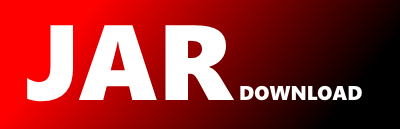
com.pulumi.azurenative.peering.inputs.BgpSessionArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.peering.inputs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.Integer;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* The properties that define a BGP session.
*
*/
public final class BgpSessionArgs extends com.pulumi.resources.ResourceArgs {
public static final BgpSessionArgs Empty = new BgpSessionArgs();
/**
* The maximum number of prefixes advertised over the IPv4 session.
*
*/
@Import(name="maxPrefixesAdvertisedV4")
private @Nullable Output maxPrefixesAdvertisedV4;
/**
* @return The maximum number of prefixes advertised over the IPv4 session.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy