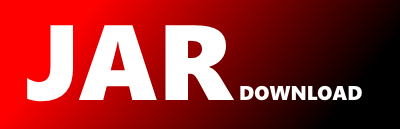
com.pulumi.azurenative.scvmm.outputs.InfrastructureProfileResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.scvmm.outputs;
import com.pulumi.azurenative.scvmm.outputs.CheckpointResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class InfrastructureProfileResponse {
/**
* @return Gets or sets the bios guid for the vm.
*
*/
private @Nullable String biosGuid;
/**
* @return Type of checkpoint supported for the vm.
*
*/
private @Nullable String checkpointType;
/**
* @return Checkpoints in the vm.
*
*/
private @Nullable List checkpoints;
/**
* @return ARM Id of the cloud resource to use for deploying the vm.
*
*/
private @Nullable String cloudId;
/**
* @return Gets or sets the generation for the vm.
*
*/
private @Nullable Integer generation;
/**
* @return Gets or sets the inventory Item ID for the resource.
*
*/
private @Nullable String inventoryItemId;
/**
* @return Last restored checkpoint in the vm.
*
*/
private CheckpointResponse lastRestoredVMCheckpoint;
/**
* @return ARM Id of the template resource to use for deploying the vm.
*
*/
private @Nullable String templateId;
/**
* @return Unique ID of the virtual machine.
*
*/
private @Nullable String uuid;
/**
* @return VMName is the name of VM on the SCVMM server.
*
*/
private @Nullable String vmName;
/**
* @return ARM Id of the vmmServer resource in which this resource resides.
*
*/
private @Nullable String vmmServerId;
private InfrastructureProfileResponse() {}
/**
* @return Gets or sets the bios guid for the vm.
*
*/
public Optional biosGuid() {
return Optional.ofNullable(this.biosGuid);
}
/**
* @return Type of checkpoint supported for the vm.
*
*/
public Optional checkpointType() {
return Optional.ofNullable(this.checkpointType);
}
/**
* @return Checkpoints in the vm.
*
*/
public List checkpoints() {
return this.checkpoints == null ? List.of() : this.checkpoints;
}
/**
* @return ARM Id of the cloud resource to use for deploying the vm.
*
*/
public Optional cloudId() {
return Optional.ofNullable(this.cloudId);
}
/**
* @return Gets or sets the generation for the vm.
*
*/
public Optional generation() {
return Optional.ofNullable(this.generation);
}
/**
* @return Gets or sets the inventory Item ID for the resource.
*
*/
public Optional inventoryItemId() {
return Optional.ofNullable(this.inventoryItemId);
}
/**
* @return Last restored checkpoint in the vm.
*
*/
public CheckpointResponse lastRestoredVMCheckpoint() {
return this.lastRestoredVMCheckpoint;
}
/**
* @return ARM Id of the template resource to use for deploying the vm.
*
*/
public Optional templateId() {
return Optional.ofNullable(this.templateId);
}
/**
* @return Unique ID of the virtual machine.
*
*/
public Optional uuid() {
return Optional.ofNullable(this.uuid);
}
/**
* @return VMName is the name of VM on the SCVMM server.
*
*/
public Optional vmName() {
return Optional.ofNullable(this.vmName);
}
/**
* @return ARM Id of the vmmServer resource in which this resource resides.
*
*/
public Optional vmmServerId() {
return Optional.ofNullable(this.vmmServerId);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(InfrastructureProfileResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable String biosGuid;
private @Nullable String checkpointType;
private @Nullable List checkpoints;
private @Nullable String cloudId;
private @Nullable Integer generation;
private @Nullable String inventoryItemId;
private CheckpointResponse lastRestoredVMCheckpoint;
private @Nullable String templateId;
private @Nullable String uuid;
private @Nullable String vmName;
private @Nullable String vmmServerId;
public Builder() {}
public Builder(InfrastructureProfileResponse defaults) {
Objects.requireNonNull(defaults);
this.biosGuid = defaults.biosGuid;
this.checkpointType = defaults.checkpointType;
this.checkpoints = defaults.checkpoints;
this.cloudId = defaults.cloudId;
this.generation = defaults.generation;
this.inventoryItemId = defaults.inventoryItemId;
this.lastRestoredVMCheckpoint = defaults.lastRestoredVMCheckpoint;
this.templateId = defaults.templateId;
this.uuid = defaults.uuid;
this.vmName = defaults.vmName;
this.vmmServerId = defaults.vmmServerId;
}
@CustomType.Setter
public Builder biosGuid(@Nullable String biosGuid) {
this.biosGuid = biosGuid;
return this;
}
@CustomType.Setter
public Builder checkpointType(@Nullable String checkpointType) {
this.checkpointType = checkpointType;
return this;
}
@CustomType.Setter
public Builder checkpoints(@Nullable List checkpoints) {
this.checkpoints = checkpoints;
return this;
}
public Builder checkpoints(CheckpointResponse... checkpoints) {
return checkpoints(List.of(checkpoints));
}
@CustomType.Setter
public Builder cloudId(@Nullable String cloudId) {
this.cloudId = cloudId;
return this;
}
@CustomType.Setter
public Builder generation(@Nullable Integer generation) {
this.generation = generation;
return this;
}
@CustomType.Setter
public Builder inventoryItemId(@Nullable String inventoryItemId) {
this.inventoryItemId = inventoryItemId;
return this;
}
@CustomType.Setter
public Builder lastRestoredVMCheckpoint(CheckpointResponse lastRestoredVMCheckpoint) {
if (lastRestoredVMCheckpoint == null) {
throw new MissingRequiredPropertyException("InfrastructureProfileResponse", "lastRestoredVMCheckpoint");
}
this.lastRestoredVMCheckpoint = lastRestoredVMCheckpoint;
return this;
}
@CustomType.Setter
public Builder templateId(@Nullable String templateId) {
this.templateId = templateId;
return this;
}
@CustomType.Setter
public Builder uuid(@Nullable String uuid) {
this.uuid = uuid;
return this;
}
@CustomType.Setter
public Builder vmName(@Nullable String vmName) {
this.vmName = vmName;
return this;
}
@CustomType.Setter
public Builder vmmServerId(@Nullable String vmmServerId) {
this.vmmServerId = vmmServerId;
return this;
}
public InfrastructureProfileResponse build() {
final var _resultValue = new InfrastructureProfileResponse();
_resultValue.biosGuid = biosGuid;
_resultValue.checkpointType = checkpointType;
_resultValue.checkpoints = checkpoints;
_resultValue.cloudId = cloudId;
_resultValue.generation = generation;
_resultValue.inventoryItemId = inventoryItemId;
_resultValue.lastRestoredVMCheckpoint = lastRestoredVMCheckpoint;
_resultValue.templateId = templateId;
_resultValue.uuid = uuid;
_resultValue.vmName = vmName;
_resultValue.vmmServerId = vmmServerId;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy