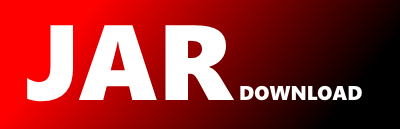
com.pulumi.azurenative.sql.outputs.GetJobStepResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.sql.outputs;
import com.pulumi.azurenative.sql.outputs.JobStepActionResponse;
import com.pulumi.azurenative.sql.outputs.JobStepExecutionOptionsResponse;
import com.pulumi.azurenative.sql.outputs.JobStepOutputResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Integer;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class GetJobStepResult {
/**
* @return The action payload of the job step.
*
*/
private JobStepActionResponse action;
/**
* @return The resource ID of the job credential that will be used to connect to the targets.
*
*/
private String credential;
/**
* @return Execution options for the job step.
*
*/
private @Nullable JobStepExecutionOptionsResponse executionOptions;
/**
* @return Resource ID.
*
*/
private String id;
/**
* @return Resource name.
*
*/
private String name;
/**
* @return Output destination properties of the job step.
*
*/
private @Nullable JobStepOutputResponse output;
/**
* @return The job step's index within the job. If not specified when creating the job step, it will be created as the last step. If not specified when updating the job step, the step id is not modified.
*
*/
private @Nullable Integer stepId;
/**
* @return The resource ID of the target group that the job step will be executed on.
*
*/
private String targetGroup;
/**
* @return Resource type.
*
*/
private String type;
private GetJobStepResult() {}
/**
* @return The action payload of the job step.
*
*/
public JobStepActionResponse action() {
return this.action;
}
/**
* @return The resource ID of the job credential that will be used to connect to the targets.
*
*/
public String credential() {
return this.credential;
}
/**
* @return Execution options for the job step.
*
*/
public Optional executionOptions() {
return Optional.ofNullable(this.executionOptions);
}
/**
* @return Resource ID.
*
*/
public String id() {
return this.id;
}
/**
* @return Resource name.
*
*/
public String name() {
return this.name;
}
/**
* @return Output destination properties of the job step.
*
*/
public Optional output() {
return Optional.ofNullable(this.output);
}
/**
* @return The job step's index within the job. If not specified when creating the job step, it will be created as the last step. If not specified when updating the job step, the step id is not modified.
*
*/
public Optional stepId() {
return Optional.ofNullable(this.stepId);
}
/**
* @return The resource ID of the target group that the job step will be executed on.
*
*/
public String targetGroup() {
return this.targetGroup;
}
/**
* @return Resource type.
*
*/
public String type() {
return this.type;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetJobStepResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private JobStepActionResponse action;
private String credential;
private @Nullable JobStepExecutionOptionsResponse executionOptions;
private String id;
private String name;
private @Nullable JobStepOutputResponse output;
private @Nullable Integer stepId;
private String targetGroup;
private String type;
public Builder() {}
public Builder(GetJobStepResult defaults) {
Objects.requireNonNull(defaults);
this.action = defaults.action;
this.credential = defaults.credential;
this.executionOptions = defaults.executionOptions;
this.id = defaults.id;
this.name = defaults.name;
this.output = defaults.output;
this.stepId = defaults.stepId;
this.targetGroup = defaults.targetGroup;
this.type = defaults.type;
}
@CustomType.Setter
public Builder action(JobStepActionResponse action) {
if (action == null) {
throw new MissingRequiredPropertyException("GetJobStepResult", "action");
}
this.action = action;
return this;
}
@CustomType.Setter
public Builder credential(String credential) {
if (credential == null) {
throw new MissingRequiredPropertyException("GetJobStepResult", "credential");
}
this.credential = credential;
return this;
}
@CustomType.Setter
public Builder executionOptions(@Nullable JobStepExecutionOptionsResponse executionOptions) {
this.executionOptions = executionOptions;
return this;
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetJobStepResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("GetJobStepResult", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder output(@Nullable JobStepOutputResponse output) {
this.output = output;
return this;
}
@CustomType.Setter
public Builder stepId(@Nullable Integer stepId) {
this.stepId = stepId;
return this;
}
@CustomType.Setter
public Builder targetGroup(String targetGroup) {
if (targetGroup == null) {
throw new MissingRequiredPropertyException("GetJobStepResult", "targetGroup");
}
this.targetGroup = targetGroup;
return this;
}
@CustomType.Setter
public Builder type(String type) {
if (type == null) {
throw new MissingRequiredPropertyException("GetJobStepResult", "type");
}
this.type = type;
return this;
}
public GetJobStepResult build() {
final var _resultValue = new GetJobStepResult();
_resultValue.action = action;
_resultValue.credential = credential;
_resultValue.executionOptions = executionOptions;
_resultValue.id = id;
_resultValue.name = name;
_resultValue.output = output;
_resultValue.stepId = stepId;
_resultValue.targetGroup = targetGroup;
_resultValue.type = type;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy