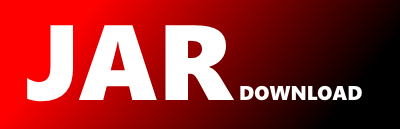
com.pulumi.azurenative.recoveryservices.outputs.InMageAzureV2ProtectedDiskDetailsResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.recoveryservices.outputs;
import com.pulumi.core.annotations.CustomType;
import java.lang.Double;
import java.lang.Integer;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class InMageAzureV2ProtectedDiskDetailsResponse {
/**
* @return The disk capacity in bytes.
*
*/
private @Nullable Double diskCapacityInBytes;
/**
* @return The disk id.
*
*/
private @Nullable String diskId;
/**
* @return The disk name.
*
*/
private @Nullable String diskName;
/**
* @return A value indicating whether disk is resized.
*
*/
private @Nullable String diskResized;
/**
* @return The disk file system capacity in bytes.
*
*/
private @Nullable Double fileSystemCapacityInBytes;
/**
* @return The health error code for the disk.
*
*/
private @Nullable String healthErrorCode;
/**
* @return The last RPO calculated time.
*
*/
private @Nullable String lastRpoCalculatedTime;
/**
* @return The Progress Health.
*
*/
private @Nullable String progressHealth;
/**
* @return The Progress Status.
*
*/
private @Nullable String progressStatus;
/**
* @return The protection stage.
*
*/
private @Nullable String protectionStage;
/**
* @return The PS data transit in MB.
*
*/
private @Nullable Double psDataInMegaBytes;
/**
* @return The resync duration in seconds.
*
*/
private @Nullable Double resyncDurationInSeconds;
/**
* @return The resync last 15 minutes transferred bytes.
*
*/
private @Nullable Double resyncLast15MinutesTransferredBytes;
/**
* @return The last data transfer time in UTC.
*
*/
private @Nullable String resyncLastDataTransferTimeUTC;
/**
* @return The resync processed bytes.
*
*/
private @Nullable Double resyncProcessedBytes;
/**
* @return The resync progress percentage.
*
*/
private @Nullable Integer resyncProgressPercentage;
/**
* @return A value indicating whether resync is required for this disk.
*
*/
private @Nullable String resyncRequired;
/**
* @return The resync start time.
*
*/
private @Nullable String resyncStartTime;
/**
* @return The resync total transferred bytes.
*
*/
private @Nullable Double resyncTotalTransferredBytes;
/**
* @return The RPO in seconds.
*
*/
private @Nullable Double rpoInSeconds;
/**
* @return The seconds to take for switch provider.
*
*/
private @Nullable Double secondsToTakeSwitchProvider;
/**
* @return The source data transit in MB.
*
*/
private @Nullable Double sourceDataInMegaBytes;
/**
* @return The target data transit in MB.
*
*/
private @Nullable Double targetDataInMegaBytes;
private InMageAzureV2ProtectedDiskDetailsResponse() {}
/**
* @return The disk capacity in bytes.
*
*/
public Optional diskCapacityInBytes() {
return Optional.ofNullable(this.diskCapacityInBytes);
}
/**
* @return The disk id.
*
*/
public Optional diskId() {
return Optional.ofNullable(this.diskId);
}
/**
* @return The disk name.
*
*/
public Optional diskName() {
return Optional.ofNullable(this.diskName);
}
/**
* @return A value indicating whether disk is resized.
*
*/
public Optional diskResized() {
return Optional.ofNullable(this.diskResized);
}
/**
* @return The disk file system capacity in bytes.
*
*/
public Optional fileSystemCapacityInBytes() {
return Optional.ofNullable(this.fileSystemCapacityInBytes);
}
/**
* @return The health error code for the disk.
*
*/
public Optional healthErrorCode() {
return Optional.ofNullable(this.healthErrorCode);
}
/**
* @return The last RPO calculated time.
*
*/
public Optional lastRpoCalculatedTime() {
return Optional.ofNullable(this.lastRpoCalculatedTime);
}
/**
* @return The Progress Health.
*
*/
public Optional progressHealth() {
return Optional.ofNullable(this.progressHealth);
}
/**
* @return The Progress Status.
*
*/
public Optional progressStatus() {
return Optional.ofNullable(this.progressStatus);
}
/**
* @return The protection stage.
*
*/
public Optional protectionStage() {
return Optional.ofNullable(this.protectionStage);
}
/**
* @return The PS data transit in MB.
*
*/
public Optional psDataInMegaBytes() {
return Optional.ofNullable(this.psDataInMegaBytes);
}
/**
* @return The resync duration in seconds.
*
*/
public Optional resyncDurationInSeconds() {
return Optional.ofNullable(this.resyncDurationInSeconds);
}
/**
* @return The resync last 15 minutes transferred bytes.
*
*/
public Optional resyncLast15MinutesTransferredBytes() {
return Optional.ofNullable(this.resyncLast15MinutesTransferredBytes);
}
/**
* @return The last data transfer time in UTC.
*
*/
public Optional resyncLastDataTransferTimeUTC() {
return Optional.ofNullable(this.resyncLastDataTransferTimeUTC);
}
/**
* @return The resync processed bytes.
*
*/
public Optional resyncProcessedBytes() {
return Optional.ofNullable(this.resyncProcessedBytes);
}
/**
* @return The resync progress percentage.
*
*/
public Optional resyncProgressPercentage() {
return Optional.ofNullable(this.resyncProgressPercentage);
}
/**
* @return A value indicating whether resync is required for this disk.
*
*/
public Optional resyncRequired() {
return Optional.ofNullable(this.resyncRequired);
}
/**
* @return The resync start time.
*
*/
public Optional resyncStartTime() {
return Optional.ofNullable(this.resyncStartTime);
}
/**
* @return The resync total transferred bytes.
*
*/
public Optional resyncTotalTransferredBytes() {
return Optional.ofNullable(this.resyncTotalTransferredBytes);
}
/**
* @return The RPO in seconds.
*
*/
public Optional rpoInSeconds() {
return Optional.ofNullable(this.rpoInSeconds);
}
/**
* @return The seconds to take for switch provider.
*
*/
public Optional secondsToTakeSwitchProvider() {
return Optional.ofNullable(this.secondsToTakeSwitchProvider);
}
/**
* @return The source data transit in MB.
*
*/
public Optional sourceDataInMegaBytes() {
return Optional.ofNullable(this.sourceDataInMegaBytes);
}
/**
* @return The target data transit in MB.
*
*/
public Optional targetDataInMegaBytes() {
return Optional.ofNullable(this.targetDataInMegaBytes);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(InMageAzureV2ProtectedDiskDetailsResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable Double diskCapacityInBytes;
private @Nullable String diskId;
private @Nullable String diskName;
private @Nullable String diskResized;
private @Nullable Double fileSystemCapacityInBytes;
private @Nullable String healthErrorCode;
private @Nullable String lastRpoCalculatedTime;
private @Nullable String progressHealth;
private @Nullable String progressStatus;
private @Nullable String protectionStage;
private @Nullable Double psDataInMegaBytes;
private @Nullable Double resyncDurationInSeconds;
private @Nullable Double resyncLast15MinutesTransferredBytes;
private @Nullable String resyncLastDataTransferTimeUTC;
private @Nullable Double resyncProcessedBytes;
private @Nullable Integer resyncProgressPercentage;
private @Nullable String resyncRequired;
private @Nullable String resyncStartTime;
private @Nullable Double resyncTotalTransferredBytes;
private @Nullable Double rpoInSeconds;
private @Nullable Double secondsToTakeSwitchProvider;
private @Nullable Double sourceDataInMegaBytes;
private @Nullable Double targetDataInMegaBytes;
public Builder() {}
public Builder(InMageAzureV2ProtectedDiskDetailsResponse defaults) {
Objects.requireNonNull(defaults);
this.diskCapacityInBytes = defaults.diskCapacityInBytes;
this.diskId = defaults.diskId;
this.diskName = defaults.diskName;
this.diskResized = defaults.diskResized;
this.fileSystemCapacityInBytes = defaults.fileSystemCapacityInBytes;
this.healthErrorCode = defaults.healthErrorCode;
this.lastRpoCalculatedTime = defaults.lastRpoCalculatedTime;
this.progressHealth = defaults.progressHealth;
this.progressStatus = defaults.progressStatus;
this.protectionStage = defaults.protectionStage;
this.psDataInMegaBytes = defaults.psDataInMegaBytes;
this.resyncDurationInSeconds = defaults.resyncDurationInSeconds;
this.resyncLast15MinutesTransferredBytes = defaults.resyncLast15MinutesTransferredBytes;
this.resyncLastDataTransferTimeUTC = defaults.resyncLastDataTransferTimeUTC;
this.resyncProcessedBytes = defaults.resyncProcessedBytes;
this.resyncProgressPercentage = defaults.resyncProgressPercentage;
this.resyncRequired = defaults.resyncRequired;
this.resyncStartTime = defaults.resyncStartTime;
this.resyncTotalTransferredBytes = defaults.resyncTotalTransferredBytes;
this.rpoInSeconds = defaults.rpoInSeconds;
this.secondsToTakeSwitchProvider = defaults.secondsToTakeSwitchProvider;
this.sourceDataInMegaBytes = defaults.sourceDataInMegaBytes;
this.targetDataInMegaBytes = defaults.targetDataInMegaBytes;
}
@CustomType.Setter
public Builder diskCapacityInBytes(@Nullable Double diskCapacityInBytes) {
this.diskCapacityInBytes = diskCapacityInBytes;
return this;
}
@CustomType.Setter
public Builder diskId(@Nullable String diskId) {
this.diskId = diskId;
return this;
}
@CustomType.Setter
public Builder diskName(@Nullable String diskName) {
this.diskName = diskName;
return this;
}
@CustomType.Setter
public Builder diskResized(@Nullable String diskResized) {
this.diskResized = diskResized;
return this;
}
@CustomType.Setter
public Builder fileSystemCapacityInBytes(@Nullable Double fileSystemCapacityInBytes) {
this.fileSystemCapacityInBytes = fileSystemCapacityInBytes;
return this;
}
@CustomType.Setter
public Builder healthErrorCode(@Nullable String healthErrorCode) {
this.healthErrorCode = healthErrorCode;
return this;
}
@CustomType.Setter
public Builder lastRpoCalculatedTime(@Nullable String lastRpoCalculatedTime) {
this.lastRpoCalculatedTime = lastRpoCalculatedTime;
return this;
}
@CustomType.Setter
public Builder progressHealth(@Nullable String progressHealth) {
this.progressHealth = progressHealth;
return this;
}
@CustomType.Setter
public Builder progressStatus(@Nullable String progressStatus) {
this.progressStatus = progressStatus;
return this;
}
@CustomType.Setter
public Builder protectionStage(@Nullable String protectionStage) {
this.protectionStage = protectionStage;
return this;
}
@CustomType.Setter
public Builder psDataInMegaBytes(@Nullable Double psDataInMegaBytes) {
this.psDataInMegaBytes = psDataInMegaBytes;
return this;
}
@CustomType.Setter
public Builder resyncDurationInSeconds(@Nullable Double resyncDurationInSeconds) {
this.resyncDurationInSeconds = resyncDurationInSeconds;
return this;
}
@CustomType.Setter
public Builder resyncLast15MinutesTransferredBytes(@Nullable Double resyncLast15MinutesTransferredBytes) {
this.resyncLast15MinutesTransferredBytes = resyncLast15MinutesTransferredBytes;
return this;
}
@CustomType.Setter
public Builder resyncLastDataTransferTimeUTC(@Nullable String resyncLastDataTransferTimeUTC) {
this.resyncLastDataTransferTimeUTC = resyncLastDataTransferTimeUTC;
return this;
}
@CustomType.Setter
public Builder resyncProcessedBytes(@Nullable Double resyncProcessedBytes) {
this.resyncProcessedBytes = resyncProcessedBytes;
return this;
}
@CustomType.Setter
public Builder resyncProgressPercentage(@Nullable Integer resyncProgressPercentage) {
this.resyncProgressPercentage = resyncProgressPercentage;
return this;
}
@CustomType.Setter
public Builder resyncRequired(@Nullable String resyncRequired) {
this.resyncRequired = resyncRequired;
return this;
}
@CustomType.Setter
public Builder resyncStartTime(@Nullable String resyncStartTime) {
this.resyncStartTime = resyncStartTime;
return this;
}
@CustomType.Setter
public Builder resyncTotalTransferredBytes(@Nullable Double resyncTotalTransferredBytes) {
this.resyncTotalTransferredBytes = resyncTotalTransferredBytes;
return this;
}
@CustomType.Setter
public Builder rpoInSeconds(@Nullable Double rpoInSeconds) {
this.rpoInSeconds = rpoInSeconds;
return this;
}
@CustomType.Setter
public Builder secondsToTakeSwitchProvider(@Nullable Double secondsToTakeSwitchProvider) {
this.secondsToTakeSwitchProvider = secondsToTakeSwitchProvider;
return this;
}
@CustomType.Setter
public Builder sourceDataInMegaBytes(@Nullable Double sourceDataInMegaBytes) {
this.sourceDataInMegaBytes = sourceDataInMegaBytes;
return this;
}
@CustomType.Setter
public Builder targetDataInMegaBytes(@Nullable Double targetDataInMegaBytes) {
this.targetDataInMegaBytes = targetDataInMegaBytes;
return this;
}
public InMageAzureV2ProtectedDiskDetailsResponse build() {
final var _resultValue = new InMageAzureV2ProtectedDiskDetailsResponse();
_resultValue.diskCapacityInBytes = diskCapacityInBytes;
_resultValue.diskId = diskId;
_resultValue.diskName = diskName;
_resultValue.diskResized = diskResized;
_resultValue.fileSystemCapacityInBytes = fileSystemCapacityInBytes;
_resultValue.healthErrorCode = healthErrorCode;
_resultValue.lastRpoCalculatedTime = lastRpoCalculatedTime;
_resultValue.progressHealth = progressHealth;
_resultValue.progressStatus = progressStatus;
_resultValue.protectionStage = protectionStage;
_resultValue.psDataInMegaBytes = psDataInMegaBytes;
_resultValue.resyncDurationInSeconds = resyncDurationInSeconds;
_resultValue.resyncLast15MinutesTransferredBytes = resyncLast15MinutesTransferredBytes;
_resultValue.resyncLastDataTransferTimeUTC = resyncLastDataTransferTimeUTC;
_resultValue.resyncProcessedBytes = resyncProcessedBytes;
_resultValue.resyncProgressPercentage = resyncProgressPercentage;
_resultValue.resyncRequired = resyncRequired;
_resultValue.resyncStartTime = resyncStartTime;
_resultValue.resyncTotalTransferredBytes = resyncTotalTransferredBytes;
_resultValue.rpoInSeconds = rpoInSeconds;
_resultValue.secondsToTakeSwitchProvider = secondsToTakeSwitchProvider;
_resultValue.sourceDataInMegaBytes = sourceDataInMegaBytes;
_resultValue.targetDataInMegaBytes = targetDataInMegaBytes;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy