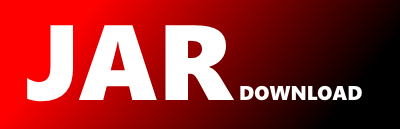
com.pulumi.azurenative.resources.outputs.GetDeploymentStackAtManagementGroupResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.resources.outputs;
import com.pulumi.azurenative.resources.outputs.DenySettingsResponse;
import com.pulumi.azurenative.resources.outputs.DeploymentStackPropertiesResponseActionOnUnmanage;
import com.pulumi.azurenative.resources.outputs.DeploymentStacksDebugSettingResponse;
import com.pulumi.azurenative.resources.outputs.DeploymentStacksParametersLinkResponse;
import com.pulumi.azurenative.resources.outputs.ErrorResponseResponse;
import com.pulumi.azurenative.resources.outputs.ManagedResourceReferenceResponse;
import com.pulumi.azurenative.resources.outputs.ResourceReferenceExtendedResponse;
import com.pulumi.azurenative.resources.outputs.ResourceReferenceResponse;
import com.pulumi.azurenative.resources.outputs.SystemDataResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Object;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class GetDeploymentStackAtManagementGroupResult {
/**
* @return Defines the behavior of resources that are not managed immediately after the stack is updated.
*
*/
private DeploymentStackPropertiesResponseActionOnUnmanage actionOnUnmanage;
/**
* @return The debug setting of the deployment.
*
*/
private @Nullable DeploymentStacksDebugSettingResponse debugSetting;
/**
* @return An array of resources that were deleted during the most recent update.
*
*/
private List deletedResources;
/**
* @return Defines how resources deployed by the stack are locked.
*
*/
private DenySettingsResponse denySettings;
/**
* @return The resourceId of the deployment resource created by the deployment stack.
*
*/
private String deploymentId;
/**
* @return The scope at which the initial deployment should be created. If a scope is not specified, it will default to the scope of the deployment stack. Valid scopes are: management group (format: '/providers/Microsoft.Management/managementGroups/{managementGroupId}'), subscription (format: '/subscriptions/{subscriptionId}'), resource group (format: '/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}').
*
*/
private @Nullable String deploymentScope;
/**
* @return Deployment stack description.
*
*/
private @Nullable String description;
/**
* @return An array of resources that were detached during the most recent update.
*
*/
private List detachedResources;
/**
* @return The duration of the deployment stack update.
*
*/
private String duration;
/**
* @return Common error response for all Azure Resource Manager APIs to return error details for failed operations. (This also follows the OData error response format.).
*
*/
private @Nullable ErrorResponseResponse error;
/**
* @return An array of resources that failed to reach goal state during the most recent update.
*
*/
private List failedResources;
/**
* @return String Id used to locate any resource on Azure.
*
*/
private String id;
/**
* @return The location of the deployment stack. It cannot be changed after creation. It must be one of the supported Azure locations.
*
*/
private @Nullable String location;
/**
* @return Name of this resource.
*
*/
private String name;
/**
* @return The outputs of the underlying deployment.
*
*/
private Object outputs;
/**
* @return Name and value pairs that define the deployment parameters for the template. Use this element when providing the parameter values directly in the request, rather than linking to an existing parameter file. Use either the parametersLink property or the parameters property, but not both. It can be a JObject or a well formed JSON string.
*
*/
private @Nullable Object parameters;
/**
* @return The URI of parameters file. Use this element to link to an existing parameters file. Use either the parametersLink property or the parameters property, but not both.
*
*/
private @Nullable DeploymentStacksParametersLinkResponse parametersLink;
/**
* @return State of the deployment stack.
*
*/
private String provisioningState;
/**
* @return An array of resources currently managed by the deployment stack.
*
*/
private List resources;
/**
* @return Azure Resource Manager metadata containing createdBy and modifiedBy information.
*
*/
private SystemDataResponse systemData;
/**
* @return Deployment stack resource tags.
*
*/
private @Nullable Map tags;
/**
* @return Type of this resource.
*
*/
private String type;
private GetDeploymentStackAtManagementGroupResult() {}
/**
* @return Defines the behavior of resources that are not managed immediately after the stack is updated.
*
*/
public DeploymentStackPropertiesResponseActionOnUnmanage actionOnUnmanage() {
return this.actionOnUnmanage;
}
/**
* @return The debug setting of the deployment.
*
*/
public Optional debugSetting() {
return Optional.ofNullable(this.debugSetting);
}
/**
* @return An array of resources that were deleted during the most recent update.
*
*/
public List deletedResources() {
return this.deletedResources;
}
/**
* @return Defines how resources deployed by the stack are locked.
*
*/
public DenySettingsResponse denySettings() {
return this.denySettings;
}
/**
* @return The resourceId of the deployment resource created by the deployment stack.
*
*/
public String deploymentId() {
return this.deploymentId;
}
/**
* @return The scope at which the initial deployment should be created. If a scope is not specified, it will default to the scope of the deployment stack. Valid scopes are: management group (format: '/providers/Microsoft.Management/managementGroups/{managementGroupId}'), subscription (format: '/subscriptions/{subscriptionId}'), resource group (format: '/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}').
*
*/
public Optional deploymentScope() {
return Optional.ofNullable(this.deploymentScope);
}
/**
* @return Deployment stack description.
*
*/
public Optional description() {
return Optional.ofNullable(this.description);
}
/**
* @return An array of resources that were detached during the most recent update.
*
*/
public List detachedResources() {
return this.detachedResources;
}
/**
* @return The duration of the deployment stack update.
*
*/
public String duration() {
return this.duration;
}
/**
* @return Common error response for all Azure Resource Manager APIs to return error details for failed operations. (This also follows the OData error response format.).
*
*/
public Optional error() {
return Optional.ofNullable(this.error);
}
/**
* @return An array of resources that failed to reach goal state during the most recent update.
*
*/
public List failedResources() {
return this.failedResources;
}
/**
* @return String Id used to locate any resource on Azure.
*
*/
public String id() {
return this.id;
}
/**
* @return The location of the deployment stack. It cannot be changed after creation. It must be one of the supported Azure locations.
*
*/
public Optional location() {
return Optional.ofNullable(this.location);
}
/**
* @return Name of this resource.
*
*/
public String name() {
return this.name;
}
/**
* @return The outputs of the underlying deployment.
*
*/
public Object outputs() {
return this.outputs;
}
/**
* @return Name and value pairs that define the deployment parameters for the template. Use this element when providing the parameter values directly in the request, rather than linking to an existing parameter file. Use either the parametersLink property or the parameters property, but not both. It can be a JObject or a well formed JSON string.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy