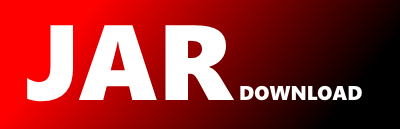
com.pulumi.azurenative.recoveryservices.outputs.InMageRcmSyncDetailsResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.recoveryservices.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Double;
import java.lang.Integer;
import java.lang.String;
import java.util.Objects;
@CustomType
public final class InMageRcmSyncDetailsResponse {
/**
* @return The bytes transferred in last 15 minutes from source VM to azure.
*
*/
private Double last15MinutesTransferredBytes;
/**
* @return The time of the last data transfer from source VM to azure.
*
*/
private String lastDataTransferTimeUtc;
/**
* @return The last refresh time.
*
*/
private String lastRefreshTime;
/**
* @return The total processed bytes. This includes bytes that are transferred from source VM to azure and matched bytes.
*
*/
private Double processedBytes;
/**
* @return The progress health.
*
*/
private String progressHealth;
/**
* @return Progress in percentage. Progress percentage is calculated based on processed bytes.
*
*/
private Integer progressPercentage;
/**
* @return The start time.
*
*/
private String startTime;
/**
* @return The transferred bytes from source VM to azure for the disk.
*
*/
private Double transferredBytes;
private InMageRcmSyncDetailsResponse() {}
/**
* @return The bytes transferred in last 15 minutes from source VM to azure.
*
*/
public Double last15MinutesTransferredBytes() {
return this.last15MinutesTransferredBytes;
}
/**
* @return The time of the last data transfer from source VM to azure.
*
*/
public String lastDataTransferTimeUtc() {
return this.lastDataTransferTimeUtc;
}
/**
* @return The last refresh time.
*
*/
public String lastRefreshTime() {
return this.lastRefreshTime;
}
/**
* @return The total processed bytes. This includes bytes that are transferred from source VM to azure and matched bytes.
*
*/
public Double processedBytes() {
return this.processedBytes;
}
/**
* @return The progress health.
*
*/
public String progressHealth() {
return this.progressHealth;
}
/**
* @return Progress in percentage. Progress percentage is calculated based on processed bytes.
*
*/
public Integer progressPercentage() {
return this.progressPercentage;
}
/**
* @return The start time.
*
*/
public String startTime() {
return this.startTime;
}
/**
* @return The transferred bytes from source VM to azure for the disk.
*
*/
public Double transferredBytes() {
return this.transferredBytes;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(InMageRcmSyncDetailsResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private Double last15MinutesTransferredBytes;
private String lastDataTransferTimeUtc;
private String lastRefreshTime;
private Double processedBytes;
private String progressHealth;
private Integer progressPercentage;
private String startTime;
private Double transferredBytes;
public Builder() {}
public Builder(InMageRcmSyncDetailsResponse defaults) {
Objects.requireNonNull(defaults);
this.last15MinutesTransferredBytes = defaults.last15MinutesTransferredBytes;
this.lastDataTransferTimeUtc = defaults.lastDataTransferTimeUtc;
this.lastRefreshTime = defaults.lastRefreshTime;
this.processedBytes = defaults.processedBytes;
this.progressHealth = defaults.progressHealth;
this.progressPercentage = defaults.progressPercentage;
this.startTime = defaults.startTime;
this.transferredBytes = defaults.transferredBytes;
}
@CustomType.Setter
public Builder last15MinutesTransferredBytes(Double last15MinutesTransferredBytes) {
if (last15MinutesTransferredBytes == null) {
throw new MissingRequiredPropertyException("InMageRcmSyncDetailsResponse", "last15MinutesTransferredBytes");
}
this.last15MinutesTransferredBytes = last15MinutesTransferredBytes;
return this;
}
@CustomType.Setter
public Builder lastDataTransferTimeUtc(String lastDataTransferTimeUtc) {
if (lastDataTransferTimeUtc == null) {
throw new MissingRequiredPropertyException("InMageRcmSyncDetailsResponse", "lastDataTransferTimeUtc");
}
this.lastDataTransferTimeUtc = lastDataTransferTimeUtc;
return this;
}
@CustomType.Setter
public Builder lastRefreshTime(String lastRefreshTime) {
if (lastRefreshTime == null) {
throw new MissingRequiredPropertyException("InMageRcmSyncDetailsResponse", "lastRefreshTime");
}
this.lastRefreshTime = lastRefreshTime;
return this;
}
@CustomType.Setter
public Builder processedBytes(Double processedBytes) {
if (processedBytes == null) {
throw new MissingRequiredPropertyException("InMageRcmSyncDetailsResponse", "processedBytes");
}
this.processedBytes = processedBytes;
return this;
}
@CustomType.Setter
public Builder progressHealth(String progressHealth) {
if (progressHealth == null) {
throw new MissingRequiredPropertyException("InMageRcmSyncDetailsResponse", "progressHealth");
}
this.progressHealth = progressHealth;
return this;
}
@CustomType.Setter
public Builder progressPercentage(Integer progressPercentage) {
if (progressPercentage == null) {
throw new MissingRequiredPropertyException("InMageRcmSyncDetailsResponse", "progressPercentage");
}
this.progressPercentage = progressPercentage;
return this;
}
@CustomType.Setter
public Builder startTime(String startTime) {
if (startTime == null) {
throw new MissingRequiredPropertyException("InMageRcmSyncDetailsResponse", "startTime");
}
this.startTime = startTime;
return this;
}
@CustomType.Setter
public Builder transferredBytes(Double transferredBytes) {
if (transferredBytes == null) {
throw new MissingRequiredPropertyException("InMageRcmSyncDetailsResponse", "transferredBytes");
}
this.transferredBytes = transferredBytes;
return this;
}
public InMageRcmSyncDetailsResponse build() {
final var _resultValue = new InMageRcmSyncDetailsResponse();
_resultValue.last15MinutesTransferredBytes = last15MinutesTransferredBytes;
_resultValue.lastDataTransferTimeUtc = lastDataTransferTimeUtc;
_resultValue.lastRefreshTime = lastRefreshTime;
_resultValue.processedBytes = processedBytes;
_resultValue.progressHealth = progressHealth;
_resultValue.progressPercentage = progressPercentage;
_resultValue.startTime = startTime;
_resultValue.transferredBytes = transferredBytes;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy