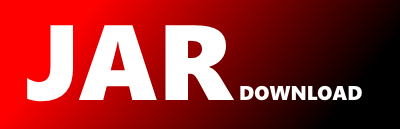
com.pulumi.azurenative.securityinsights.inputs.DeploymentArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.securityinsights.inputs;
import com.pulumi.azurenative.securityinsights.enums.DeploymentResult;
import com.pulumi.azurenative.securityinsights.enums.DeploymentState;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Description about a deployment.
*
*/
public final class DeploymentArgs extends com.pulumi.resources.ResourceArgs {
public static final DeploymentArgs Empty = new DeploymentArgs();
/**
* Deployment identifier.
*
*/
@Import(name="deploymentId")
private @Nullable Output deploymentId;
/**
* @return Deployment identifier.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy