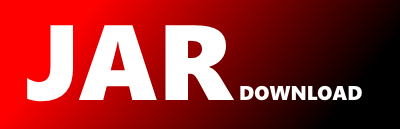
com.pulumi.azurenative.orbital.ContactProfileArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.orbital;
import com.pulumi.azurenative.orbital.enums.AutoTrackingConfiguration;
import com.pulumi.azurenative.orbital.inputs.ContactProfileLinkArgs;
import com.pulumi.azurenative.orbital.inputs.ContactProfileThirdPartyConfigurationArgs;
import com.pulumi.azurenative.orbital.inputs.ContactProfilesPropertiesNetworkConfigurationArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Double;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class ContactProfileArgs extends com.pulumi.resources.ResourceArgs {
public static final ContactProfileArgs Empty = new ContactProfileArgs();
/**
* Auto-tracking configuration.
*
*/
@Import(name="autoTrackingConfiguration")
private @Nullable Output autoTrackingConfiguration;
/**
* @return Auto-tracking configuration.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy