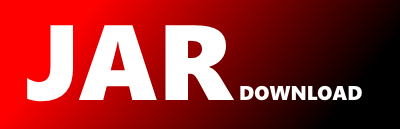
com.pulumi.azurenative.recoveryservices.outputs.InMageRcmFailbackReplicationDetailsResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.recoveryservices.outputs;
import com.pulumi.azurenative.recoveryservices.outputs.InMageRcmFailbackDiscoveredProtectedVmDetailsResponse;
import com.pulumi.azurenative.recoveryservices.outputs.InMageRcmFailbackMobilityAgentDetailsResponse;
import com.pulumi.azurenative.recoveryservices.outputs.InMageRcmFailbackNicDetailsResponse;
import com.pulumi.azurenative.recoveryservices.outputs.InMageRcmFailbackProtectedDiskDetailsResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.Double;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class InMageRcmFailbackReplicationDetailsResponse {
/**
* @return The ARM Id of the azure VM.
*
*/
private String azureVirtualMachineId;
/**
* @return The discovered VM information.
*
*/
private @Nullable InMageRcmFailbackDiscoveredProtectedVmDetailsResponse discoveredVmDetails;
/**
* @return The initial replication processed bytes. This includes sum of total bytes transferred and matched bytes on all selected disks in source VM.
*
*/
private Double initialReplicationProcessedBytes;
/**
* @return The initial replication progress health.
*
*/
private String initialReplicationProgressHealth;
/**
* @return The initial replication progress percentage.
*
*/
private Integer initialReplicationProgressPercentage;
/**
* @return The initial replication transferred bytes from source VM to target for all selected disks on source VM.
*
*/
private Double initialReplicationTransferredBytes;
/**
* @return Gets the Instance type.
* Expected value is 'InMageRcmFailback'.
*
*/
private String instanceType;
/**
* @return The virtual machine internal identifier.
*
*/
private String internalIdentifier;
/**
* @return A value indicating whether agent registration was successful after failover.
*
*/
private Boolean isAgentRegistrationSuccessfulAfterFailover;
/**
* @return The last planned failover start time.
*
*/
private String lastPlannedFailoverStartTime;
/**
* @return The last planned failover status.
*
*/
private String lastPlannedFailoverStatus;
/**
* @return The policy friendly name used by the forward replication.
*
*/
private String lastUsedPolicyFriendlyName;
/**
* @return The policy Id used by the forward replication.
*
*/
private String lastUsedPolicyId;
/**
* @return The log storage account ARM Id.
*
*/
private String logStorageAccountId;
/**
* @return The mobility agent information.
*
*/
private @Nullable InMageRcmFailbackMobilityAgentDetailsResponse mobilityAgentDetails;
/**
* @return The multi VM group name.
*
*/
private String multiVmGroupName;
/**
* @return The type of the OS on the VM.
*
*/
private String osType;
/**
* @return The list of protected disks.
*
*/
private @Nullable List protectedDisks;
/**
* @return The reprotect agent Id.
*
*/
private String reprotectAgentId;
/**
* @return The reprotect agent name.
*
*/
private String reprotectAgentName;
/**
* @return The resync processed bytes. This includes sum of total bytes transferred and matched bytes on all selected disks in source VM.
*
*/
private Double resyncProcessedBytes;
/**
* @return The resync progress health.
*
*/
private String resyncProgressHealth;
/**
* @return The resync progress percentage.
*
*/
private Integer resyncProgressPercentage;
/**
* @return A value indicating whether resync is required.
*
*/
private String resyncRequired;
/**
* @return The resync state.
*
*/
private String resyncState;
/**
* @return The resync transferred bytes from source VM to target for all selected disks on source VM.
*
*/
private Double resyncTransferredBytes;
/**
* @return The target datastore name.
*
*/
private String targetDataStoreName;
/**
* @return The target VM name.
*
*/
private String targetVmName;
/**
* @return The target vCenter Id.
*
*/
private String targetvCenterId;
/**
* @return The network details.
*
*/
private @Nullable List vmNics;
private InMageRcmFailbackReplicationDetailsResponse() {}
/**
* @return The ARM Id of the azure VM.
*
*/
public String azureVirtualMachineId() {
return this.azureVirtualMachineId;
}
/**
* @return The discovered VM information.
*
*/
public Optional discoveredVmDetails() {
return Optional.ofNullable(this.discoveredVmDetails);
}
/**
* @return The initial replication processed bytes. This includes sum of total bytes transferred and matched bytes on all selected disks in source VM.
*
*/
public Double initialReplicationProcessedBytes() {
return this.initialReplicationProcessedBytes;
}
/**
* @return The initial replication progress health.
*
*/
public String initialReplicationProgressHealth() {
return this.initialReplicationProgressHealth;
}
/**
* @return The initial replication progress percentage.
*
*/
public Integer initialReplicationProgressPercentage() {
return this.initialReplicationProgressPercentage;
}
/**
* @return The initial replication transferred bytes from source VM to target for all selected disks on source VM.
*
*/
public Double initialReplicationTransferredBytes() {
return this.initialReplicationTransferredBytes;
}
/**
* @return Gets the Instance type.
* Expected value is 'InMageRcmFailback'.
*
*/
public String instanceType() {
return this.instanceType;
}
/**
* @return The virtual machine internal identifier.
*
*/
public String internalIdentifier() {
return this.internalIdentifier;
}
/**
* @return A value indicating whether agent registration was successful after failover.
*
*/
public Boolean isAgentRegistrationSuccessfulAfterFailover() {
return this.isAgentRegistrationSuccessfulAfterFailover;
}
/**
* @return The last planned failover start time.
*
*/
public String lastPlannedFailoverStartTime() {
return this.lastPlannedFailoverStartTime;
}
/**
* @return The last planned failover status.
*
*/
public String lastPlannedFailoverStatus() {
return this.lastPlannedFailoverStatus;
}
/**
* @return The policy friendly name used by the forward replication.
*
*/
public String lastUsedPolicyFriendlyName() {
return this.lastUsedPolicyFriendlyName;
}
/**
* @return The policy Id used by the forward replication.
*
*/
public String lastUsedPolicyId() {
return this.lastUsedPolicyId;
}
/**
* @return The log storage account ARM Id.
*
*/
public String logStorageAccountId() {
return this.logStorageAccountId;
}
/**
* @return The mobility agent information.
*
*/
public Optional mobilityAgentDetails() {
return Optional.ofNullable(this.mobilityAgentDetails);
}
/**
* @return The multi VM group name.
*
*/
public String multiVmGroupName() {
return this.multiVmGroupName;
}
/**
* @return The type of the OS on the VM.
*
*/
public String osType() {
return this.osType;
}
/**
* @return The list of protected disks.
*
*/
public List protectedDisks() {
return this.protectedDisks == null ? List.of() : this.protectedDisks;
}
/**
* @return The reprotect agent Id.
*
*/
public String reprotectAgentId() {
return this.reprotectAgentId;
}
/**
* @return The reprotect agent name.
*
*/
public String reprotectAgentName() {
return this.reprotectAgentName;
}
/**
* @return The resync processed bytes. This includes sum of total bytes transferred and matched bytes on all selected disks in source VM.
*
*/
public Double resyncProcessedBytes() {
return this.resyncProcessedBytes;
}
/**
* @return The resync progress health.
*
*/
public String resyncProgressHealth() {
return this.resyncProgressHealth;
}
/**
* @return The resync progress percentage.
*
*/
public Integer resyncProgressPercentage() {
return this.resyncProgressPercentage;
}
/**
* @return A value indicating whether resync is required.
*
*/
public String resyncRequired() {
return this.resyncRequired;
}
/**
* @return The resync state.
*
*/
public String resyncState() {
return this.resyncState;
}
/**
* @return The resync transferred bytes from source VM to target for all selected disks on source VM.
*
*/
public Double resyncTransferredBytes() {
return this.resyncTransferredBytes;
}
/**
* @return The target datastore name.
*
*/
public String targetDataStoreName() {
return this.targetDataStoreName;
}
/**
* @return The target VM name.
*
*/
public String targetVmName() {
return this.targetVmName;
}
/**
* @return The target vCenter Id.
*
*/
public String targetvCenterId() {
return this.targetvCenterId;
}
/**
* @return The network details.
*
*/
public List vmNics() {
return this.vmNics == null ? List.of() : this.vmNics;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(InMageRcmFailbackReplicationDetailsResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String azureVirtualMachineId;
private @Nullable InMageRcmFailbackDiscoveredProtectedVmDetailsResponse discoveredVmDetails;
private Double initialReplicationProcessedBytes;
private String initialReplicationProgressHealth;
private Integer initialReplicationProgressPercentage;
private Double initialReplicationTransferredBytes;
private String instanceType;
private String internalIdentifier;
private Boolean isAgentRegistrationSuccessfulAfterFailover;
private String lastPlannedFailoverStartTime;
private String lastPlannedFailoverStatus;
private String lastUsedPolicyFriendlyName;
private String lastUsedPolicyId;
private String logStorageAccountId;
private @Nullable InMageRcmFailbackMobilityAgentDetailsResponse mobilityAgentDetails;
private String multiVmGroupName;
private String osType;
private @Nullable List protectedDisks;
private String reprotectAgentId;
private String reprotectAgentName;
private Double resyncProcessedBytes;
private String resyncProgressHealth;
private Integer resyncProgressPercentage;
private String resyncRequired;
private String resyncState;
private Double resyncTransferredBytes;
private String targetDataStoreName;
private String targetVmName;
private String targetvCenterId;
private @Nullable List vmNics;
public Builder() {}
public Builder(InMageRcmFailbackReplicationDetailsResponse defaults) {
Objects.requireNonNull(defaults);
this.azureVirtualMachineId = defaults.azureVirtualMachineId;
this.discoveredVmDetails = defaults.discoveredVmDetails;
this.initialReplicationProcessedBytes = defaults.initialReplicationProcessedBytes;
this.initialReplicationProgressHealth = defaults.initialReplicationProgressHealth;
this.initialReplicationProgressPercentage = defaults.initialReplicationProgressPercentage;
this.initialReplicationTransferredBytes = defaults.initialReplicationTransferredBytes;
this.instanceType = defaults.instanceType;
this.internalIdentifier = defaults.internalIdentifier;
this.isAgentRegistrationSuccessfulAfterFailover = defaults.isAgentRegistrationSuccessfulAfterFailover;
this.lastPlannedFailoverStartTime = defaults.lastPlannedFailoverStartTime;
this.lastPlannedFailoverStatus = defaults.lastPlannedFailoverStatus;
this.lastUsedPolicyFriendlyName = defaults.lastUsedPolicyFriendlyName;
this.lastUsedPolicyId = defaults.lastUsedPolicyId;
this.logStorageAccountId = defaults.logStorageAccountId;
this.mobilityAgentDetails = defaults.mobilityAgentDetails;
this.multiVmGroupName = defaults.multiVmGroupName;
this.osType = defaults.osType;
this.protectedDisks = defaults.protectedDisks;
this.reprotectAgentId = defaults.reprotectAgentId;
this.reprotectAgentName = defaults.reprotectAgentName;
this.resyncProcessedBytes = defaults.resyncProcessedBytes;
this.resyncProgressHealth = defaults.resyncProgressHealth;
this.resyncProgressPercentage = defaults.resyncProgressPercentage;
this.resyncRequired = defaults.resyncRequired;
this.resyncState = defaults.resyncState;
this.resyncTransferredBytes = defaults.resyncTransferredBytes;
this.targetDataStoreName = defaults.targetDataStoreName;
this.targetVmName = defaults.targetVmName;
this.targetvCenterId = defaults.targetvCenterId;
this.vmNics = defaults.vmNics;
}
@CustomType.Setter
public Builder azureVirtualMachineId(String azureVirtualMachineId) {
if (azureVirtualMachineId == null) {
throw new MissingRequiredPropertyException("InMageRcmFailbackReplicationDetailsResponse", "azureVirtualMachineId");
}
this.azureVirtualMachineId = azureVirtualMachineId;
return this;
}
@CustomType.Setter
public Builder discoveredVmDetails(@Nullable InMageRcmFailbackDiscoveredProtectedVmDetailsResponse discoveredVmDetails) {
this.discoveredVmDetails = discoveredVmDetails;
return this;
}
@CustomType.Setter
public Builder initialReplicationProcessedBytes(Double initialReplicationProcessedBytes) {
if (initialReplicationProcessedBytes == null) {
throw new MissingRequiredPropertyException("InMageRcmFailbackReplicationDetailsResponse", "initialReplicationProcessedBytes");
}
this.initialReplicationProcessedBytes = initialReplicationProcessedBytes;
return this;
}
@CustomType.Setter
public Builder initialReplicationProgressHealth(String initialReplicationProgressHealth) {
if (initialReplicationProgressHealth == null) {
throw new MissingRequiredPropertyException("InMageRcmFailbackReplicationDetailsResponse", "initialReplicationProgressHealth");
}
this.initialReplicationProgressHealth = initialReplicationProgressHealth;
return this;
}
@CustomType.Setter
public Builder initialReplicationProgressPercentage(Integer initialReplicationProgressPercentage) {
if (initialReplicationProgressPercentage == null) {
throw new MissingRequiredPropertyException("InMageRcmFailbackReplicationDetailsResponse", "initialReplicationProgressPercentage");
}
this.initialReplicationProgressPercentage = initialReplicationProgressPercentage;
return this;
}
@CustomType.Setter
public Builder initialReplicationTransferredBytes(Double initialReplicationTransferredBytes) {
if (initialReplicationTransferredBytes == null) {
throw new MissingRequiredPropertyException("InMageRcmFailbackReplicationDetailsResponse", "initialReplicationTransferredBytes");
}
this.initialReplicationTransferredBytes = initialReplicationTransferredBytes;
return this;
}
@CustomType.Setter
public Builder instanceType(String instanceType) {
if (instanceType == null) {
throw new MissingRequiredPropertyException("InMageRcmFailbackReplicationDetailsResponse", "instanceType");
}
this.instanceType = instanceType;
return this;
}
@CustomType.Setter
public Builder internalIdentifier(String internalIdentifier) {
if (internalIdentifier == null) {
throw new MissingRequiredPropertyException("InMageRcmFailbackReplicationDetailsResponse", "internalIdentifier");
}
this.internalIdentifier = internalIdentifier;
return this;
}
@CustomType.Setter
public Builder isAgentRegistrationSuccessfulAfterFailover(Boolean isAgentRegistrationSuccessfulAfterFailover) {
if (isAgentRegistrationSuccessfulAfterFailover == null) {
throw new MissingRequiredPropertyException("InMageRcmFailbackReplicationDetailsResponse", "isAgentRegistrationSuccessfulAfterFailover");
}
this.isAgentRegistrationSuccessfulAfterFailover = isAgentRegistrationSuccessfulAfterFailover;
return this;
}
@CustomType.Setter
public Builder lastPlannedFailoverStartTime(String lastPlannedFailoverStartTime) {
if (lastPlannedFailoverStartTime == null) {
throw new MissingRequiredPropertyException("InMageRcmFailbackReplicationDetailsResponse", "lastPlannedFailoverStartTime");
}
this.lastPlannedFailoverStartTime = lastPlannedFailoverStartTime;
return this;
}
@CustomType.Setter
public Builder lastPlannedFailoverStatus(String lastPlannedFailoverStatus) {
if (lastPlannedFailoverStatus == null) {
throw new MissingRequiredPropertyException("InMageRcmFailbackReplicationDetailsResponse", "lastPlannedFailoverStatus");
}
this.lastPlannedFailoverStatus = lastPlannedFailoverStatus;
return this;
}
@CustomType.Setter
public Builder lastUsedPolicyFriendlyName(String lastUsedPolicyFriendlyName) {
if (lastUsedPolicyFriendlyName == null) {
throw new MissingRequiredPropertyException("InMageRcmFailbackReplicationDetailsResponse", "lastUsedPolicyFriendlyName");
}
this.lastUsedPolicyFriendlyName = lastUsedPolicyFriendlyName;
return this;
}
@CustomType.Setter
public Builder lastUsedPolicyId(String lastUsedPolicyId) {
if (lastUsedPolicyId == null) {
throw new MissingRequiredPropertyException("InMageRcmFailbackReplicationDetailsResponse", "lastUsedPolicyId");
}
this.lastUsedPolicyId = lastUsedPolicyId;
return this;
}
@CustomType.Setter
public Builder logStorageAccountId(String logStorageAccountId) {
if (logStorageAccountId == null) {
throw new MissingRequiredPropertyException("InMageRcmFailbackReplicationDetailsResponse", "logStorageAccountId");
}
this.logStorageAccountId = logStorageAccountId;
return this;
}
@CustomType.Setter
public Builder mobilityAgentDetails(@Nullable InMageRcmFailbackMobilityAgentDetailsResponse mobilityAgentDetails) {
this.mobilityAgentDetails = mobilityAgentDetails;
return this;
}
@CustomType.Setter
public Builder multiVmGroupName(String multiVmGroupName) {
if (multiVmGroupName == null) {
throw new MissingRequiredPropertyException("InMageRcmFailbackReplicationDetailsResponse", "multiVmGroupName");
}
this.multiVmGroupName = multiVmGroupName;
return this;
}
@CustomType.Setter
public Builder osType(String osType) {
if (osType == null) {
throw new MissingRequiredPropertyException("InMageRcmFailbackReplicationDetailsResponse", "osType");
}
this.osType = osType;
return this;
}
@CustomType.Setter
public Builder protectedDisks(@Nullable List protectedDisks) {
this.protectedDisks = protectedDisks;
return this;
}
public Builder protectedDisks(InMageRcmFailbackProtectedDiskDetailsResponse... protectedDisks) {
return protectedDisks(List.of(protectedDisks));
}
@CustomType.Setter
public Builder reprotectAgentId(String reprotectAgentId) {
if (reprotectAgentId == null) {
throw new MissingRequiredPropertyException("InMageRcmFailbackReplicationDetailsResponse", "reprotectAgentId");
}
this.reprotectAgentId = reprotectAgentId;
return this;
}
@CustomType.Setter
public Builder reprotectAgentName(String reprotectAgentName) {
if (reprotectAgentName == null) {
throw new MissingRequiredPropertyException("InMageRcmFailbackReplicationDetailsResponse", "reprotectAgentName");
}
this.reprotectAgentName = reprotectAgentName;
return this;
}
@CustomType.Setter
public Builder resyncProcessedBytes(Double resyncProcessedBytes) {
if (resyncProcessedBytes == null) {
throw new MissingRequiredPropertyException("InMageRcmFailbackReplicationDetailsResponse", "resyncProcessedBytes");
}
this.resyncProcessedBytes = resyncProcessedBytes;
return this;
}
@CustomType.Setter
public Builder resyncProgressHealth(String resyncProgressHealth) {
if (resyncProgressHealth == null) {
throw new MissingRequiredPropertyException("InMageRcmFailbackReplicationDetailsResponse", "resyncProgressHealth");
}
this.resyncProgressHealth = resyncProgressHealth;
return this;
}
@CustomType.Setter
public Builder resyncProgressPercentage(Integer resyncProgressPercentage) {
if (resyncProgressPercentage == null) {
throw new MissingRequiredPropertyException("InMageRcmFailbackReplicationDetailsResponse", "resyncProgressPercentage");
}
this.resyncProgressPercentage = resyncProgressPercentage;
return this;
}
@CustomType.Setter
public Builder resyncRequired(String resyncRequired) {
if (resyncRequired == null) {
throw new MissingRequiredPropertyException("InMageRcmFailbackReplicationDetailsResponse", "resyncRequired");
}
this.resyncRequired = resyncRequired;
return this;
}
@CustomType.Setter
public Builder resyncState(String resyncState) {
if (resyncState == null) {
throw new MissingRequiredPropertyException("InMageRcmFailbackReplicationDetailsResponse", "resyncState");
}
this.resyncState = resyncState;
return this;
}
@CustomType.Setter
public Builder resyncTransferredBytes(Double resyncTransferredBytes) {
if (resyncTransferredBytes == null) {
throw new MissingRequiredPropertyException("InMageRcmFailbackReplicationDetailsResponse", "resyncTransferredBytes");
}
this.resyncTransferredBytes = resyncTransferredBytes;
return this;
}
@CustomType.Setter
public Builder targetDataStoreName(String targetDataStoreName) {
if (targetDataStoreName == null) {
throw new MissingRequiredPropertyException("InMageRcmFailbackReplicationDetailsResponse", "targetDataStoreName");
}
this.targetDataStoreName = targetDataStoreName;
return this;
}
@CustomType.Setter
public Builder targetVmName(String targetVmName) {
if (targetVmName == null) {
throw new MissingRequiredPropertyException("InMageRcmFailbackReplicationDetailsResponse", "targetVmName");
}
this.targetVmName = targetVmName;
return this;
}
@CustomType.Setter
public Builder targetvCenterId(String targetvCenterId) {
if (targetvCenterId == null) {
throw new MissingRequiredPropertyException("InMageRcmFailbackReplicationDetailsResponse", "targetvCenterId");
}
this.targetvCenterId = targetvCenterId;
return this;
}
@CustomType.Setter
public Builder vmNics(@Nullable List vmNics) {
this.vmNics = vmNics;
return this;
}
public Builder vmNics(InMageRcmFailbackNicDetailsResponse... vmNics) {
return vmNics(List.of(vmNics));
}
public InMageRcmFailbackReplicationDetailsResponse build() {
final var _resultValue = new InMageRcmFailbackReplicationDetailsResponse();
_resultValue.azureVirtualMachineId = azureVirtualMachineId;
_resultValue.discoveredVmDetails = discoveredVmDetails;
_resultValue.initialReplicationProcessedBytes = initialReplicationProcessedBytes;
_resultValue.initialReplicationProgressHealth = initialReplicationProgressHealth;
_resultValue.initialReplicationProgressPercentage = initialReplicationProgressPercentage;
_resultValue.initialReplicationTransferredBytes = initialReplicationTransferredBytes;
_resultValue.instanceType = instanceType;
_resultValue.internalIdentifier = internalIdentifier;
_resultValue.isAgentRegistrationSuccessfulAfterFailover = isAgentRegistrationSuccessfulAfterFailover;
_resultValue.lastPlannedFailoverStartTime = lastPlannedFailoverStartTime;
_resultValue.lastPlannedFailoverStatus = lastPlannedFailoverStatus;
_resultValue.lastUsedPolicyFriendlyName = lastUsedPolicyFriendlyName;
_resultValue.lastUsedPolicyId = lastUsedPolicyId;
_resultValue.logStorageAccountId = logStorageAccountId;
_resultValue.mobilityAgentDetails = mobilityAgentDetails;
_resultValue.multiVmGroupName = multiVmGroupName;
_resultValue.osType = osType;
_resultValue.protectedDisks = protectedDisks;
_resultValue.reprotectAgentId = reprotectAgentId;
_resultValue.reprotectAgentName = reprotectAgentName;
_resultValue.resyncProcessedBytes = resyncProcessedBytes;
_resultValue.resyncProgressHealth = resyncProgressHealth;
_resultValue.resyncProgressPercentage = resyncProgressPercentage;
_resultValue.resyncRequired = resyncRequired;
_resultValue.resyncState = resyncState;
_resultValue.resyncTransferredBytes = resyncTransferredBytes;
_resultValue.targetDataStoreName = targetDataStoreName;
_resultValue.targetVmName = targetVmName;
_resultValue.targetvCenterId = targetvCenterId;
_resultValue.vmNics = vmNics;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy