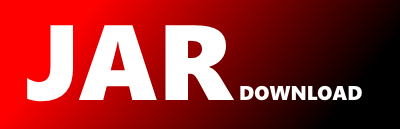
com.pulumi.azurenative.security.outputs.GetAlertsSuppressionRuleResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.security.outputs;
import com.pulumi.azurenative.security.outputs.SuppressionAlertsScopeResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class GetAlertsSuppressionRuleResult {
/**
* @return Type of the alert to automatically suppress. For all alert types, use '*'
*
*/
private String alertType;
/**
* @return Any comment regarding the rule
*
*/
private @Nullable String comment;
/**
* @return Expiration date of the rule, if value is not provided or provided as null there will no expiration at all
*
*/
private @Nullable String expirationDateUtc;
/**
* @return Resource Id
*
*/
private String id;
/**
* @return The last time this rule was modified
*
*/
private String lastModifiedUtc;
/**
* @return Resource name
*
*/
private String name;
/**
* @return The reason for dismissing the alert
*
*/
private String reason;
/**
* @return Possible states of the rule
*
*/
private String state;
/**
* @return The suppression conditions
*
*/
private @Nullable SuppressionAlertsScopeResponse suppressionAlertsScope;
/**
* @return Resource type
*
*/
private String type;
private GetAlertsSuppressionRuleResult() {}
/**
* @return Type of the alert to automatically suppress. For all alert types, use '*'
*
*/
public String alertType() {
return this.alertType;
}
/**
* @return Any comment regarding the rule
*
*/
public Optional comment() {
return Optional.ofNullable(this.comment);
}
/**
* @return Expiration date of the rule, if value is not provided or provided as null there will no expiration at all
*
*/
public Optional expirationDateUtc() {
return Optional.ofNullable(this.expirationDateUtc);
}
/**
* @return Resource Id
*
*/
public String id() {
return this.id;
}
/**
* @return The last time this rule was modified
*
*/
public String lastModifiedUtc() {
return this.lastModifiedUtc;
}
/**
* @return Resource name
*
*/
public String name() {
return this.name;
}
/**
* @return The reason for dismissing the alert
*
*/
public String reason() {
return this.reason;
}
/**
* @return Possible states of the rule
*
*/
public String state() {
return this.state;
}
/**
* @return The suppression conditions
*
*/
public Optional suppressionAlertsScope() {
return Optional.ofNullable(this.suppressionAlertsScope);
}
/**
* @return Resource type
*
*/
public String type() {
return this.type;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetAlertsSuppressionRuleResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String alertType;
private @Nullable String comment;
private @Nullable String expirationDateUtc;
private String id;
private String lastModifiedUtc;
private String name;
private String reason;
private String state;
private @Nullable SuppressionAlertsScopeResponse suppressionAlertsScope;
private String type;
public Builder() {}
public Builder(GetAlertsSuppressionRuleResult defaults) {
Objects.requireNonNull(defaults);
this.alertType = defaults.alertType;
this.comment = defaults.comment;
this.expirationDateUtc = defaults.expirationDateUtc;
this.id = defaults.id;
this.lastModifiedUtc = defaults.lastModifiedUtc;
this.name = defaults.name;
this.reason = defaults.reason;
this.state = defaults.state;
this.suppressionAlertsScope = defaults.suppressionAlertsScope;
this.type = defaults.type;
}
@CustomType.Setter
public Builder alertType(String alertType) {
if (alertType == null) {
throw new MissingRequiredPropertyException("GetAlertsSuppressionRuleResult", "alertType");
}
this.alertType = alertType;
return this;
}
@CustomType.Setter
public Builder comment(@Nullable String comment) {
this.comment = comment;
return this;
}
@CustomType.Setter
public Builder expirationDateUtc(@Nullable String expirationDateUtc) {
this.expirationDateUtc = expirationDateUtc;
return this;
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetAlertsSuppressionRuleResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder lastModifiedUtc(String lastModifiedUtc) {
if (lastModifiedUtc == null) {
throw new MissingRequiredPropertyException("GetAlertsSuppressionRuleResult", "lastModifiedUtc");
}
this.lastModifiedUtc = lastModifiedUtc;
return this;
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("GetAlertsSuppressionRuleResult", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder reason(String reason) {
if (reason == null) {
throw new MissingRequiredPropertyException("GetAlertsSuppressionRuleResult", "reason");
}
this.reason = reason;
return this;
}
@CustomType.Setter
public Builder state(String state) {
if (state == null) {
throw new MissingRequiredPropertyException("GetAlertsSuppressionRuleResult", "state");
}
this.state = state;
return this;
}
@CustomType.Setter
public Builder suppressionAlertsScope(@Nullable SuppressionAlertsScopeResponse suppressionAlertsScope) {
this.suppressionAlertsScope = suppressionAlertsScope;
return this;
}
@CustomType.Setter
public Builder type(String type) {
if (type == null) {
throw new MissingRequiredPropertyException("GetAlertsSuppressionRuleResult", "type");
}
this.type = type;
return this;
}
public GetAlertsSuppressionRuleResult build() {
final var _resultValue = new GetAlertsSuppressionRuleResult();
_resultValue.alertType = alertType;
_resultValue.comment = comment;
_resultValue.expirationDateUtc = expirationDateUtc;
_resultValue.id = id;
_resultValue.lastModifiedUtc = lastModifiedUtc;
_resultValue.name = name;
_resultValue.reason = reason;
_resultValue.state = state;
_resultValue.suppressionAlertsScope = suppressionAlertsScope;
_resultValue.type = type;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy