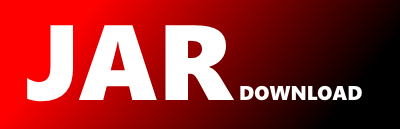
com.pulumi.azurenative.sql.ServerAdvisor Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.sql;
import com.pulumi.azurenative.Utilities;
import com.pulumi.azurenative.sql.ServerAdvisorArgs;
import com.pulumi.azurenative.sql.outputs.RecommendedActionResponse;
import com.pulumi.core.Alias;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.String;
import java.util.List;
import javax.annotation.Nullable;
/**
* Database, Server or Elastic Pool Advisor.
* Azure REST API version: 2021-11-01. Prior API version in Azure Native 1.x: 2020-11-01-preview.
*
* Other available API versions: 2014-04-01, 2022-11-01-preview, 2023-02-01-preview, 2023-05-01-preview, 2023-08-01-preview, 2024-05-01-preview.
*
* ## Example Usage
* ### Update server advisor
*
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.sql.ServerAdvisor;
* import com.pulumi.azurenative.sql.ServerAdvisorArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var serverAdvisor = new ServerAdvisor("serverAdvisor", ServerAdvisorArgs.builder()
* .advisorName("CreateIndex")
* .autoExecuteStatus("Disabled")
* .resourceGroupName("workloadinsight-demos")
* .serverName("misosisvr")
* .build());
*
* }
* }
*
* }
*
*
* ## Import
*
* An existing resource can be imported using its type token, name, and identifier, e.g.
*
* ```sh
* $ pulumi import azure-native:sql:ServerAdvisor CreateIndex /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Sql/servers/{serverName}/advisors/{advisorName}
* ```
*
*/
@ResourceType(type="azure-native:sql:ServerAdvisor")
public class ServerAdvisor extends com.pulumi.resources.CustomResource {
/**
* Gets the status of availability of this advisor to customers. Possible values are 'GA', 'PublicPreview', 'LimitedPublicPreview' and 'PrivatePreview'.
*
*/
@Export(name="advisorStatus", refs={String.class}, tree="[0]")
private Output advisorStatus;
/**
* @return Gets the status of availability of this advisor to customers. Possible values are 'GA', 'PublicPreview', 'LimitedPublicPreview' and 'PrivatePreview'.
*
*/
public Output advisorStatus() {
return this.advisorStatus;
}
/**
* Gets the auto-execute status (whether to let the system execute the recommendations) of this advisor. Possible values are 'Enabled' and 'Disabled'
*
*/
@Export(name="autoExecuteStatus", refs={String.class}, tree="[0]")
private Output autoExecuteStatus;
/**
* @return Gets the auto-execute status (whether to let the system execute the recommendations) of this advisor. Possible values are 'Enabled' and 'Disabled'
*
*/
public Output autoExecuteStatus() {
return this.autoExecuteStatus;
}
/**
* Gets the resource from which current value of auto-execute status is inherited. Auto-execute status can be set on (and inherited from) different levels in the resource hierarchy. Possible values are 'Subscription', 'Server', 'ElasticPool', 'Database' and 'Default' (when status is not explicitly set on any level).
*
*/
@Export(name="autoExecuteStatusInheritedFrom", refs={String.class}, tree="[0]")
private Output autoExecuteStatusInheritedFrom;
/**
* @return Gets the resource from which current value of auto-execute status is inherited. Auto-execute status can be set on (and inherited from) different levels in the resource hierarchy. Possible values are 'Subscription', 'Server', 'ElasticPool', 'Database' and 'Default' (when status is not explicitly set on any level).
*
*/
public Output autoExecuteStatusInheritedFrom() {
return this.autoExecuteStatusInheritedFrom;
}
/**
* Resource kind.
*
*/
@Export(name="kind", refs={String.class}, tree="[0]")
private Output kind;
/**
* @return Resource kind.
*
*/
public Output kind() {
return this.kind;
}
/**
* Gets the time when the current resource was analyzed for recommendations by this advisor.
*
*/
@Export(name="lastChecked", refs={String.class}, tree="[0]")
private Output lastChecked;
/**
* @return Gets the time when the current resource was analyzed for recommendations by this advisor.
*
*/
public Output lastChecked() {
return this.lastChecked;
}
/**
* Resource location.
*
*/
@Export(name="location", refs={String.class}, tree="[0]")
private Output location;
/**
* @return Resource location.
*
*/
public Output location() {
return this.location;
}
/**
* Resource name.
*
*/
@Export(name="name", refs={String.class}, tree="[0]")
private Output name;
/**
* @return Resource name.
*
*/
public Output name() {
return this.name;
}
/**
* Gets that status of recommendations for this advisor and reason for not having any recommendations. Possible values include, but are not limited to, 'Ok' (Recommendations available),LowActivity (not enough workload to analyze), 'DbSeemsTuned' (Database is doing well), etc.
*
*/
@Export(name="recommendationsStatus", refs={String.class}, tree="[0]")
private Output recommendationsStatus;
/**
* @return Gets that status of recommendations for this advisor and reason for not having any recommendations. Possible values include, but are not limited to, 'Ok' (Recommendations available),LowActivity (not enough workload to analyze), 'DbSeemsTuned' (Database is doing well), etc.
*
*/
public Output recommendationsStatus() {
return this.recommendationsStatus;
}
/**
* Gets the recommended actions for this advisor.
*
*/
@Export(name="recommendedActions", refs={List.class,RecommendedActionResponse.class}, tree="[0,1]")
private Output> recommendedActions;
/**
* @return Gets the recommended actions for this advisor.
*
*/
public Output> recommendedActions() {
return this.recommendedActions;
}
/**
* Resource type.
*
*/
@Export(name="type", refs={String.class}, tree="[0]")
private Output type;
/**
* @return Resource type.
*
*/
public Output type() {
return this.type;
}
/**
*
* @param name The _unique_ name of the resulting resource.
*/
public ServerAdvisor(java.lang.String name) {
this(name, ServerAdvisorArgs.Empty);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
*/
public ServerAdvisor(java.lang.String name, ServerAdvisorArgs args) {
this(name, args, null);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
* @param options A bag of options that control this resource's behavior.
*/
public ServerAdvisor(java.lang.String name, ServerAdvisorArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("azure-native:sql:ServerAdvisor", name, makeArgs(args, options), makeResourceOptions(options, Codegen.empty()), false);
}
private ServerAdvisor(java.lang.String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("azure-native:sql:ServerAdvisor", name, null, makeResourceOptions(options, id), false);
}
private static ServerAdvisorArgs makeArgs(ServerAdvisorArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
if (options != null && options.getUrn().isPresent()) {
return null;
}
return args == null ? ServerAdvisorArgs.Empty : args;
}
private static com.pulumi.resources.CustomResourceOptions makeResourceOptions(@Nullable com.pulumi.resources.CustomResourceOptions options, @Nullable Output id) {
var defaultOptions = com.pulumi.resources.CustomResourceOptions.builder()
.version(Utilities.getVersion())
.aliases(List.of(
Output.of(Alias.builder().type("azure-native:sql/v20140401:ServerAdvisor").build()),
Output.of(Alias.builder().type("azure-native:sql/v20150501preview:ServerAdvisor").build()),
Output.of(Alias.builder().type("azure-native:sql/v20200202preview:ServerAdvisor").build()),
Output.of(Alias.builder().type("azure-native:sql/v20200801preview:ServerAdvisor").build()),
Output.of(Alias.builder().type("azure-native:sql/v20201101preview:ServerAdvisor").build()),
Output.of(Alias.builder().type("azure-native:sql/v20210201preview:ServerAdvisor").build()),
Output.of(Alias.builder().type("azure-native:sql/v20210501preview:ServerAdvisor").build()),
Output.of(Alias.builder().type("azure-native:sql/v20210801preview:ServerAdvisor").build()),
Output.of(Alias.builder().type("azure-native:sql/v20211101:ServerAdvisor").build()),
Output.of(Alias.builder().type("azure-native:sql/v20211101preview:ServerAdvisor").build()),
Output.of(Alias.builder().type("azure-native:sql/v20220201preview:ServerAdvisor").build()),
Output.of(Alias.builder().type("azure-native:sql/v20220501preview:ServerAdvisor").build()),
Output.of(Alias.builder().type("azure-native:sql/v20220801preview:ServerAdvisor").build()),
Output.of(Alias.builder().type("azure-native:sql/v20221101preview:ServerAdvisor").build()),
Output.of(Alias.builder().type("azure-native:sql/v20230201preview:ServerAdvisor").build()),
Output.of(Alias.builder().type("azure-native:sql/v20230501preview:ServerAdvisor").build()),
Output.of(Alias.builder().type("azure-native:sql/v20230801preview:ServerAdvisor").build()),
Output.of(Alias.builder().type("azure-native:sql/v20240501preview:ServerAdvisor").build())
))
.build();
return com.pulumi.resources.CustomResourceOptions.merge(defaultOptions, options, id);
}
/**
* Get an existing Host resource's state with the given name, ID, and optional extra
* properties used to qualify the lookup.
*
* @param name The _unique_ name of the resulting resource.
* @param id The _unique_ provider ID of the resource to lookup.
* @param options Optional settings to control the behavior of the CustomResource.
*/
public static ServerAdvisor get(java.lang.String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
return new ServerAdvisor(name, id, options);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy