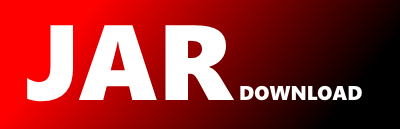
com.pulumi.azurenative.recoveryservices.outputs.VMwareDetailsResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.recoveryservices.outputs;
import com.pulumi.azurenative.recoveryservices.outputs.InMageFabricSwitchProviderBlockingErrorDetailsResponse;
import com.pulumi.azurenative.recoveryservices.outputs.MasterTargetServerResponse;
import com.pulumi.azurenative.recoveryservices.outputs.ProcessServerResponse;
import com.pulumi.azurenative.recoveryservices.outputs.RunAsAccountResponse;
import com.pulumi.azurenative.recoveryservices.outputs.VersionDetailsResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Double;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class VMwareDetailsResponse {
/**
* @return The number of source and target servers configured to talk to this CS.
*
*/
private @Nullable String agentCount;
/**
* @return Agent expiry date.
*
*/
private @Nullable String agentExpiryDate;
/**
* @return The agent Version.
*
*/
private @Nullable String agentVersion;
/**
* @return The agent version details.
*
*/
private @Nullable VersionDetailsResponse agentVersionDetails;
/**
* @return The available memory.
*
*/
private @Nullable Double availableMemoryInBytes;
/**
* @return The available space.
*
*/
private @Nullable Double availableSpaceInBytes;
/**
* @return The percentage of the CPU load.
*
*/
private @Nullable String cpuLoad;
/**
* @return The CPU load status.
*
*/
private @Nullable String cpuLoadStatus;
/**
* @return The CS service status.
*
*/
private @Nullable String csServiceStatus;
/**
* @return The database server load.
*
*/
private @Nullable String databaseServerLoad;
/**
* @return The database server load status.
*
*/
private @Nullable String databaseServerLoadStatus;
/**
* @return The host name.
*
*/
private @Nullable String hostName;
/**
* @return Gets the class type. Overridden in derived classes.
* Expected value is 'VMware'.
*
*/
private String instanceType;
/**
* @return The IP address.
*
*/
private @Nullable String ipAddress;
/**
* @return The last heartbeat received from CS server.
*
*/
private @Nullable String lastHeartbeat;
/**
* @return The list of Master Target servers associated with the fabric.
*
*/
private @Nullable List masterTargetServers;
/**
* @return The memory usage status.
*
*/
private @Nullable String memoryUsageStatus;
/**
* @return The number of process servers.
*
*/
private @Nullable String processServerCount;
/**
* @return The list of Process Servers associated with the fabric.
*
*/
private @Nullable List processServers;
/**
* @return The number of protected servers.
*
*/
private @Nullable String protectedServers;
/**
* @return PS template version.
*
*/
private @Nullable String psTemplateVersion;
/**
* @return The number of replication pairs configured in this CS.
*
*/
private @Nullable String replicationPairCount;
/**
* @return The list of run as accounts created on the server.
*
*/
private @Nullable List runAsAccounts;
/**
* @return The space usage status.
*
*/
private @Nullable String spaceUsageStatus;
/**
* @return CS SSL cert expiry date.
*
*/
private @Nullable String sslCertExpiryDate;
/**
* @return CS SSL cert expiry date.
*
*/
private @Nullable Integer sslCertExpiryRemainingDays;
/**
* @return The switch provider blocking error information.
*
*/
private @Nullable List switchProviderBlockingErrorDetails;
/**
* @return The percentage of the system load.
*
*/
private @Nullable String systemLoad;
/**
* @return The system load status.
*
*/
private @Nullable String systemLoadStatus;
/**
* @return The total memory.
*
*/
private @Nullable Double totalMemoryInBytes;
/**
* @return The total space.
*
*/
private @Nullable Double totalSpaceInBytes;
/**
* @return Version status.
*
*/
private @Nullable String versionStatus;
/**
* @return The web load.
*
*/
private @Nullable String webLoad;
/**
* @return The web load status.
*
*/
private @Nullable String webLoadStatus;
private VMwareDetailsResponse() {}
/**
* @return The number of source and target servers configured to talk to this CS.
*
*/
public Optional agentCount() {
return Optional.ofNullable(this.agentCount);
}
/**
* @return Agent expiry date.
*
*/
public Optional agentExpiryDate() {
return Optional.ofNullable(this.agentExpiryDate);
}
/**
* @return The agent Version.
*
*/
public Optional agentVersion() {
return Optional.ofNullable(this.agentVersion);
}
/**
* @return The agent version details.
*
*/
public Optional agentVersionDetails() {
return Optional.ofNullable(this.agentVersionDetails);
}
/**
* @return The available memory.
*
*/
public Optional availableMemoryInBytes() {
return Optional.ofNullable(this.availableMemoryInBytes);
}
/**
* @return The available space.
*
*/
public Optional availableSpaceInBytes() {
return Optional.ofNullable(this.availableSpaceInBytes);
}
/**
* @return The percentage of the CPU load.
*
*/
public Optional cpuLoad() {
return Optional.ofNullable(this.cpuLoad);
}
/**
* @return The CPU load status.
*
*/
public Optional cpuLoadStatus() {
return Optional.ofNullable(this.cpuLoadStatus);
}
/**
* @return The CS service status.
*
*/
public Optional csServiceStatus() {
return Optional.ofNullable(this.csServiceStatus);
}
/**
* @return The database server load.
*
*/
public Optional databaseServerLoad() {
return Optional.ofNullable(this.databaseServerLoad);
}
/**
* @return The database server load status.
*
*/
public Optional databaseServerLoadStatus() {
return Optional.ofNullable(this.databaseServerLoadStatus);
}
/**
* @return The host name.
*
*/
public Optional hostName() {
return Optional.ofNullable(this.hostName);
}
/**
* @return Gets the class type. Overridden in derived classes.
* Expected value is 'VMware'.
*
*/
public String instanceType() {
return this.instanceType;
}
/**
* @return The IP address.
*
*/
public Optional ipAddress() {
return Optional.ofNullable(this.ipAddress);
}
/**
* @return The last heartbeat received from CS server.
*
*/
public Optional lastHeartbeat() {
return Optional.ofNullable(this.lastHeartbeat);
}
/**
* @return The list of Master Target servers associated with the fabric.
*
*/
public List masterTargetServers() {
return this.masterTargetServers == null ? List.of() : this.masterTargetServers;
}
/**
* @return The memory usage status.
*
*/
public Optional memoryUsageStatus() {
return Optional.ofNullable(this.memoryUsageStatus);
}
/**
* @return The number of process servers.
*
*/
public Optional processServerCount() {
return Optional.ofNullable(this.processServerCount);
}
/**
* @return The list of Process Servers associated with the fabric.
*
*/
public List processServers() {
return this.processServers == null ? List.of() : this.processServers;
}
/**
* @return The number of protected servers.
*
*/
public Optional protectedServers() {
return Optional.ofNullable(this.protectedServers);
}
/**
* @return PS template version.
*
*/
public Optional psTemplateVersion() {
return Optional.ofNullable(this.psTemplateVersion);
}
/**
* @return The number of replication pairs configured in this CS.
*
*/
public Optional replicationPairCount() {
return Optional.ofNullable(this.replicationPairCount);
}
/**
* @return The list of run as accounts created on the server.
*
*/
public List runAsAccounts() {
return this.runAsAccounts == null ? List.of() : this.runAsAccounts;
}
/**
* @return The space usage status.
*
*/
public Optional spaceUsageStatus() {
return Optional.ofNullable(this.spaceUsageStatus);
}
/**
* @return CS SSL cert expiry date.
*
*/
public Optional sslCertExpiryDate() {
return Optional.ofNullable(this.sslCertExpiryDate);
}
/**
* @return CS SSL cert expiry date.
*
*/
public Optional sslCertExpiryRemainingDays() {
return Optional.ofNullable(this.sslCertExpiryRemainingDays);
}
/**
* @return The switch provider blocking error information.
*
*/
public List switchProviderBlockingErrorDetails() {
return this.switchProviderBlockingErrorDetails == null ? List.of() : this.switchProviderBlockingErrorDetails;
}
/**
* @return The percentage of the system load.
*
*/
public Optional systemLoad() {
return Optional.ofNullable(this.systemLoad);
}
/**
* @return The system load status.
*
*/
public Optional systemLoadStatus() {
return Optional.ofNullable(this.systemLoadStatus);
}
/**
* @return The total memory.
*
*/
public Optional totalMemoryInBytes() {
return Optional.ofNullable(this.totalMemoryInBytes);
}
/**
* @return The total space.
*
*/
public Optional totalSpaceInBytes() {
return Optional.ofNullable(this.totalSpaceInBytes);
}
/**
* @return Version status.
*
*/
public Optional versionStatus() {
return Optional.ofNullable(this.versionStatus);
}
/**
* @return The web load.
*
*/
public Optional webLoad() {
return Optional.ofNullable(this.webLoad);
}
/**
* @return The web load status.
*
*/
public Optional webLoadStatus() {
return Optional.ofNullable(this.webLoadStatus);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(VMwareDetailsResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable String agentCount;
private @Nullable String agentExpiryDate;
private @Nullable String agentVersion;
private @Nullable VersionDetailsResponse agentVersionDetails;
private @Nullable Double availableMemoryInBytes;
private @Nullable Double availableSpaceInBytes;
private @Nullable String cpuLoad;
private @Nullable String cpuLoadStatus;
private @Nullable String csServiceStatus;
private @Nullable String databaseServerLoad;
private @Nullable String databaseServerLoadStatus;
private @Nullable String hostName;
private String instanceType;
private @Nullable String ipAddress;
private @Nullable String lastHeartbeat;
private @Nullable List masterTargetServers;
private @Nullable String memoryUsageStatus;
private @Nullable String processServerCount;
private @Nullable List processServers;
private @Nullable String protectedServers;
private @Nullable String psTemplateVersion;
private @Nullable String replicationPairCount;
private @Nullable List runAsAccounts;
private @Nullable String spaceUsageStatus;
private @Nullable String sslCertExpiryDate;
private @Nullable Integer sslCertExpiryRemainingDays;
private @Nullable List switchProviderBlockingErrorDetails;
private @Nullable String systemLoad;
private @Nullable String systemLoadStatus;
private @Nullable Double totalMemoryInBytes;
private @Nullable Double totalSpaceInBytes;
private @Nullable String versionStatus;
private @Nullable String webLoad;
private @Nullable String webLoadStatus;
public Builder() {}
public Builder(VMwareDetailsResponse defaults) {
Objects.requireNonNull(defaults);
this.agentCount = defaults.agentCount;
this.agentExpiryDate = defaults.agentExpiryDate;
this.agentVersion = defaults.agentVersion;
this.agentVersionDetails = defaults.agentVersionDetails;
this.availableMemoryInBytes = defaults.availableMemoryInBytes;
this.availableSpaceInBytes = defaults.availableSpaceInBytes;
this.cpuLoad = defaults.cpuLoad;
this.cpuLoadStatus = defaults.cpuLoadStatus;
this.csServiceStatus = defaults.csServiceStatus;
this.databaseServerLoad = defaults.databaseServerLoad;
this.databaseServerLoadStatus = defaults.databaseServerLoadStatus;
this.hostName = defaults.hostName;
this.instanceType = defaults.instanceType;
this.ipAddress = defaults.ipAddress;
this.lastHeartbeat = defaults.lastHeartbeat;
this.masterTargetServers = defaults.masterTargetServers;
this.memoryUsageStatus = defaults.memoryUsageStatus;
this.processServerCount = defaults.processServerCount;
this.processServers = defaults.processServers;
this.protectedServers = defaults.protectedServers;
this.psTemplateVersion = defaults.psTemplateVersion;
this.replicationPairCount = defaults.replicationPairCount;
this.runAsAccounts = defaults.runAsAccounts;
this.spaceUsageStatus = defaults.spaceUsageStatus;
this.sslCertExpiryDate = defaults.sslCertExpiryDate;
this.sslCertExpiryRemainingDays = defaults.sslCertExpiryRemainingDays;
this.switchProviderBlockingErrorDetails = defaults.switchProviderBlockingErrorDetails;
this.systemLoad = defaults.systemLoad;
this.systemLoadStatus = defaults.systemLoadStatus;
this.totalMemoryInBytes = defaults.totalMemoryInBytes;
this.totalSpaceInBytes = defaults.totalSpaceInBytes;
this.versionStatus = defaults.versionStatus;
this.webLoad = defaults.webLoad;
this.webLoadStatus = defaults.webLoadStatus;
}
@CustomType.Setter
public Builder agentCount(@Nullable String agentCount) {
this.agentCount = agentCount;
return this;
}
@CustomType.Setter
public Builder agentExpiryDate(@Nullable String agentExpiryDate) {
this.agentExpiryDate = agentExpiryDate;
return this;
}
@CustomType.Setter
public Builder agentVersion(@Nullable String agentVersion) {
this.agentVersion = agentVersion;
return this;
}
@CustomType.Setter
public Builder agentVersionDetails(@Nullable VersionDetailsResponse agentVersionDetails) {
this.agentVersionDetails = agentVersionDetails;
return this;
}
@CustomType.Setter
public Builder availableMemoryInBytes(@Nullable Double availableMemoryInBytes) {
this.availableMemoryInBytes = availableMemoryInBytes;
return this;
}
@CustomType.Setter
public Builder availableSpaceInBytes(@Nullable Double availableSpaceInBytes) {
this.availableSpaceInBytes = availableSpaceInBytes;
return this;
}
@CustomType.Setter
public Builder cpuLoad(@Nullable String cpuLoad) {
this.cpuLoad = cpuLoad;
return this;
}
@CustomType.Setter
public Builder cpuLoadStatus(@Nullable String cpuLoadStatus) {
this.cpuLoadStatus = cpuLoadStatus;
return this;
}
@CustomType.Setter
public Builder csServiceStatus(@Nullable String csServiceStatus) {
this.csServiceStatus = csServiceStatus;
return this;
}
@CustomType.Setter
public Builder databaseServerLoad(@Nullable String databaseServerLoad) {
this.databaseServerLoad = databaseServerLoad;
return this;
}
@CustomType.Setter
public Builder databaseServerLoadStatus(@Nullable String databaseServerLoadStatus) {
this.databaseServerLoadStatus = databaseServerLoadStatus;
return this;
}
@CustomType.Setter
public Builder hostName(@Nullable String hostName) {
this.hostName = hostName;
return this;
}
@CustomType.Setter
public Builder instanceType(String instanceType) {
if (instanceType == null) {
throw new MissingRequiredPropertyException("VMwareDetailsResponse", "instanceType");
}
this.instanceType = instanceType;
return this;
}
@CustomType.Setter
public Builder ipAddress(@Nullable String ipAddress) {
this.ipAddress = ipAddress;
return this;
}
@CustomType.Setter
public Builder lastHeartbeat(@Nullable String lastHeartbeat) {
this.lastHeartbeat = lastHeartbeat;
return this;
}
@CustomType.Setter
public Builder masterTargetServers(@Nullable List masterTargetServers) {
this.masterTargetServers = masterTargetServers;
return this;
}
public Builder masterTargetServers(MasterTargetServerResponse... masterTargetServers) {
return masterTargetServers(List.of(masterTargetServers));
}
@CustomType.Setter
public Builder memoryUsageStatus(@Nullable String memoryUsageStatus) {
this.memoryUsageStatus = memoryUsageStatus;
return this;
}
@CustomType.Setter
public Builder processServerCount(@Nullable String processServerCount) {
this.processServerCount = processServerCount;
return this;
}
@CustomType.Setter
public Builder processServers(@Nullable List processServers) {
this.processServers = processServers;
return this;
}
public Builder processServers(ProcessServerResponse... processServers) {
return processServers(List.of(processServers));
}
@CustomType.Setter
public Builder protectedServers(@Nullable String protectedServers) {
this.protectedServers = protectedServers;
return this;
}
@CustomType.Setter
public Builder psTemplateVersion(@Nullable String psTemplateVersion) {
this.psTemplateVersion = psTemplateVersion;
return this;
}
@CustomType.Setter
public Builder replicationPairCount(@Nullable String replicationPairCount) {
this.replicationPairCount = replicationPairCount;
return this;
}
@CustomType.Setter
public Builder runAsAccounts(@Nullable List runAsAccounts) {
this.runAsAccounts = runAsAccounts;
return this;
}
public Builder runAsAccounts(RunAsAccountResponse... runAsAccounts) {
return runAsAccounts(List.of(runAsAccounts));
}
@CustomType.Setter
public Builder spaceUsageStatus(@Nullable String spaceUsageStatus) {
this.spaceUsageStatus = spaceUsageStatus;
return this;
}
@CustomType.Setter
public Builder sslCertExpiryDate(@Nullable String sslCertExpiryDate) {
this.sslCertExpiryDate = sslCertExpiryDate;
return this;
}
@CustomType.Setter
public Builder sslCertExpiryRemainingDays(@Nullable Integer sslCertExpiryRemainingDays) {
this.sslCertExpiryRemainingDays = sslCertExpiryRemainingDays;
return this;
}
@CustomType.Setter
public Builder switchProviderBlockingErrorDetails(@Nullable List switchProviderBlockingErrorDetails) {
this.switchProviderBlockingErrorDetails = switchProviderBlockingErrorDetails;
return this;
}
public Builder switchProviderBlockingErrorDetails(InMageFabricSwitchProviderBlockingErrorDetailsResponse... switchProviderBlockingErrorDetails) {
return switchProviderBlockingErrorDetails(List.of(switchProviderBlockingErrorDetails));
}
@CustomType.Setter
public Builder systemLoad(@Nullable String systemLoad) {
this.systemLoad = systemLoad;
return this;
}
@CustomType.Setter
public Builder systemLoadStatus(@Nullable String systemLoadStatus) {
this.systemLoadStatus = systemLoadStatus;
return this;
}
@CustomType.Setter
public Builder totalMemoryInBytes(@Nullable Double totalMemoryInBytes) {
this.totalMemoryInBytes = totalMemoryInBytes;
return this;
}
@CustomType.Setter
public Builder totalSpaceInBytes(@Nullable Double totalSpaceInBytes) {
this.totalSpaceInBytes = totalSpaceInBytes;
return this;
}
@CustomType.Setter
public Builder versionStatus(@Nullable String versionStatus) {
this.versionStatus = versionStatus;
return this;
}
@CustomType.Setter
public Builder webLoad(@Nullable String webLoad) {
this.webLoad = webLoad;
return this;
}
@CustomType.Setter
public Builder webLoadStatus(@Nullable String webLoadStatus) {
this.webLoadStatus = webLoadStatus;
return this;
}
public VMwareDetailsResponse build() {
final var _resultValue = new VMwareDetailsResponse();
_resultValue.agentCount = agentCount;
_resultValue.agentExpiryDate = agentExpiryDate;
_resultValue.agentVersion = agentVersion;
_resultValue.agentVersionDetails = agentVersionDetails;
_resultValue.availableMemoryInBytes = availableMemoryInBytes;
_resultValue.availableSpaceInBytes = availableSpaceInBytes;
_resultValue.cpuLoad = cpuLoad;
_resultValue.cpuLoadStatus = cpuLoadStatus;
_resultValue.csServiceStatus = csServiceStatus;
_resultValue.databaseServerLoad = databaseServerLoad;
_resultValue.databaseServerLoadStatus = databaseServerLoadStatus;
_resultValue.hostName = hostName;
_resultValue.instanceType = instanceType;
_resultValue.ipAddress = ipAddress;
_resultValue.lastHeartbeat = lastHeartbeat;
_resultValue.masterTargetServers = masterTargetServers;
_resultValue.memoryUsageStatus = memoryUsageStatus;
_resultValue.processServerCount = processServerCount;
_resultValue.processServers = processServers;
_resultValue.protectedServers = protectedServers;
_resultValue.psTemplateVersion = psTemplateVersion;
_resultValue.replicationPairCount = replicationPairCount;
_resultValue.runAsAccounts = runAsAccounts;
_resultValue.spaceUsageStatus = spaceUsageStatus;
_resultValue.sslCertExpiryDate = sslCertExpiryDate;
_resultValue.sslCertExpiryRemainingDays = sslCertExpiryRemainingDays;
_resultValue.switchProviderBlockingErrorDetails = switchProviderBlockingErrorDetails;
_resultValue.systemLoad = systemLoad;
_resultValue.systemLoadStatus = systemLoadStatus;
_resultValue.totalMemoryInBytes = totalMemoryInBytes;
_resultValue.totalSpaceInBytes = totalSpaceInBytes;
_resultValue.versionStatus = versionStatus;
_resultValue.webLoad = webLoad;
_resultValue.webLoadStatus = webLoadStatus;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy