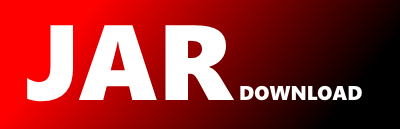
com.pulumi.azurenative.peering.outputs.GetPrefixResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.peering.outputs;
import com.pulumi.azurenative.peering.outputs.PeeringServicePrefixEventResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class GetPrefixResult {
/**
* @return The error message for validation state
*
*/
private String errorMessage;
/**
* @return The list of events for peering service prefix
*
*/
private List events;
/**
* @return The ID of the resource.
*
*/
private String id;
/**
* @return The prefix learned type
*
*/
private String learnedType;
/**
* @return The name of the resource.
*
*/
private String name;
/**
* @return The peering service prefix key
*
*/
private @Nullable String peeringServicePrefixKey;
/**
* @return The prefix from which your traffic originates.
*
*/
private @Nullable String prefix;
/**
* @return The prefix validation state
*
*/
private String prefixValidationState;
/**
* @return The provisioning state of the resource.
*
*/
private String provisioningState;
/**
* @return The type of the resource.
*
*/
private String type;
private GetPrefixResult() {}
/**
* @return The error message for validation state
*
*/
public String errorMessage() {
return this.errorMessage;
}
/**
* @return The list of events for peering service prefix
*
*/
public List events() {
return this.events;
}
/**
* @return The ID of the resource.
*
*/
public String id() {
return this.id;
}
/**
* @return The prefix learned type
*
*/
public String learnedType() {
return this.learnedType;
}
/**
* @return The name of the resource.
*
*/
public String name() {
return this.name;
}
/**
* @return The peering service prefix key
*
*/
public Optional peeringServicePrefixKey() {
return Optional.ofNullable(this.peeringServicePrefixKey);
}
/**
* @return The prefix from which your traffic originates.
*
*/
public Optional prefix() {
return Optional.ofNullable(this.prefix);
}
/**
* @return The prefix validation state
*
*/
public String prefixValidationState() {
return this.prefixValidationState;
}
/**
* @return The provisioning state of the resource.
*
*/
public String provisioningState() {
return this.provisioningState;
}
/**
* @return The type of the resource.
*
*/
public String type() {
return this.type;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetPrefixResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String errorMessage;
private List events;
private String id;
private String learnedType;
private String name;
private @Nullable String peeringServicePrefixKey;
private @Nullable String prefix;
private String prefixValidationState;
private String provisioningState;
private String type;
public Builder() {}
public Builder(GetPrefixResult defaults) {
Objects.requireNonNull(defaults);
this.errorMessage = defaults.errorMessage;
this.events = defaults.events;
this.id = defaults.id;
this.learnedType = defaults.learnedType;
this.name = defaults.name;
this.peeringServicePrefixKey = defaults.peeringServicePrefixKey;
this.prefix = defaults.prefix;
this.prefixValidationState = defaults.prefixValidationState;
this.provisioningState = defaults.provisioningState;
this.type = defaults.type;
}
@CustomType.Setter
public Builder errorMessage(String errorMessage) {
if (errorMessage == null) {
throw new MissingRequiredPropertyException("GetPrefixResult", "errorMessage");
}
this.errorMessage = errorMessage;
return this;
}
@CustomType.Setter
public Builder events(List events) {
if (events == null) {
throw new MissingRequiredPropertyException("GetPrefixResult", "events");
}
this.events = events;
return this;
}
public Builder events(PeeringServicePrefixEventResponse... events) {
return events(List.of(events));
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetPrefixResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder learnedType(String learnedType) {
if (learnedType == null) {
throw new MissingRequiredPropertyException("GetPrefixResult", "learnedType");
}
this.learnedType = learnedType;
return this;
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("GetPrefixResult", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder peeringServicePrefixKey(@Nullable String peeringServicePrefixKey) {
this.peeringServicePrefixKey = peeringServicePrefixKey;
return this;
}
@CustomType.Setter
public Builder prefix(@Nullable String prefix) {
this.prefix = prefix;
return this;
}
@CustomType.Setter
public Builder prefixValidationState(String prefixValidationState) {
if (prefixValidationState == null) {
throw new MissingRequiredPropertyException("GetPrefixResult", "prefixValidationState");
}
this.prefixValidationState = prefixValidationState;
return this;
}
@CustomType.Setter
public Builder provisioningState(String provisioningState) {
if (provisioningState == null) {
throw new MissingRequiredPropertyException("GetPrefixResult", "provisioningState");
}
this.provisioningState = provisioningState;
return this;
}
@CustomType.Setter
public Builder type(String type) {
if (type == null) {
throw new MissingRequiredPropertyException("GetPrefixResult", "type");
}
this.type = type;
return this;
}
public GetPrefixResult build() {
final var _resultValue = new GetPrefixResult();
_resultValue.errorMessage = errorMessage;
_resultValue.events = events;
_resultValue.id = id;
_resultValue.learnedType = learnedType;
_resultValue.name = name;
_resultValue.peeringServicePrefixKey = peeringServicePrefixKey;
_resultValue.prefix = prefix;
_resultValue.prefixValidationState = prefixValidationState;
_resultValue.provisioningState = provisioningState;
_resultValue.type = type;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy