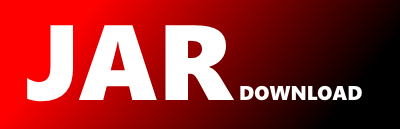
com.pulumi.azurenative.policyinsights.outputs.RemediationDeploymentResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.policyinsights.outputs;
import com.pulumi.azurenative.policyinsights.outputs.ErrorDefinitionResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.Objects;
@CustomType
public final class RemediationDeploymentResponse {
/**
* @return The time at which the remediation was created.
*
*/
private String createdOn;
/**
* @return Resource ID of the template deployment that will remediate the resource.
*
*/
private String deploymentId;
/**
* @return Error encountered while remediated the resource.
*
*/
private ErrorDefinitionResponse error;
/**
* @return The time at which the remediation deployment was last updated.
*
*/
private String lastUpdatedOn;
/**
* @return Resource ID of the resource that is being remediated by the deployment.
*
*/
private String remediatedResourceId;
/**
* @return Location of the resource that is being remediated.
*
*/
private String resourceLocation;
/**
* @return Status of the remediation deployment.
*
*/
private String status;
private RemediationDeploymentResponse() {}
/**
* @return The time at which the remediation was created.
*
*/
public String createdOn() {
return this.createdOn;
}
/**
* @return Resource ID of the template deployment that will remediate the resource.
*
*/
public String deploymentId() {
return this.deploymentId;
}
/**
* @return Error encountered while remediated the resource.
*
*/
public ErrorDefinitionResponse error() {
return this.error;
}
/**
* @return The time at which the remediation deployment was last updated.
*
*/
public String lastUpdatedOn() {
return this.lastUpdatedOn;
}
/**
* @return Resource ID of the resource that is being remediated by the deployment.
*
*/
public String remediatedResourceId() {
return this.remediatedResourceId;
}
/**
* @return Location of the resource that is being remediated.
*
*/
public String resourceLocation() {
return this.resourceLocation;
}
/**
* @return Status of the remediation deployment.
*
*/
public String status() {
return this.status;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(RemediationDeploymentResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String createdOn;
private String deploymentId;
private ErrorDefinitionResponse error;
private String lastUpdatedOn;
private String remediatedResourceId;
private String resourceLocation;
private String status;
public Builder() {}
public Builder(RemediationDeploymentResponse defaults) {
Objects.requireNonNull(defaults);
this.createdOn = defaults.createdOn;
this.deploymentId = defaults.deploymentId;
this.error = defaults.error;
this.lastUpdatedOn = defaults.lastUpdatedOn;
this.remediatedResourceId = defaults.remediatedResourceId;
this.resourceLocation = defaults.resourceLocation;
this.status = defaults.status;
}
@CustomType.Setter
public Builder createdOn(String createdOn) {
if (createdOn == null) {
throw new MissingRequiredPropertyException("RemediationDeploymentResponse", "createdOn");
}
this.createdOn = createdOn;
return this;
}
@CustomType.Setter
public Builder deploymentId(String deploymentId) {
if (deploymentId == null) {
throw new MissingRequiredPropertyException("RemediationDeploymentResponse", "deploymentId");
}
this.deploymentId = deploymentId;
return this;
}
@CustomType.Setter
public Builder error(ErrorDefinitionResponse error) {
if (error == null) {
throw new MissingRequiredPropertyException("RemediationDeploymentResponse", "error");
}
this.error = error;
return this;
}
@CustomType.Setter
public Builder lastUpdatedOn(String lastUpdatedOn) {
if (lastUpdatedOn == null) {
throw new MissingRequiredPropertyException("RemediationDeploymentResponse", "lastUpdatedOn");
}
this.lastUpdatedOn = lastUpdatedOn;
return this;
}
@CustomType.Setter
public Builder remediatedResourceId(String remediatedResourceId) {
if (remediatedResourceId == null) {
throw new MissingRequiredPropertyException("RemediationDeploymentResponse", "remediatedResourceId");
}
this.remediatedResourceId = remediatedResourceId;
return this;
}
@CustomType.Setter
public Builder resourceLocation(String resourceLocation) {
if (resourceLocation == null) {
throw new MissingRequiredPropertyException("RemediationDeploymentResponse", "resourceLocation");
}
this.resourceLocation = resourceLocation;
return this;
}
@CustomType.Setter
public Builder status(String status) {
if (status == null) {
throw new MissingRequiredPropertyException("RemediationDeploymentResponse", "status");
}
this.status = status;
return this;
}
public RemediationDeploymentResponse build() {
final var _resultValue = new RemediationDeploymentResponse();
_resultValue.createdOn = createdOn;
_resultValue.deploymentId = deploymentId;
_resultValue.error = error;
_resultValue.lastUpdatedOn = lastUpdatedOn;
_resultValue.remediatedResourceId = remediatedResourceId;
_resultValue.resourceLocation = resourceLocation;
_resultValue.status = status;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy