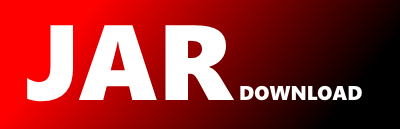
com.pulumi.azurenative.servicebus.Queue Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.servicebus;
import com.pulumi.azurenative.Utilities;
import com.pulumi.azurenative.servicebus.QueueArgs;
import com.pulumi.azurenative.servicebus.outputs.MessageCountDetailsResponse;
import com.pulumi.azurenative.servicebus.outputs.SystemDataResponse;
import com.pulumi.core.Alias;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.Boolean;
import java.lang.Double;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Description of queue Resource.
* Azure REST API version: 2022-01-01-preview. Prior API version in Azure Native 1.x: 2017-04-01.
*
* Other available API versions: 2015-08-01, 2022-10-01-preview, 2023-01-01-preview, 2024-01-01.
*
* ## Example Usage
* ### QueueCreate
*
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.servicebus.Queue;
* import com.pulumi.azurenative.servicebus.QueueArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var queue = new Queue("queue", QueueArgs.builder()
* .enablePartitioning(true)
* .namespaceName("sdk-Namespace-3174")
* .queueName("sdk-Queues-5647")
* .resourceGroupName("ArunMonocle")
* .build());
*
* }
* }
*
* }
*
*
* ## Import
*
* An existing resource can be imported using its type token, name, and identifier, e.g.
*
* ```sh
* $ pulumi import azure-native:servicebus:Queue sdk-Queues-5647 /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.ServiceBus/namespaces/{namespaceName}/queues/{queueName}
* ```
*
*/
@ResourceType(type="azure-native:servicebus:Queue")
public class Queue extends com.pulumi.resources.CustomResource {
/**
* Last time a message was sent, or the last time there was a receive request to this queue.
*
*/
@Export(name="accessedAt", refs={String.class}, tree="[0]")
private Output accessedAt;
/**
* @return Last time a message was sent, or the last time there was a receive request to this queue.
*
*/
public Output accessedAt() {
return this.accessedAt;
}
/**
* ISO 8061 timeSpan idle interval after which the queue is automatically deleted. The minimum duration is 5 minutes.
*
*/
@Export(name="autoDeleteOnIdle", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> autoDeleteOnIdle;
/**
* @return ISO 8061 timeSpan idle interval after which the queue is automatically deleted. The minimum duration is 5 minutes.
*
*/
public Output> autoDeleteOnIdle() {
return Codegen.optional(this.autoDeleteOnIdle);
}
/**
* Message Count Details.
*
*/
@Export(name="countDetails", refs={MessageCountDetailsResponse.class}, tree="[0]")
private Output countDetails;
/**
* @return Message Count Details.
*
*/
public Output countDetails() {
return this.countDetails;
}
/**
* The exact time the message was created.
*
*/
@Export(name="createdAt", refs={String.class}, tree="[0]")
private Output createdAt;
/**
* @return The exact time the message was created.
*
*/
public Output createdAt() {
return this.createdAt;
}
/**
* A value that indicates whether this queue has dead letter support when a message expires.
*
*/
@Export(name="deadLetteringOnMessageExpiration", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> deadLetteringOnMessageExpiration;
/**
* @return A value that indicates whether this queue has dead letter support when a message expires.
*
*/
public Output> deadLetteringOnMessageExpiration() {
return Codegen.optional(this.deadLetteringOnMessageExpiration);
}
/**
* ISO 8601 default message timespan to live value. This is the duration after which the message expires, starting from when the message is sent to Service Bus. This is the default value used when TimeToLive is not set on a message itself.
*
*/
@Export(name="defaultMessageTimeToLive", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> defaultMessageTimeToLive;
/**
* @return ISO 8601 default message timespan to live value. This is the duration after which the message expires, starting from when the message is sent to Service Bus. This is the default value used when TimeToLive is not set on a message itself.
*
*/
public Output> defaultMessageTimeToLive() {
return Codegen.optional(this.defaultMessageTimeToLive);
}
/**
* ISO 8601 timeSpan structure that defines the duration of the duplicate detection history. The default value is 10 minutes.
*
*/
@Export(name="duplicateDetectionHistoryTimeWindow", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> duplicateDetectionHistoryTimeWindow;
/**
* @return ISO 8601 timeSpan structure that defines the duration of the duplicate detection history. The default value is 10 minutes.
*
*/
public Output> duplicateDetectionHistoryTimeWindow() {
return Codegen.optional(this.duplicateDetectionHistoryTimeWindow);
}
/**
* Value that indicates whether server-side batched operations are enabled.
*
*/
@Export(name="enableBatchedOperations", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> enableBatchedOperations;
/**
* @return Value that indicates whether server-side batched operations are enabled.
*
*/
public Output> enableBatchedOperations() {
return Codegen.optional(this.enableBatchedOperations);
}
/**
* A value that indicates whether Express Entities are enabled. An express queue holds a message in memory temporarily before writing it to persistent storage.
*
*/
@Export(name="enableExpress", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> enableExpress;
/**
* @return A value that indicates whether Express Entities are enabled. An express queue holds a message in memory temporarily before writing it to persistent storage.
*
*/
public Output> enableExpress() {
return Codegen.optional(this.enableExpress);
}
/**
* A value that indicates whether the queue is to be partitioned across multiple message brokers.
*
*/
@Export(name="enablePartitioning", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> enablePartitioning;
/**
* @return A value that indicates whether the queue is to be partitioned across multiple message brokers.
*
*/
public Output> enablePartitioning() {
return Codegen.optional(this.enablePartitioning);
}
/**
* Queue/Topic name to forward the Dead Letter message
*
*/
@Export(name="forwardDeadLetteredMessagesTo", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> forwardDeadLetteredMessagesTo;
/**
* @return Queue/Topic name to forward the Dead Letter message
*
*/
public Output> forwardDeadLetteredMessagesTo() {
return Codegen.optional(this.forwardDeadLetteredMessagesTo);
}
/**
* Queue/Topic name to forward the messages
*
*/
@Export(name="forwardTo", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> forwardTo;
/**
* @return Queue/Topic name to forward the messages
*
*/
public Output> forwardTo() {
return Codegen.optional(this.forwardTo);
}
/**
* The geo-location where the resource lives
*
*/
@Export(name="location", refs={String.class}, tree="[0]")
private Output location;
/**
* @return The geo-location where the resource lives
*
*/
public Output location() {
return this.location;
}
/**
* ISO 8601 timespan duration of a peek-lock; that is, the amount of time that the message is locked for other receivers. The maximum value for LockDuration is 5 minutes; the default value is 1 minute.
*
*/
@Export(name="lockDuration", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> lockDuration;
/**
* @return ISO 8601 timespan duration of a peek-lock; that is, the amount of time that the message is locked for other receivers. The maximum value for LockDuration is 5 minutes; the default value is 1 minute.
*
*/
public Output> lockDuration() {
return Codegen.optional(this.lockDuration);
}
/**
* The maximum delivery count. A message is automatically deadlettered after this number of deliveries. default value is 10.
*
*/
@Export(name="maxDeliveryCount", refs={Integer.class}, tree="[0]")
private Output* @Nullable */ Integer> maxDeliveryCount;
/**
* @return The maximum delivery count. A message is automatically deadlettered after this number of deliveries. default value is 10.
*
*/
public Output> maxDeliveryCount() {
return Codegen.optional(this.maxDeliveryCount);
}
/**
* Maximum size (in KB) of the message payload that can be accepted by the queue. This property is only used in Premium today and default is 1024.
*
*/
@Export(name="maxMessageSizeInKilobytes", refs={Double.class}, tree="[0]")
private Output* @Nullable */ Double> maxMessageSizeInKilobytes;
/**
* @return Maximum size (in KB) of the message payload that can be accepted by the queue. This property is only used in Premium today and default is 1024.
*
*/
public Output> maxMessageSizeInKilobytes() {
return Codegen.optional(this.maxMessageSizeInKilobytes);
}
/**
* The maximum size of the queue in megabytes, which is the size of memory allocated for the queue. Default is 1024.
*
*/
@Export(name="maxSizeInMegabytes", refs={Integer.class}, tree="[0]")
private Output* @Nullable */ Integer> maxSizeInMegabytes;
/**
* @return The maximum size of the queue in megabytes, which is the size of memory allocated for the queue. Default is 1024.
*
*/
public Output> maxSizeInMegabytes() {
return Codegen.optional(this.maxSizeInMegabytes);
}
/**
* The number of messages in the queue.
*
*/
@Export(name="messageCount", refs={Double.class}, tree="[0]")
private Output messageCount;
/**
* @return The number of messages in the queue.
*
*/
public Output messageCount() {
return this.messageCount;
}
/**
* The name of the resource
*
*/
@Export(name="name", refs={String.class}, tree="[0]")
private Output name;
/**
* @return The name of the resource
*
*/
public Output name() {
return this.name;
}
/**
* A value indicating if this queue requires duplicate detection.
*
*/
@Export(name="requiresDuplicateDetection", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> requiresDuplicateDetection;
/**
* @return A value indicating if this queue requires duplicate detection.
*
*/
public Output> requiresDuplicateDetection() {
return Codegen.optional(this.requiresDuplicateDetection);
}
/**
* A value that indicates whether the queue supports the concept of sessions.
*
*/
@Export(name="requiresSession", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> requiresSession;
/**
* @return A value that indicates whether the queue supports the concept of sessions.
*
*/
public Output> requiresSession() {
return Codegen.optional(this.requiresSession);
}
/**
* The size of the queue, in bytes.
*
*/
@Export(name="sizeInBytes", refs={Double.class}, tree="[0]")
private Output sizeInBytes;
/**
* @return The size of the queue, in bytes.
*
*/
public Output sizeInBytes() {
return this.sizeInBytes;
}
/**
* Enumerates the possible values for the status of a messaging entity.
*
*/
@Export(name="status", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> status;
/**
* @return Enumerates the possible values for the status of a messaging entity.
*
*/
public Output> status() {
return Codegen.optional(this.status);
}
/**
* The system meta data relating to this resource.
*
*/
@Export(name="systemData", refs={SystemDataResponse.class}, tree="[0]")
private Output systemData;
/**
* @return The system meta data relating to this resource.
*
*/
public Output systemData() {
return this.systemData;
}
/**
* The type of the resource. E.g. "Microsoft.EventHub/Namespaces" or "Microsoft.EventHub/Namespaces/EventHubs"
*
*/
@Export(name="type", refs={String.class}, tree="[0]")
private Output type;
/**
* @return The type of the resource. E.g. "Microsoft.EventHub/Namespaces" or "Microsoft.EventHub/Namespaces/EventHubs"
*
*/
public Output type() {
return this.type;
}
/**
* The exact time the message was updated.
*
*/
@Export(name="updatedAt", refs={String.class}, tree="[0]")
private Output updatedAt;
/**
* @return The exact time the message was updated.
*
*/
public Output updatedAt() {
return this.updatedAt;
}
/**
*
* @param name The _unique_ name of the resulting resource.
*/
public Queue(java.lang.String name) {
this(name, QueueArgs.Empty);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
*/
public Queue(java.lang.String name, QueueArgs args) {
this(name, args, null);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
* @param options A bag of options that control this resource's behavior.
*/
public Queue(java.lang.String name, QueueArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("azure-native:servicebus:Queue", name, makeArgs(args, options), makeResourceOptions(options, Codegen.empty()), false);
}
private Queue(java.lang.String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("azure-native:servicebus:Queue", name, null, makeResourceOptions(options, id), false);
}
private static QueueArgs makeArgs(QueueArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
if (options != null && options.getUrn().isPresent()) {
return null;
}
return args == null ? QueueArgs.Empty : args;
}
private static com.pulumi.resources.CustomResourceOptions makeResourceOptions(@Nullable com.pulumi.resources.CustomResourceOptions options, @Nullable Output id) {
var defaultOptions = com.pulumi.resources.CustomResourceOptions.builder()
.version(Utilities.getVersion())
.aliases(List.of(
Output.of(Alias.builder().type("azure-native:servicebus/v20140901:Queue").build()),
Output.of(Alias.builder().type("azure-native:servicebus/v20150801:Queue").build()),
Output.of(Alias.builder().type("azure-native:servicebus/v20170401:Queue").build()),
Output.of(Alias.builder().type("azure-native:servicebus/v20180101preview:Queue").build()),
Output.of(Alias.builder().type("azure-native:servicebus/v20210101preview:Queue").build()),
Output.of(Alias.builder().type("azure-native:servicebus/v20210601preview:Queue").build()),
Output.of(Alias.builder().type("azure-native:servicebus/v20211101:Queue").build()),
Output.of(Alias.builder().type("azure-native:servicebus/v20220101preview:Queue").build()),
Output.of(Alias.builder().type("azure-native:servicebus/v20221001preview:Queue").build()),
Output.of(Alias.builder().type("azure-native:servicebus/v20230101preview:Queue").build()),
Output.of(Alias.builder().type("azure-native:servicebus/v20240101:Queue").build())
))
.build();
return com.pulumi.resources.CustomResourceOptions.merge(defaultOptions, options, id);
}
/**
* Get an existing Host resource's state with the given name, ID, and optional extra
* properties used to qualify the lookup.
*
* @param name The _unique_ name of the resulting resource.
* @param id The _unique_ provider ID of the resource to lookup.
* @param options Optional settings to control the behavior of the CustomResource.
*/
public static Queue get(java.lang.String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
return new Queue(name, id, options);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy