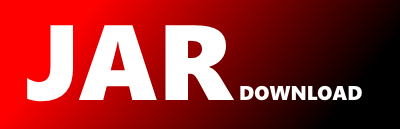
com.pulumi.azurenative.servicenetworking.ServicenetworkingFunctions Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.servicenetworking;
import com.pulumi.azurenative.Utilities;
import com.pulumi.azurenative.servicenetworking.inputs.GetAssociationsInterfaceArgs;
import com.pulumi.azurenative.servicenetworking.inputs.GetAssociationsInterfacePlainArgs;
import com.pulumi.azurenative.servicenetworking.inputs.GetFrontendsInterfaceArgs;
import com.pulumi.azurenative.servicenetworking.inputs.GetFrontendsInterfacePlainArgs;
import com.pulumi.azurenative.servicenetworking.inputs.GetSecurityPoliciesInterfaceArgs;
import com.pulumi.azurenative.servicenetworking.inputs.GetSecurityPoliciesInterfacePlainArgs;
import com.pulumi.azurenative.servicenetworking.inputs.GetTrafficControllerInterfaceArgs;
import com.pulumi.azurenative.servicenetworking.inputs.GetTrafficControllerInterfacePlainArgs;
import com.pulumi.azurenative.servicenetworking.outputs.GetAssociationsInterfaceResult;
import com.pulumi.azurenative.servicenetworking.outputs.GetFrontendsInterfaceResult;
import com.pulumi.azurenative.servicenetworking.outputs.GetSecurityPoliciesInterfaceResult;
import com.pulumi.azurenative.servicenetworking.outputs.GetTrafficControllerInterfaceResult;
import com.pulumi.core.Output;
import com.pulumi.core.TypeShape;
import com.pulumi.deployment.Deployment;
import com.pulumi.deployment.InvokeOptions;
import java.util.concurrent.CompletableFuture;
public final class ServicenetworkingFunctions {
/**
* Get a Association
* Azure REST API version: 2023-05-01-preview.
*
* Other available API versions: 2022-10-01-preview, 2023-11-01, 2024-05-01-preview.
*
*/
public static Output getAssociationsInterface(GetAssociationsInterfaceArgs args) {
return getAssociationsInterface(args, InvokeOptions.Empty);
}
/**
* Get a Association
* Azure REST API version: 2023-05-01-preview.
*
* Other available API versions: 2022-10-01-preview, 2023-11-01, 2024-05-01-preview.
*
*/
public static CompletableFuture getAssociationsInterfacePlain(GetAssociationsInterfacePlainArgs args) {
return getAssociationsInterfacePlain(args, InvokeOptions.Empty);
}
/**
* Get a Association
* Azure REST API version: 2023-05-01-preview.
*
* Other available API versions: 2022-10-01-preview, 2023-11-01, 2024-05-01-preview.
*
*/
public static Output getAssociationsInterface(GetAssociationsInterfaceArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:servicenetworking:getAssociationsInterface", TypeShape.of(GetAssociationsInterfaceResult.class), args, Utilities.withVersion(options));
}
/**
* Get a Association
* Azure REST API version: 2023-05-01-preview.
*
* Other available API versions: 2022-10-01-preview, 2023-11-01, 2024-05-01-preview.
*
*/
public static CompletableFuture getAssociationsInterfacePlain(GetAssociationsInterfacePlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:servicenetworking:getAssociationsInterface", TypeShape.of(GetAssociationsInterfaceResult.class), args, Utilities.withVersion(options));
}
/**
* Get a Frontend
* Azure REST API version: 2023-05-01-preview.
*
* Other available API versions: 2022-10-01-preview, 2023-11-01, 2024-05-01-preview.
*
*/
public static Output getFrontendsInterface(GetFrontendsInterfaceArgs args) {
return getFrontendsInterface(args, InvokeOptions.Empty);
}
/**
* Get a Frontend
* Azure REST API version: 2023-05-01-preview.
*
* Other available API versions: 2022-10-01-preview, 2023-11-01, 2024-05-01-preview.
*
*/
public static CompletableFuture getFrontendsInterfacePlain(GetFrontendsInterfacePlainArgs args) {
return getFrontendsInterfacePlain(args, InvokeOptions.Empty);
}
/**
* Get a Frontend
* Azure REST API version: 2023-05-01-preview.
*
* Other available API versions: 2022-10-01-preview, 2023-11-01, 2024-05-01-preview.
*
*/
public static Output getFrontendsInterface(GetFrontendsInterfaceArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:servicenetworking:getFrontendsInterface", TypeShape.of(GetFrontendsInterfaceResult.class), args, Utilities.withVersion(options));
}
/**
* Get a Frontend
* Azure REST API version: 2023-05-01-preview.
*
* Other available API versions: 2022-10-01-preview, 2023-11-01, 2024-05-01-preview.
*
*/
public static CompletableFuture getFrontendsInterfacePlain(GetFrontendsInterfacePlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:servicenetworking:getFrontendsInterface", TypeShape.of(GetFrontendsInterfaceResult.class), args, Utilities.withVersion(options));
}
/**
* Get a SecurityPolicy
* Azure REST API version: 2024-05-01-preview.
*
*/
public static Output getSecurityPoliciesInterface(GetSecurityPoliciesInterfaceArgs args) {
return getSecurityPoliciesInterface(args, InvokeOptions.Empty);
}
/**
* Get a SecurityPolicy
* Azure REST API version: 2024-05-01-preview.
*
*/
public static CompletableFuture getSecurityPoliciesInterfacePlain(GetSecurityPoliciesInterfacePlainArgs args) {
return getSecurityPoliciesInterfacePlain(args, InvokeOptions.Empty);
}
/**
* Get a SecurityPolicy
* Azure REST API version: 2024-05-01-preview.
*
*/
public static Output getSecurityPoliciesInterface(GetSecurityPoliciesInterfaceArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:servicenetworking:getSecurityPoliciesInterface", TypeShape.of(GetSecurityPoliciesInterfaceResult.class), args, Utilities.withVersion(options));
}
/**
* Get a SecurityPolicy
* Azure REST API version: 2024-05-01-preview.
*
*/
public static CompletableFuture getSecurityPoliciesInterfacePlain(GetSecurityPoliciesInterfacePlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:servicenetworking:getSecurityPoliciesInterface", TypeShape.of(GetSecurityPoliciesInterfaceResult.class), args, Utilities.withVersion(options));
}
/**
* Get a TrafficController
* Azure REST API version: 2023-05-01-preview.
*
* Other available API versions: 2022-10-01-preview, 2023-11-01, 2024-05-01-preview.
*
*/
public static Output getTrafficControllerInterface(GetTrafficControllerInterfaceArgs args) {
return getTrafficControllerInterface(args, InvokeOptions.Empty);
}
/**
* Get a TrafficController
* Azure REST API version: 2023-05-01-preview.
*
* Other available API versions: 2022-10-01-preview, 2023-11-01, 2024-05-01-preview.
*
*/
public static CompletableFuture getTrafficControllerInterfacePlain(GetTrafficControllerInterfacePlainArgs args) {
return getTrafficControllerInterfacePlain(args, InvokeOptions.Empty);
}
/**
* Get a TrafficController
* Azure REST API version: 2023-05-01-preview.
*
* Other available API versions: 2022-10-01-preview, 2023-11-01, 2024-05-01-preview.
*
*/
public static Output getTrafficControllerInterface(GetTrafficControllerInterfaceArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:servicenetworking:getTrafficControllerInterface", TypeShape.of(GetTrafficControllerInterfaceResult.class), args, Utilities.withVersion(options));
}
/**
* Get a TrafficController
* Azure REST API version: 2023-05-01-preview.
*
* Other available API versions: 2022-10-01-preview, 2023-11-01, 2024-05-01-preview.
*
*/
public static CompletableFuture getTrafficControllerInterfacePlain(GetTrafficControllerInterfacePlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:servicenetworking:getTrafficControllerInterface", TypeShape.of(GetTrafficControllerInterfaceResult.class), args, Utilities.withVersion(options));
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy