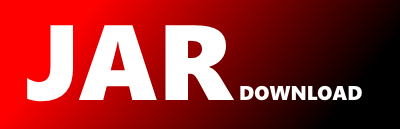
com.pulumi.azurenative.security.inputs.DefenderForServersGcpOfferingArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.security.inputs;
import com.pulumi.azurenative.security.inputs.DefenderForServersGcpOfferingArcAutoProvisioningArgs;
import com.pulumi.azurenative.security.inputs.DefenderForServersGcpOfferingDefenderForServersArgs;
import com.pulumi.azurenative.security.inputs.DefenderForServersGcpOfferingMdeAutoProvisioningArgs;
import com.pulumi.azurenative.security.inputs.DefenderForServersGcpOfferingSubPlanArgs;
import com.pulumi.azurenative.security.inputs.DefenderForServersGcpOfferingVaAutoProvisioningArgs;
import com.pulumi.azurenative.security.inputs.DefenderForServersGcpOfferingVmScannersArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.core.internal.Codegen;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* The Defender for Servers GCP offering configurations
*
*/
public final class DefenderForServersGcpOfferingArgs extends com.pulumi.resources.ResourceArgs {
public static final DefenderForServersGcpOfferingArgs Empty = new DefenderForServersGcpOfferingArgs();
/**
* The ARC autoprovisioning configuration
*
*/
@Import(name="arcAutoProvisioning")
private @Nullable Output arcAutoProvisioning;
/**
* @return The ARC autoprovisioning configuration
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy