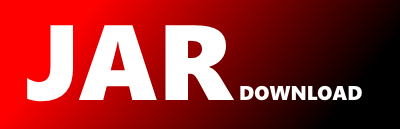
com.pulumi.azurenative.portal.inputs.UserPropertiesArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.portal.inputs;
import com.pulumi.azurenative.portal.enums.OsType;
import com.pulumi.azurenative.portal.enums.ShellType;
import com.pulumi.azurenative.portal.inputs.StorageProfileArgs;
import com.pulumi.azurenative.portal.inputs.TerminalSettingsArgs;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.Objects;
/**
* The cloud shell user settings properties.
*
*/
public final class UserPropertiesArgs extends com.pulumi.resources.ResourceArgs {
public static final UserPropertiesArgs Empty = new UserPropertiesArgs();
/**
* The preferred location of the cloud shell.
*
*/
@Import(name="preferredLocation", required=true)
private Output preferredLocation;
/**
* @return The preferred location of the cloud shell.
*
*/
public Output preferredLocation() {
return this.preferredLocation;
}
/**
* The operating system type of the cloud shell. Deprecated, use preferredShellType.
*
*/
@Import(name="preferredOsType", required=true)
private Output> preferredOsType;
/**
* @return The operating system type of the cloud shell. Deprecated, use preferredShellType.
*
*/
public Output> preferredOsType() {
return this.preferredOsType;
}
/**
* The shell type of the cloud shell.
*
*/
@Import(name="preferredShellType", required=true)
private Output> preferredShellType;
/**
* @return The shell type of the cloud shell.
*
*/
public Output> preferredShellType() {
return this.preferredShellType;
}
/**
* The storage profile of the user settings.
*
*/
@Import(name="storageProfile", required=true)
private Output storageProfile;
/**
* @return The storage profile of the user settings.
*
*/
public Output storageProfile() {
return this.storageProfile;
}
/**
* Settings for terminal appearance.
*
*/
@Import(name="terminalSettings", required=true)
private Output terminalSettings;
/**
* @return Settings for terminal appearance.
*
*/
public Output terminalSettings() {
return this.terminalSettings;
}
private UserPropertiesArgs() {}
private UserPropertiesArgs(UserPropertiesArgs $) {
this.preferredLocation = $.preferredLocation;
this.preferredOsType = $.preferredOsType;
this.preferredShellType = $.preferredShellType;
this.storageProfile = $.storageProfile;
this.terminalSettings = $.terminalSettings;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(UserPropertiesArgs defaults) {
return new Builder(defaults);
}
public static final class Builder {
private UserPropertiesArgs $;
public Builder() {
$ = new UserPropertiesArgs();
}
public Builder(UserPropertiesArgs defaults) {
$ = new UserPropertiesArgs(Objects.requireNonNull(defaults));
}
/**
* @param preferredLocation The preferred location of the cloud shell.
*
* @return builder
*
*/
public Builder preferredLocation(Output preferredLocation) {
$.preferredLocation = preferredLocation;
return this;
}
/**
* @param preferredLocation The preferred location of the cloud shell.
*
* @return builder
*
*/
public Builder preferredLocation(String preferredLocation) {
return preferredLocation(Output.of(preferredLocation));
}
/**
* @param preferredOsType The operating system type of the cloud shell. Deprecated, use preferredShellType.
*
* @return builder
*
*/
public Builder preferredOsType(Output> preferredOsType) {
$.preferredOsType = preferredOsType;
return this;
}
/**
* @param preferredOsType The operating system type of the cloud shell. Deprecated, use preferredShellType.
*
* @return builder
*
*/
public Builder preferredOsType(Either preferredOsType) {
return preferredOsType(Output.of(preferredOsType));
}
/**
* @param preferredOsType The operating system type of the cloud shell. Deprecated, use preferredShellType.
*
* @return builder
*
*/
public Builder preferredOsType(String preferredOsType) {
return preferredOsType(Either.ofLeft(preferredOsType));
}
/**
* @param preferredOsType The operating system type of the cloud shell. Deprecated, use preferredShellType.
*
* @return builder
*
*/
public Builder preferredOsType(OsType preferredOsType) {
return preferredOsType(Either.ofRight(preferredOsType));
}
/**
* @param preferredShellType The shell type of the cloud shell.
*
* @return builder
*
*/
public Builder preferredShellType(Output> preferredShellType) {
$.preferredShellType = preferredShellType;
return this;
}
/**
* @param preferredShellType The shell type of the cloud shell.
*
* @return builder
*
*/
public Builder preferredShellType(Either preferredShellType) {
return preferredShellType(Output.of(preferredShellType));
}
/**
* @param preferredShellType The shell type of the cloud shell.
*
* @return builder
*
*/
public Builder preferredShellType(String preferredShellType) {
return preferredShellType(Either.ofLeft(preferredShellType));
}
/**
* @param preferredShellType The shell type of the cloud shell.
*
* @return builder
*
*/
public Builder preferredShellType(ShellType preferredShellType) {
return preferredShellType(Either.ofRight(preferredShellType));
}
/**
* @param storageProfile The storage profile of the user settings.
*
* @return builder
*
*/
public Builder storageProfile(Output storageProfile) {
$.storageProfile = storageProfile;
return this;
}
/**
* @param storageProfile The storage profile of the user settings.
*
* @return builder
*
*/
public Builder storageProfile(StorageProfileArgs storageProfile) {
return storageProfile(Output.of(storageProfile));
}
/**
* @param terminalSettings Settings for terminal appearance.
*
* @return builder
*
*/
public Builder terminalSettings(Output terminalSettings) {
$.terminalSettings = terminalSettings;
return this;
}
/**
* @param terminalSettings Settings for terminal appearance.
*
* @return builder
*
*/
public Builder terminalSettings(TerminalSettingsArgs terminalSettings) {
return terminalSettings(Output.of(terminalSettings));
}
public UserPropertiesArgs build() {
if ($.preferredLocation == null) {
throw new MissingRequiredPropertyException("UserPropertiesArgs", "preferredLocation");
}
if ($.preferredOsType == null) {
throw new MissingRequiredPropertyException("UserPropertiesArgs", "preferredOsType");
}
if ($.preferredShellType == null) {
throw new MissingRequiredPropertyException("UserPropertiesArgs", "preferredShellType");
}
if ($.storageProfile == null) {
throw new MissingRequiredPropertyException("UserPropertiesArgs", "storageProfile");
}
if ($.terminalSettings == null) {
throw new MissingRequiredPropertyException("UserPropertiesArgs", "terminalSettings");
}
return $;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy