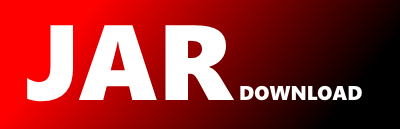
com.pulumi.azurenative.recoveryservices.inputs.HyperVReplicaAzureEnableProtectionInputArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.recoveryservices.inputs;
import com.pulumi.azurenative.recoveryservices.enums.DiskAccountType;
import com.pulumi.azurenative.recoveryservices.enums.LicenseType;
import com.pulumi.azurenative.recoveryservices.enums.SqlServerLicenseType;
import com.pulumi.azurenative.recoveryservices.inputs.HyperVReplicaAzureDiskInputDetailsArgs;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.core.internal.Codegen;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* HyperVReplicaAzure specific enable protection input.
*
*/
public final class HyperVReplicaAzureEnableProtectionInputArgs extends com.pulumi.resources.ResourceArgs {
public static final HyperVReplicaAzureEnableProtectionInputArgs Empty = new HyperVReplicaAzureEnableProtectionInputArgs();
/**
* The DiskEncryptionSet ARM Id.
*
*/
@Import(name="diskEncryptionSetId")
private @Nullable Output diskEncryptionSetId;
/**
* @return The DiskEncryptionSet ARM Id.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy