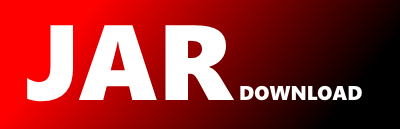
com.pulumi.azurenative.peering.inputs.DirectConnectionArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.peering.inputs;
import com.pulumi.azurenative.peering.enums.SessionAddressProvider;
import com.pulumi.azurenative.peering.inputs.BgpSessionArgs;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* The properties that define a direct connection.
*
*/
public final class DirectConnectionArgs extends com.pulumi.resources.ResourceArgs {
public static final DirectConnectionArgs Empty = new DirectConnectionArgs();
/**
* The bandwidth of the connection.
*
*/
@Import(name="bandwidthInMbps")
private @Nullable Output bandwidthInMbps;
/**
* @return The bandwidth of the connection.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy