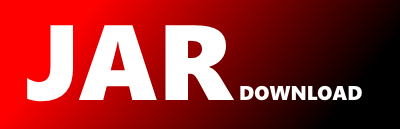
com.pulumi.azurenative.security.outputs.GitLabGroupPropertiesResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.security.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class GitLabGroupPropertiesResponse {
/**
* @return Gets or sets the human readable fully-qualified name of the Group object.
*
* This contains the entire namespace hierarchy as seen on GitLab UI where namespaces are separated by the '/' character.
*
*/
private String fullyQualifiedFriendlyName;
/**
* @return Gets or sets the fully-qualified name of the Group object.
*
* This contains the entire namespace hierarchy where namespaces are separated by the '$' character.
*
*/
private String fullyQualifiedName;
/**
* @return Details about resource onboarding status across all connectors.
*
* OnboardedByOtherConnector - this resource has already been onboarded to another connector. This is only applicable to top-level resources.
* Onboarded - this resource has already been onboarded by the specified connector.
* NotOnboarded - this resource has not been onboarded to any connector.
* NotApplicable - the onboarding state is not applicable to the current endpoint.
*
*/
private @Nullable String onboardingState;
/**
* @return The provisioning state of the resource.
*
* Pending - Provisioning pending.
* Failed - Provisioning failed.
* Succeeded - Successful provisioning.
* Canceled - Provisioning canceled.
* PendingDeletion - Deletion pending.
* DeletionSuccess - Deletion successful.
* DeletionFailure - Deletion failure.
*
*/
private @Nullable String provisioningState;
/**
* @return Gets or sets resource status message.
*
*/
private String provisioningStatusMessage;
/**
* @return Gets or sets time when resource was last checked.
*
*/
private String provisioningStatusUpdateTimeUtc;
/**
* @return Gets or sets the url of the GitLab Group.
*
*/
private String url;
private GitLabGroupPropertiesResponse() {}
/**
* @return Gets or sets the human readable fully-qualified name of the Group object.
*
* This contains the entire namespace hierarchy as seen on GitLab UI where namespaces are separated by the '/' character.
*
*/
public String fullyQualifiedFriendlyName() {
return this.fullyQualifiedFriendlyName;
}
/**
* @return Gets or sets the fully-qualified name of the Group object.
*
* This contains the entire namespace hierarchy where namespaces are separated by the '$' character.
*
*/
public String fullyQualifiedName() {
return this.fullyQualifiedName;
}
/**
* @return Details about resource onboarding status across all connectors.
*
* OnboardedByOtherConnector - this resource has already been onboarded to another connector. This is only applicable to top-level resources.
* Onboarded - this resource has already been onboarded by the specified connector.
* NotOnboarded - this resource has not been onboarded to any connector.
* NotApplicable - the onboarding state is not applicable to the current endpoint.
*
*/
public Optional onboardingState() {
return Optional.ofNullable(this.onboardingState);
}
/**
* @return The provisioning state of the resource.
*
* Pending - Provisioning pending.
* Failed - Provisioning failed.
* Succeeded - Successful provisioning.
* Canceled - Provisioning canceled.
* PendingDeletion - Deletion pending.
* DeletionSuccess - Deletion successful.
* DeletionFailure - Deletion failure.
*
*/
public Optional provisioningState() {
return Optional.ofNullable(this.provisioningState);
}
/**
* @return Gets or sets resource status message.
*
*/
public String provisioningStatusMessage() {
return this.provisioningStatusMessage;
}
/**
* @return Gets or sets time when resource was last checked.
*
*/
public String provisioningStatusUpdateTimeUtc() {
return this.provisioningStatusUpdateTimeUtc;
}
/**
* @return Gets or sets the url of the GitLab Group.
*
*/
public String url() {
return this.url;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GitLabGroupPropertiesResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String fullyQualifiedFriendlyName;
private String fullyQualifiedName;
private @Nullable String onboardingState;
private @Nullable String provisioningState;
private String provisioningStatusMessage;
private String provisioningStatusUpdateTimeUtc;
private String url;
public Builder() {}
public Builder(GitLabGroupPropertiesResponse defaults) {
Objects.requireNonNull(defaults);
this.fullyQualifiedFriendlyName = defaults.fullyQualifiedFriendlyName;
this.fullyQualifiedName = defaults.fullyQualifiedName;
this.onboardingState = defaults.onboardingState;
this.provisioningState = defaults.provisioningState;
this.provisioningStatusMessage = defaults.provisioningStatusMessage;
this.provisioningStatusUpdateTimeUtc = defaults.provisioningStatusUpdateTimeUtc;
this.url = defaults.url;
}
@CustomType.Setter
public Builder fullyQualifiedFriendlyName(String fullyQualifiedFriendlyName) {
if (fullyQualifiedFriendlyName == null) {
throw new MissingRequiredPropertyException("GitLabGroupPropertiesResponse", "fullyQualifiedFriendlyName");
}
this.fullyQualifiedFriendlyName = fullyQualifiedFriendlyName;
return this;
}
@CustomType.Setter
public Builder fullyQualifiedName(String fullyQualifiedName) {
if (fullyQualifiedName == null) {
throw new MissingRequiredPropertyException("GitLabGroupPropertiesResponse", "fullyQualifiedName");
}
this.fullyQualifiedName = fullyQualifiedName;
return this;
}
@CustomType.Setter
public Builder onboardingState(@Nullable String onboardingState) {
this.onboardingState = onboardingState;
return this;
}
@CustomType.Setter
public Builder provisioningState(@Nullable String provisioningState) {
this.provisioningState = provisioningState;
return this;
}
@CustomType.Setter
public Builder provisioningStatusMessage(String provisioningStatusMessage) {
if (provisioningStatusMessage == null) {
throw new MissingRequiredPropertyException("GitLabGroupPropertiesResponse", "provisioningStatusMessage");
}
this.provisioningStatusMessage = provisioningStatusMessage;
return this;
}
@CustomType.Setter
public Builder provisioningStatusUpdateTimeUtc(String provisioningStatusUpdateTimeUtc) {
if (provisioningStatusUpdateTimeUtc == null) {
throw new MissingRequiredPropertyException("GitLabGroupPropertiesResponse", "provisioningStatusUpdateTimeUtc");
}
this.provisioningStatusUpdateTimeUtc = provisioningStatusUpdateTimeUtc;
return this;
}
@CustomType.Setter
public Builder url(String url) {
if (url == null) {
throw new MissingRequiredPropertyException("GitLabGroupPropertiesResponse", "url");
}
this.url = url;
return this;
}
public GitLabGroupPropertiesResponse build() {
final var _resultValue = new GitLabGroupPropertiesResponse();
_resultValue.fullyQualifiedFriendlyName = fullyQualifiedFriendlyName;
_resultValue.fullyQualifiedName = fullyQualifiedName;
_resultValue.onboardingState = onboardingState;
_resultValue.provisioningState = provisioningState;
_resultValue.provisioningStatusMessage = provisioningStatusMessage;
_resultValue.provisioningStatusUpdateTimeUtc = provisioningStatusUpdateTimeUtc;
_resultValue.url = url;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy