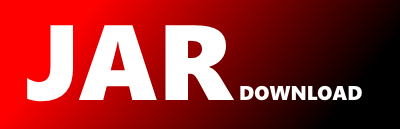
com.pulumi.azurenative.security.outputs.GetGovernanceAssignmentResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.security.outputs;
import com.pulumi.azurenative.security.outputs.GovernanceAssignmentAdditionalDataResponse;
import com.pulumi.azurenative.security.outputs.GovernanceEmailNotificationResponse;
import com.pulumi.azurenative.security.outputs.RemediationEtaResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class GetGovernanceAssignmentResult {
/**
* @return The additional data for the governance assignment - e.g. links to ticket (optional), see example
*
*/
private @Nullable GovernanceAssignmentAdditionalDataResponse additionalData;
/**
* @return The email notifications settings for the governance rule, states whether to disable notifications for mangers and owners
*
*/
private @Nullable GovernanceEmailNotificationResponse governanceEmailNotification;
/**
* @return Resource Id
*
*/
private String id;
/**
* @return Defines whether there is a grace period on the governance assignment
*
*/
private @Nullable Boolean isGracePeriod;
/**
* @return Resource name
*
*/
private String name;
/**
* @return The Owner for the governance assignment - e.g. user{@literal @}contoso.com - see example
*
*/
private @Nullable String owner;
/**
* @return The remediation due-date - after this date Secure Score will be affected (in case of active grace-period)
*
*/
private String remediationDueDate;
/**
* @return The ETA (estimated time of arrival) for remediation (optional), see example
*
*/
private @Nullable RemediationEtaResponse remediationEta;
/**
* @return Resource type
*
*/
private String type;
private GetGovernanceAssignmentResult() {}
/**
* @return The additional data for the governance assignment - e.g. links to ticket (optional), see example
*
*/
public Optional additionalData() {
return Optional.ofNullable(this.additionalData);
}
/**
* @return The email notifications settings for the governance rule, states whether to disable notifications for mangers and owners
*
*/
public Optional governanceEmailNotification() {
return Optional.ofNullable(this.governanceEmailNotification);
}
/**
* @return Resource Id
*
*/
public String id() {
return this.id;
}
/**
* @return Defines whether there is a grace period on the governance assignment
*
*/
public Optional isGracePeriod() {
return Optional.ofNullable(this.isGracePeriod);
}
/**
* @return Resource name
*
*/
public String name() {
return this.name;
}
/**
* @return The Owner for the governance assignment - e.g. user{@literal @}contoso.com - see example
*
*/
public Optional owner() {
return Optional.ofNullable(this.owner);
}
/**
* @return The remediation due-date - after this date Secure Score will be affected (in case of active grace-period)
*
*/
public String remediationDueDate() {
return this.remediationDueDate;
}
/**
* @return The ETA (estimated time of arrival) for remediation (optional), see example
*
*/
public Optional remediationEta() {
return Optional.ofNullable(this.remediationEta);
}
/**
* @return Resource type
*
*/
public String type() {
return this.type;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetGovernanceAssignmentResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable GovernanceAssignmentAdditionalDataResponse additionalData;
private @Nullable GovernanceEmailNotificationResponse governanceEmailNotification;
private String id;
private @Nullable Boolean isGracePeriod;
private String name;
private @Nullable String owner;
private String remediationDueDate;
private @Nullable RemediationEtaResponse remediationEta;
private String type;
public Builder() {}
public Builder(GetGovernanceAssignmentResult defaults) {
Objects.requireNonNull(defaults);
this.additionalData = defaults.additionalData;
this.governanceEmailNotification = defaults.governanceEmailNotification;
this.id = defaults.id;
this.isGracePeriod = defaults.isGracePeriod;
this.name = defaults.name;
this.owner = defaults.owner;
this.remediationDueDate = defaults.remediationDueDate;
this.remediationEta = defaults.remediationEta;
this.type = defaults.type;
}
@CustomType.Setter
public Builder additionalData(@Nullable GovernanceAssignmentAdditionalDataResponse additionalData) {
this.additionalData = additionalData;
return this;
}
@CustomType.Setter
public Builder governanceEmailNotification(@Nullable GovernanceEmailNotificationResponse governanceEmailNotification) {
this.governanceEmailNotification = governanceEmailNotification;
return this;
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetGovernanceAssignmentResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder isGracePeriod(@Nullable Boolean isGracePeriod) {
this.isGracePeriod = isGracePeriod;
return this;
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("GetGovernanceAssignmentResult", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder owner(@Nullable String owner) {
this.owner = owner;
return this;
}
@CustomType.Setter
public Builder remediationDueDate(String remediationDueDate) {
if (remediationDueDate == null) {
throw new MissingRequiredPropertyException("GetGovernanceAssignmentResult", "remediationDueDate");
}
this.remediationDueDate = remediationDueDate;
return this;
}
@CustomType.Setter
public Builder remediationEta(@Nullable RemediationEtaResponse remediationEta) {
this.remediationEta = remediationEta;
return this;
}
@CustomType.Setter
public Builder type(String type) {
if (type == null) {
throw new MissingRequiredPropertyException("GetGovernanceAssignmentResult", "type");
}
this.type = type;
return this;
}
public GetGovernanceAssignmentResult build() {
final var _resultValue = new GetGovernanceAssignmentResult();
_resultValue.additionalData = additionalData;
_resultValue.governanceEmailNotification = governanceEmailNotification;
_resultValue.id = id;
_resultValue.isGracePeriod = isGracePeriod;
_resultValue.name = name;
_resultValue.owner = owner;
_resultValue.remediationDueDate = remediationDueDate;
_resultValue.remediationEta = remediationEta;
_resultValue.type = type;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy