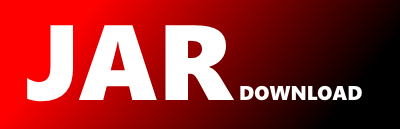
com.pulumi.azurenative.sql.outputs.GetServerAdvisorResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.sql.outputs;
import com.pulumi.azurenative.sql.outputs.RecommendedActionResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Objects;
@CustomType
public final class GetServerAdvisorResult {
/**
* @return Gets the status of availability of this advisor to customers. Possible values are 'GA', 'PublicPreview', 'LimitedPublicPreview' and 'PrivatePreview'.
*
*/
private String advisorStatus;
/**
* @return Gets the auto-execute status (whether to let the system execute the recommendations) of this advisor. Possible values are 'Enabled' and 'Disabled'
*
*/
private String autoExecuteStatus;
/**
* @return Gets the resource from which current value of auto-execute status is inherited. Auto-execute status can be set on (and inherited from) different levels in the resource hierarchy. Possible values are 'Subscription', 'Server', 'ElasticPool', 'Database' and 'Default' (when status is not explicitly set on any level).
*
*/
private String autoExecuteStatusInheritedFrom;
/**
* @return Resource ID.
*
*/
private String id;
/**
* @return Resource kind.
*
*/
private String kind;
/**
* @return Gets the time when the current resource was analyzed for recommendations by this advisor.
*
*/
private String lastChecked;
/**
* @return Resource location.
*
*/
private String location;
/**
* @return Resource name.
*
*/
private String name;
/**
* @return Gets that status of recommendations for this advisor and reason for not having any recommendations. Possible values include, but are not limited to, 'Ok' (Recommendations available),LowActivity (not enough workload to analyze), 'DbSeemsTuned' (Database is doing well), etc.
*
*/
private String recommendationsStatus;
/**
* @return Gets the recommended actions for this advisor.
*
*/
private List recommendedActions;
/**
* @return Resource type.
*
*/
private String type;
private GetServerAdvisorResult() {}
/**
* @return Gets the status of availability of this advisor to customers. Possible values are 'GA', 'PublicPreview', 'LimitedPublicPreview' and 'PrivatePreview'.
*
*/
public String advisorStatus() {
return this.advisorStatus;
}
/**
* @return Gets the auto-execute status (whether to let the system execute the recommendations) of this advisor. Possible values are 'Enabled' and 'Disabled'
*
*/
public String autoExecuteStatus() {
return this.autoExecuteStatus;
}
/**
* @return Gets the resource from which current value of auto-execute status is inherited. Auto-execute status can be set on (and inherited from) different levels in the resource hierarchy. Possible values are 'Subscription', 'Server', 'ElasticPool', 'Database' and 'Default' (when status is not explicitly set on any level).
*
*/
public String autoExecuteStatusInheritedFrom() {
return this.autoExecuteStatusInheritedFrom;
}
/**
* @return Resource ID.
*
*/
public String id() {
return this.id;
}
/**
* @return Resource kind.
*
*/
public String kind() {
return this.kind;
}
/**
* @return Gets the time when the current resource was analyzed for recommendations by this advisor.
*
*/
public String lastChecked() {
return this.lastChecked;
}
/**
* @return Resource location.
*
*/
public String location() {
return this.location;
}
/**
* @return Resource name.
*
*/
public String name() {
return this.name;
}
/**
* @return Gets that status of recommendations for this advisor and reason for not having any recommendations. Possible values include, but are not limited to, 'Ok' (Recommendations available),LowActivity (not enough workload to analyze), 'DbSeemsTuned' (Database is doing well), etc.
*
*/
public String recommendationsStatus() {
return this.recommendationsStatus;
}
/**
* @return Gets the recommended actions for this advisor.
*
*/
public List recommendedActions() {
return this.recommendedActions;
}
/**
* @return Resource type.
*
*/
public String type() {
return this.type;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetServerAdvisorResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String advisorStatus;
private String autoExecuteStatus;
private String autoExecuteStatusInheritedFrom;
private String id;
private String kind;
private String lastChecked;
private String location;
private String name;
private String recommendationsStatus;
private List recommendedActions;
private String type;
public Builder() {}
public Builder(GetServerAdvisorResult defaults) {
Objects.requireNonNull(defaults);
this.advisorStatus = defaults.advisorStatus;
this.autoExecuteStatus = defaults.autoExecuteStatus;
this.autoExecuteStatusInheritedFrom = defaults.autoExecuteStatusInheritedFrom;
this.id = defaults.id;
this.kind = defaults.kind;
this.lastChecked = defaults.lastChecked;
this.location = defaults.location;
this.name = defaults.name;
this.recommendationsStatus = defaults.recommendationsStatus;
this.recommendedActions = defaults.recommendedActions;
this.type = defaults.type;
}
@CustomType.Setter
public Builder advisorStatus(String advisorStatus) {
if (advisorStatus == null) {
throw new MissingRequiredPropertyException("GetServerAdvisorResult", "advisorStatus");
}
this.advisorStatus = advisorStatus;
return this;
}
@CustomType.Setter
public Builder autoExecuteStatus(String autoExecuteStatus) {
if (autoExecuteStatus == null) {
throw new MissingRequiredPropertyException("GetServerAdvisorResult", "autoExecuteStatus");
}
this.autoExecuteStatus = autoExecuteStatus;
return this;
}
@CustomType.Setter
public Builder autoExecuteStatusInheritedFrom(String autoExecuteStatusInheritedFrom) {
if (autoExecuteStatusInheritedFrom == null) {
throw new MissingRequiredPropertyException("GetServerAdvisorResult", "autoExecuteStatusInheritedFrom");
}
this.autoExecuteStatusInheritedFrom = autoExecuteStatusInheritedFrom;
return this;
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetServerAdvisorResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder kind(String kind) {
if (kind == null) {
throw new MissingRequiredPropertyException("GetServerAdvisorResult", "kind");
}
this.kind = kind;
return this;
}
@CustomType.Setter
public Builder lastChecked(String lastChecked) {
if (lastChecked == null) {
throw new MissingRequiredPropertyException("GetServerAdvisorResult", "lastChecked");
}
this.lastChecked = lastChecked;
return this;
}
@CustomType.Setter
public Builder location(String location) {
if (location == null) {
throw new MissingRequiredPropertyException("GetServerAdvisorResult", "location");
}
this.location = location;
return this;
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("GetServerAdvisorResult", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder recommendationsStatus(String recommendationsStatus) {
if (recommendationsStatus == null) {
throw new MissingRequiredPropertyException("GetServerAdvisorResult", "recommendationsStatus");
}
this.recommendationsStatus = recommendationsStatus;
return this;
}
@CustomType.Setter
public Builder recommendedActions(List recommendedActions) {
if (recommendedActions == null) {
throw new MissingRequiredPropertyException("GetServerAdvisorResult", "recommendedActions");
}
this.recommendedActions = recommendedActions;
return this;
}
public Builder recommendedActions(RecommendedActionResponse... recommendedActions) {
return recommendedActions(List.of(recommendedActions));
}
@CustomType.Setter
public Builder type(String type) {
if (type == null) {
throw new MissingRequiredPropertyException("GetServerAdvisorResult", "type");
}
this.type = type;
return this;
}
public GetServerAdvisorResult build() {
final var _resultValue = new GetServerAdvisorResult();
_resultValue.advisorStatus = advisorStatus;
_resultValue.autoExecuteStatus = autoExecuteStatus;
_resultValue.autoExecuteStatusInheritedFrom = autoExecuteStatusInheritedFrom;
_resultValue.id = id;
_resultValue.kind = kind;
_resultValue.lastChecked = lastChecked;
_resultValue.location = location;
_resultValue.name = name;
_resultValue.recommendationsStatus = recommendationsStatus;
_resultValue.recommendedActions = recommendedActions;
_resultValue.type = type;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy