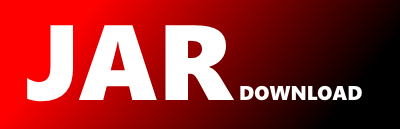
com.pulumi.azurenative.securityinsights.outputs.GetIncidentResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.securityinsights.outputs;
import com.pulumi.azurenative.securityinsights.outputs.IncidentAdditionalDataResponse;
import com.pulumi.azurenative.securityinsights.outputs.IncidentLabelResponse;
import com.pulumi.azurenative.securityinsights.outputs.IncidentOwnerInfoResponse;
import com.pulumi.azurenative.securityinsights.outputs.SystemDataResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class GetIncidentResult {
/**
* @return Additional data on the incident
*
*/
private IncidentAdditionalDataResponse additionalData;
/**
* @return The reason the incident was closed
*
*/
private @Nullable String classification;
/**
* @return Describes the reason the incident was closed
*
*/
private @Nullable String classificationComment;
/**
* @return The classification reason the incident was closed with
*
*/
private @Nullable String classificationReason;
/**
* @return The time the incident was created
*
*/
private String createdTimeUtc;
/**
* @return The description of the incident
*
*/
private @Nullable String description;
/**
* @return Etag of the azure resource
*
*/
private @Nullable String etag;
/**
* @return The time of the first activity in the incident
*
*/
private @Nullable String firstActivityTimeUtc;
/**
* @return Fully qualified resource ID for the resource. E.g. "/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/{resourceProviderNamespace}/{resourceType}/{resourceName}"
*
*/
private String id;
/**
* @return A sequential number
*
*/
private Integer incidentNumber;
/**
* @return The deep-link url to the incident in Azure portal
*
*/
private String incidentUrl;
/**
* @return List of labels relevant to this incident
*
*/
private @Nullable List labels;
/**
* @return The time of the last activity in the incident
*
*/
private @Nullable String lastActivityTimeUtc;
/**
* @return The last time the incident was updated
*
*/
private String lastModifiedTimeUtc;
/**
* @return The name of the resource
*
*/
private String name;
/**
* @return Describes a user that the incident is assigned to
*
*/
private @Nullable IncidentOwnerInfoResponse owner;
/**
* @return The incident ID assigned by the incident provider
*
*/
private String providerIncidentId;
/**
* @return The name of the source provider that generated the incident
*
*/
private String providerName;
/**
* @return List of resource ids of Analytic rules related to the incident
*
*/
private List relatedAnalyticRuleIds;
/**
* @return The severity of the incident
*
*/
private String severity;
/**
* @return The status of the incident
*
*/
private String status;
/**
* @return Azure Resource Manager metadata containing createdBy and modifiedBy information.
*
*/
private SystemDataResponse systemData;
/**
* @return The title of the incident
*
*/
private String title;
/**
* @return The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
private String type;
private GetIncidentResult() {}
/**
* @return Additional data on the incident
*
*/
public IncidentAdditionalDataResponse additionalData() {
return this.additionalData;
}
/**
* @return The reason the incident was closed
*
*/
public Optional classification() {
return Optional.ofNullable(this.classification);
}
/**
* @return Describes the reason the incident was closed
*
*/
public Optional classificationComment() {
return Optional.ofNullable(this.classificationComment);
}
/**
* @return The classification reason the incident was closed with
*
*/
public Optional classificationReason() {
return Optional.ofNullable(this.classificationReason);
}
/**
* @return The time the incident was created
*
*/
public String createdTimeUtc() {
return this.createdTimeUtc;
}
/**
* @return The description of the incident
*
*/
public Optional description() {
return Optional.ofNullable(this.description);
}
/**
* @return Etag of the azure resource
*
*/
public Optional etag() {
return Optional.ofNullable(this.etag);
}
/**
* @return The time of the first activity in the incident
*
*/
public Optional firstActivityTimeUtc() {
return Optional.ofNullable(this.firstActivityTimeUtc);
}
/**
* @return Fully qualified resource ID for the resource. E.g. "/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/{resourceProviderNamespace}/{resourceType}/{resourceName}"
*
*/
public String id() {
return this.id;
}
/**
* @return A sequential number
*
*/
public Integer incidentNumber() {
return this.incidentNumber;
}
/**
* @return The deep-link url to the incident in Azure portal
*
*/
public String incidentUrl() {
return this.incidentUrl;
}
/**
* @return List of labels relevant to this incident
*
*/
public List labels() {
return this.labels == null ? List.of() : this.labels;
}
/**
* @return The time of the last activity in the incident
*
*/
public Optional lastActivityTimeUtc() {
return Optional.ofNullable(this.lastActivityTimeUtc);
}
/**
* @return The last time the incident was updated
*
*/
public String lastModifiedTimeUtc() {
return this.lastModifiedTimeUtc;
}
/**
* @return The name of the resource
*
*/
public String name() {
return this.name;
}
/**
* @return Describes a user that the incident is assigned to
*
*/
public Optional owner() {
return Optional.ofNullable(this.owner);
}
/**
* @return The incident ID assigned by the incident provider
*
*/
public String providerIncidentId() {
return this.providerIncidentId;
}
/**
* @return The name of the source provider that generated the incident
*
*/
public String providerName() {
return this.providerName;
}
/**
* @return List of resource ids of Analytic rules related to the incident
*
*/
public List relatedAnalyticRuleIds() {
return this.relatedAnalyticRuleIds;
}
/**
* @return The severity of the incident
*
*/
public String severity() {
return this.severity;
}
/**
* @return The status of the incident
*
*/
public String status() {
return this.status;
}
/**
* @return Azure Resource Manager metadata containing createdBy and modifiedBy information.
*
*/
public SystemDataResponse systemData() {
return this.systemData;
}
/**
* @return The title of the incident
*
*/
public String title() {
return this.title;
}
/**
* @return The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
public String type() {
return this.type;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetIncidentResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private IncidentAdditionalDataResponse additionalData;
private @Nullable String classification;
private @Nullable String classificationComment;
private @Nullable String classificationReason;
private String createdTimeUtc;
private @Nullable String description;
private @Nullable String etag;
private @Nullable String firstActivityTimeUtc;
private String id;
private Integer incidentNumber;
private String incidentUrl;
private @Nullable List labels;
private @Nullable String lastActivityTimeUtc;
private String lastModifiedTimeUtc;
private String name;
private @Nullable IncidentOwnerInfoResponse owner;
private String providerIncidentId;
private String providerName;
private List relatedAnalyticRuleIds;
private String severity;
private String status;
private SystemDataResponse systemData;
private String title;
private String type;
public Builder() {}
public Builder(GetIncidentResult defaults) {
Objects.requireNonNull(defaults);
this.additionalData = defaults.additionalData;
this.classification = defaults.classification;
this.classificationComment = defaults.classificationComment;
this.classificationReason = defaults.classificationReason;
this.createdTimeUtc = defaults.createdTimeUtc;
this.description = defaults.description;
this.etag = defaults.etag;
this.firstActivityTimeUtc = defaults.firstActivityTimeUtc;
this.id = defaults.id;
this.incidentNumber = defaults.incidentNumber;
this.incidentUrl = defaults.incidentUrl;
this.labels = defaults.labels;
this.lastActivityTimeUtc = defaults.lastActivityTimeUtc;
this.lastModifiedTimeUtc = defaults.lastModifiedTimeUtc;
this.name = defaults.name;
this.owner = defaults.owner;
this.providerIncidentId = defaults.providerIncidentId;
this.providerName = defaults.providerName;
this.relatedAnalyticRuleIds = defaults.relatedAnalyticRuleIds;
this.severity = defaults.severity;
this.status = defaults.status;
this.systemData = defaults.systemData;
this.title = defaults.title;
this.type = defaults.type;
}
@CustomType.Setter
public Builder additionalData(IncidentAdditionalDataResponse additionalData) {
if (additionalData == null) {
throw new MissingRequiredPropertyException("GetIncidentResult", "additionalData");
}
this.additionalData = additionalData;
return this;
}
@CustomType.Setter
public Builder classification(@Nullable String classification) {
this.classification = classification;
return this;
}
@CustomType.Setter
public Builder classificationComment(@Nullable String classificationComment) {
this.classificationComment = classificationComment;
return this;
}
@CustomType.Setter
public Builder classificationReason(@Nullable String classificationReason) {
this.classificationReason = classificationReason;
return this;
}
@CustomType.Setter
public Builder createdTimeUtc(String createdTimeUtc) {
if (createdTimeUtc == null) {
throw new MissingRequiredPropertyException("GetIncidentResult", "createdTimeUtc");
}
this.createdTimeUtc = createdTimeUtc;
return this;
}
@CustomType.Setter
public Builder description(@Nullable String description) {
this.description = description;
return this;
}
@CustomType.Setter
public Builder etag(@Nullable String etag) {
this.etag = etag;
return this;
}
@CustomType.Setter
public Builder firstActivityTimeUtc(@Nullable String firstActivityTimeUtc) {
this.firstActivityTimeUtc = firstActivityTimeUtc;
return this;
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetIncidentResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder incidentNumber(Integer incidentNumber) {
if (incidentNumber == null) {
throw new MissingRequiredPropertyException("GetIncidentResult", "incidentNumber");
}
this.incidentNumber = incidentNumber;
return this;
}
@CustomType.Setter
public Builder incidentUrl(String incidentUrl) {
if (incidentUrl == null) {
throw new MissingRequiredPropertyException("GetIncidentResult", "incidentUrl");
}
this.incidentUrl = incidentUrl;
return this;
}
@CustomType.Setter
public Builder labels(@Nullable List labels) {
this.labels = labels;
return this;
}
public Builder labels(IncidentLabelResponse... labels) {
return labels(List.of(labels));
}
@CustomType.Setter
public Builder lastActivityTimeUtc(@Nullable String lastActivityTimeUtc) {
this.lastActivityTimeUtc = lastActivityTimeUtc;
return this;
}
@CustomType.Setter
public Builder lastModifiedTimeUtc(String lastModifiedTimeUtc) {
if (lastModifiedTimeUtc == null) {
throw new MissingRequiredPropertyException("GetIncidentResult", "lastModifiedTimeUtc");
}
this.lastModifiedTimeUtc = lastModifiedTimeUtc;
return this;
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("GetIncidentResult", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder owner(@Nullable IncidentOwnerInfoResponse owner) {
this.owner = owner;
return this;
}
@CustomType.Setter
public Builder providerIncidentId(String providerIncidentId) {
if (providerIncidentId == null) {
throw new MissingRequiredPropertyException("GetIncidentResult", "providerIncidentId");
}
this.providerIncidentId = providerIncidentId;
return this;
}
@CustomType.Setter
public Builder providerName(String providerName) {
if (providerName == null) {
throw new MissingRequiredPropertyException("GetIncidentResult", "providerName");
}
this.providerName = providerName;
return this;
}
@CustomType.Setter
public Builder relatedAnalyticRuleIds(List relatedAnalyticRuleIds) {
if (relatedAnalyticRuleIds == null) {
throw new MissingRequiredPropertyException("GetIncidentResult", "relatedAnalyticRuleIds");
}
this.relatedAnalyticRuleIds = relatedAnalyticRuleIds;
return this;
}
public Builder relatedAnalyticRuleIds(String... relatedAnalyticRuleIds) {
return relatedAnalyticRuleIds(List.of(relatedAnalyticRuleIds));
}
@CustomType.Setter
public Builder severity(String severity) {
if (severity == null) {
throw new MissingRequiredPropertyException("GetIncidentResult", "severity");
}
this.severity = severity;
return this;
}
@CustomType.Setter
public Builder status(String status) {
if (status == null) {
throw new MissingRequiredPropertyException("GetIncidentResult", "status");
}
this.status = status;
return this;
}
@CustomType.Setter
public Builder systemData(SystemDataResponse systemData) {
if (systemData == null) {
throw new MissingRequiredPropertyException("GetIncidentResult", "systemData");
}
this.systemData = systemData;
return this;
}
@CustomType.Setter
public Builder title(String title) {
if (title == null) {
throw new MissingRequiredPropertyException("GetIncidentResult", "title");
}
this.title = title;
return this;
}
@CustomType.Setter
public Builder type(String type) {
if (type == null) {
throw new MissingRequiredPropertyException("GetIncidentResult", "type");
}
this.type = type;
return this;
}
public GetIncidentResult build() {
final var _resultValue = new GetIncidentResult();
_resultValue.additionalData = additionalData;
_resultValue.classification = classification;
_resultValue.classificationComment = classificationComment;
_resultValue.classificationReason = classificationReason;
_resultValue.createdTimeUtc = createdTimeUtc;
_resultValue.description = description;
_resultValue.etag = etag;
_resultValue.firstActivityTimeUtc = firstActivityTimeUtc;
_resultValue.id = id;
_resultValue.incidentNumber = incidentNumber;
_resultValue.incidentUrl = incidentUrl;
_resultValue.labels = labels;
_resultValue.lastActivityTimeUtc = lastActivityTimeUtc;
_resultValue.lastModifiedTimeUtc = lastModifiedTimeUtc;
_resultValue.name = name;
_resultValue.owner = owner;
_resultValue.providerIncidentId = providerIncidentId;
_resultValue.providerName = providerName;
_resultValue.relatedAnalyticRuleIds = relatedAnalyticRuleIds;
_resultValue.severity = severity;
_resultValue.status = status;
_resultValue.systemData = systemData;
_resultValue.title = title;
_resultValue.type = type;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy