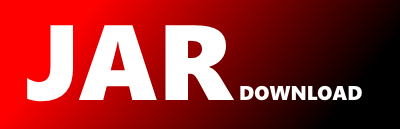
com.pulumi.azurenative.orbital.OrbitalFunctions Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.orbital;
import com.pulumi.azurenative.Utilities;
import com.pulumi.azurenative.orbital.inputs.GetContactArgs;
import com.pulumi.azurenative.orbital.inputs.GetContactPlainArgs;
import com.pulumi.azurenative.orbital.inputs.GetContactProfileArgs;
import com.pulumi.azurenative.orbital.inputs.GetContactProfilePlainArgs;
import com.pulumi.azurenative.orbital.inputs.GetEdgeSiteArgs;
import com.pulumi.azurenative.orbital.inputs.GetEdgeSitePlainArgs;
import com.pulumi.azurenative.orbital.inputs.GetGroundStationArgs;
import com.pulumi.azurenative.orbital.inputs.GetGroundStationPlainArgs;
import com.pulumi.azurenative.orbital.inputs.GetL2ConnectionArgs;
import com.pulumi.azurenative.orbital.inputs.GetL2ConnectionPlainArgs;
import com.pulumi.azurenative.orbital.inputs.GetSpacecraftArgs;
import com.pulumi.azurenative.orbital.inputs.GetSpacecraftPlainArgs;
import com.pulumi.azurenative.orbital.inputs.ListEdgeSiteL2ConnectionsArgs;
import com.pulumi.azurenative.orbital.inputs.ListEdgeSiteL2ConnectionsPlainArgs;
import com.pulumi.azurenative.orbital.inputs.ListGroundStationL2ConnectionsArgs;
import com.pulumi.azurenative.orbital.inputs.ListGroundStationL2ConnectionsPlainArgs;
import com.pulumi.azurenative.orbital.inputs.ListSpacecraftAvailableContactsArgs;
import com.pulumi.azurenative.orbital.inputs.ListSpacecraftAvailableContactsPlainArgs;
import com.pulumi.azurenative.orbital.outputs.GetContactProfileResult;
import com.pulumi.azurenative.orbital.outputs.GetContactResult;
import com.pulumi.azurenative.orbital.outputs.GetEdgeSiteResult;
import com.pulumi.azurenative.orbital.outputs.GetGroundStationResult;
import com.pulumi.azurenative.orbital.outputs.GetL2ConnectionResult;
import com.pulumi.azurenative.orbital.outputs.GetSpacecraftResult;
import com.pulumi.azurenative.orbital.outputs.ListEdgeSiteL2ConnectionsResult;
import com.pulumi.azurenative.orbital.outputs.ListGroundStationL2ConnectionsResult;
import com.pulumi.azurenative.orbital.outputs.ListSpacecraftAvailableContactsResult;
import com.pulumi.core.Output;
import com.pulumi.core.TypeShape;
import com.pulumi.deployment.Deployment;
import com.pulumi.deployment.InvokeOptions;
import java.util.concurrent.CompletableFuture;
public final class OrbitalFunctions {
/**
* Gets the specified contact in a specified resource group.
* Azure REST API version: 2022-11-01.
*
* Other available API versions: 2022-03-01.
*
*/
public static Output getContact(GetContactArgs args) {
return getContact(args, InvokeOptions.Empty);
}
/**
* Gets the specified contact in a specified resource group.
* Azure REST API version: 2022-11-01.
*
* Other available API versions: 2022-03-01.
*
*/
public static CompletableFuture getContactPlain(GetContactPlainArgs args) {
return getContactPlain(args, InvokeOptions.Empty);
}
/**
* Gets the specified contact in a specified resource group.
* Azure REST API version: 2022-11-01.
*
* Other available API versions: 2022-03-01.
*
*/
public static Output getContact(GetContactArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:orbital:getContact", TypeShape.of(GetContactResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the specified contact in a specified resource group.
* Azure REST API version: 2022-11-01.
*
* Other available API versions: 2022-03-01.
*
*/
public static CompletableFuture getContactPlain(GetContactPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:orbital:getContact", TypeShape.of(GetContactResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the specified contact Profile in a specified resource group.
* Azure REST API version: 2022-11-01.
*
* Other available API versions: 2022-03-01.
*
*/
public static Output getContactProfile(GetContactProfileArgs args) {
return getContactProfile(args, InvokeOptions.Empty);
}
/**
* Gets the specified contact Profile in a specified resource group.
* Azure REST API version: 2022-11-01.
*
* Other available API versions: 2022-03-01.
*
*/
public static CompletableFuture getContactProfilePlain(GetContactProfilePlainArgs args) {
return getContactProfilePlain(args, InvokeOptions.Empty);
}
/**
* Gets the specified contact Profile in a specified resource group.
* Azure REST API version: 2022-11-01.
*
* Other available API versions: 2022-03-01.
*
*/
public static Output getContactProfile(GetContactProfileArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:orbital:getContactProfile", TypeShape.of(GetContactProfileResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the specified contact Profile in a specified resource group.
* Azure REST API version: 2022-11-01.
*
* Other available API versions: 2022-03-01.
*
*/
public static CompletableFuture getContactProfilePlain(GetContactProfilePlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:orbital:getContactProfile", TypeShape.of(GetContactProfileResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the specified edge site in a specified resource group.
* Azure REST API version: 2024-03-01-preview.
*
* Other available API versions: 2024-03-01.
*
*/
public static Output getEdgeSite(GetEdgeSiteArgs args) {
return getEdgeSite(args, InvokeOptions.Empty);
}
/**
* Gets the specified edge site in a specified resource group.
* Azure REST API version: 2024-03-01-preview.
*
* Other available API versions: 2024-03-01.
*
*/
public static CompletableFuture getEdgeSitePlain(GetEdgeSitePlainArgs args) {
return getEdgeSitePlain(args, InvokeOptions.Empty);
}
/**
* Gets the specified edge site in a specified resource group.
* Azure REST API version: 2024-03-01-preview.
*
* Other available API versions: 2024-03-01.
*
*/
public static Output getEdgeSite(GetEdgeSiteArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:orbital:getEdgeSite", TypeShape.of(GetEdgeSiteResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the specified edge site in a specified resource group.
* Azure REST API version: 2024-03-01-preview.
*
* Other available API versions: 2024-03-01.
*
*/
public static CompletableFuture getEdgeSitePlain(GetEdgeSitePlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:orbital:getEdgeSite", TypeShape.of(GetEdgeSiteResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the specified ground station in a specified resource group.
* Azure REST API version: 2024-03-01-preview.
*
* Other available API versions: 2024-03-01.
*
*/
public static Output getGroundStation(GetGroundStationArgs args) {
return getGroundStation(args, InvokeOptions.Empty);
}
/**
* Gets the specified ground station in a specified resource group.
* Azure REST API version: 2024-03-01-preview.
*
* Other available API versions: 2024-03-01.
*
*/
public static CompletableFuture getGroundStationPlain(GetGroundStationPlainArgs args) {
return getGroundStationPlain(args, InvokeOptions.Empty);
}
/**
* Gets the specified ground station in a specified resource group.
* Azure REST API version: 2024-03-01-preview.
*
* Other available API versions: 2024-03-01.
*
*/
public static Output getGroundStation(GetGroundStationArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:orbital:getGroundStation", TypeShape.of(GetGroundStationResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the specified ground station in a specified resource group.
* Azure REST API version: 2024-03-01-preview.
*
* Other available API versions: 2024-03-01.
*
*/
public static CompletableFuture getGroundStationPlain(GetGroundStationPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:orbital:getGroundStation", TypeShape.of(GetGroundStationResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the specified L2 connection in a specified resource group.
* Azure REST API version: 2024-03-01-preview.
*
* Other available API versions: 2024-03-01.
*
*/
public static Output getL2Connection(GetL2ConnectionArgs args) {
return getL2Connection(args, InvokeOptions.Empty);
}
/**
* Gets the specified L2 connection in a specified resource group.
* Azure REST API version: 2024-03-01-preview.
*
* Other available API versions: 2024-03-01.
*
*/
public static CompletableFuture getL2ConnectionPlain(GetL2ConnectionPlainArgs args) {
return getL2ConnectionPlain(args, InvokeOptions.Empty);
}
/**
* Gets the specified L2 connection in a specified resource group.
* Azure REST API version: 2024-03-01-preview.
*
* Other available API versions: 2024-03-01.
*
*/
public static Output getL2Connection(GetL2ConnectionArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:orbital:getL2Connection", TypeShape.of(GetL2ConnectionResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the specified L2 connection in a specified resource group.
* Azure REST API version: 2024-03-01-preview.
*
* Other available API versions: 2024-03-01.
*
*/
public static CompletableFuture getL2ConnectionPlain(GetL2ConnectionPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:orbital:getL2Connection", TypeShape.of(GetL2ConnectionResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the specified spacecraft in a specified resource group.
* Azure REST API version: 2022-11-01.
*
* Other available API versions: 2022-03-01.
*
*/
public static Output getSpacecraft(GetSpacecraftArgs args) {
return getSpacecraft(args, InvokeOptions.Empty);
}
/**
* Gets the specified spacecraft in a specified resource group.
* Azure REST API version: 2022-11-01.
*
* Other available API versions: 2022-03-01.
*
*/
public static CompletableFuture getSpacecraftPlain(GetSpacecraftPlainArgs args) {
return getSpacecraftPlain(args, InvokeOptions.Empty);
}
/**
* Gets the specified spacecraft in a specified resource group.
* Azure REST API version: 2022-11-01.
*
* Other available API versions: 2022-03-01.
*
*/
public static Output getSpacecraft(GetSpacecraftArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:orbital:getSpacecraft", TypeShape.of(GetSpacecraftResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the specified spacecraft in a specified resource group.
* Azure REST API version: 2022-11-01.
*
* Other available API versions: 2022-03-01.
*
*/
public static CompletableFuture getSpacecraftPlain(GetSpacecraftPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:orbital:getSpacecraft", TypeShape.of(GetSpacecraftResult.class), args, Utilities.withVersion(options));
}
/**
* Returns a list of L2 Connections attached to an edge site.
* Azure REST API version: 2024-03-01-preview.
*
* Other available API versions: 2024-03-01.
*
*/
public static Output listEdgeSiteL2Connections(ListEdgeSiteL2ConnectionsArgs args) {
return listEdgeSiteL2Connections(args, InvokeOptions.Empty);
}
/**
* Returns a list of L2 Connections attached to an edge site.
* Azure REST API version: 2024-03-01-preview.
*
* Other available API versions: 2024-03-01.
*
*/
public static CompletableFuture listEdgeSiteL2ConnectionsPlain(ListEdgeSiteL2ConnectionsPlainArgs args) {
return listEdgeSiteL2ConnectionsPlain(args, InvokeOptions.Empty);
}
/**
* Returns a list of L2 Connections attached to an edge site.
* Azure REST API version: 2024-03-01-preview.
*
* Other available API versions: 2024-03-01.
*
*/
public static Output listEdgeSiteL2Connections(ListEdgeSiteL2ConnectionsArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:orbital:listEdgeSiteL2Connections", TypeShape.of(ListEdgeSiteL2ConnectionsResult.class), args, Utilities.withVersion(options));
}
/**
* Returns a list of L2 Connections attached to an edge site.
* Azure REST API version: 2024-03-01-preview.
*
* Other available API versions: 2024-03-01.
*
*/
public static CompletableFuture listEdgeSiteL2ConnectionsPlain(ListEdgeSiteL2ConnectionsPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:orbital:listEdgeSiteL2Connections", TypeShape.of(ListEdgeSiteL2ConnectionsResult.class), args, Utilities.withVersion(options));
}
/**
* Returns a list of L2 Connections attached to an ground station.
* Azure REST API version: 2024-03-01-preview.
*
* Other available API versions: 2024-03-01.
*
*/
public static Output listGroundStationL2Connections(ListGroundStationL2ConnectionsArgs args) {
return listGroundStationL2Connections(args, InvokeOptions.Empty);
}
/**
* Returns a list of L2 Connections attached to an ground station.
* Azure REST API version: 2024-03-01-preview.
*
* Other available API versions: 2024-03-01.
*
*/
public static CompletableFuture listGroundStationL2ConnectionsPlain(ListGroundStationL2ConnectionsPlainArgs args) {
return listGroundStationL2ConnectionsPlain(args, InvokeOptions.Empty);
}
/**
* Returns a list of L2 Connections attached to an ground station.
* Azure REST API version: 2024-03-01-preview.
*
* Other available API versions: 2024-03-01.
*
*/
public static Output listGroundStationL2Connections(ListGroundStationL2ConnectionsArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:orbital:listGroundStationL2Connections", TypeShape.of(ListGroundStationL2ConnectionsResult.class), args, Utilities.withVersion(options));
}
/**
* Returns a list of L2 Connections attached to an ground station.
* Azure REST API version: 2024-03-01-preview.
*
* Other available API versions: 2024-03-01.
*
*/
public static CompletableFuture listGroundStationL2ConnectionsPlain(ListGroundStationL2ConnectionsPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:orbital:listGroundStationL2Connections", TypeShape.of(ListGroundStationL2ConnectionsResult.class), args, Utilities.withVersion(options));
}
/**
* Returns list of available contacts. A contact is available if the spacecraft is visible from the ground station for more than the minimum viable contact duration provided in the contact profile.
* Azure REST API version: 2022-11-01.
*
* Other available API versions: 2022-03-01.
*
*/
public static Output listSpacecraftAvailableContacts(ListSpacecraftAvailableContactsArgs args) {
return listSpacecraftAvailableContacts(args, InvokeOptions.Empty);
}
/**
* Returns list of available contacts. A contact is available if the spacecraft is visible from the ground station for more than the minimum viable contact duration provided in the contact profile.
* Azure REST API version: 2022-11-01.
*
* Other available API versions: 2022-03-01.
*
*/
public static CompletableFuture listSpacecraftAvailableContactsPlain(ListSpacecraftAvailableContactsPlainArgs args) {
return listSpacecraftAvailableContactsPlain(args, InvokeOptions.Empty);
}
/**
* Returns list of available contacts. A contact is available if the spacecraft is visible from the ground station for more than the minimum viable contact duration provided in the contact profile.
* Azure REST API version: 2022-11-01.
*
* Other available API versions: 2022-03-01.
*
*/
public static Output listSpacecraftAvailableContacts(ListSpacecraftAvailableContactsArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:orbital:listSpacecraftAvailableContacts", TypeShape.of(ListSpacecraftAvailableContactsResult.class), args, Utilities.withVersion(options));
}
/**
* Returns list of available contacts. A contact is available if the spacecraft is visible from the ground station for more than the minimum viable contact duration provided in the contact profile.
* Azure REST API version: 2022-11-01.
*
* Other available API versions: 2022-03-01.
*
*/
public static CompletableFuture listSpacecraftAvailableContactsPlain(ListSpacecraftAvailableContactsPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:orbital:listSpacecraftAvailableContacts", TypeShape.of(ListSpacecraftAvailableContactsResult.class), args, Utilities.withVersion(options));
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy