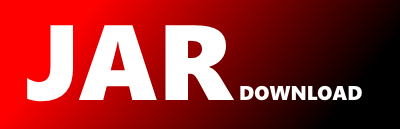
com.pulumi.azurenative.recoveryservices.outputs.AzureSqlProtectedItemResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.recoveryservices.outputs;
import com.pulumi.azurenative.recoveryservices.outputs.AzureSqlProtectedItemExtendedInfoResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class AzureSqlProtectedItemResponse {
/**
* @return Type of backup management for the backed up item.
*
*/
private String backupManagementType;
/**
* @return Name of the backup set the backup item belongs to
*
*/
private @Nullable String backupSetName;
/**
* @return Unique name of container
*
*/
private @Nullable String containerName;
/**
* @return Create mode to indicate recovery of existing soft deleted data source or creation of new data source.
*
*/
private @Nullable String createMode;
/**
* @return Time for deferred deletion in UTC
*
*/
private @Nullable String deferredDeleteTimeInUTC;
/**
* @return Time remaining before the DS marked for deferred delete is permanently deleted
*
*/
private @Nullable String deferredDeleteTimeRemaining;
/**
* @return Additional information for this backup item.
*
*/
private @Nullable AzureSqlProtectedItemExtendedInfoResponse extendedInfo;
/**
* @return Flag to identify whether datasource is protected in archive
*
*/
private @Nullable Boolean isArchiveEnabled;
/**
* @return Flag to identify whether the deferred deleted DS is to be purged soon
*
*/
private @Nullable Boolean isDeferredDeleteScheduleUpcoming;
/**
* @return Flag to identify that deferred deleted DS is to be moved into Pause state
*
*/
private @Nullable Boolean isRehydrate;
/**
* @return Flag to identify whether the DS is scheduled for deferred delete
*
*/
private @Nullable Boolean isScheduledForDeferredDelete;
/**
* @return Timestamp when the last (latest) backup copy was created for this backup item.
*
*/
private @Nullable String lastRecoveryPoint;
/**
* @return ID of the backup policy with which this item is backed up.
*
*/
private @Nullable String policyId;
/**
* @return Name of the policy used for protection
*
*/
private @Nullable String policyName;
/**
* @return Internal ID of a backup item. Used by Azure SQL Backup engine to contact Recovery Services.
*
*/
private @Nullable String protectedItemDataId;
/**
* @return backup item type.
* Expected value is 'Microsoft.Sql/servers/databases'.
*
*/
private String protectedItemType;
/**
* @return Backup state of the backed up item.
*
*/
private @Nullable String protectionState;
/**
* @return ResourceGuardOperationRequests on which LAC check will be performed
*
*/
private @Nullable List resourceGuardOperationRequests;
/**
* @return Soft delete retention period in days
*
*/
private @Nullable Integer softDeleteRetentionPeriodInDays;
/**
* @return ARM ID of the resource to be backed up.
*
*/
private @Nullable String sourceResourceId;
/**
* @return ID of the vault which protects this item
*
*/
private String vaultId;
/**
* @return Type of workload this item represents.
*
*/
private String workloadType;
private AzureSqlProtectedItemResponse() {}
/**
* @return Type of backup management for the backed up item.
*
*/
public String backupManagementType() {
return this.backupManagementType;
}
/**
* @return Name of the backup set the backup item belongs to
*
*/
public Optional backupSetName() {
return Optional.ofNullable(this.backupSetName);
}
/**
* @return Unique name of container
*
*/
public Optional containerName() {
return Optional.ofNullable(this.containerName);
}
/**
* @return Create mode to indicate recovery of existing soft deleted data source or creation of new data source.
*
*/
public Optional createMode() {
return Optional.ofNullable(this.createMode);
}
/**
* @return Time for deferred deletion in UTC
*
*/
public Optional deferredDeleteTimeInUTC() {
return Optional.ofNullable(this.deferredDeleteTimeInUTC);
}
/**
* @return Time remaining before the DS marked for deferred delete is permanently deleted
*
*/
public Optional deferredDeleteTimeRemaining() {
return Optional.ofNullable(this.deferredDeleteTimeRemaining);
}
/**
* @return Additional information for this backup item.
*
*/
public Optional extendedInfo() {
return Optional.ofNullable(this.extendedInfo);
}
/**
* @return Flag to identify whether datasource is protected in archive
*
*/
public Optional isArchiveEnabled() {
return Optional.ofNullable(this.isArchiveEnabled);
}
/**
* @return Flag to identify whether the deferred deleted DS is to be purged soon
*
*/
public Optional isDeferredDeleteScheduleUpcoming() {
return Optional.ofNullable(this.isDeferredDeleteScheduleUpcoming);
}
/**
* @return Flag to identify that deferred deleted DS is to be moved into Pause state
*
*/
public Optional isRehydrate() {
return Optional.ofNullable(this.isRehydrate);
}
/**
* @return Flag to identify whether the DS is scheduled for deferred delete
*
*/
public Optional isScheduledForDeferredDelete() {
return Optional.ofNullable(this.isScheduledForDeferredDelete);
}
/**
* @return Timestamp when the last (latest) backup copy was created for this backup item.
*
*/
public Optional lastRecoveryPoint() {
return Optional.ofNullable(this.lastRecoveryPoint);
}
/**
* @return ID of the backup policy with which this item is backed up.
*
*/
public Optional policyId() {
return Optional.ofNullable(this.policyId);
}
/**
* @return Name of the policy used for protection
*
*/
public Optional policyName() {
return Optional.ofNullable(this.policyName);
}
/**
* @return Internal ID of a backup item. Used by Azure SQL Backup engine to contact Recovery Services.
*
*/
public Optional protectedItemDataId() {
return Optional.ofNullable(this.protectedItemDataId);
}
/**
* @return backup item type.
* Expected value is 'Microsoft.Sql/servers/databases'.
*
*/
public String protectedItemType() {
return this.protectedItemType;
}
/**
* @return Backup state of the backed up item.
*
*/
public Optional protectionState() {
return Optional.ofNullable(this.protectionState);
}
/**
* @return ResourceGuardOperationRequests on which LAC check will be performed
*
*/
public List resourceGuardOperationRequests() {
return this.resourceGuardOperationRequests == null ? List.of() : this.resourceGuardOperationRequests;
}
/**
* @return Soft delete retention period in days
*
*/
public Optional softDeleteRetentionPeriodInDays() {
return Optional.ofNullable(this.softDeleteRetentionPeriodInDays);
}
/**
* @return ARM ID of the resource to be backed up.
*
*/
public Optional sourceResourceId() {
return Optional.ofNullable(this.sourceResourceId);
}
/**
* @return ID of the vault which protects this item
*
*/
public String vaultId() {
return this.vaultId;
}
/**
* @return Type of workload this item represents.
*
*/
public String workloadType() {
return this.workloadType;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(AzureSqlProtectedItemResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String backupManagementType;
private @Nullable String backupSetName;
private @Nullable String containerName;
private @Nullable String createMode;
private @Nullable String deferredDeleteTimeInUTC;
private @Nullable String deferredDeleteTimeRemaining;
private @Nullable AzureSqlProtectedItemExtendedInfoResponse extendedInfo;
private @Nullable Boolean isArchiveEnabled;
private @Nullable Boolean isDeferredDeleteScheduleUpcoming;
private @Nullable Boolean isRehydrate;
private @Nullable Boolean isScheduledForDeferredDelete;
private @Nullable String lastRecoveryPoint;
private @Nullable String policyId;
private @Nullable String policyName;
private @Nullable String protectedItemDataId;
private String protectedItemType;
private @Nullable String protectionState;
private @Nullable List resourceGuardOperationRequests;
private @Nullable Integer softDeleteRetentionPeriodInDays;
private @Nullable String sourceResourceId;
private String vaultId;
private String workloadType;
public Builder() {}
public Builder(AzureSqlProtectedItemResponse defaults) {
Objects.requireNonNull(defaults);
this.backupManagementType = defaults.backupManagementType;
this.backupSetName = defaults.backupSetName;
this.containerName = defaults.containerName;
this.createMode = defaults.createMode;
this.deferredDeleteTimeInUTC = defaults.deferredDeleteTimeInUTC;
this.deferredDeleteTimeRemaining = defaults.deferredDeleteTimeRemaining;
this.extendedInfo = defaults.extendedInfo;
this.isArchiveEnabled = defaults.isArchiveEnabled;
this.isDeferredDeleteScheduleUpcoming = defaults.isDeferredDeleteScheduleUpcoming;
this.isRehydrate = defaults.isRehydrate;
this.isScheduledForDeferredDelete = defaults.isScheduledForDeferredDelete;
this.lastRecoveryPoint = defaults.lastRecoveryPoint;
this.policyId = defaults.policyId;
this.policyName = defaults.policyName;
this.protectedItemDataId = defaults.protectedItemDataId;
this.protectedItemType = defaults.protectedItemType;
this.protectionState = defaults.protectionState;
this.resourceGuardOperationRequests = defaults.resourceGuardOperationRequests;
this.softDeleteRetentionPeriodInDays = defaults.softDeleteRetentionPeriodInDays;
this.sourceResourceId = defaults.sourceResourceId;
this.vaultId = defaults.vaultId;
this.workloadType = defaults.workloadType;
}
@CustomType.Setter
public Builder backupManagementType(String backupManagementType) {
if (backupManagementType == null) {
throw new MissingRequiredPropertyException("AzureSqlProtectedItemResponse", "backupManagementType");
}
this.backupManagementType = backupManagementType;
return this;
}
@CustomType.Setter
public Builder backupSetName(@Nullable String backupSetName) {
this.backupSetName = backupSetName;
return this;
}
@CustomType.Setter
public Builder containerName(@Nullable String containerName) {
this.containerName = containerName;
return this;
}
@CustomType.Setter
public Builder createMode(@Nullable String createMode) {
this.createMode = createMode;
return this;
}
@CustomType.Setter
public Builder deferredDeleteTimeInUTC(@Nullable String deferredDeleteTimeInUTC) {
this.deferredDeleteTimeInUTC = deferredDeleteTimeInUTC;
return this;
}
@CustomType.Setter
public Builder deferredDeleteTimeRemaining(@Nullable String deferredDeleteTimeRemaining) {
this.deferredDeleteTimeRemaining = deferredDeleteTimeRemaining;
return this;
}
@CustomType.Setter
public Builder extendedInfo(@Nullable AzureSqlProtectedItemExtendedInfoResponse extendedInfo) {
this.extendedInfo = extendedInfo;
return this;
}
@CustomType.Setter
public Builder isArchiveEnabled(@Nullable Boolean isArchiveEnabled) {
this.isArchiveEnabled = isArchiveEnabled;
return this;
}
@CustomType.Setter
public Builder isDeferredDeleteScheduleUpcoming(@Nullable Boolean isDeferredDeleteScheduleUpcoming) {
this.isDeferredDeleteScheduleUpcoming = isDeferredDeleteScheduleUpcoming;
return this;
}
@CustomType.Setter
public Builder isRehydrate(@Nullable Boolean isRehydrate) {
this.isRehydrate = isRehydrate;
return this;
}
@CustomType.Setter
public Builder isScheduledForDeferredDelete(@Nullable Boolean isScheduledForDeferredDelete) {
this.isScheduledForDeferredDelete = isScheduledForDeferredDelete;
return this;
}
@CustomType.Setter
public Builder lastRecoveryPoint(@Nullable String lastRecoveryPoint) {
this.lastRecoveryPoint = lastRecoveryPoint;
return this;
}
@CustomType.Setter
public Builder policyId(@Nullable String policyId) {
this.policyId = policyId;
return this;
}
@CustomType.Setter
public Builder policyName(@Nullable String policyName) {
this.policyName = policyName;
return this;
}
@CustomType.Setter
public Builder protectedItemDataId(@Nullable String protectedItemDataId) {
this.protectedItemDataId = protectedItemDataId;
return this;
}
@CustomType.Setter
public Builder protectedItemType(String protectedItemType) {
if (protectedItemType == null) {
throw new MissingRequiredPropertyException("AzureSqlProtectedItemResponse", "protectedItemType");
}
this.protectedItemType = protectedItemType;
return this;
}
@CustomType.Setter
public Builder protectionState(@Nullable String protectionState) {
this.protectionState = protectionState;
return this;
}
@CustomType.Setter
public Builder resourceGuardOperationRequests(@Nullable List resourceGuardOperationRequests) {
this.resourceGuardOperationRequests = resourceGuardOperationRequests;
return this;
}
public Builder resourceGuardOperationRequests(String... resourceGuardOperationRequests) {
return resourceGuardOperationRequests(List.of(resourceGuardOperationRequests));
}
@CustomType.Setter
public Builder softDeleteRetentionPeriodInDays(@Nullable Integer softDeleteRetentionPeriodInDays) {
this.softDeleteRetentionPeriodInDays = softDeleteRetentionPeriodInDays;
return this;
}
@CustomType.Setter
public Builder sourceResourceId(@Nullable String sourceResourceId) {
this.sourceResourceId = sourceResourceId;
return this;
}
@CustomType.Setter
public Builder vaultId(String vaultId) {
if (vaultId == null) {
throw new MissingRequiredPropertyException("AzureSqlProtectedItemResponse", "vaultId");
}
this.vaultId = vaultId;
return this;
}
@CustomType.Setter
public Builder workloadType(String workloadType) {
if (workloadType == null) {
throw new MissingRequiredPropertyException("AzureSqlProtectedItemResponse", "workloadType");
}
this.workloadType = workloadType;
return this;
}
public AzureSqlProtectedItemResponse build() {
final var _resultValue = new AzureSqlProtectedItemResponse();
_resultValue.backupManagementType = backupManagementType;
_resultValue.backupSetName = backupSetName;
_resultValue.containerName = containerName;
_resultValue.createMode = createMode;
_resultValue.deferredDeleteTimeInUTC = deferredDeleteTimeInUTC;
_resultValue.deferredDeleteTimeRemaining = deferredDeleteTimeRemaining;
_resultValue.extendedInfo = extendedInfo;
_resultValue.isArchiveEnabled = isArchiveEnabled;
_resultValue.isDeferredDeleteScheduleUpcoming = isDeferredDeleteScheduleUpcoming;
_resultValue.isRehydrate = isRehydrate;
_resultValue.isScheduledForDeferredDelete = isScheduledForDeferredDelete;
_resultValue.lastRecoveryPoint = lastRecoveryPoint;
_resultValue.policyId = policyId;
_resultValue.policyName = policyName;
_resultValue.protectedItemDataId = protectedItemDataId;
_resultValue.protectedItemType = protectedItemType;
_resultValue.protectionState = protectionState;
_resultValue.resourceGuardOperationRequests = resourceGuardOperationRequests;
_resultValue.softDeleteRetentionPeriodInDays = softDeleteRetentionPeriodInDays;
_resultValue.sourceResourceId = sourceResourceId;
_resultValue.vaultId = vaultId;
_resultValue.workloadType = workloadType;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy