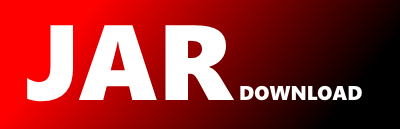
com.pulumi.azurenative.orbital.inputs.EndPointArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.orbital.inputs;
import com.pulumi.azurenative.orbital.enums.Protocol;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.Objects;
/**
* Customer end point to store and retrieve data during a contact with the spacecraft.
*
*/
public final class EndPointArgs extends com.pulumi.resources.ResourceArgs {
public static final EndPointArgs Empty = new EndPointArgs();
/**
* Name of an end point.
*
*/
@Import(name="endPointName", required=true)
private Output endPointName;
/**
* @return Name of an end point.
*
*/
public Output endPointName() {
return this.endPointName;
}
/**
* IP Address (IPv4).
*
*/
@Import(name="ipAddress", required=true)
private Output ipAddress;
/**
* @return IP Address (IPv4).
*
*/
public Output ipAddress() {
return this.ipAddress;
}
/**
* TCP port to listen on to receive data.
*
*/
@Import(name="port", required=true)
private Output port;
/**
* @return TCP port to listen on to receive data.
*
*/
public Output port() {
return this.port;
}
/**
* Protocol either UDP or TCP.
*
*/
@Import(name="protocol", required=true)
private Output> protocol;
/**
* @return Protocol either UDP or TCP.
*
*/
public Output> protocol() {
return this.protocol;
}
private EndPointArgs() {}
private EndPointArgs(EndPointArgs $) {
this.endPointName = $.endPointName;
this.ipAddress = $.ipAddress;
this.port = $.port;
this.protocol = $.protocol;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(EndPointArgs defaults) {
return new Builder(defaults);
}
public static final class Builder {
private EndPointArgs $;
public Builder() {
$ = new EndPointArgs();
}
public Builder(EndPointArgs defaults) {
$ = new EndPointArgs(Objects.requireNonNull(defaults));
}
/**
* @param endPointName Name of an end point.
*
* @return builder
*
*/
public Builder endPointName(Output endPointName) {
$.endPointName = endPointName;
return this;
}
/**
* @param endPointName Name of an end point.
*
* @return builder
*
*/
public Builder endPointName(String endPointName) {
return endPointName(Output.of(endPointName));
}
/**
* @param ipAddress IP Address (IPv4).
*
* @return builder
*
*/
public Builder ipAddress(Output ipAddress) {
$.ipAddress = ipAddress;
return this;
}
/**
* @param ipAddress IP Address (IPv4).
*
* @return builder
*
*/
public Builder ipAddress(String ipAddress) {
return ipAddress(Output.of(ipAddress));
}
/**
* @param port TCP port to listen on to receive data.
*
* @return builder
*
*/
public Builder port(Output port) {
$.port = port;
return this;
}
/**
* @param port TCP port to listen on to receive data.
*
* @return builder
*
*/
public Builder port(String port) {
return port(Output.of(port));
}
/**
* @param protocol Protocol either UDP or TCP.
*
* @return builder
*
*/
public Builder protocol(Output> protocol) {
$.protocol = protocol;
return this;
}
/**
* @param protocol Protocol either UDP or TCP.
*
* @return builder
*
*/
public Builder protocol(Either protocol) {
return protocol(Output.of(protocol));
}
/**
* @param protocol Protocol either UDP or TCP.
*
* @return builder
*
*/
public Builder protocol(String protocol) {
return protocol(Either.ofLeft(protocol));
}
/**
* @param protocol Protocol either UDP or TCP.
*
* @return builder
*
*/
public Builder protocol(Protocol protocol) {
return protocol(Either.ofRight(protocol));
}
public EndPointArgs build() {
if ($.endPointName == null) {
throw new MissingRequiredPropertyException("EndPointArgs", "endPointName");
}
if ($.ipAddress == null) {
throw new MissingRequiredPropertyException("EndPointArgs", "ipAddress");
}
if ($.port == null) {
throw new MissingRequiredPropertyException("EndPointArgs", "port");
}
if ($.protocol == null) {
throw new MissingRequiredPropertyException("EndPointArgs", "protocol");
}
return $;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy