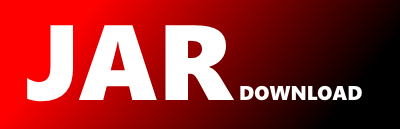
com.pulumi.azurenative.servicefabricmesh.ServicefabricmeshFunctions Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.servicefabricmesh;
import com.pulumi.azurenative.Utilities;
import com.pulumi.azurenative.servicefabricmesh.inputs.GetApplicationArgs;
import com.pulumi.azurenative.servicefabricmesh.inputs.GetApplicationPlainArgs;
import com.pulumi.azurenative.servicefabricmesh.inputs.GetGatewayArgs;
import com.pulumi.azurenative.servicefabricmesh.inputs.GetGatewayPlainArgs;
import com.pulumi.azurenative.servicefabricmesh.inputs.GetNetworkArgs;
import com.pulumi.azurenative.servicefabricmesh.inputs.GetNetworkPlainArgs;
import com.pulumi.azurenative.servicefabricmesh.inputs.GetSecretArgs;
import com.pulumi.azurenative.servicefabricmesh.inputs.GetSecretPlainArgs;
import com.pulumi.azurenative.servicefabricmesh.inputs.GetSecretValueArgs;
import com.pulumi.azurenative.servicefabricmesh.inputs.GetSecretValuePlainArgs;
import com.pulumi.azurenative.servicefabricmesh.inputs.GetVolumeArgs;
import com.pulumi.azurenative.servicefabricmesh.inputs.GetVolumePlainArgs;
import com.pulumi.azurenative.servicefabricmesh.inputs.ListSecretValueArgs;
import com.pulumi.azurenative.servicefabricmesh.inputs.ListSecretValuePlainArgs;
import com.pulumi.azurenative.servicefabricmesh.outputs.GetApplicationResult;
import com.pulumi.azurenative.servicefabricmesh.outputs.GetGatewayResult;
import com.pulumi.azurenative.servicefabricmesh.outputs.GetNetworkResult;
import com.pulumi.azurenative.servicefabricmesh.outputs.GetSecretResult;
import com.pulumi.azurenative.servicefabricmesh.outputs.GetSecretValueResult;
import com.pulumi.azurenative.servicefabricmesh.outputs.GetVolumeResult;
import com.pulumi.azurenative.servicefabricmesh.outputs.ListSecretValueResult;
import com.pulumi.core.Output;
import com.pulumi.core.TypeShape;
import com.pulumi.deployment.Deployment;
import com.pulumi.deployment.InvokeOptions;
import java.util.concurrent.CompletableFuture;
public final class ServicefabricmeshFunctions {
/**
* Gets the information about the application resource with the given name. The information include the description and other properties of the application.
* Azure REST API version: 2018-09-01-preview.
*
* Other available API versions: 2018-07-01-preview.
*
*/
public static Output getApplication(GetApplicationArgs args) {
return getApplication(args, InvokeOptions.Empty);
}
/**
* Gets the information about the application resource with the given name. The information include the description and other properties of the application.
* Azure REST API version: 2018-09-01-preview.
*
* Other available API versions: 2018-07-01-preview.
*
*/
public static CompletableFuture getApplicationPlain(GetApplicationPlainArgs args) {
return getApplicationPlain(args, InvokeOptions.Empty);
}
/**
* Gets the information about the application resource with the given name. The information include the description and other properties of the application.
* Azure REST API version: 2018-09-01-preview.
*
* Other available API versions: 2018-07-01-preview.
*
*/
public static Output getApplication(GetApplicationArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:servicefabricmesh:getApplication", TypeShape.of(GetApplicationResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the information about the application resource with the given name. The information include the description and other properties of the application.
* Azure REST API version: 2018-09-01-preview.
*
* Other available API versions: 2018-07-01-preview.
*
*/
public static CompletableFuture getApplicationPlain(GetApplicationPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:servicefabricmesh:getApplication", TypeShape.of(GetApplicationResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the information about the gateway resource with the given name. The information include the description and other properties of the gateway.
* Azure REST API version: 2018-09-01-preview.
*
*/
public static Output getGateway(GetGatewayArgs args) {
return getGateway(args, InvokeOptions.Empty);
}
/**
* Gets the information about the gateway resource with the given name. The information include the description and other properties of the gateway.
* Azure REST API version: 2018-09-01-preview.
*
*/
public static CompletableFuture getGatewayPlain(GetGatewayPlainArgs args) {
return getGatewayPlain(args, InvokeOptions.Empty);
}
/**
* Gets the information about the gateway resource with the given name. The information include the description and other properties of the gateway.
* Azure REST API version: 2018-09-01-preview.
*
*/
public static Output getGateway(GetGatewayArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:servicefabricmesh:getGateway", TypeShape.of(GetGatewayResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the information about the gateway resource with the given name. The information include the description and other properties of the gateway.
* Azure REST API version: 2018-09-01-preview.
*
*/
public static CompletableFuture getGatewayPlain(GetGatewayPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:servicefabricmesh:getGateway", TypeShape.of(GetGatewayResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the information about the network resource with the given name. The information include the description and other properties of the network.
* Azure REST API version: 2018-09-01-preview.
*
* Other available API versions: 2018-07-01-preview.
*
*/
public static Output getNetwork(GetNetworkArgs args) {
return getNetwork(args, InvokeOptions.Empty);
}
/**
* Gets the information about the network resource with the given name. The information include the description and other properties of the network.
* Azure REST API version: 2018-09-01-preview.
*
* Other available API versions: 2018-07-01-preview.
*
*/
public static CompletableFuture getNetworkPlain(GetNetworkPlainArgs args) {
return getNetworkPlain(args, InvokeOptions.Empty);
}
/**
* Gets the information about the network resource with the given name. The information include the description and other properties of the network.
* Azure REST API version: 2018-09-01-preview.
*
* Other available API versions: 2018-07-01-preview.
*
*/
public static Output getNetwork(GetNetworkArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:servicefabricmesh:getNetwork", TypeShape.of(GetNetworkResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the information about the network resource with the given name. The information include the description and other properties of the network.
* Azure REST API version: 2018-09-01-preview.
*
* Other available API versions: 2018-07-01-preview.
*
*/
public static CompletableFuture getNetworkPlain(GetNetworkPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:servicefabricmesh:getNetwork", TypeShape.of(GetNetworkResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the information about the secret resource with the given name. The information include the description and other properties of the secret.
* Azure REST API version: 2018-09-01-preview.
*
*/
public static Output getSecret(GetSecretArgs args) {
return getSecret(args, InvokeOptions.Empty);
}
/**
* Gets the information about the secret resource with the given name. The information include the description and other properties of the secret.
* Azure REST API version: 2018-09-01-preview.
*
*/
public static CompletableFuture getSecretPlain(GetSecretPlainArgs args) {
return getSecretPlain(args, InvokeOptions.Empty);
}
/**
* Gets the information about the secret resource with the given name. The information include the description and other properties of the secret.
* Azure REST API version: 2018-09-01-preview.
*
*/
public static Output getSecret(GetSecretArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:servicefabricmesh:getSecret", TypeShape.of(GetSecretResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the information about the secret resource with the given name. The information include the description and other properties of the secret.
* Azure REST API version: 2018-09-01-preview.
*
*/
public static CompletableFuture getSecretPlain(GetSecretPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:servicefabricmesh:getSecret", TypeShape.of(GetSecretResult.class), args, Utilities.withVersion(options));
}
/**
* Get the information about the specified named secret value resources. The information does not include the actual value of the secret.
* Azure REST API version: 2018-09-01-preview.
*
*/
public static Output getSecretValue(GetSecretValueArgs args) {
return getSecretValue(args, InvokeOptions.Empty);
}
/**
* Get the information about the specified named secret value resources. The information does not include the actual value of the secret.
* Azure REST API version: 2018-09-01-preview.
*
*/
public static CompletableFuture getSecretValuePlain(GetSecretValuePlainArgs args) {
return getSecretValuePlain(args, InvokeOptions.Empty);
}
/**
* Get the information about the specified named secret value resources. The information does not include the actual value of the secret.
* Azure REST API version: 2018-09-01-preview.
*
*/
public static Output getSecretValue(GetSecretValueArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:servicefabricmesh:getSecretValue", TypeShape.of(GetSecretValueResult.class), args, Utilities.withVersion(options));
}
/**
* Get the information about the specified named secret value resources. The information does not include the actual value of the secret.
* Azure REST API version: 2018-09-01-preview.
*
*/
public static CompletableFuture getSecretValuePlain(GetSecretValuePlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:servicefabricmesh:getSecretValue", TypeShape.of(GetSecretValueResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the information about the volume resource with the given name. The information include the description and other properties of the volume.
* Azure REST API version: 2018-09-01-preview.
*
* Other available API versions: 2018-07-01-preview.
*
*/
public static Output getVolume(GetVolumeArgs args) {
return getVolume(args, InvokeOptions.Empty);
}
/**
* Gets the information about the volume resource with the given name. The information include the description and other properties of the volume.
* Azure REST API version: 2018-09-01-preview.
*
* Other available API versions: 2018-07-01-preview.
*
*/
public static CompletableFuture getVolumePlain(GetVolumePlainArgs args) {
return getVolumePlain(args, InvokeOptions.Empty);
}
/**
* Gets the information about the volume resource with the given name. The information include the description and other properties of the volume.
* Azure REST API version: 2018-09-01-preview.
*
* Other available API versions: 2018-07-01-preview.
*
*/
public static Output getVolume(GetVolumeArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:servicefabricmesh:getVolume", TypeShape.of(GetVolumeResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the information about the volume resource with the given name. The information include the description and other properties of the volume.
* Azure REST API version: 2018-09-01-preview.
*
* Other available API versions: 2018-07-01-preview.
*
*/
public static CompletableFuture getVolumePlain(GetVolumePlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:servicefabricmesh:getVolume", TypeShape.of(GetVolumeResult.class), args, Utilities.withVersion(options));
}
/**
* Lists the decrypted value of the specified named value of the secret resource. This is a privileged operation.
* Azure REST API version: 2018-09-01-preview.
*
*/
public static Output listSecretValue(ListSecretValueArgs args) {
return listSecretValue(args, InvokeOptions.Empty);
}
/**
* Lists the decrypted value of the specified named value of the secret resource. This is a privileged operation.
* Azure REST API version: 2018-09-01-preview.
*
*/
public static CompletableFuture listSecretValuePlain(ListSecretValuePlainArgs args) {
return listSecretValuePlain(args, InvokeOptions.Empty);
}
/**
* Lists the decrypted value of the specified named value of the secret resource. This is a privileged operation.
* Azure REST API version: 2018-09-01-preview.
*
*/
public static Output listSecretValue(ListSecretValueArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:servicefabricmesh:listSecretValue", TypeShape.of(ListSecretValueResult.class), args, Utilities.withVersion(options));
}
/**
* Lists the decrypted value of the specified named value of the secret resource. This is a privileged operation.
* Azure REST API version: 2018-09-01-preview.
*
*/
public static CompletableFuture listSecretValuePlain(ListSecretValuePlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:servicefabricmesh:listSecretValue", TypeShape.of(ListSecretValueResult.class), args, Utilities.withVersion(options));
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy