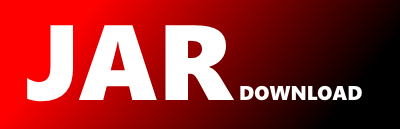
com.pulumi.azurenative.servicefabricmesh.ApplicationArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.servicefabricmesh;
import com.pulumi.azurenative.servicefabricmesh.inputs.DiagnosticsDescriptionArgs;
import com.pulumi.azurenative.servicefabricmesh.inputs.ServiceResourceDescriptionArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class ApplicationArgs extends com.pulumi.resources.ResourceArgs {
public static final ApplicationArgs Empty = new ApplicationArgs();
/**
* The identity of the application.
*
*/
@Import(name="applicationResourceName")
private @Nullable Output applicationResourceName;
/**
* @return The identity of the application.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy