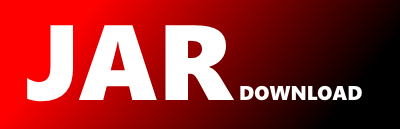
com.pulumi.azurenative.recoveryservices.outputs.AzureFabricSpecificDetailsResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.recoveryservices.outputs;
import com.pulumi.azurenative.recoveryservices.outputs.A2AExtendedLocationDetailsResponse;
import com.pulumi.azurenative.recoveryservices.outputs.A2AFabricSpecificLocationDetailsResponse;
import com.pulumi.azurenative.recoveryservices.outputs.A2AZoneDetailsResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class AzureFabricSpecificDetailsResponse {
/**
* @return The container Ids for the Azure fabric.
*
*/
private @Nullable List containerIds;
/**
* @return The ExtendedLocations.
*
*/
private @Nullable List extendedLocations;
/**
* @return Gets the class type. Overridden in derived classes.
* Expected value is 'Azure'.
*
*/
private String instanceType;
/**
* @return The Location for the Azure fabric.
*
*/
private @Nullable String location;
/**
* @return The location details.
*
*/
private @Nullable List locationDetails;
/**
* @return The zones.
*
*/
private @Nullable List zones;
private AzureFabricSpecificDetailsResponse() {}
/**
* @return The container Ids for the Azure fabric.
*
*/
public List containerIds() {
return this.containerIds == null ? List.of() : this.containerIds;
}
/**
* @return The ExtendedLocations.
*
*/
public List extendedLocations() {
return this.extendedLocations == null ? List.of() : this.extendedLocations;
}
/**
* @return Gets the class type. Overridden in derived classes.
* Expected value is 'Azure'.
*
*/
public String instanceType() {
return this.instanceType;
}
/**
* @return The Location for the Azure fabric.
*
*/
public Optional location() {
return Optional.ofNullable(this.location);
}
/**
* @return The location details.
*
*/
public List locationDetails() {
return this.locationDetails == null ? List.of() : this.locationDetails;
}
/**
* @return The zones.
*
*/
public List zones() {
return this.zones == null ? List.of() : this.zones;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(AzureFabricSpecificDetailsResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable List containerIds;
private @Nullable List extendedLocations;
private String instanceType;
private @Nullable String location;
private @Nullable List locationDetails;
private @Nullable List zones;
public Builder() {}
public Builder(AzureFabricSpecificDetailsResponse defaults) {
Objects.requireNonNull(defaults);
this.containerIds = defaults.containerIds;
this.extendedLocations = defaults.extendedLocations;
this.instanceType = defaults.instanceType;
this.location = defaults.location;
this.locationDetails = defaults.locationDetails;
this.zones = defaults.zones;
}
@CustomType.Setter
public Builder containerIds(@Nullable List containerIds) {
this.containerIds = containerIds;
return this;
}
public Builder containerIds(String... containerIds) {
return containerIds(List.of(containerIds));
}
@CustomType.Setter
public Builder extendedLocations(@Nullable List extendedLocations) {
this.extendedLocations = extendedLocations;
return this;
}
public Builder extendedLocations(A2AExtendedLocationDetailsResponse... extendedLocations) {
return extendedLocations(List.of(extendedLocations));
}
@CustomType.Setter
public Builder instanceType(String instanceType) {
if (instanceType == null) {
throw new MissingRequiredPropertyException("AzureFabricSpecificDetailsResponse", "instanceType");
}
this.instanceType = instanceType;
return this;
}
@CustomType.Setter
public Builder location(@Nullable String location) {
this.location = location;
return this;
}
@CustomType.Setter
public Builder locationDetails(@Nullable List locationDetails) {
this.locationDetails = locationDetails;
return this;
}
public Builder locationDetails(A2AFabricSpecificLocationDetailsResponse... locationDetails) {
return locationDetails(List.of(locationDetails));
}
@CustomType.Setter
public Builder zones(@Nullable List zones) {
this.zones = zones;
return this;
}
public Builder zones(A2AZoneDetailsResponse... zones) {
return zones(List.of(zones));
}
public AzureFabricSpecificDetailsResponse build() {
final var _resultValue = new AzureFabricSpecificDetailsResponse();
_resultValue.containerIds = containerIds;
_resultValue.extendedLocations = extendedLocations;
_resultValue.instanceType = instanceType;
_resultValue.location = location;
_resultValue.locationDetails = locationDetails;
_resultValue.zones = zones;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy