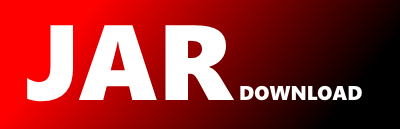
com.pulumi.azurenative.securityinsights.outputs.GetFileImportResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.securityinsights.outputs;
import com.pulumi.azurenative.securityinsights.outputs.FileMetadataResponse;
import com.pulumi.azurenative.securityinsights.outputs.SystemDataResponse;
import com.pulumi.azurenative.securityinsights.outputs.ValidationErrorResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Objects;
@CustomType
public final class GetFileImportResult {
/**
* @return The content type of this file.
*
*/
private String contentType;
/**
* @return The time the file was imported.
*
*/
private String createdTimeUTC;
/**
* @return Represents the error file (if the import was ingested with errors or failed the validation).
*
*/
private FileMetadataResponse errorFile;
/**
* @return An ordered list of some of the errors that were encountered during validation.
*
*/
private List errorsPreview;
/**
* @return The time the files associated with this import are deleted from the storage account.
*
*/
private String filesValidUntilTimeUTC;
/**
* @return Fully qualified resource ID for the resource. Ex - /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/{resourceProviderNamespace}/{resourceType}/{resourceName}
*
*/
private String id;
/**
* @return Represents the imported file.
*
*/
private FileMetadataResponse importFile;
/**
* @return The time the file import record is soft deleted from the database and history.
*
*/
private String importValidUntilTimeUTC;
/**
* @return The number of records that have been successfully ingested.
*
*/
private Integer ingestedRecordCount;
/**
* @return Describes how to ingest the records in the file.
*
*/
private String ingestionMode;
/**
* @return The name of the resource
*
*/
private String name;
/**
* @return The source for the data in the file.
*
*/
private String source;
/**
* @return The state of the file import.
*
*/
private String state;
/**
* @return Azure Resource Manager metadata containing createdBy and modifiedBy information.
*
*/
private SystemDataResponse systemData;
/**
* @return The number of records in the file.
*
*/
private Integer totalRecordCount;
/**
* @return The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
private String type;
/**
* @return The number of records that have passed validation.
*
*/
private Integer validRecordCount;
private GetFileImportResult() {}
/**
* @return The content type of this file.
*
*/
public String contentType() {
return this.contentType;
}
/**
* @return The time the file was imported.
*
*/
public String createdTimeUTC() {
return this.createdTimeUTC;
}
/**
* @return Represents the error file (if the import was ingested with errors or failed the validation).
*
*/
public FileMetadataResponse errorFile() {
return this.errorFile;
}
/**
* @return An ordered list of some of the errors that were encountered during validation.
*
*/
public List errorsPreview() {
return this.errorsPreview;
}
/**
* @return The time the files associated with this import are deleted from the storage account.
*
*/
public String filesValidUntilTimeUTC() {
return this.filesValidUntilTimeUTC;
}
/**
* @return Fully qualified resource ID for the resource. Ex - /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/{resourceProviderNamespace}/{resourceType}/{resourceName}
*
*/
public String id() {
return this.id;
}
/**
* @return Represents the imported file.
*
*/
public FileMetadataResponse importFile() {
return this.importFile;
}
/**
* @return The time the file import record is soft deleted from the database and history.
*
*/
public String importValidUntilTimeUTC() {
return this.importValidUntilTimeUTC;
}
/**
* @return The number of records that have been successfully ingested.
*
*/
public Integer ingestedRecordCount() {
return this.ingestedRecordCount;
}
/**
* @return Describes how to ingest the records in the file.
*
*/
public String ingestionMode() {
return this.ingestionMode;
}
/**
* @return The name of the resource
*
*/
public String name() {
return this.name;
}
/**
* @return The source for the data in the file.
*
*/
public String source() {
return this.source;
}
/**
* @return The state of the file import.
*
*/
public String state() {
return this.state;
}
/**
* @return Azure Resource Manager metadata containing createdBy and modifiedBy information.
*
*/
public SystemDataResponse systemData() {
return this.systemData;
}
/**
* @return The number of records in the file.
*
*/
public Integer totalRecordCount() {
return this.totalRecordCount;
}
/**
* @return The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
public String type() {
return this.type;
}
/**
* @return The number of records that have passed validation.
*
*/
public Integer validRecordCount() {
return this.validRecordCount;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetFileImportResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String contentType;
private String createdTimeUTC;
private FileMetadataResponse errorFile;
private List errorsPreview;
private String filesValidUntilTimeUTC;
private String id;
private FileMetadataResponse importFile;
private String importValidUntilTimeUTC;
private Integer ingestedRecordCount;
private String ingestionMode;
private String name;
private String source;
private String state;
private SystemDataResponse systemData;
private Integer totalRecordCount;
private String type;
private Integer validRecordCount;
public Builder() {}
public Builder(GetFileImportResult defaults) {
Objects.requireNonNull(defaults);
this.contentType = defaults.contentType;
this.createdTimeUTC = defaults.createdTimeUTC;
this.errorFile = defaults.errorFile;
this.errorsPreview = defaults.errorsPreview;
this.filesValidUntilTimeUTC = defaults.filesValidUntilTimeUTC;
this.id = defaults.id;
this.importFile = defaults.importFile;
this.importValidUntilTimeUTC = defaults.importValidUntilTimeUTC;
this.ingestedRecordCount = defaults.ingestedRecordCount;
this.ingestionMode = defaults.ingestionMode;
this.name = defaults.name;
this.source = defaults.source;
this.state = defaults.state;
this.systemData = defaults.systemData;
this.totalRecordCount = defaults.totalRecordCount;
this.type = defaults.type;
this.validRecordCount = defaults.validRecordCount;
}
@CustomType.Setter
public Builder contentType(String contentType) {
if (contentType == null) {
throw new MissingRequiredPropertyException("GetFileImportResult", "contentType");
}
this.contentType = contentType;
return this;
}
@CustomType.Setter
public Builder createdTimeUTC(String createdTimeUTC) {
if (createdTimeUTC == null) {
throw new MissingRequiredPropertyException("GetFileImportResult", "createdTimeUTC");
}
this.createdTimeUTC = createdTimeUTC;
return this;
}
@CustomType.Setter
public Builder errorFile(FileMetadataResponse errorFile) {
if (errorFile == null) {
throw new MissingRequiredPropertyException("GetFileImportResult", "errorFile");
}
this.errorFile = errorFile;
return this;
}
@CustomType.Setter
public Builder errorsPreview(List errorsPreview) {
if (errorsPreview == null) {
throw new MissingRequiredPropertyException("GetFileImportResult", "errorsPreview");
}
this.errorsPreview = errorsPreview;
return this;
}
public Builder errorsPreview(ValidationErrorResponse... errorsPreview) {
return errorsPreview(List.of(errorsPreview));
}
@CustomType.Setter
public Builder filesValidUntilTimeUTC(String filesValidUntilTimeUTC) {
if (filesValidUntilTimeUTC == null) {
throw new MissingRequiredPropertyException("GetFileImportResult", "filesValidUntilTimeUTC");
}
this.filesValidUntilTimeUTC = filesValidUntilTimeUTC;
return this;
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetFileImportResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder importFile(FileMetadataResponse importFile) {
if (importFile == null) {
throw new MissingRequiredPropertyException("GetFileImportResult", "importFile");
}
this.importFile = importFile;
return this;
}
@CustomType.Setter
public Builder importValidUntilTimeUTC(String importValidUntilTimeUTC) {
if (importValidUntilTimeUTC == null) {
throw new MissingRequiredPropertyException("GetFileImportResult", "importValidUntilTimeUTC");
}
this.importValidUntilTimeUTC = importValidUntilTimeUTC;
return this;
}
@CustomType.Setter
public Builder ingestedRecordCount(Integer ingestedRecordCount) {
if (ingestedRecordCount == null) {
throw new MissingRequiredPropertyException("GetFileImportResult", "ingestedRecordCount");
}
this.ingestedRecordCount = ingestedRecordCount;
return this;
}
@CustomType.Setter
public Builder ingestionMode(String ingestionMode) {
if (ingestionMode == null) {
throw new MissingRequiredPropertyException("GetFileImportResult", "ingestionMode");
}
this.ingestionMode = ingestionMode;
return this;
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("GetFileImportResult", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder source(String source) {
if (source == null) {
throw new MissingRequiredPropertyException("GetFileImportResult", "source");
}
this.source = source;
return this;
}
@CustomType.Setter
public Builder state(String state) {
if (state == null) {
throw new MissingRequiredPropertyException("GetFileImportResult", "state");
}
this.state = state;
return this;
}
@CustomType.Setter
public Builder systemData(SystemDataResponse systemData) {
if (systemData == null) {
throw new MissingRequiredPropertyException("GetFileImportResult", "systemData");
}
this.systemData = systemData;
return this;
}
@CustomType.Setter
public Builder totalRecordCount(Integer totalRecordCount) {
if (totalRecordCount == null) {
throw new MissingRequiredPropertyException("GetFileImportResult", "totalRecordCount");
}
this.totalRecordCount = totalRecordCount;
return this;
}
@CustomType.Setter
public Builder type(String type) {
if (type == null) {
throw new MissingRequiredPropertyException("GetFileImportResult", "type");
}
this.type = type;
return this;
}
@CustomType.Setter
public Builder validRecordCount(Integer validRecordCount) {
if (validRecordCount == null) {
throw new MissingRequiredPropertyException("GetFileImportResult", "validRecordCount");
}
this.validRecordCount = validRecordCount;
return this;
}
public GetFileImportResult build() {
final var _resultValue = new GetFileImportResult();
_resultValue.contentType = contentType;
_resultValue.createdTimeUTC = createdTimeUTC;
_resultValue.errorFile = errorFile;
_resultValue.errorsPreview = errorsPreview;
_resultValue.filesValidUntilTimeUTC = filesValidUntilTimeUTC;
_resultValue.id = id;
_resultValue.importFile = importFile;
_resultValue.importValidUntilTimeUTC = importValidUntilTimeUTC;
_resultValue.ingestedRecordCount = ingestedRecordCount;
_resultValue.ingestionMode = ingestionMode;
_resultValue.name = name;
_resultValue.source = source;
_resultValue.state = state;
_resultValue.systemData = systemData;
_resultValue.totalRecordCount = totalRecordCount;
_resultValue.type = type;
_resultValue.validRecordCount = validRecordCount;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy