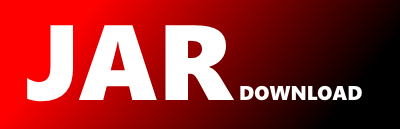
com.pulumi.azurenative.sql.JobStepArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.sql;
import com.pulumi.azurenative.sql.inputs.JobStepActionArgs;
import com.pulumi.azurenative.sql.inputs.JobStepExecutionOptionsArgs;
import com.pulumi.azurenative.sql.inputs.JobStepOutputArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Integer;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class JobStepArgs extends com.pulumi.resources.ResourceArgs {
public static final JobStepArgs Empty = new JobStepArgs();
/**
* The action payload of the job step.
*
*/
@Import(name="action", required=true)
private Output action;
/**
* @return The action payload of the job step.
*
*/
public Output action() {
return this.action;
}
/**
* The resource ID of the job credential that will be used to connect to the targets.
*
*/
@Import(name="credential", required=true)
private Output credential;
/**
* @return The resource ID of the job credential that will be used to connect to the targets.
*
*/
public Output credential() {
return this.credential;
}
/**
* Execution options for the job step.
*
*/
@Import(name="executionOptions")
private @Nullable Output executionOptions;
/**
* @return Execution options for the job step.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy