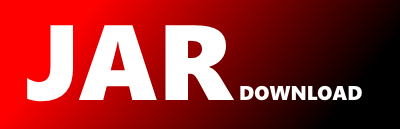
com.pulumi.azurenative.sql.outputs.GetDatabaseSecurityAlertPolicyResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.sql.outputs;
import com.pulumi.azurenative.sql.outputs.SystemDataResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class GetDatabaseSecurityAlertPolicyResult {
/**
* @return Specifies the UTC creation time of the policy.
*
*/
private String creationTime;
/**
* @return Specifies an array of alerts that are disabled. Allowed values are: Sql_Injection, Sql_Injection_Vulnerability, Access_Anomaly, Data_Exfiltration, Unsafe_Action, Brute_Force
*
*/
private @Nullable List disabledAlerts;
/**
* @return Specifies that the alert is sent to the account administrators.
*
*/
private @Nullable Boolean emailAccountAdmins;
/**
* @return Specifies an array of e-mail addresses to which the alert is sent.
*
*/
private @Nullable List emailAddresses;
/**
* @return Resource ID.
*
*/
private String id;
/**
* @return Resource name.
*
*/
private String name;
/**
* @return Specifies the number of days to keep in the Threat Detection audit logs.
*
*/
private @Nullable Integer retentionDays;
/**
* @return Specifies the state of the policy, whether it is enabled or disabled or a policy has not been applied yet on the specific database.
*
*/
private String state;
/**
* @return Specifies the identifier key of the Threat Detection audit storage account.
*
*/
private @Nullable String storageAccountAccessKey;
/**
* @return Specifies the blob storage endpoint (e.g. https://MyAccount.blob.core.windows.net). This blob storage will hold all Threat Detection audit logs.
*
*/
private @Nullable String storageEndpoint;
/**
* @return SystemData of SecurityAlertPolicyResource.
*
*/
private SystemDataResponse systemData;
/**
* @return Resource type.
*
*/
private String type;
private GetDatabaseSecurityAlertPolicyResult() {}
/**
* @return Specifies the UTC creation time of the policy.
*
*/
public String creationTime() {
return this.creationTime;
}
/**
* @return Specifies an array of alerts that are disabled. Allowed values are: Sql_Injection, Sql_Injection_Vulnerability, Access_Anomaly, Data_Exfiltration, Unsafe_Action, Brute_Force
*
*/
public List disabledAlerts() {
return this.disabledAlerts == null ? List.of() : this.disabledAlerts;
}
/**
* @return Specifies that the alert is sent to the account administrators.
*
*/
public Optional emailAccountAdmins() {
return Optional.ofNullable(this.emailAccountAdmins);
}
/**
* @return Specifies an array of e-mail addresses to which the alert is sent.
*
*/
public List emailAddresses() {
return this.emailAddresses == null ? List.of() : this.emailAddresses;
}
/**
* @return Resource ID.
*
*/
public String id() {
return this.id;
}
/**
* @return Resource name.
*
*/
public String name() {
return this.name;
}
/**
* @return Specifies the number of days to keep in the Threat Detection audit logs.
*
*/
public Optional retentionDays() {
return Optional.ofNullable(this.retentionDays);
}
/**
* @return Specifies the state of the policy, whether it is enabled or disabled or a policy has not been applied yet on the specific database.
*
*/
public String state() {
return this.state;
}
/**
* @return Specifies the identifier key of the Threat Detection audit storage account.
*
*/
public Optional storageAccountAccessKey() {
return Optional.ofNullable(this.storageAccountAccessKey);
}
/**
* @return Specifies the blob storage endpoint (e.g. https://MyAccount.blob.core.windows.net). This blob storage will hold all Threat Detection audit logs.
*
*/
public Optional storageEndpoint() {
return Optional.ofNullable(this.storageEndpoint);
}
/**
* @return SystemData of SecurityAlertPolicyResource.
*
*/
public SystemDataResponse systemData() {
return this.systemData;
}
/**
* @return Resource type.
*
*/
public String type() {
return this.type;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetDatabaseSecurityAlertPolicyResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String creationTime;
private @Nullable List disabledAlerts;
private @Nullable Boolean emailAccountAdmins;
private @Nullable List emailAddresses;
private String id;
private String name;
private @Nullable Integer retentionDays;
private String state;
private @Nullable String storageAccountAccessKey;
private @Nullable String storageEndpoint;
private SystemDataResponse systemData;
private String type;
public Builder() {}
public Builder(GetDatabaseSecurityAlertPolicyResult defaults) {
Objects.requireNonNull(defaults);
this.creationTime = defaults.creationTime;
this.disabledAlerts = defaults.disabledAlerts;
this.emailAccountAdmins = defaults.emailAccountAdmins;
this.emailAddresses = defaults.emailAddresses;
this.id = defaults.id;
this.name = defaults.name;
this.retentionDays = defaults.retentionDays;
this.state = defaults.state;
this.storageAccountAccessKey = defaults.storageAccountAccessKey;
this.storageEndpoint = defaults.storageEndpoint;
this.systemData = defaults.systemData;
this.type = defaults.type;
}
@CustomType.Setter
public Builder creationTime(String creationTime) {
if (creationTime == null) {
throw new MissingRequiredPropertyException("GetDatabaseSecurityAlertPolicyResult", "creationTime");
}
this.creationTime = creationTime;
return this;
}
@CustomType.Setter
public Builder disabledAlerts(@Nullable List disabledAlerts) {
this.disabledAlerts = disabledAlerts;
return this;
}
public Builder disabledAlerts(String... disabledAlerts) {
return disabledAlerts(List.of(disabledAlerts));
}
@CustomType.Setter
public Builder emailAccountAdmins(@Nullable Boolean emailAccountAdmins) {
this.emailAccountAdmins = emailAccountAdmins;
return this;
}
@CustomType.Setter
public Builder emailAddresses(@Nullable List emailAddresses) {
this.emailAddresses = emailAddresses;
return this;
}
public Builder emailAddresses(String... emailAddresses) {
return emailAddresses(List.of(emailAddresses));
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetDatabaseSecurityAlertPolicyResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("GetDatabaseSecurityAlertPolicyResult", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder retentionDays(@Nullable Integer retentionDays) {
this.retentionDays = retentionDays;
return this;
}
@CustomType.Setter
public Builder state(String state) {
if (state == null) {
throw new MissingRequiredPropertyException("GetDatabaseSecurityAlertPolicyResult", "state");
}
this.state = state;
return this;
}
@CustomType.Setter
public Builder storageAccountAccessKey(@Nullable String storageAccountAccessKey) {
this.storageAccountAccessKey = storageAccountAccessKey;
return this;
}
@CustomType.Setter
public Builder storageEndpoint(@Nullable String storageEndpoint) {
this.storageEndpoint = storageEndpoint;
return this;
}
@CustomType.Setter
public Builder systemData(SystemDataResponse systemData) {
if (systemData == null) {
throw new MissingRequiredPropertyException("GetDatabaseSecurityAlertPolicyResult", "systemData");
}
this.systemData = systemData;
return this;
}
@CustomType.Setter
public Builder type(String type) {
if (type == null) {
throw new MissingRequiredPropertyException("GetDatabaseSecurityAlertPolicyResult", "type");
}
this.type = type;
return this;
}
public GetDatabaseSecurityAlertPolicyResult build() {
final var _resultValue = new GetDatabaseSecurityAlertPolicyResult();
_resultValue.creationTime = creationTime;
_resultValue.disabledAlerts = disabledAlerts;
_resultValue.emailAccountAdmins = emailAccountAdmins;
_resultValue.emailAddresses = emailAddresses;
_resultValue.id = id;
_resultValue.name = name;
_resultValue.retentionDays = retentionDays;
_resultValue.state = state;
_resultValue.storageAccountAccessKey = storageAccountAccessKey;
_resultValue.storageEndpoint = storageEndpoint;
_resultValue.systemData = systemData;
_resultValue.type = type;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy