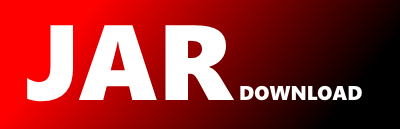
com.pulumi.azurenative.security.APICollectionByAzureApiManagementService Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.security;
import com.pulumi.azurenative.Utilities;
import com.pulumi.azurenative.security.APICollectionByAzureApiManagementServiceArgs;
import com.pulumi.core.Alias;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.Double;
import java.lang.String;
import java.util.List;
import javax.annotation.Nullable;
/**
* An API collection as represented by Microsoft Defender for APIs.
* Azure REST API version: 2023-11-15.
*
* ## Example Usage
* ### Onboard an Azure API Management API to Microsoft Defender for APIs
*
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.security.APICollectionByAzureApiManagementService;
* import com.pulumi.azurenative.security.APICollectionByAzureApiManagementServiceArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var apiCollectionByAzureApiManagementService = new APICollectionByAzureApiManagementService("apiCollectionByAzureApiManagementService", APICollectionByAzureApiManagementServiceArgs.builder()
* .apiId("echo-api")
* .resourceGroupName("rg1")
* .serviceName("apimService1")
* .build());
*
* }
* }
*
* }
*
*
* ## Import
*
* An existing resource can be imported using its type token, name, and identifier, e.g.
*
* ```sh
* $ pulumi import azure-native:security:APICollectionByAzureApiManagementService echo-api /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.ApiManagement/service/{serviceName}/providers/Microsoft.Security/apiCollections/{apiId}
* ```
*
*/
@ResourceType(type="azure-native:security:APICollectionByAzureApiManagementService")
public class APICollectionByAzureApiManagementService extends com.pulumi.resources.CustomResource {
/**
* The base URI for this API collection. All endpoints of this API collection extend this base URI.
*
*/
@Export(name="baseUrl", refs={String.class}, tree="[0]")
private Output baseUrl;
/**
* @return The base URI for this API collection. All endpoints of this API collection extend this base URI.
*
*/
public Output baseUrl() {
return this.baseUrl;
}
/**
* The resource Id of the resource from where this API collection was discovered.
*
*/
@Export(name="discoveredVia", refs={String.class}, tree="[0]")
private Output discoveredVia;
/**
* @return The resource Id of the resource from where this API collection was discovered.
*
*/
public Output discoveredVia() {
return this.discoveredVia;
}
/**
* The display name of the API collection.
*
*/
@Export(name="displayName", refs={String.class}, tree="[0]")
private Output displayName;
/**
* @return The display name of the API collection.
*
*/
public Output displayName() {
return this.displayName;
}
/**
* Resource name
*
*/
@Export(name="name", refs={String.class}, tree="[0]")
private Output name;
/**
* @return Resource name
*
*/
public Output name() {
return this.name;
}
/**
* The number of API endpoints discovered in this API collection.
*
*/
@Export(name="numberOfApiEndpoints", refs={Double.class}, tree="[0]")
private Output numberOfApiEndpoints;
/**
* @return The number of API endpoints discovered in this API collection.
*
*/
public Output numberOfApiEndpoints() {
return this.numberOfApiEndpoints;
}
/**
* The number of API endpoints in this API collection which are exposing sensitive data in their requests and/or responses.
*
*/
@Export(name="numberOfApiEndpointsWithSensitiveDataExposed", refs={Double.class}, tree="[0]")
private Output numberOfApiEndpointsWithSensitiveDataExposed;
/**
* @return The number of API endpoints in this API collection which are exposing sensitive data in their requests and/or responses.
*
*/
public Output numberOfApiEndpointsWithSensitiveDataExposed() {
return this.numberOfApiEndpointsWithSensitiveDataExposed;
}
/**
* The number of API endpoints in this API collection for which API traffic from the internet was observed.
*
*/
@Export(name="numberOfExternalApiEndpoints", refs={Double.class}, tree="[0]")
private Output numberOfExternalApiEndpoints;
/**
* @return The number of API endpoints in this API collection for which API traffic from the internet was observed.
*
*/
public Output numberOfExternalApiEndpoints() {
return this.numberOfExternalApiEndpoints;
}
/**
* The number of API endpoints in this API collection that have not received any API traffic in the last 30 days.
*
*/
@Export(name="numberOfInactiveApiEndpoints", refs={Double.class}, tree="[0]")
private Output numberOfInactiveApiEndpoints;
/**
* @return The number of API endpoints in this API collection that have not received any API traffic in the last 30 days.
*
*/
public Output numberOfInactiveApiEndpoints() {
return this.numberOfInactiveApiEndpoints;
}
/**
* The number of API endpoints in this API collection that are unauthenticated.
*
*/
@Export(name="numberOfUnauthenticatedApiEndpoints", refs={Double.class}, tree="[0]")
private Output numberOfUnauthenticatedApiEndpoints;
/**
* @return The number of API endpoints in this API collection that are unauthenticated.
*
*/
public Output numberOfUnauthenticatedApiEndpoints() {
return this.numberOfUnauthenticatedApiEndpoints;
}
/**
* Gets the provisioning state of the API collection.
*
*/
@Export(name="provisioningState", refs={String.class}, tree="[0]")
private Output provisioningState;
/**
* @return Gets the provisioning state of the API collection.
*
*/
public Output provisioningState() {
return this.provisioningState;
}
/**
* The highest priority sensitivity label from Microsoft Purview in this API collection.
*
*/
@Export(name="sensitivityLabel", refs={String.class}, tree="[0]")
private Output sensitivityLabel;
/**
* @return The highest priority sensitivity label from Microsoft Purview in this API collection.
*
*/
public Output sensitivityLabel() {
return this.sensitivityLabel;
}
/**
* Resource type
*
*/
@Export(name="type", refs={String.class}, tree="[0]")
private Output type;
/**
* @return Resource type
*
*/
public Output type() {
return this.type;
}
/**
*
* @param name The _unique_ name of the resulting resource.
*/
public APICollectionByAzureApiManagementService(java.lang.String name) {
this(name, APICollectionByAzureApiManagementServiceArgs.Empty);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
*/
public APICollectionByAzureApiManagementService(java.lang.String name, APICollectionByAzureApiManagementServiceArgs args) {
this(name, args, null);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
* @param options A bag of options that control this resource's behavior.
*/
public APICollectionByAzureApiManagementService(java.lang.String name, APICollectionByAzureApiManagementServiceArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("azure-native:security:APICollectionByAzureApiManagementService", name, makeArgs(args, options), makeResourceOptions(options, Codegen.empty()), false);
}
private APICollectionByAzureApiManagementService(java.lang.String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("azure-native:security:APICollectionByAzureApiManagementService", name, null, makeResourceOptions(options, id), false);
}
private static APICollectionByAzureApiManagementServiceArgs makeArgs(APICollectionByAzureApiManagementServiceArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
if (options != null && options.getUrn().isPresent()) {
return null;
}
return args == null ? APICollectionByAzureApiManagementServiceArgs.Empty : args;
}
private static com.pulumi.resources.CustomResourceOptions makeResourceOptions(@Nullable com.pulumi.resources.CustomResourceOptions options, @Nullable Output id) {
var defaultOptions = com.pulumi.resources.CustomResourceOptions.builder()
.version(Utilities.getVersion())
.aliases(List.of(
Output.of(Alias.builder().type("azure-native:security/v20221120preview:APICollectionByAzureApiManagementService").build()),
Output.of(Alias.builder().type("azure-native:security/v20231115:APICollectionByAzureApiManagementService").build())
))
.build();
return com.pulumi.resources.CustomResourceOptions.merge(defaultOptions, options, id);
}
/**
* Get an existing Host resource's state with the given name, ID, and optional extra
* properties used to qualify the lookup.
*
* @param name The _unique_ name of the resulting resource.
* @param id The _unique_ provider ID of the resource to lookup.
* @param options Optional settings to control the behavior of the CustomResource.
*/
public static APICollectionByAzureApiManagementService get(java.lang.String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
return new APICollectionByAzureApiManagementService(name, id, options);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy