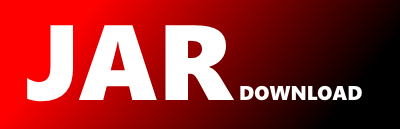
com.pulumi.azurenative.servicebus.TopicArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.servicebus;
import com.pulumi.azurenative.servicebus.enums.EntityStatus;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.Double;
import java.lang.Integer;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class TopicArgs extends com.pulumi.resources.ResourceArgs {
public static final TopicArgs Empty = new TopicArgs();
/**
* ISO 8601 timespan idle interval after which the topic is automatically deleted. The minimum duration is 5 minutes.
*
*/
@Import(name="autoDeleteOnIdle")
private @Nullable Output autoDeleteOnIdle;
/**
* @return ISO 8601 timespan idle interval after which the topic is automatically deleted. The minimum duration is 5 minutes.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy