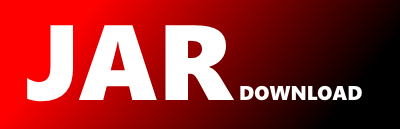
com.pulumi.azurenative.securityinsights.outputs.EnrichmentDomainWhoisContactResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.securityinsights.outputs;
import com.pulumi.core.annotations.CustomType;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class EnrichmentDomainWhoisContactResponse {
/**
* @return The city for this contact
*
*/
private @Nullable String city;
/**
* @return The country for this contact
*
*/
private @Nullable String country;
/**
* @return The email address for this contact
*
*/
private @Nullable String email;
/**
* @return The fax number for this contact
*
*/
private @Nullable String fax;
/**
* @return The name of this contact
*
*/
private @Nullable String name;
/**
* @return The organization for this contact
*
*/
private @Nullable String org;
/**
* @return The phone number for this contact
*
*/
private @Nullable String phone;
/**
* @return The postal code for this contact
*
*/
private @Nullable String postal;
/**
* @return The state for this contact
*
*/
private @Nullable String state;
/**
* @return A list describing the street address for this contact
*
*/
private @Nullable List street;
private EnrichmentDomainWhoisContactResponse() {}
/**
* @return The city for this contact
*
*/
public Optional city() {
return Optional.ofNullable(this.city);
}
/**
* @return The country for this contact
*
*/
public Optional country() {
return Optional.ofNullable(this.country);
}
/**
* @return The email address for this contact
*
*/
public Optional email() {
return Optional.ofNullable(this.email);
}
/**
* @return The fax number for this contact
*
*/
public Optional fax() {
return Optional.ofNullable(this.fax);
}
/**
* @return The name of this contact
*
*/
public Optional name() {
return Optional.ofNullable(this.name);
}
/**
* @return The organization for this contact
*
*/
public Optional org() {
return Optional.ofNullable(this.org);
}
/**
* @return The phone number for this contact
*
*/
public Optional phone() {
return Optional.ofNullable(this.phone);
}
/**
* @return The postal code for this contact
*
*/
public Optional postal() {
return Optional.ofNullable(this.postal);
}
/**
* @return The state for this contact
*
*/
public Optional state() {
return Optional.ofNullable(this.state);
}
/**
* @return A list describing the street address for this contact
*
*/
public List street() {
return this.street == null ? List.of() : this.street;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(EnrichmentDomainWhoisContactResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable String city;
private @Nullable String country;
private @Nullable String email;
private @Nullable String fax;
private @Nullable String name;
private @Nullable String org;
private @Nullable String phone;
private @Nullable String postal;
private @Nullable String state;
private @Nullable List street;
public Builder() {}
public Builder(EnrichmentDomainWhoisContactResponse defaults) {
Objects.requireNonNull(defaults);
this.city = defaults.city;
this.country = defaults.country;
this.email = defaults.email;
this.fax = defaults.fax;
this.name = defaults.name;
this.org = defaults.org;
this.phone = defaults.phone;
this.postal = defaults.postal;
this.state = defaults.state;
this.street = defaults.street;
}
@CustomType.Setter
public Builder city(@Nullable String city) {
this.city = city;
return this;
}
@CustomType.Setter
public Builder country(@Nullable String country) {
this.country = country;
return this;
}
@CustomType.Setter
public Builder email(@Nullable String email) {
this.email = email;
return this;
}
@CustomType.Setter
public Builder fax(@Nullable String fax) {
this.fax = fax;
return this;
}
@CustomType.Setter
public Builder name(@Nullable String name) {
this.name = name;
return this;
}
@CustomType.Setter
public Builder org(@Nullable String org) {
this.org = org;
return this;
}
@CustomType.Setter
public Builder phone(@Nullable String phone) {
this.phone = phone;
return this;
}
@CustomType.Setter
public Builder postal(@Nullable String postal) {
this.postal = postal;
return this;
}
@CustomType.Setter
public Builder state(@Nullable String state) {
this.state = state;
return this;
}
@CustomType.Setter
public Builder street(@Nullable List street) {
this.street = street;
return this;
}
public Builder street(String... street) {
return street(List.of(street));
}
public EnrichmentDomainWhoisContactResponse build() {
final var _resultValue = new EnrichmentDomainWhoisContactResponse();
_resultValue.city = city;
_resultValue.country = country;
_resultValue.email = email;
_resultValue.fax = fax;
_resultValue.name = name;
_resultValue.org = org;
_resultValue.phone = phone;
_resultValue.postal = postal;
_resultValue.state = state;
_resultValue.street = street;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy