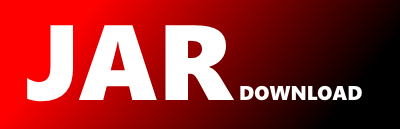
com.pulumi.azurenative.policyinsights.AttestationAtResourceGroupArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.policyinsights;
import com.pulumi.azurenative.policyinsights.enums.ComplianceState;
import com.pulumi.azurenative.policyinsights.inputs.AttestationEvidenceArgs;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Object;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class AttestationAtResourceGroupArgs extends com.pulumi.resources.ResourceArgs {
public static final AttestationAtResourceGroupArgs Empty = new AttestationAtResourceGroupArgs();
/**
* The time the evidence was assessed
*
*/
@Import(name="assessmentDate")
private @Nullable Output assessmentDate;
/**
* @return The time the evidence was assessed
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy