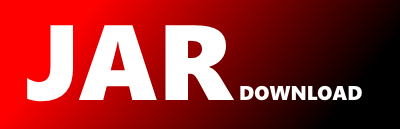
com.pulumi.azurenative.recoveryservices.outputs.VMwareCbtMigrationDetailsResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.recoveryservices.outputs;
import com.pulumi.azurenative.recoveryservices.outputs.VMwareCbtNicDetailsResponse;
import com.pulumi.azurenative.recoveryservices.outputs.VMwareCbtProtectedDiskDetailsResponse;
import com.pulumi.azurenative.recoveryservices.outputs.VMwareCbtSecurityProfilePropertiesResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Double;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class VMwareCbtMigrationDetailsResponse {
/**
* @return The confidential VM key vault Id for ADE installation.
*
*/
private @Nullable String confidentialVmKeyVaultId;
/**
* @return The data mover run as account Id.
*
*/
private String dataMoverRunAsAccountId;
/**
* @return The firmware type.
*
*/
private String firmwareType;
/**
* @return The initial seeding progress percentage.
*
*/
private Integer initialSeedingProgressPercentage;
/**
* @return The initial seeding retry count.
*
*/
private Double initialSeedingRetryCount;
/**
* @return Gets the instance type.
* Expected value is 'VMwareCbt'.
*
*/
private String instanceType;
/**
* @return The last recovery point Id.
*
*/
private String lastRecoveryPointId;
/**
* @return The last recovery point received time.
*
*/
private String lastRecoveryPointReceived;
/**
* @return License Type of the VM to be used.
*
*/
private @Nullable String licenseType;
/**
* @return The migration progress percentage.
*
*/
private Integer migrationProgressPercentage;
/**
* @return The recovery point Id to which the VM was migrated.
*
*/
private String migrationRecoveryPointId;
/**
* @return The name of the OS on the VM.
*
*/
private String osName;
/**
* @return The type of the OS on the VM.
*
*/
private String osType;
/**
* @return A value indicating whether auto resync is to be done.
*
*/
private @Nullable String performAutoResync;
/**
* @return The list of protected disks.
*
*/
private @Nullable List protectedDisks;
/**
* @return The resume progress percentage.
*
*/
private Integer resumeProgressPercentage;
/**
* @return The resume retry count.
*
*/
private Double resumeRetryCount;
/**
* @return The resync progress percentage.
*
*/
private Integer resyncProgressPercentage;
/**
* @return A value indicating whether resync is required.
*
*/
private String resyncRequired;
/**
* @return The resync retry count.
*
*/
private Double resyncRetryCount;
/**
* @return The resync state.
*
*/
private String resyncState;
/**
* @return The tags for the seed disks.
*
*/
private @Nullable Map seedDiskTags;
/**
* @return The snapshot run as account Id.
*
*/
private String snapshotRunAsAccountId;
/**
* @return The SQL Server license type.
*
*/
private @Nullable String sqlServerLicenseType;
/**
* @return The replication storage account ARM Id. This is applicable only for the blob based replication test hook.
*
*/
private String storageAccountId;
/**
* @return List of supported inplace OS Upgrade versions.
*
*/
private @Nullable List supportedOSVersions;
/**
* @return The target availability set Id.
*
*/
private @Nullable String targetAvailabilitySetId;
/**
* @return The target availability zone.
*
*/
private @Nullable String targetAvailabilityZone;
/**
* @return The target boot diagnostics storage account ARM Id.
*
*/
private @Nullable String targetBootDiagnosticsStorageAccountId;
/**
* @return The tags for the target disks.
*
*/
private @Nullable Map targetDiskTags;
/**
* @return The target generation.
*
*/
private String targetGeneration;
/**
* @return The target location.
*
*/
private String targetLocation;
/**
* @return The target network Id.
*
*/
private @Nullable String targetNetworkId;
/**
* @return The tags for the target NICs.
*
*/
private @Nullable Map targetNicTags;
/**
* @return The target proximity placement group Id.
*
*/
private @Nullable String targetProximityPlacementGroupId;
/**
* @return The target resource group Id.
*
*/
private @Nullable String targetResourceGroupId;
/**
* @return Target VM name.
*
*/
private @Nullable String targetVmName;
/**
* @return The target VM security profile.
*
*/
private @Nullable VMwareCbtSecurityProfilePropertiesResponse targetVmSecurityProfile;
/**
* @return The target VM size.
*
*/
private @Nullable String targetVmSize;
/**
* @return The target VM tags.
*
*/
private @Nullable Map targetVmTags;
/**
* @return The test network Id.
*
*/
private @Nullable String testNetworkId;
/**
* @return The network details.
*
*/
private @Nullable List vmNics;
/**
* @return The ARM Id of the VM discovered in VMware.
*
*/
private String vmwareMachineId;
private VMwareCbtMigrationDetailsResponse() {}
/**
* @return The confidential VM key vault Id for ADE installation.
*
*/
public Optional confidentialVmKeyVaultId() {
return Optional.ofNullable(this.confidentialVmKeyVaultId);
}
/**
* @return The data mover run as account Id.
*
*/
public String dataMoverRunAsAccountId() {
return this.dataMoverRunAsAccountId;
}
/**
* @return The firmware type.
*
*/
public String firmwareType() {
return this.firmwareType;
}
/**
* @return The initial seeding progress percentage.
*
*/
public Integer initialSeedingProgressPercentage() {
return this.initialSeedingProgressPercentage;
}
/**
* @return The initial seeding retry count.
*
*/
public Double initialSeedingRetryCount() {
return this.initialSeedingRetryCount;
}
/**
* @return Gets the instance type.
* Expected value is 'VMwareCbt'.
*
*/
public String instanceType() {
return this.instanceType;
}
/**
* @return The last recovery point Id.
*
*/
public String lastRecoveryPointId() {
return this.lastRecoveryPointId;
}
/**
* @return The last recovery point received time.
*
*/
public String lastRecoveryPointReceived() {
return this.lastRecoveryPointReceived;
}
/**
* @return License Type of the VM to be used.
*
*/
public Optional licenseType() {
return Optional.ofNullable(this.licenseType);
}
/**
* @return The migration progress percentage.
*
*/
public Integer migrationProgressPercentage() {
return this.migrationProgressPercentage;
}
/**
* @return The recovery point Id to which the VM was migrated.
*
*/
public String migrationRecoveryPointId() {
return this.migrationRecoveryPointId;
}
/**
* @return The name of the OS on the VM.
*
*/
public String osName() {
return this.osName;
}
/**
* @return The type of the OS on the VM.
*
*/
public String osType() {
return this.osType;
}
/**
* @return A value indicating whether auto resync is to be done.
*
*/
public Optional performAutoResync() {
return Optional.ofNullable(this.performAutoResync);
}
/**
* @return The list of protected disks.
*
*/
public List protectedDisks() {
return this.protectedDisks == null ? List.of() : this.protectedDisks;
}
/**
* @return The resume progress percentage.
*
*/
public Integer resumeProgressPercentage() {
return this.resumeProgressPercentage;
}
/**
* @return The resume retry count.
*
*/
public Double resumeRetryCount() {
return this.resumeRetryCount;
}
/**
* @return The resync progress percentage.
*
*/
public Integer resyncProgressPercentage() {
return this.resyncProgressPercentage;
}
/**
* @return A value indicating whether resync is required.
*
*/
public String resyncRequired() {
return this.resyncRequired;
}
/**
* @return The resync retry count.
*
*/
public Double resyncRetryCount() {
return this.resyncRetryCount;
}
/**
* @return The resync state.
*
*/
public String resyncState() {
return this.resyncState;
}
/**
* @return The tags for the seed disks.
*
*/
public Map seedDiskTags() {
return this.seedDiskTags == null ? Map.of() : this.seedDiskTags;
}
/**
* @return The snapshot run as account Id.
*
*/
public String snapshotRunAsAccountId() {
return this.snapshotRunAsAccountId;
}
/**
* @return The SQL Server license type.
*
*/
public Optional sqlServerLicenseType() {
return Optional.ofNullable(this.sqlServerLicenseType);
}
/**
* @return The replication storage account ARM Id. This is applicable only for the blob based replication test hook.
*
*/
public String storageAccountId() {
return this.storageAccountId;
}
/**
* @return List of supported inplace OS Upgrade versions.
*
*/
public List supportedOSVersions() {
return this.supportedOSVersions == null ? List.of() : this.supportedOSVersions;
}
/**
* @return The target availability set Id.
*
*/
public Optional targetAvailabilitySetId() {
return Optional.ofNullable(this.targetAvailabilitySetId);
}
/**
* @return The target availability zone.
*
*/
public Optional targetAvailabilityZone() {
return Optional.ofNullable(this.targetAvailabilityZone);
}
/**
* @return The target boot diagnostics storage account ARM Id.
*
*/
public Optional targetBootDiagnosticsStorageAccountId() {
return Optional.ofNullable(this.targetBootDiagnosticsStorageAccountId);
}
/**
* @return The tags for the target disks.
*
*/
public Map targetDiskTags() {
return this.targetDiskTags == null ? Map.of() : this.targetDiskTags;
}
/**
* @return The target generation.
*
*/
public String targetGeneration() {
return this.targetGeneration;
}
/**
* @return The target location.
*
*/
public String targetLocation() {
return this.targetLocation;
}
/**
* @return The target network Id.
*
*/
public Optional targetNetworkId() {
return Optional.ofNullable(this.targetNetworkId);
}
/**
* @return The tags for the target NICs.
*
*/
public Map targetNicTags() {
return this.targetNicTags == null ? Map.of() : this.targetNicTags;
}
/**
* @return The target proximity placement group Id.
*
*/
public Optional targetProximityPlacementGroupId() {
return Optional.ofNullable(this.targetProximityPlacementGroupId);
}
/**
* @return The target resource group Id.
*
*/
public Optional targetResourceGroupId() {
return Optional.ofNullable(this.targetResourceGroupId);
}
/**
* @return Target VM name.
*
*/
public Optional targetVmName() {
return Optional.ofNullable(this.targetVmName);
}
/**
* @return The target VM security profile.
*
*/
public Optional targetVmSecurityProfile() {
return Optional.ofNullable(this.targetVmSecurityProfile);
}
/**
* @return The target VM size.
*
*/
public Optional targetVmSize() {
return Optional.ofNullable(this.targetVmSize);
}
/**
* @return The target VM tags.
*
*/
public Map targetVmTags() {
return this.targetVmTags == null ? Map.of() : this.targetVmTags;
}
/**
* @return The test network Id.
*
*/
public Optional testNetworkId() {
return Optional.ofNullable(this.testNetworkId);
}
/**
* @return The network details.
*
*/
public List vmNics() {
return this.vmNics == null ? List.of() : this.vmNics;
}
/**
* @return The ARM Id of the VM discovered in VMware.
*
*/
public String vmwareMachineId() {
return this.vmwareMachineId;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(VMwareCbtMigrationDetailsResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable String confidentialVmKeyVaultId;
private String dataMoverRunAsAccountId;
private String firmwareType;
private Integer initialSeedingProgressPercentage;
private Double initialSeedingRetryCount;
private String instanceType;
private String lastRecoveryPointId;
private String lastRecoveryPointReceived;
private @Nullable String licenseType;
private Integer migrationProgressPercentage;
private String migrationRecoveryPointId;
private String osName;
private String osType;
private @Nullable String performAutoResync;
private @Nullable List protectedDisks;
private Integer resumeProgressPercentage;
private Double resumeRetryCount;
private Integer resyncProgressPercentage;
private String resyncRequired;
private Double resyncRetryCount;
private String resyncState;
private @Nullable Map seedDiskTags;
private String snapshotRunAsAccountId;
private @Nullable String sqlServerLicenseType;
private String storageAccountId;
private @Nullable List supportedOSVersions;
private @Nullable String targetAvailabilitySetId;
private @Nullable String targetAvailabilityZone;
private @Nullable String targetBootDiagnosticsStorageAccountId;
private @Nullable Map targetDiskTags;
private String targetGeneration;
private String targetLocation;
private @Nullable String targetNetworkId;
private @Nullable Map targetNicTags;
private @Nullable String targetProximityPlacementGroupId;
private @Nullable String targetResourceGroupId;
private @Nullable String targetVmName;
private @Nullable VMwareCbtSecurityProfilePropertiesResponse targetVmSecurityProfile;
private @Nullable String targetVmSize;
private @Nullable Map targetVmTags;
private @Nullable String testNetworkId;
private @Nullable List vmNics;
private String vmwareMachineId;
public Builder() {}
public Builder(VMwareCbtMigrationDetailsResponse defaults) {
Objects.requireNonNull(defaults);
this.confidentialVmKeyVaultId = defaults.confidentialVmKeyVaultId;
this.dataMoverRunAsAccountId = defaults.dataMoverRunAsAccountId;
this.firmwareType = defaults.firmwareType;
this.initialSeedingProgressPercentage = defaults.initialSeedingProgressPercentage;
this.initialSeedingRetryCount = defaults.initialSeedingRetryCount;
this.instanceType = defaults.instanceType;
this.lastRecoveryPointId = defaults.lastRecoveryPointId;
this.lastRecoveryPointReceived = defaults.lastRecoveryPointReceived;
this.licenseType = defaults.licenseType;
this.migrationProgressPercentage = defaults.migrationProgressPercentage;
this.migrationRecoveryPointId = defaults.migrationRecoveryPointId;
this.osName = defaults.osName;
this.osType = defaults.osType;
this.performAutoResync = defaults.performAutoResync;
this.protectedDisks = defaults.protectedDisks;
this.resumeProgressPercentage = defaults.resumeProgressPercentage;
this.resumeRetryCount = defaults.resumeRetryCount;
this.resyncProgressPercentage = defaults.resyncProgressPercentage;
this.resyncRequired = defaults.resyncRequired;
this.resyncRetryCount = defaults.resyncRetryCount;
this.resyncState = defaults.resyncState;
this.seedDiskTags = defaults.seedDiskTags;
this.snapshotRunAsAccountId = defaults.snapshotRunAsAccountId;
this.sqlServerLicenseType = defaults.sqlServerLicenseType;
this.storageAccountId = defaults.storageAccountId;
this.supportedOSVersions = defaults.supportedOSVersions;
this.targetAvailabilitySetId = defaults.targetAvailabilitySetId;
this.targetAvailabilityZone = defaults.targetAvailabilityZone;
this.targetBootDiagnosticsStorageAccountId = defaults.targetBootDiagnosticsStorageAccountId;
this.targetDiskTags = defaults.targetDiskTags;
this.targetGeneration = defaults.targetGeneration;
this.targetLocation = defaults.targetLocation;
this.targetNetworkId = defaults.targetNetworkId;
this.targetNicTags = defaults.targetNicTags;
this.targetProximityPlacementGroupId = defaults.targetProximityPlacementGroupId;
this.targetResourceGroupId = defaults.targetResourceGroupId;
this.targetVmName = defaults.targetVmName;
this.targetVmSecurityProfile = defaults.targetVmSecurityProfile;
this.targetVmSize = defaults.targetVmSize;
this.targetVmTags = defaults.targetVmTags;
this.testNetworkId = defaults.testNetworkId;
this.vmNics = defaults.vmNics;
this.vmwareMachineId = defaults.vmwareMachineId;
}
@CustomType.Setter
public Builder confidentialVmKeyVaultId(@Nullable String confidentialVmKeyVaultId) {
this.confidentialVmKeyVaultId = confidentialVmKeyVaultId;
return this;
}
@CustomType.Setter
public Builder dataMoverRunAsAccountId(String dataMoverRunAsAccountId) {
if (dataMoverRunAsAccountId == null) {
throw new MissingRequiredPropertyException("VMwareCbtMigrationDetailsResponse", "dataMoverRunAsAccountId");
}
this.dataMoverRunAsAccountId = dataMoverRunAsAccountId;
return this;
}
@CustomType.Setter
public Builder firmwareType(String firmwareType) {
if (firmwareType == null) {
throw new MissingRequiredPropertyException("VMwareCbtMigrationDetailsResponse", "firmwareType");
}
this.firmwareType = firmwareType;
return this;
}
@CustomType.Setter
public Builder initialSeedingProgressPercentage(Integer initialSeedingProgressPercentage) {
if (initialSeedingProgressPercentage == null) {
throw new MissingRequiredPropertyException("VMwareCbtMigrationDetailsResponse", "initialSeedingProgressPercentage");
}
this.initialSeedingProgressPercentage = initialSeedingProgressPercentage;
return this;
}
@CustomType.Setter
public Builder initialSeedingRetryCount(Double initialSeedingRetryCount) {
if (initialSeedingRetryCount == null) {
throw new MissingRequiredPropertyException("VMwareCbtMigrationDetailsResponse", "initialSeedingRetryCount");
}
this.initialSeedingRetryCount = initialSeedingRetryCount;
return this;
}
@CustomType.Setter
public Builder instanceType(String instanceType) {
if (instanceType == null) {
throw new MissingRequiredPropertyException("VMwareCbtMigrationDetailsResponse", "instanceType");
}
this.instanceType = instanceType;
return this;
}
@CustomType.Setter
public Builder lastRecoveryPointId(String lastRecoveryPointId) {
if (lastRecoveryPointId == null) {
throw new MissingRequiredPropertyException("VMwareCbtMigrationDetailsResponse", "lastRecoveryPointId");
}
this.lastRecoveryPointId = lastRecoveryPointId;
return this;
}
@CustomType.Setter
public Builder lastRecoveryPointReceived(String lastRecoveryPointReceived) {
if (lastRecoveryPointReceived == null) {
throw new MissingRequiredPropertyException("VMwareCbtMigrationDetailsResponse", "lastRecoveryPointReceived");
}
this.lastRecoveryPointReceived = lastRecoveryPointReceived;
return this;
}
@CustomType.Setter
public Builder licenseType(@Nullable String licenseType) {
this.licenseType = licenseType;
return this;
}
@CustomType.Setter
public Builder migrationProgressPercentage(Integer migrationProgressPercentage) {
if (migrationProgressPercentage == null) {
throw new MissingRequiredPropertyException("VMwareCbtMigrationDetailsResponse", "migrationProgressPercentage");
}
this.migrationProgressPercentage = migrationProgressPercentage;
return this;
}
@CustomType.Setter
public Builder migrationRecoveryPointId(String migrationRecoveryPointId) {
if (migrationRecoveryPointId == null) {
throw new MissingRequiredPropertyException("VMwareCbtMigrationDetailsResponse", "migrationRecoveryPointId");
}
this.migrationRecoveryPointId = migrationRecoveryPointId;
return this;
}
@CustomType.Setter
public Builder osName(String osName) {
if (osName == null) {
throw new MissingRequiredPropertyException("VMwareCbtMigrationDetailsResponse", "osName");
}
this.osName = osName;
return this;
}
@CustomType.Setter
public Builder osType(String osType) {
if (osType == null) {
throw new MissingRequiredPropertyException("VMwareCbtMigrationDetailsResponse", "osType");
}
this.osType = osType;
return this;
}
@CustomType.Setter
public Builder performAutoResync(@Nullable String performAutoResync) {
this.performAutoResync = performAutoResync;
return this;
}
@CustomType.Setter
public Builder protectedDisks(@Nullable List protectedDisks) {
this.protectedDisks = protectedDisks;
return this;
}
public Builder protectedDisks(VMwareCbtProtectedDiskDetailsResponse... protectedDisks) {
return protectedDisks(List.of(protectedDisks));
}
@CustomType.Setter
public Builder resumeProgressPercentage(Integer resumeProgressPercentage) {
if (resumeProgressPercentage == null) {
throw new MissingRequiredPropertyException("VMwareCbtMigrationDetailsResponse", "resumeProgressPercentage");
}
this.resumeProgressPercentage = resumeProgressPercentage;
return this;
}
@CustomType.Setter
public Builder resumeRetryCount(Double resumeRetryCount) {
if (resumeRetryCount == null) {
throw new MissingRequiredPropertyException("VMwareCbtMigrationDetailsResponse", "resumeRetryCount");
}
this.resumeRetryCount = resumeRetryCount;
return this;
}
@CustomType.Setter
public Builder resyncProgressPercentage(Integer resyncProgressPercentage) {
if (resyncProgressPercentage == null) {
throw new MissingRequiredPropertyException("VMwareCbtMigrationDetailsResponse", "resyncProgressPercentage");
}
this.resyncProgressPercentage = resyncProgressPercentage;
return this;
}
@CustomType.Setter
public Builder resyncRequired(String resyncRequired) {
if (resyncRequired == null) {
throw new MissingRequiredPropertyException("VMwareCbtMigrationDetailsResponse", "resyncRequired");
}
this.resyncRequired = resyncRequired;
return this;
}
@CustomType.Setter
public Builder resyncRetryCount(Double resyncRetryCount) {
if (resyncRetryCount == null) {
throw new MissingRequiredPropertyException("VMwareCbtMigrationDetailsResponse", "resyncRetryCount");
}
this.resyncRetryCount = resyncRetryCount;
return this;
}
@CustomType.Setter
public Builder resyncState(String resyncState) {
if (resyncState == null) {
throw new MissingRequiredPropertyException("VMwareCbtMigrationDetailsResponse", "resyncState");
}
this.resyncState = resyncState;
return this;
}
@CustomType.Setter
public Builder seedDiskTags(@Nullable Map seedDiskTags) {
this.seedDiskTags = seedDiskTags;
return this;
}
@CustomType.Setter
public Builder snapshotRunAsAccountId(String snapshotRunAsAccountId) {
if (snapshotRunAsAccountId == null) {
throw new MissingRequiredPropertyException("VMwareCbtMigrationDetailsResponse", "snapshotRunAsAccountId");
}
this.snapshotRunAsAccountId = snapshotRunAsAccountId;
return this;
}
@CustomType.Setter
public Builder sqlServerLicenseType(@Nullable String sqlServerLicenseType) {
this.sqlServerLicenseType = sqlServerLicenseType;
return this;
}
@CustomType.Setter
public Builder storageAccountId(String storageAccountId) {
if (storageAccountId == null) {
throw new MissingRequiredPropertyException("VMwareCbtMigrationDetailsResponse", "storageAccountId");
}
this.storageAccountId = storageAccountId;
return this;
}
@CustomType.Setter
public Builder supportedOSVersions(@Nullable List supportedOSVersions) {
this.supportedOSVersions = supportedOSVersions;
return this;
}
public Builder supportedOSVersions(String... supportedOSVersions) {
return supportedOSVersions(List.of(supportedOSVersions));
}
@CustomType.Setter
public Builder targetAvailabilitySetId(@Nullable String targetAvailabilitySetId) {
this.targetAvailabilitySetId = targetAvailabilitySetId;
return this;
}
@CustomType.Setter
public Builder targetAvailabilityZone(@Nullable String targetAvailabilityZone) {
this.targetAvailabilityZone = targetAvailabilityZone;
return this;
}
@CustomType.Setter
public Builder targetBootDiagnosticsStorageAccountId(@Nullable String targetBootDiagnosticsStorageAccountId) {
this.targetBootDiagnosticsStorageAccountId = targetBootDiagnosticsStorageAccountId;
return this;
}
@CustomType.Setter
public Builder targetDiskTags(@Nullable Map targetDiskTags) {
this.targetDiskTags = targetDiskTags;
return this;
}
@CustomType.Setter
public Builder targetGeneration(String targetGeneration) {
if (targetGeneration == null) {
throw new MissingRequiredPropertyException("VMwareCbtMigrationDetailsResponse", "targetGeneration");
}
this.targetGeneration = targetGeneration;
return this;
}
@CustomType.Setter
public Builder targetLocation(String targetLocation) {
if (targetLocation == null) {
throw new MissingRequiredPropertyException("VMwareCbtMigrationDetailsResponse", "targetLocation");
}
this.targetLocation = targetLocation;
return this;
}
@CustomType.Setter
public Builder targetNetworkId(@Nullable String targetNetworkId) {
this.targetNetworkId = targetNetworkId;
return this;
}
@CustomType.Setter
public Builder targetNicTags(@Nullable Map targetNicTags) {
this.targetNicTags = targetNicTags;
return this;
}
@CustomType.Setter
public Builder targetProximityPlacementGroupId(@Nullable String targetProximityPlacementGroupId) {
this.targetProximityPlacementGroupId = targetProximityPlacementGroupId;
return this;
}
@CustomType.Setter
public Builder targetResourceGroupId(@Nullable String targetResourceGroupId) {
this.targetResourceGroupId = targetResourceGroupId;
return this;
}
@CustomType.Setter
public Builder targetVmName(@Nullable String targetVmName) {
this.targetVmName = targetVmName;
return this;
}
@CustomType.Setter
public Builder targetVmSecurityProfile(@Nullable VMwareCbtSecurityProfilePropertiesResponse targetVmSecurityProfile) {
this.targetVmSecurityProfile = targetVmSecurityProfile;
return this;
}
@CustomType.Setter
public Builder targetVmSize(@Nullable String targetVmSize) {
this.targetVmSize = targetVmSize;
return this;
}
@CustomType.Setter
public Builder targetVmTags(@Nullable Map targetVmTags) {
this.targetVmTags = targetVmTags;
return this;
}
@CustomType.Setter
public Builder testNetworkId(@Nullable String testNetworkId) {
this.testNetworkId = testNetworkId;
return this;
}
@CustomType.Setter
public Builder vmNics(@Nullable List vmNics) {
this.vmNics = vmNics;
return this;
}
public Builder vmNics(VMwareCbtNicDetailsResponse... vmNics) {
return vmNics(List.of(vmNics));
}
@CustomType.Setter
public Builder vmwareMachineId(String vmwareMachineId) {
if (vmwareMachineId == null) {
throw new MissingRequiredPropertyException("VMwareCbtMigrationDetailsResponse", "vmwareMachineId");
}
this.vmwareMachineId = vmwareMachineId;
return this;
}
public VMwareCbtMigrationDetailsResponse build() {
final var _resultValue = new VMwareCbtMigrationDetailsResponse();
_resultValue.confidentialVmKeyVaultId = confidentialVmKeyVaultId;
_resultValue.dataMoverRunAsAccountId = dataMoverRunAsAccountId;
_resultValue.firmwareType = firmwareType;
_resultValue.initialSeedingProgressPercentage = initialSeedingProgressPercentage;
_resultValue.initialSeedingRetryCount = initialSeedingRetryCount;
_resultValue.instanceType = instanceType;
_resultValue.lastRecoveryPointId = lastRecoveryPointId;
_resultValue.lastRecoveryPointReceived = lastRecoveryPointReceived;
_resultValue.licenseType = licenseType;
_resultValue.migrationProgressPercentage = migrationProgressPercentage;
_resultValue.migrationRecoveryPointId = migrationRecoveryPointId;
_resultValue.osName = osName;
_resultValue.osType = osType;
_resultValue.performAutoResync = performAutoResync;
_resultValue.protectedDisks = protectedDisks;
_resultValue.resumeProgressPercentage = resumeProgressPercentage;
_resultValue.resumeRetryCount = resumeRetryCount;
_resultValue.resyncProgressPercentage = resyncProgressPercentage;
_resultValue.resyncRequired = resyncRequired;
_resultValue.resyncRetryCount = resyncRetryCount;
_resultValue.resyncState = resyncState;
_resultValue.seedDiskTags = seedDiskTags;
_resultValue.snapshotRunAsAccountId = snapshotRunAsAccountId;
_resultValue.sqlServerLicenseType = sqlServerLicenseType;
_resultValue.storageAccountId = storageAccountId;
_resultValue.supportedOSVersions = supportedOSVersions;
_resultValue.targetAvailabilitySetId = targetAvailabilitySetId;
_resultValue.targetAvailabilityZone = targetAvailabilityZone;
_resultValue.targetBootDiagnosticsStorageAccountId = targetBootDiagnosticsStorageAccountId;
_resultValue.targetDiskTags = targetDiskTags;
_resultValue.targetGeneration = targetGeneration;
_resultValue.targetLocation = targetLocation;
_resultValue.targetNetworkId = targetNetworkId;
_resultValue.targetNicTags = targetNicTags;
_resultValue.targetProximityPlacementGroupId = targetProximityPlacementGroupId;
_resultValue.targetResourceGroupId = targetResourceGroupId;
_resultValue.targetVmName = targetVmName;
_resultValue.targetVmSecurityProfile = targetVmSecurityProfile;
_resultValue.targetVmSize = targetVmSize;
_resultValue.targetVmTags = targetVmTags;
_resultValue.testNetworkId = testNetworkId;
_resultValue.vmNics = vmNics;
_resultValue.vmwareMachineId = vmwareMachineId;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy