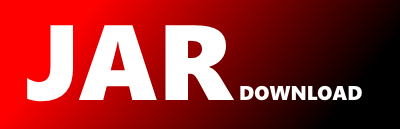
com.pulumi.azurenative.operationalinsights.QueryPack Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.operationalinsights;
import com.pulumi.azurenative.Utilities;
import com.pulumi.azurenative.operationalinsights.QueryPackArgs;
import com.pulumi.core.Alias;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* An Log Analytics QueryPack definition.
* Azure REST API version: 2019-09-01. Prior API version in Azure Native 1.x: 2019-09-01.
*
* Other available API versions: 2019-09-01-preview.
*
* ## Example Usage
* ### QueryPackCreate
*
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.operationalinsights.QueryPack;
* import com.pulumi.azurenative.operationalinsights.QueryPackArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var queryPack = new QueryPack("queryPack", QueryPackArgs.builder()
* .location("South Central US")
* .queryPackName("my-querypack")
* .resourceGroupName("my-resource-group")
* .build());
*
* }
* }
*
* }
*
* ### QueryPackUpdate
*
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.operationalinsights.QueryPack;
* import com.pulumi.azurenative.operationalinsights.QueryPackArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var queryPack = new QueryPack("queryPack", QueryPackArgs.builder()
* .location("South Central US")
* .queryPackName("my-querypack")
* .resourceGroupName("my-resource-group")
* .tags(Map.of("Tag1", "Value1"))
* .build());
*
* }
* }
*
* }
*
*
* ## Import
*
* An existing resource can be imported using its type token, name, and identifier, e.g.
*
* ```sh
* $ pulumi import azure-native:operationalinsights:QueryPack my-querypack /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.OperationalInsights/queryPacks/{queryPackName}
* ```
*
*/
@ResourceType(type="azure-native:operationalinsights:QueryPack")
public class QueryPack extends com.pulumi.resources.CustomResource {
/**
* Resource location
*
*/
@Export(name="location", refs={String.class}, tree="[0]")
private Output location;
/**
* @return Resource location
*
*/
public Output location() {
return this.location;
}
/**
* Azure resource name
*
*/
@Export(name="name", refs={String.class}, tree="[0]")
private Output name;
/**
* @return Azure resource name
*
*/
public Output name() {
return this.name;
}
/**
* Current state of this QueryPack: whether or not is has been provisioned within the resource group it is defined. Users cannot change this value but are able to read from it. Values will include Succeeded, Deploying, Canceled, and Failed.
*
*/
@Export(name="provisioningState", refs={String.class}, tree="[0]")
private Output provisioningState;
/**
* @return Current state of this QueryPack: whether or not is has been provisioned within the resource group it is defined. Users cannot change this value but are able to read from it. Values will include Succeeded, Deploying, Canceled, and Failed.
*
*/
public Output provisioningState() {
return this.provisioningState;
}
/**
* The unique ID of your application. This field cannot be changed.
*
*/
@Export(name="queryPackId", refs={String.class}, tree="[0]")
private Output queryPackId;
/**
* @return The unique ID of your application. This field cannot be changed.
*
*/
public Output queryPackId() {
return this.queryPackId;
}
/**
* Resource tags
*
*/
@Export(name="tags", refs={Map.class,String.class}, tree="[0,1,1]")
private Output* @Nullable */ Map> tags;
/**
* @return Resource tags
*
*/
public Output>> tags() {
return Codegen.optional(this.tags);
}
/**
* Creation Date for the Log Analytics QueryPack, in ISO 8601 format.
*
*/
@Export(name="timeCreated", refs={String.class}, tree="[0]")
private Output timeCreated;
/**
* @return Creation Date for the Log Analytics QueryPack, in ISO 8601 format.
*
*/
public Output timeCreated() {
return this.timeCreated;
}
/**
* Last modified date of the Log Analytics QueryPack, in ISO 8601 format.
*
*/
@Export(name="timeModified", refs={String.class}, tree="[0]")
private Output timeModified;
/**
* @return Last modified date of the Log Analytics QueryPack, in ISO 8601 format.
*
*/
public Output timeModified() {
return this.timeModified;
}
/**
* Azure resource type
*
*/
@Export(name="type", refs={String.class}, tree="[0]")
private Output type;
/**
* @return Azure resource type
*
*/
public Output type() {
return this.type;
}
/**
*
* @param name The _unique_ name of the resulting resource.
*/
public QueryPack(java.lang.String name) {
this(name, QueryPackArgs.Empty);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
*/
public QueryPack(java.lang.String name, QueryPackArgs args) {
this(name, args, null);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
* @param options A bag of options that control this resource's behavior.
*/
public QueryPack(java.lang.String name, QueryPackArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("azure-native:operationalinsights:QueryPack", name, makeArgs(args, options), makeResourceOptions(options, Codegen.empty()), false);
}
private QueryPack(java.lang.String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("azure-native:operationalinsights:QueryPack", name, null, makeResourceOptions(options, id), false);
}
private static QueryPackArgs makeArgs(QueryPackArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
if (options != null && options.getUrn().isPresent()) {
return null;
}
return args == null ? QueryPackArgs.Empty : args;
}
private static com.pulumi.resources.CustomResourceOptions makeResourceOptions(@Nullable com.pulumi.resources.CustomResourceOptions options, @Nullable Output id) {
var defaultOptions = com.pulumi.resources.CustomResourceOptions.builder()
.version(Utilities.getVersion())
.aliases(List.of(
Output.of(Alias.builder().type("azure-native:operationalinsights/v20190901:QueryPack").build()),
Output.of(Alias.builder().type("azure-native:operationalinsights/v20190901preview:QueryPack").build())
))
.build();
return com.pulumi.resources.CustomResourceOptions.merge(defaultOptions, options, id);
}
/**
* Get an existing Host resource's state with the given name, ID, and optional extra
* properties used to qualify the lookup.
*
* @param name The _unique_ name of the resulting resource.
* @param id The _unique_ provider ID of the resource to lookup.
* @param options Optional settings to control the behavior of the CustomResource.
*/
public static QueryPack get(java.lang.String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
return new QueryPack(name, id, options);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy