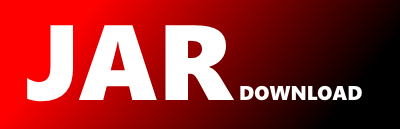
com.pulumi.azurenative.sql.outputs.GetElasticPoolResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.sql.outputs;
import com.pulumi.azurenative.sql.outputs.ElasticPoolPerDatabaseSettingsResponse;
import com.pulumi.azurenative.sql.outputs.SkuResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.Double;
import java.lang.Integer;
import java.lang.String;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class GetElasticPoolResult {
/**
* @return The creation date of the elastic pool (ISO8601 format).
*
*/
private String creationDate;
/**
* @return The number of secondary replicas associated with the elastic pool that are used to provide high availability. Applicable only to Hyperscale elastic pools.
*
*/
private @Nullable Integer highAvailabilityReplicaCount;
/**
* @return Resource ID.
*
*/
private String id;
/**
* @return Kind of elastic pool. This is metadata used for the Azure portal experience.
*
*/
private String kind;
/**
* @return The license type to apply for this elastic pool.
*
*/
private @Nullable String licenseType;
/**
* @return Resource location.
*
*/
private String location;
/**
* @return Maintenance configuration id assigned to the elastic pool. This configuration defines the period when the maintenance updates will will occur.
*
*/
private @Nullable String maintenanceConfigurationId;
/**
* @return The storage limit for the database elastic pool in bytes.
*
*/
private @Nullable Double maxSizeBytes;
/**
* @return Minimal capacity that serverless pool will not shrink below, if not paused
*
*/
private @Nullable Double minCapacity;
/**
* @return Resource name.
*
*/
private String name;
/**
* @return The per database settings for the elastic pool.
*
*/
private @Nullable ElasticPoolPerDatabaseSettingsResponse perDatabaseSettings;
/**
* @return The elastic pool SKU.
*
* The list of SKUs may vary by region and support offer. To determine the SKUs (including the SKU name, tier/edition, family, and capacity) that are available to your subscription in an Azure region, use the `Capabilities_ListByLocation` REST API or the following command:
*
*/
private @Nullable SkuResponse sku;
/**
* @return The state of the elastic pool.
*
*/
private String state;
/**
* @return Resource tags.
*
*/
private @Nullable Map tags;
/**
* @return Resource type.
*
*/
private String type;
/**
* @return Whether or not this elastic pool is zone redundant, which means the replicas of this elastic pool will be spread across multiple availability zones.
*
*/
private @Nullable Boolean zoneRedundant;
private GetElasticPoolResult() {}
/**
* @return The creation date of the elastic pool (ISO8601 format).
*
*/
public String creationDate() {
return this.creationDate;
}
/**
* @return The number of secondary replicas associated with the elastic pool that are used to provide high availability. Applicable only to Hyperscale elastic pools.
*
*/
public Optional highAvailabilityReplicaCount() {
return Optional.ofNullable(this.highAvailabilityReplicaCount);
}
/**
* @return Resource ID.
*
*/
public String id() {
return this.id;
}
/**
* @return Kind of elastic pool. This is metadata used for the Azure portal experience.
*
*/
public String kind() {
return this.kind;
}
/**
* @return The license type to apply for this elastic pool.
*
*/
public Optional licenseType() {
return Optional.ofNullable(this.licenseType);
}
/**
* @return Resource location.
*
*/
public String location() {
return this.location;
}
/**
* @return Maintenance configuration id assigned to the elastic pool. This configuration defines the period when the maintenance updates will will occur.
*
*/
public Optional maintenanceConfigurationId() {
return Optional.ofNullable(this.maintenanceConfigurationId);
}
/**
* @return The storage limit for the database elastic pool in bytes.
*
*/
public Optional maxSizeBytes() {
return Optional.ofNullable(this.maxSizeBytes);
}
/**
* @return Minimal capacity that serverless pool will not shrink below, if not paused
*
*/
public Optional minCapacity() {
return Optional.ofNullable(this.minCapacity);
}
/**
* @return Resource name.
*
*/
public String name() {
return this.name;
}
/**
* @return The per database settings for the elastic pool.
*
*/
public Optional perDatabaseSettings() {
return Optional.ofNullable(this.perDatabaseSettings);
}
/**
* @return The elastic pool SKU.
*
* The list of SKUs may vary by region and support offer. To determine the SKUs (including the SKU name, tier/edition, family, and capacity) that are available to your subscription in an Azure region, use the `Capabilities_ListByLocation` REST API or the following command:
*
*/
public Optional sku() {
return Optional.ofNullable(this.sku);
}
/**
* @return The state of the elastic pool.
*
*/
public String state() {
return this.state;
}
/**
* @return Resource tags.
*
*/
public Map tags() {
return this.tags == null ? Map.of() : this.tags;
}
/**
* @return Resource type.
*
*/
public String type() {
return this.type;
}
/**
* @return Whether or not this elastic pool is zone redundant, which means the replicas of this elastic pool will be spread across multiple availability zones.
*
*/
public Optional zoneRedundant() {
return Optional.ofNullable(this.zoneRedundant);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetElasticPoolResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String creationDate;
private @Nullable Integer highAvailabilityReplicaCount;
private String id;
private String kind;
private @Nullable String licenseType;
private String location;
private @Nullable String maintenanceConfigurationId;
private @Nullable Double maxSizeBytes;
private @Nullable Double minCapacity;
private String name;
private @Nullable ElasticPoolPerDatabaseSettingsResponse perDatabaseSettings;
private @Nullable SkuResponse sku;
private String state;
private @Nullable Map tags;
private String type;
private @Nullable Boolean zoneRedundant;
public Builder() {}
public Builder(GetElasticPoolResult defaults) {
Objects.requireNonNull(defaults);
this.creationDate = defaults.creationDate;
this.highAvailabilityReplicaCount = defaults.highAvailabilityReplicaCount;
this.id = defaults.id;
this.kind = defaults.kind;
this.licenseType = defaults.licenseType;
this.location = defaults.location;
this.maintenanceConfigurationId = defaults.maintenanceConfigurationId;
this.maxSizeBytes = defaults.maxSizeBytes;
this.minCapacity = defaults.minCapacity;
this.name = defaults.name;
this.perDatabaseSettings = defaults.perDatabaseSettings;
this.sku = defaults.sku;
this.state = defaults.state;
this.tags = defaults.tags;
this.type = defaults.type;
this.zoneRedundant = defaults.zoneRedundant;
}
@CustomType.Setter
public Builder creationDate(String creationDate) {
if (creationDate == null) {
throw new MissingRequiredPropertyException("GetElasticPoolResult", "creationDate");
}
this.creationDate = creationDate;
return this;
}
@CustomType.Setter
public Builder highAvailabilityReplicaCount(@Nullable Integer highAvailabilityReplicaCount) {
this.highAvailabilityReplicaCount = highAvailabilityReplicaCount;
return this;
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetElasticPoolResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder kind(String kind) {
if (kind == null) {
throw new MissingRequiredPropertyException("GetElasticPoolResult", "kind");
}
this.kind = kind;
return this;
}
@CustomType.Setter
public Builder licenseType(@Nullable String licenseType) {
this.licenseType = licenseType;
return this;
}
@CustomType.Setter
public Builder location(String location) {
if (location == null) {
throw new MissingRequiredPropertyException("GetElasticPoolResult", "location");
}
this.location = location;
return this;
}
@CustomType.Setter
public Builder maintenanceConfigurationId(@Nullable String maintenanceConfigurationId) {
this.maintenanceConfigurationId = maintenanceConfigurationId;
return this;
}
@CustomType.Setter
public Builder maxSizeBytes(@Nullable Double maxSizeBytes) {
this.maxSizeBytes = maxSizeBytes;
return this;
}
@CustomType.Setter
public Builder minCapacity(@Nullable Double minCapacity) {
this.minCapacity = minCapacity;
return this;
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("GetElasticPoolResult", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder perDatabaseSettings(@Nullable ElasticPoolPerDatabaseSettingsResponse perDatabaseSettings) {
this.perDatabaseSettings = perDatabaseSettings;
return this;
}
@CustomType.Setter
public Builder sku(@Nullable SkuResponse sku) {
this.sku = sku;
return this;
}
@CustomType.Setter
public Builder state(String state) {
if (state == null) {
throw new MissingRequiredPropertyException("GetElasticPoolResult", "state");
}
this.state = state;
return this;
}
@CustomType.Setter
public Builder tags(@Nullable Map tags) {
this.tags = tags;
return this;
}
@CustomType.Setter
public Builder type(String type) {
if (type == null) {
throw new MissingRequiredPropertyException("GetElasticPoolResult", "type");
}
this.type = type;
return this;
}
@CustomType.Setter
public Builder zoneRedundant(@Nullable Boolean zoneRedundant) {
this.zoneRedundant = zoneRedundant;
return this;
}
public GetElasticPoolResult build() {
final var _resultValue = new GetElasticPoolResult();
_resultValue.creationDate = creationDate;
_resultValue.highAvailabilityReplicaCount = highAvailabilityReplicaCount;
_resultValue.id = id;
_resultValue.kind = kind;
_resultValue.licenseType = licenseType;
_resultValue.location = location;
_resultValue.maintenanceConfigurationId = maintenanceConfigurationId;
_resultValue.maxSizeBytes = maxSizeBytes;
_resultValue.minCapacity = minCapacity;
_resultValue.name = name;
_resultValue.perDatabaseSettings = perDatabaseSettings;
_resultValue.sku = sku;
_resultValue.state = state;
_resultValue.tags = tags;
_resultValue.type = type;
_resultValue.zoneRedundant = zoneRedundant;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy