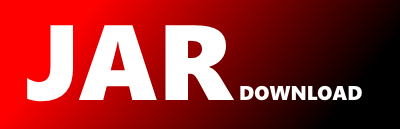
com.pulumi.azurenative.operationalinsights.outputs.GetClusterResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.operationalinsights.outputs;
import com.pulumi.azurenative.operationalinsights.outputs.AssociatedWorkspaceResponse;
import com.pulumi.azurenative.operationalinsights.outputs.CapacityReservationPropertiesResponse;
import com.pulumi.azurenative.operationalinsights.outputs.ClusterSkuResponse;
import com.pulumi.azurenative.operationalinsights.outputs.IdentityResponse;
import com.pulumi.azurenative.operationalinsights.outputs.KeyVaultPropertiesResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class GetClusterResult {
/**
* @return The list of Log Analytics workspaces associated with the cluster
*
*/
private @Nullable List associatedWorkspaces;
/**
* @return The cluster's billing type.
*
*/
private @Nullable String billingType;
/**
* @return Additional properties for capacity reservation
*
*/
private @Nullable CapacityReservationPropertiesResponse capacityReservationProperties;
/**
* @return The ID associated with the cluster.
*
*/
private String clusterId;
/**
* @return The cluster creation time
*
*/
private String createdDate;
/**
* @return Fully qualified resource ID for the resource. Ex - /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/{resourceProviderNamespace}/{resourceType}/{resourceName}
*
*/
private String id;
/**
* @return The identity of the resource.
*
*/
private @Nullable IdentityResponse identity;
/**
* @return Sets whether the cluster will support availability zones. This can be set as true only in regions where Azure Data Explorer support Availability Zones. This Property can not be modified after cluster creation. Default value is 'true' if region supports Availability Zones.
*
*/
private @Nullable Boolean isAvailabilityZonesEnabled;
/**
* @return Configures whether cluster will use double encryption. This Property can not be modified after cluster creation. Default value is 'true'
*
*/
private @Nullable Boolean isDoubleEncryptionEnabled;
/**
* @return The associated key properties.
*
*/
private @Nullable KeyVaultPropertiesResponse keyVaultProperties;
/**
* @return The last time the cluster was updated.
*
*/
private String lastModifiedDate;
/**
* @return The geo-location where the resource lives
*
*/
private String location;
/**
* @return The name of the resource
*
*/
private String name;
/**
* @return The provisioning state of the cluster.
*
*/
private String provisioningState;
/**
* @return The sku properties.
*
*/
private @Nullable ClusterSkuResponse sku;
/**
* @return Resource tags.
*
*/
private @Nullable Map tags;
/**
* @return The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
private String type;
private GetClusterResult() {}
/**
* @return The list of Log Analytics workspaces associated with the cluster
*
*/
public List associatedWorkspaces() {
return this.associatedWorkspaces == null ? List.of() : this.associatedWorkspaces;
}
/**
* @return The cluster's billing type.
*
*/
public Optional billingType() {
return Optional.ofNullable(this.billingType);
}
/**
* @return Additional properties for capacity reservation
*
*/
public Optional capacityReservationProperties() {
return Optional.ofNullable(this.capacityReservationProperties);
}
/**
* @return The ID associated with the cluster.
*
*/
public String clusterId() {
return this.clusterId;
}
/**
* @return The cluster creation time
*
*/
public String createdDate() {
return this.createdDate;
}
/**
* @return Fully qualified resource ID for the resource. Ex - /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/{resourceProviderNamespace}/{resourceType}/{resourceName}
*
*/
public String id() {
return this.id;
}
/**
* @return The identity of the resource.
*
*/
public Optional identity() {
return Optional.ofNullable(this.identity);
}
/**
* @return Sets whether the cluster will support availability zones. This can be set as true only in regions where Azure Data Explorer support Availability Zones. This Property can not be modified after cluster creation. Default value is 'true' if region supports Availability Zones.
*
*/
public Optional isAvailabilityZonesEnabled() {
return Optional.ofNullable(this.isAvailabilityZonesEnabled);
}
/**
* @return Configures whether cluster will use double encryption. This Property can not be modified after cluster creation. Default value is 'true'
*
*/
public Optional isDoubleEncryptionEnabled() {
return Optional.ofNullable(this.isDoubleEncryptionEnabled);
}
/**
* @return The associated key properties.
*
*/
public Optional keyVaultProperties() {
return Optional.ofNullable(this.keyVaultProperties);
}
/**
* @return The last time the cluster was updated.
*
*/
public String lastModifiedDate() {
return this.lastModifiedDate;
}
/**
* @return The geo-location where the resource lives
*
*/
public String location() {
return this.location;
}
/**
* @return The name of the resource
*
*/
public String name() {
return this.name;
}
/**
* @return The provisioning state of the cluster.
*
*/
public String provisioningState() {
return this.provisioningState;
}
/**
* @return The sku properties.
*
*/
public Optional sku() {
return Optional.ofNullable(this.sku);
}
/**
* @return Resource tags.
*
*/
public Map tags() {
return this.tags == null ? Map.of() : this.tags;
}
/**
* @return The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
public String type() {
return this.type;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetClusterResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable List associatedWorkspaces;
private @Nullable String billingType;
private @Nullable CapacityReservationPropertiesResponse capacityReservationProperties;
private String clusterId;
private String createdDate;
private String id;
private @Nullable IdentityResponse identity;
private @Nullable Boolean isAvailabilityZonesEnabled;
private @Nullable Boolean isDoubleEncryptionEnabled;
private @Nullable KeyVaultPropertiesResponse keyVaultProperties;
private String lastModifiedDate;
private String location;
private String name;
private String provisioningState;
private @Nullable ClusterSkuResponse sku;
private @Nullable Map tags;
private String type;
public Builder() {}
public Builder(GetClusterResult defaults) {
Objects.requireNonNull(defaults);
this.associatedWorkspaces = defaults.associatedWorkspaces;
this.billingType = defaults.billingType;
this.capacityReservationProperties = defaults.capacityReservationProperties;
this.clusterId = defaults.clusterId;
this.createdDate = defaults.createdDate;
this.id = defaults.id;
this.identity = defaults.identity;
this.isAvailabilityZonesEnabled = defaults.isAvailabilityZonesEnabled;
this.isDoubleEncryptionEnabled = defaults.isDoubleEncryptionEnabled;
this.keyVaultProperties = defaults.keyVaultProperties;
this.lastModifiedDate = defaults.lastModifiedDate;
this.location = defaults.location;
this.name = defaults.name;
this.provisioningState = defaults.provisioningState;
this.sku = defaults.sku;
this.tags = defaults.tags;
this.type = defaults.type;
}
@CustomType.Setter
public Builder associatedWorkspaces(@Nullable List associatedWorkspaces) {
this.associatedWorkspaces = associatedWorkspaces;
return this;
}
public Builder associatedWorkspaces(AssociatedWorkspaceResponse... associatedWorkspaces) {
return associatedWorkspaces(List.of(associatedWorkspaces));
}
@CustomType.Setter
public Builder billingType(@Nullable String billingType) {
this.billingType = billingType;
return this;
}
@CustomType.Setter
public Builder capacityReservationProperties(@Nullable CapacityReservationPropertiesResponse capacityReservationProperties) {
this.capacityReservationProperties = capacityReservationProperties;
return this;
}
@CustomType.Setter
public Builder clusterId(String clusterId) {
if (clusterId == null) {
throw new MissingRequiredPropertyException("GetClusterResult", "clusterId");
}
this.clusterId = clusterId;
return this;
}
@CustomType.Setter
public Builder createdDate(String createdDate) {
if (createdDate == null) {
throw new MissingRequiredPropertyException("GetClusterResult", "createdDate");
}
this.createdDate = createdDate;
return this;
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetClusterResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder identity(@Nullable IdentityResponse identity) {
this.identity = identity;
return this;
}
@CustomType.Setter
public Builder isAvailabilityZonesEnabled(@Nullable Boolean isAvailabilityZonesEnabled) {
this.isAvailabilityZonesEnabled = isAvailabilityZonesEnabled;
return this;
}
@CustomType.Setter
public Builder isDoubleEncryptionEnabled(@Nullable Boolean isDoubleEncryptionEnabled) {
this.isDoubleEncryptionEnabled = isDoubleEncryptionEnabled;
return this;
}
@CustomType.Setter
public Builder keyVaultProperties(@Nullable KeyVaultPropertiesResponse keyVaultProperties) {
this.keyVaultProperties = keyVaultProperties;
return this;
}
@CustomType.Setter
public Builder lastModifiedDate(String lastModifiedDate) {
if (lastModifiedDate == null) {
throw new MissingRequiredPropertyException("GetClusterResult", "lastModifiedDate");
}
this.lastModifiedDate = lastModifiedDate;
return this;
}
@CustomType.Setter
public Builder location(String location) {
if (location == null) {
throw new MissingRequiredPropertyException("GetClusterResult", "location");
}
this.location = location;
return this;
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("GetClusterResult", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder provisioningState(String provisioningState) {
if (provisioningState == null) {
throw new MissingRequiredPropertyException("GetClusterResult", "provisioningState");
}
this.provisioningState = provisioningState;
return this;
}
@CustomType.Setter
public Builder sku(@Nullable ClusterSkuResponse sku) {
this.sku = sku;
return this;
}
@CustomType.Setter
public Builder tags(@Nullable Map tags) {
this.tags = tags;
return this;
}
@CustomType.Setter
public Builder type(String type) {
if (type == null) {
throw new MissingRequiredPropertyException("GetClusterResult", "type");
}
this.type = type;
return this;
}
public GetClusterResult build() {
final var _resultValue = new GetClusterResult();
_resultValue.associatedWorkspaces = associatedWorkspaces;
_resultValue.billingType = billingType;
_resultValue.capacityReservationProperties = capacityReservationProperties;
_resultValue.clusterId = clusterId;
_resultValue.createdDate = createdDate;
_resultValue.id = id;
_resultValue.identity = identity;
_resultValue.isAvailabilityZonesEnabled = isAvailabilityZonesEnabled;
_resultValue.isDoubleEncryptionEnabled = isDoubleEncryptionEnabled;
_resultValue.keyVaultProperties = keyVaultProperties;
_resultValue.lastModifiedDate = lastModifiedDate;
_resultValue.location = location;
_resultValue.name = name;
_resultValue.provisioningState = provisioningState;
_resultValue.sku = sku;
_resultValue.tags = tags;
_resultValue.type = type;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy