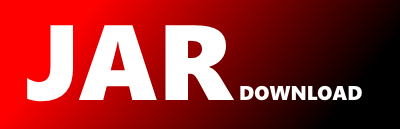
com.pulumi.azurenative.servicebus.outputs.GetQueueResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.servicebus.outputs;
import com.pulumi.azurenative.servicebus.outputs.MessageCountDetailsResponse;
import com.pulumi.azurenative.servicebus.outputs.SystemDataResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.Double;
import java.lang.Integer;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class GetQueueResult {
/**
* @return Last time a message was sent, or the last time there was a receive request to this queue.
*
*/
private String accessedAt;
/**
* @return ISO 8061 timeSpan idle interval after which the queue is automatically deleted. The minimum duration is 5 minutes.
*
*/
private @Nullable String autoDeleteOnIdle;
/**
* @return Message Count Details.
*
*/
private MessageCountDetailsResponse countDetails;
/**
* @return The exact time the message was created.
*
*/
private String createdAt;
/**
* @return A value that indicates whether this queue has dead letter support when a message expires.
*
*/
private @Nullable Boolean deadLetteringOnMessageExpiration;
/**
* @return ISO 8601 default message timespan to live value. This is the duration after which the message expires, starting from when the message is sent to Service Bus. This is the default value used when TimeToLive is not set on a message itself.
*
*/
private @Nullable String defaultMessageTimeToLive;
/**
* @return ISO 8601 timeSpan structure that defines the duration of the duplicate detection history. The default value is 10 minutes.
*
*/
private @Nullable String duplicateDetectionHistoryTimeWindow;
/**
* @return Value that indicates whether server-side batched operations are enabled.
*
*/
private @Nullable Boolean enableBatchedOperations;
/**
* @return A value that indicates whether Express Entities are enabled. An express queue holds a message in memory temporarily before writing it to persistent storage.
*
*/
private @Nullable Boolean enableExpress;
/**
* @return A value that indicates whether the queue is to be partitioned across multiple message brokers.
*
*/
private @Nullable Boolean enablePartitioning;
/**
* @return Queue/Topic name to forward the Dead Letter message
*
*/
private @Nullable String forwardDeadLetteredMessagesTo;
/**
* @return Queue/Topic name to forward the messages
*
*/
private @Nullable String forwardTo;
/**
* @return Fully qualified resource ID for the resource. Ex - /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/{resourceProviderNamespace}/{resourceType}/{resourceName}
*
*/
private String id;
/**
* @return The geo-location where the resource lives
*
*/
private String location;
/**
* @return ISO 8601 timespan duration of a peek-lock; that is, the amount of time that the message is locked for other receivers. The maximum value for LockDuration is 5 minutes; the default value is 1 minute.
*
*/
private @Nullable String lockDuration;
/**
* @return The maximum delivery count. A message is automatically deadlettered after this number of deliveries. default value is 10.
*
*/
private @Nullable Integer maxDeliveryCount;
/**
* @return Maximum size (in KB) of the message payload that can be accepted by the queue. This property is only used in Premium today and default is 1024.
*
*/
private @Nullable Double maxMessageSizeInKilobytes;
/**
* @return The maximum size of the queue in megabytes, which is the size of memory allocated for the queue. Default is 1024.
*
*/
private @Nullable Integer maxSizeInMegabytes;
/**
* @return The number of messages in the queue.
*
*/
private Double messageCount;
/**
* @return The name of the resource
*
*/
private String name;
/**
* @return A value indicating if this queue requires duplicate detection.
*
*/
private @Nullable Boolean requiresDuplicateDetection;
/**
* @return A value that indicates whether the queue supports the concept of sessions.
*
*/
private @Nullable Boolean requiresSession;
/**
* @return The size of the queue, in bytes.
*
*/
private Double sizeInBytes;
/**
* @return Enumerates the possible values for the status of a messaging entity.
*
*/
private @Nullable String status;
/**
* @return The system meta data relating to this resource.
*
*/
private SystemDataResponse systemData;
/**
* @return The type of the resource. E.g. "Microsoft.EventHub/Namespaces" or "Microsoft.EventHub/Namespaces/EventHubs"
*
*/
private String type;
/**
* @return The exact time the message was updated.
*
*/
private String updatedAt;
private GetQueueResult() {}
/**
* @return Last time a message was sent, or the last time there was a receive request to this queue.
*
*/
public String accessedAt() {
return this.accessedAt;
}
/**
* @return ISO 8061 timeSpan idle interval after which the queue is automatically deleted. The minimum duration is 5 minutes.
*
*/
public Optional autoDeleteOnIdle() {
return Optional.ofNullable(this.autoDeleteOnIdle);
}
/**
* @return Message Count Details.
*
*/
public MessageCountDetailsResponse countDetails() {
return this.countDetails;
}
/**
* @return The exact time the message was created.
*
*/
public String createdAt() {
return this.createdAt;
}
/**
* @return A value that indicates whether this queue has dead letter support when a message expires.
*
*/
public Optional deadLetteringOnMessageExpiration() {
return Optional.ofNullable(this.deadLetteringOnMessageExpiration);
}
/**
* @return ISO 8601 default message timespan to live value. This is the duration after which the message expires, starting from when the message is sent to Service Bus. This is the default value used when TimeToLive is not set on a message itself.
*
*/
public Optional defaultMessageTimeToLive() {
return Optional.ofNullable(this.defaultMessageTimeToLive);
}
/**
* @return ISO 8601 timeSpan structure that defines the duration of the duplicate detection history. The default value is 10 minutes.
*
*/
public Optional duplicateDetectionHistoryTimeWindow() {
return Optional.ofNullable(this.duplicateDetectionHistoryTimeWindow);
}
/**
* @return Value that indicates whether server-side batched operations are enabled.
*
*/
public Optional enableBatchedOperations() {
return Optional.ofNullable(this.enableBatchedOperations);
}
/**
* @return A value that indicates whether Express Entities are enabled. An express queue holds a message in memory temporarily before writing it to persistent storage.
*
*/
public Optional enableExpress() {
return Optional.ofNullable(this.enableExpress);
}
/**
* @return A value that indicates whether the queue is to be partitioned across multiple message brokers.
*
*/
public Optional enablePartitioning() {
return Optional.ofNullable(this.enablePartitioning);
}
/**
* @return Queue/Topic name to forward the Dead Letter message
*
*/
public Optional forwardDeadLetteredMessagesTo() {
return Optional.ofNullable(this.forwardDeadLetteredMessagesTo);
}
/**
* @return Queue/Topic name to forward the messages
*
*/
public Optional forwardTo() {
return Optional.ofNullable(this.forwardTo);
}
/**
* @return Fully qualified resource ID for the resource. Ex - /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/{resourceProviderNamespace}/{resourceType}/{resourceName}
*
*/
public String id() {
return this.id;
}
/**
* @return The geo-location where the resource lives
*
*/
public String location() {
return this.location;
}
/**
* @return ISO 8601 timespan duration of a peek-lock; that is, the amount of time that the message is locked for other receivers. The maximum value for LockDuration is 5 minutes; the default value is 1 minute.
*
*/
public Optional lockDuration() {
return Optional.ofNullable(this.lockDuration);
}
/**
* @return The maximum delivery count. A message is automatically deadlettered after this number of deliveries. default value is 10.
*
*/
public Optional maxDeliveryCount() {
return Optional.ofNullable(this.maxDeliveryCount);
}
/**
* @return Maximum size (in KB) of the message payload that can be accepted by the queue. This property is only used in Premium today and default is 1024.
*
*/
public Optional maxMessageSizeInKilobytes() {
return Optional.ofNullable(this.maxMessageSizeInKilobytes);
}
/**
* @return The maximum size of the queue in megabytes, which is the size of memory allocated for the queue. Default is 1024.
*
*/
public Optional maxSizeInMegabytes() {
return Optional.ofNullable(this.maxSizeInMegabytes);
}
/**
* @return The number of messages in the queue.
*
*/
public Double messageCount() {
return this.messageCount;
}
/**
* @return The name of the resource
*
*/
public String name() {
return this.name;
}
/**
* @return A value indicating if this queue requires duplicate detection.
*
*/
public Optional requiresDuplicateDetection() {
return Optional.ofNullable(this.requiresDuplicateDetection);
}
/**
* @return A value that indicates whether the queue supports the concept of sessions.
*
*/
public Optional requiresSession() {
return Optional.ofNullable(this.requiresSession);
}
/**
* @return The size of the queue, in bytes.
*
*/
public Double sizeInBytes() {
return this.sizeInBytes;
}
/**
* @return Enumerates the possible values for the status of a messaging entity.
*
*/
public Optional status() {
return Optional.ofNullable(this.status);
}
/**
* @return The system meta data relating to this resource.
*
*/
public SystemDataResponse systemData() {
return this.systemData;
}
/**
* @return The type of the resource. E.g. "Microsoft.EventHub/Namespaces" or "Microsoft.EventHub/Namespaces/EventHubs"
*
*/
public String type() {
return this.type;
}
/**
* @return The exact time the message was updated.
*
*/
public String updatedAt() {
return this.updatedAt;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetQueueResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String accessedAt;
private @Nullable String autoDeleteOnIdle;
private MessageCountDetailsResponse countDetails;
private String createdAt;
private @Nullable Boolean deadLetteringOnMessageExpiration;
private @Nullable String defaultMessageTimeToLive;
private @Nullable String duplicateDetectionHistoryTimeWindow;
private @Nullable Boolean enableBatchedOperations;
private @Nullable Boolean enableExpress;
private @Nullable Boolean enablePartitioning;
private @Nullable String forwardDeadLetteredMessagesTo;
private @Nullable String forwardTo;
private String id;
private String location;
private @Nullable String lockDuration;
private @Nullable Integer maxDeliveryCount;
private @Nullable Double maxMessageSizeInKilobytes;
private @Nullable Integer maxSizeInMegabytes;
private Double messageCount;
private String name;
private @Nullable Boolean requiresDuplicateDetection;
private @Nullable Boolean requiresSession;
private Double sizeInBytes;
private @Nullable String status;
private SystemDataResponse systemData;
private String type;
private String updatedAt;
public Builder() {}
public Builder(GetQueueResult defaults) {
Objects.requireNonNull(defaults);
this.accessedAt = defaults.accessedAt;
this.autoDeleteOnIdle = defaults.autoDeleteOnIdle;
this.countDetails = defaults.countDetails;
this.createdAt = defaults.createdAt;
this.deadLetteringOnMessageExpiration = defaults.deadLetteringOnMessageExpiration;
this.defaultMessageTimeToLive = defaults.defaultMessageTimeToLive;
this.duplicateDetectionHistoryTimeWindow = defaults.duplicateDetectionHistoryTimeWindow;
this.enableBatchedOperations = defaults.enableBatchedOperations;
this.enableExpress = defaults.enableExpress;
this.enablePartitioning = defaults.enablePartitioning;
this.forwardDeadLetteredMessagesTo = defaults.forwardDeadLetteredMessagesTo;
this.forwardTo = defaults.forwardTo;
this.id = defaults.id;
this.location = defaults.location;
this.lockDuration = defaults.lockDuration;
this.maxDeliveryCount = defaults.maxDeliveryCount;
this.maxMessageSizeInKilobytes = defaults.maxMessageSizeInKilobytes;
this.maxSizeInMegabytes = defaults.maxSizeInMegabytes;
this.messageCount = defaults.messageCount;
this.name = defaults.name;
this.requiresDuplicateDetection = defaults.requiresDuplicateDetection;
this.requiresSession = defaults.requiresSession;
this.sizeInBytes = defaults.sizeInBytes;
this.status = defaults.status;
this.systemData = defaults.systemData;
this.type = defaults.type;
this.updatedAt = defaults.updatedAt;
}
@CustomType.Setter
public Builder accessedAt(String accessedAt) {
if (accessedAt == null) {
throw new MissingRequiredPropertyException("GetQueueResult", "accessedAt");
}
this.accessedAt = accessedAt;
return this;
}
@CustomType.Setter
public Builder autoDeleteOnIdle(@Nullable String autoDeleteOnIdle) {
this.autoDeleteOnIdle = autoDeleteOnIdle;
return this;
}
@CustomType.Setter
public Builder countDetails(MessageCountDetailsResponse countDetails) {
if (countDetails == null) {
throw new MissingRequiredPropertyException("GetQueueResult", "countDetails");
}
this.countDetails = countDetails;
return this;
}
@CustomType.Setter
public Builder createdAt(String createdAt) {
if (createdAt == null) {
throw new MissingRequiredPropertyException("GetQueueResult", "createdAt");
}
this.createdAt = createdAt;
return this;
}
@CustomType.Setter
public Builder deadLetteringOnMessageExpiration(@Nullable Boolean deadLetteringOnMessageExpiration) {
this.deadLetteringOnMessageExpiration = deadLetteringOnMessageExpiration;
return this;
}
@CustomType.Setter
public Builder defaultMessageTimeToLive(@Nullable String defaultMessageTimeToLive) {
this.defaultMessageTimeToLive = defaultMessageTimeToLive;
return this;
}
@CustomType.Setter
public Builder duplicateDetectionHistoryTimeWindow(@Nullable String duplicateDetectionHistoryTimeWindow) {
this.duplicateDetectionHistoryTimeWindow = duplicateDetectionHistoryTimeWindow;
return this;
}
@CustomType.Setter
public Builder enableBatchedOperations(@Nullable Boolean enableBatchedOperations) {
this.enableBatchedOperations = enableBatchedOperations;
return this;
}
@CustomType.Setter
public Builder enableExpress(@Nullable Boolean enableExpress) {
this.enableExpress = enableExpress;
return this;
}
@CustomType.Setter
public Builder enablePartitioning(@Nullable Boolean enablePartitioning) {
this.enablePartitioning = enablePartitioning;
return this;
}
@CustomType.Setter
public Builder forwardDeadLetteredMessagesTo(@Nullable String forwardDeadLetteredMessagesTo) {
this.forwardDeadLetteredMessagesTo = forwardDeadLetteredMessagesTo;
return this;
}
@CustomType.Setter
public Builder forwardTo(@Nullable String forwardTo) {
this.forwardTo = forwardTo;
return this;
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetQueueResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder location(String location) {
if (location == null) {
throw new MissingRequiredPropertyException("GetQueueResult", "location");
}
this.location = location;
return this;
}
@CustomType.Setter
public Builder lockDuration(@Nullable String lockDuration) {
this.lockDuration = lockDuration;
return this;
}
@CustomType.Setter
public Builder maxDeliveryCount(@Nullable Integer maxDeliveryCount) {
this.maxDeliveryCount = maxDeliveryCount;
return this;
}
@CustomType.Setter
public Builder maxMessageSizeInKilobytes(@Nullable Double maxMessageSizeInKilobytes) {
this.maxMessageSizeInKilobytes = maxMessageSizeInKilobytes;
return this;
}
@CustomType.Setter
public Builder maxSizeInMegabytes(@Nullable Integer maxSizeInMegabytes) {
this.maxSizeInMegabytes = maxSizeInMegabytes;
return this;
}
@CustomType.Setter
public Builder messageCount(Double messageCount) {
if (messageCount == null) {
throw new MissingRequiredPropertyException("GetQueueResult", "messageCount");
}
this.messageCount = messageCount;
return this;
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("GetQueueResult", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder requiresDuplicateDetection(@Nullable Boolean requiresDuplicateDetection) {
this.requiresDuplicateDetection = requiresDuplicateDetection;
return this;
}
@CustomType.Setter
public Builder requiresSession(@Nullable Boolean requiresSession) {
this.requiresSession = requiresSession;
return this;
}
@CustomType.Setter
public Builder sizeInBytes(Double sizeInBytes) {
if (sizeInBytes == null) {
throw new MissingRequiredPropertyException("GetQueueResult", "sizeInBytes");
}
this.sizeInBytes = sizeInBytes;
return this;
}
@CustomType.Setter
public Builder status(@Nullable String status) {
this.status = status;
return this;
}
@CustomType.Setter
public Builder systemData(SystemDataResponse systemData) {
if (systemData == null) {
throw new MissingRequiredPropertyException("GetQueueResult", "systemData");
}
this.systemData = systemData;
return this;
}
@CustomType.Setter
public Builder type(String type) {
if (type == null) {
throw new MissingRequiredPropertyException("GetQueueResult", "type");
}
this.type = type;
return this;
}
@CustomType.Setter
public Builder updatedAt(String updatedAt) {
if (updatedAt == null) {
throw new MissingRequiredPropertyException("GetQueueResult", "updatedAt");
}
this.updatedAt = updatedAt;
return this;
}
public GetQueueResult build() {
final var _resultValue = new GetQueueResult();
_resultValue.accessedAt = accessedAt;
_resultValue.autoDeleteOnIdle = autoDeleteOnIdle;
_resultValue.countDetails = countDetails;
_resultValue.createdAt = createdAt;
_resultValue.deadLetteringOnMessageExpiration = deadLetteringOnMessageExpiration;
_resultValue.defaultMessageTimeToLive = defaultMessageTimeToLive;
_resultValue.duplicateDetectionHistoryTimeWindow = duplicateDetectionHistoryTimeWindow;
_resultValue.enableBatchedOperations = enableBatchedOperations;
_resultValue.enableExpress = enableExpress;
_resultValue.enablePartitioning = enablePartitioning;
_resultValue.forwardDeadLetteredMessagesTo = forwardDeadLetteredMessagesTo;
_resultValue.forwardTo = forwardTo;
_resultValue.id = id;
_resultValue.location = location;
_resultValue.lockDuration = lockDuration;
_resultValue.maxDeliveryCount = maxDeliveryCount;
_resultValue.maxMessageSizeInKilobytes = maxMessageSizeInKilobytes;
_resultValue.maxSizeInMegabytes = maxSizeInMegabytes;
_resultValue.messageCount = messageCount;
_resultValue.name = name;
_resultValue.requiresDuplicateDetection = requiresDuplicateDetection;
_resultValue.requiresSession = requiresSession;
_resultValue.sizeInBytes = sizeInBytes;
_resultValue.status = status;
_resultValue.systemData = systemData;
_resultValue.type = type;
_resultValue.updatedAt = updatedAt;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy