedStorageAccount", TypeShape.of(GetLinkedStorageAccountResult.class), args, Utilities.withVersion(options));
}
/**
* Returns the specified machine group as it existed during the specified time interval.
* Azure REST API version: 2015-11-01-preview.
*
*/
public static Output getMachineGroup(GetMachineGroupArgs args) {
return getMachineGroup(args, InvokeOptions.Empty);
}
/**
* Returns the specified machine group as it existed during the specified time interval.
* Azure REST API version: 2015-11-01-preview.
*
*/
public static CompletableFuture getMachineGroupPlain(GetMachineGroupPlainArgs args) {
return getMachineGroupPlain(args, InvokeOptions.Empty);
}
/**
* Returns the specified machine group as it existed during the specified time interval.
* Azure REST API version: 2015-11-01-preview.
*
*/
public static Output getMachineGroup(GetMachineGroupArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:operationalinsights:getMachineGroup", TypeShape.of(GetMachineGroupResult.class), args, Utilities.withVersion(options));
}
/**
* Returns the specified machine group as it existed during the specified time interval.
* Azure REST API version: 2015-11-01-preview.
*
*/
public static CompletableFuture getMachineGroupPlain(GetMachineGroupPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:operationalinsights:getMachineGroup", TypeShape.of(GetMachineGroupResult.class), args, Utilities.withVersion(options));
}
/**
* Gets a specific Log Analytics Query defined within a Log Analytics QueryPack.
* Azure REST API version: 2019-09-01.
*
* Other available API versions: 2019-09-01-preview.
*
*/
public static Output getQuery(GetQueryArgs args) {
return getQuery(args, InvokeOptions.Empty);
}
/**
* Gets a specific Log Analytics Query defined within a Log Analytics QueryPack.
* Azure REST API version: 2019-09-01.
*
* Other available API versions: 2019-09-01-preview.
*
*/
public static CompletableFuture getQueryPlain(GetQueryPlainArgs args) {
return getQueryPlain(args, InvokeOptions.Empty);
}
/**
* Gets a specific Log Analytics Query defined within a Log Analytics QueryPack.
* Azure REST API version: 2019-09-01.
*
* Other available API versions: 2019-09-01-preview.
*
*/
public static Output getQuery(GetQueryArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:operationalinsights:getQuery", TypeShape.of(GetQueryResult.class), args, Utilities.withVersion(options));
}
/**
* Gets a specific Log Analytics Query defined within a Log Analytics QueryPack.
* Azure REST API version: 2019-09-01.
*
* Other available API versions: 2019-09-01-preview.
*
*/
public static CompletableFuture getQueryPlain(GetQueryPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:operationalinsights:getQuery", TypeShape.of(GetQueryResult.class), args, Utilities.withVersion(options));
}
/**
* Returns a Log Analytics QueryPack.
* Azure REST API version: 2019-09-01.
*
* Other available API versions: 2019-09-01-preview.
*
*/
public static Output getQueryPack(GetQueryPackArgs args) {
return getQueryPack(args, InvokeOptions.Empty);
}
/**
* Returns a Log Analytics QueryPack.
* Azure REST API version: 2019-09-01.
*
* Other available API versions: 2019-09-01-preview.
*
*/
public static CompletableFuture getQueryPackPlain(GetQueryPackPlainArgs args) {
return getQueryPackPlain(args, InvokeOptions.Empty);
}
/**
* Returns a Log Analytics QueryPack.
* Azure REST API version: 2019-09-01.
*
* Other available API versions: 2019-09-01-preview.
*
*/
public static Output getQueryPack(GetQueryPackArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:operationalinsights:getQueryPack", TypeShape.of(GetQueryPackResult.class), args, Utilities.withVersion(options));
}
/**
* Returns a Log Analytics QueryPack.
* Azure REST API version: 2019-09-01.
*
* Other available API versions: 2019-09-01-preview.
*
*/
public static CompletableFuture getQueryPackPlain(GetQueryPackPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:operationalinsights:getQueryPack", TypeShape.of(GetQueryPackResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the specified saved search for a given workspace.
* Azure REST API version: 2020-08-01.
*
* Other available API versions: 2015-03-20.
*
*/
public static Output getSavedSearch(GetSavedSearchArgs args) {
return getSavedSearch(args, InvokeOptions.Empty);
}
/**
* Gets the specified saved search for a given workspace.
* Azure REST API version: 2020-08-01.
*
* Other available API versions: 2015-03-20.
*
*/
public static CompletableFuture getSavedSearchPlain(GetSavedSearchPlainArgs args) {
return getSavedSearchPlain(args, InvokeOptions.Empty);
}
/**
* Gets the specified saved search for a given workspace.
* Azure REST API version: 2020-08-01.
*
* Other available API versions: 2015-03-20.
*
*/
public static Output getSavedSearch(GetSavedSearchArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:operationalinsights:getSavedSearch", TypeShape.of(GetSavedSearchResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the specified saved search for a given workspace.
* Azure REST API version: 2020-08-01.
*
* Other available API versions: 2015-03-20.
*
*/
public static CompletableFuture getSavedSearchPlain(GetSavedSearchPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:operationalinsights:getSavedSearch", TypeShape.of(GetSavedSearchResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the shared keys for a workspace.
* Azure REST API version: 2020-08-01.
*
*/
public static Output getSharedKeys(GetSharedKeysArgs args) {
return getSharedKeys(args, InvokeOptions.Empty);
}
/**
* Gets the shared keys for a workspace.
* Azure REST API version: 2020-08-01.
*
*/
public static CompletableFuture getSharedKeysPlain(GetSharedKeysPlainArgs args) {
return getSharedKeysPlain(args, InvokeOptions.Empty);
}
/**
* Gets the shared keys for a workspace.
* Azure REST API version: 2020-08-01.
*
*/
public static Output getSharedKeys(GetSharedKeysArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:operationalinsights:getSharedKeys", TypeShape.of(GetSharedKeysResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the shared keys for a workspace.
* Azure REST API version: 2020-08-01.
*
*/
public static CompletableFuture getSharedKeysPlain(GetSharedKeysPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:operationalinsights:getSharedKeys", TypeShape.of(GetSharedKeysResult.class), args, Utilities.withVersion(options));
}
/**
* Gets a storage insight instance.
* Azure REST API version: 2020-08-01.
*
*/
public static Output getStorageInsightConfig(GetStorageInsightConfigArgs args) {
return getStorageInsightConfig(args, InvokeOptions.Empty);
}
/**
* Gets a storage insight instance.
* Azure REST API version: 2020-08-01.
*
*/
public static CompletableFuture getStorageInsightConfigPlain(GetStorageInsightConfigPlainArgs args) {
return getStorageInsightConfigPlain(args, InvokeOptions.Empty);
}
/**
* Gets a storage insight instance.
* Azure REST API version: 2020-08-01.
*
*/
public static Output getStorageInsightConfig(GetStorageInsightConfigArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:operationalinsights:getStorageInsightConfig", TypeShape.of(GetStorageInsightConfigResult.class), args, Utilities.withVersion(options));
}
/**
* Gets a storage insight instance.
* Azure REST API version: 2020-08-01.
*
*/
public static CompletableFuture getStorageInsightConfigPlain(GetStorageInsightConfigPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:operationalinsights:getStorageInsightConfig", TypeShape.of(GetStorageInsightConfigResult.class), args, Utilities.withVersion(options));
}
/**
* Gets a Log Analytics workspace table.
* Azure REST API version: 2022-10-01.
*
*/
public static Output getTable(GetTableArgs args) {
return getTable(args, InvokeOptions.Empty);
}
/**
* Gets a Log Analytics workspace table.
* Azure REST API version: 2022-10-01.
*
*/
public static CompletableFuture getTablePlain(GetTablePlainArgs args) {
return getTablePlain(args, InvokeOptions.Empty);
}
/**
* Gets a Log Analytics workspace table.
* Azure REST API version: 2022-10-01.
*
*/
public static Output getTable(GetTableArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:operationalinsights:getTable", TypeShape.of(GetTableResult.class), args, Utilities.withVersion(options));
}
/**
* Gets a Log Analytics workspace table.
* Azure REST API version: 2022-10-01.
*
*/
public static CompletableFuture getTablePlain(GetTablePlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:operationalinsights:getTable", TypeShape.of(GetTableResult.class), args, Utilities.withVersion(options));
}
/**
* Gets a workspace instance.
* Azure REST API version: 2022-10-01.
*
* Other available API versions: 2015-11-01-preview, 2020-08-01, 2020-10-01, 2021-06-01, 2021-12-01-preview, 2023-09-01.
*
*/
public static Output getWorkspace(GetWorkspaceArgs args) {
return getWorkspace(args, InvokeOptions.Empty);
}
/**
* Gets a workspace instance.
* Azure REST API version: 2022-10-01.
*
* Other available API versions: 2015-11-01-preview, 2020-08-01, 2020-10-01, 2021-06-01, 2021-12-01-preview, 2023-09-01.
*
*/
public static CompletableFuture getWorkspacePlain(GetWorkspacePlainArgs args) {
return getWorkspacePlain(args, InvokeOptions.Empty);
}
/**
* Gets a workspace instance.
* Azure REST API version: 2022-10-01.
*
* Other available API versions: 2015-11-01-preview, 2020-08-01, 2020-10-01, 2021-06-01, 2021-12-01-preview, 2023-09-01.
*
*/
public static Output getWorkspace(GetWorkspaceArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:operationalinsights:getWorkspace", TypeShape.of(GetWorkspaceResult.class), args, Utilities.withVersion(options));
}
/**
* Gets a workspace instance.
* Azure REST API version: 2022-10-01.
*
* Other available API versions: 2015-11-01-preview, 2020-08-01, 2020-10-01, 2021-06-01, 2021-12-01-preview, 2023-09-01.
*
*/
public static CompletableFuture getWorkspacePlain(GetWorkspacePlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:operationalinsights:getWorkspace", TypeShape.of(GetWorkspaceResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the shared keys for a workspace.
* Azure REST API version: 2015-11-01-preview.
*
*/
public static Output getWorkspaceSharedKeys(GetWorkspaceSharedKeysArgs args) {
return getWorkspaceSharedKeys(args, InvokeOptions.Empty);
}
/**
* Gets the shared keys for a workspace.
* Azure REST API version: 2015-11-01-preview.
*
*/
public static CompletableFuture getWorkspaceSharedKeysPlain(GetWorkspaceSharedKeysPlainArgs args) {
return getWorkspaceSharedKeysPlain(args, InvokeOptions.Empty);
}
/**
* Gets the shared keys for a workspace.
* Azure REST API version: 2015-11-01-preview.
*
*/
public static Output getWorkspaceSharedKeys(GetWorkspaceSharedKeysArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:operationalinsights:getWorkspaceSharedKeys", TypeShape.of(GetWorkspaceSharedKeysResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the shared keys for a workspace.
* Azure REST API version: 2015-11-01-preview.
*
*/
public static CompletableFuture getWorkspaceSharedKeysPlain(GetWorkspaceSharedKeysPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:operationalinsights:getWorkspaceSharedKeys", TypeShape.of(GetWorkspaceSharedKeysResult.class), args, Utilities.withVersion(options));
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy